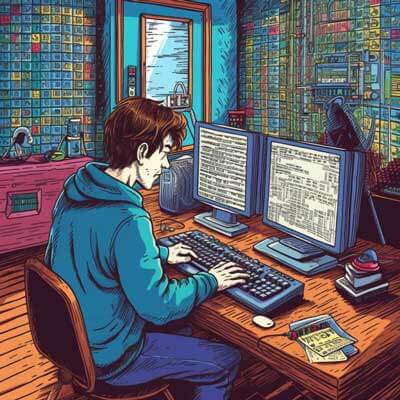
Comparing Strings in Java
In Java, you can compare strings using several different methods. Here are two common approaches:
Related Article: How to Convert JsonString to JsonObject in Java
1. Using the equals() method
The most straightforward way to compare strings in Java is by using the equals()
method. This method compares the content of two strings and returns true
if they are equal, and false
otherwise. Here’s an example:
String str1 = "Hello"; String str2 = "World"; if (str1.equals(str2)) { System.out.println("The strings are equal"); } else { System.out.println("The strings are not equal"); }
In this example, the output will be “The strings are not equal” since the content of str1
and str2
is different.
It’s important to note that the equals()
method performs a case-sensitive comparison. If you want to perform a case-insensitive comparison, you can use the equalsIgnoreCase()
method instead.
2. Using the compareTo() method
Another way to compare strings in Java is by using the compareTo()
method. This method compares two strings lexicographically and returns an integer value based on the comparison result. The returned value indicates whether the first string is less than, equal to, or greater than the second string. Here’s an example:
String str1 = "Apple"; String str2 = "Banana"; int result = str1.compareTo(str2); if (result 0) { System.out.println("str1 is greater than str2"); } else { System.out.println("str1 is equal to str2"); }
In this example, the output will be “str1 is less than str2” since “Apple” comes before “Banana” in lexicographical order.
It’s important to note that the compareTo()
method is case-sensitive as well. If you want to perform a case-insensitive comparison, you can convert the strings to lowercase or uppercase using the toLowerCase()
or toUpperCase()
methods before calling compareTo()
.
Suggestions and Best Practices
– When comparing strings in Java, it’s generally recommended to use the equals()
method for content comparison and the compareTo()
method for lexicographical comparison. However, keep in mind that the choice between these two methods depends on your specific use case.
– If you want to perform a case-insensitive comparison, consider using the equalsIgnoreCase()
method or converting the strings to lowercase or uppercase before comparison.
– To handle null values, make sure to check for null before calling any string comparison method to avoid NullPointerException
errors. You can use the Objects.equals()
method to handle null values safely.
Overall, comparing strings in Java is a fundamental operation that you will frequently encounter in your programming journey. By understanding the available methods and their differences, you can effectively compare strings and make informed decisions based on your specific requirements.
Related Article: How to Implement a Delay in Java Using java wait seconds