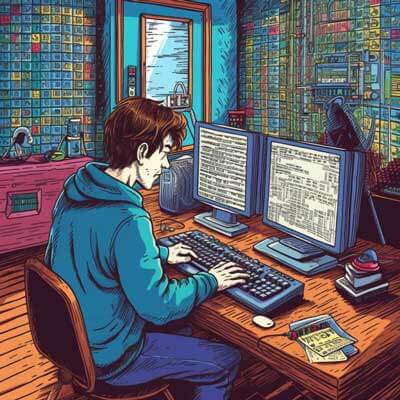
- Step 1: Import the Image File
- Step 2: Use the Image in JSX
- Step 3: Handling Different Image Formats
- Step 4: Organizing Image Files
- Step 5: Using Images from External Sources
- Step 6: Using Images with CSS
- Step 7: Using Images with Inline Styles
- Step 8: Optimizing Image Loading
- Step 9: Adding Alternative Text
To reference a local image in React, you can follow these steps:
Step 1: Import the Image File
First, you need to import the image file into your React component. You can do this by using the import
statement and specifying the path to the image file. For example, if your image file is located in the same directory as your component file, you can import it like this:
import Image from './image.jpg';
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Step 2: Use the Image in JSX
Once you have imported the image file, you can use it in your JSX code by referencing it as a component. You can use the img
tag and set the src
attribute to the imported image component. For example:
import React from 'react'; import Image from './image.jpg'; function App() { return ( <div> <img src="{Image}" alt="My Image" /> </div> ); } export default App;
In the above example, we import the image file image.jpg
and use it in the img
tag within the App
component. The src
attribute is set to the imported image component, and the alt
attribute is used to provide alternative text for the image.
Step 3: Handling Different Image Formats
React supports various image formats such as JPEG, PNG, GIF, and SVG. You can import and use these image formats in the same way as shown in the previous step. Just make sure to specify the correct file extension when importing the image file.
Step 4: Organizing Image Files
When working with multiple image files in a React project, it is a good practice to organize them in a separate folder. You can create a folder named images
or assets
in the root directory of your project and store all the image files inside it. This helps in keeping your project structure clean and makes it easier to locate and manage the image files.
Related Article: How to Use the forEach Loop with JavaScript
Step 5: Using Images from External Sources
Apart from referencing local images, you can also use images from external sources such as URLs. To use an image from an external source, you can directly set the src
attribute of the img
tag to the URL of the image. For example:
import React from 'react'; function App() { return ( <div> <img src="https://example.com/image.jpg" alt="External Image" /> </div> ); } export default App;
In the above example, we set the src
attribute to the URL of the image https://example.com/image.jpg
. This will load the image from the specified URL when the component is rendered.
Step 6: Using Images with CSS
You can also apply CSS styles to the images in your React components. To do this, you can use the className
attribute to assign a CSS class to the img
tag and define the styles in a separate CSS file. Here’s an example:
import React from 'react'; import './App.css'; import Image from './image.jpg'; function App() { return ( <div> <img src="{Image}" alt="My Image" /> </div> ); } export default App;
In the above example, we import the CSS file App.css
and apply the image
class to the img
tag. You can define the styles for the image
class in the CSS file as per your requirements.
Step 7: Using Images with Inline Styles
Alternatively, you can also apply inline styles to the img
tag using the style
attribute. This allows you to specify the styles directly as JavaScript objects. Here’s an example:
import React from 'react'; import Image from './image.jpg'; function App() { const imageStyle = { width: '200px', height: '200px', borderRadius: '50%', }; return ( <div> <img src="{Image}" alt="My Image" /> </div> ); } export default App;
In the above example, we define the imageStyle
object with the desired styles such as width, height, and border radius. We then pass this object as the value of the style
attribute in the img
tag.
Related Article: How to Use Javascript Substring, Splice, and Slice
Step 8: Optimizing Image Loading
When working with large images, it is important to optimize their loading to ensure a smooth user experience. You can use techniques like lazy loading and image compression to improve the performance of your React application. There are also libraries available, such as react-lazy-load-image-component
, that provide additional features for lazy loading images.
Step 9: Adding Alternative Text
It is essential to provide alternative text for images, especially for accessibility purposes. The alt
attribute in the img
tag is used to provide a description of the image for screen readers and in case the image fails to load. Make sure to include descriptive and meaningful alternative text for images to improve the accessibility of your React application.