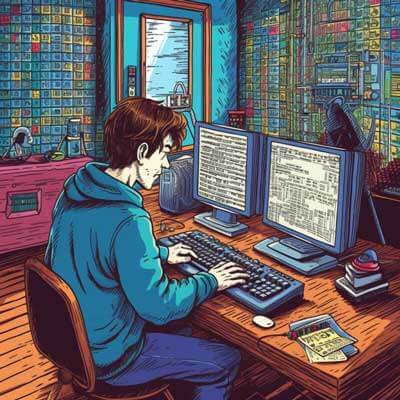
Table of Contents
To format a JavaScript date as YYYY-MM-DD, you can use various methods available in the JavaScript Date object. Here are two possible approaches:
Approach 1: Using the toISOString() method
The toISOString() method returns a string representing the date in the ISO format (YYYY-MM-DDTHH:mm:ss.sssZ). By manipulating this string, we can extract the YYYY MM DD format.
const date = new Date(); const formattedDate = date.toISOString().split('T')[0].replace(/-/g, ' '); console.log(formattedDate);
In this approach, we create a new Date object and store it in the date
variable. Then, we use the toISOString() method to get the ISO format string representation of the date. We split the string at the 'T' character to extract the date part. Finally, we replace the '-' characters with spaces using the replace() method to get the desired YYYY MM DD format.
Related Article: How to Use Async Await with a Foreach Loop in JavaScript
Approach 2: Using the getFullYear(), getMonth(), and getDate() methods
Another approach is to use the getFullYear(), getMonth(), and getDate() methods provided by the JavaScript Date object to extract the year, month, and day components respectively. We can then concatenate them with the desired formatting.
const date = new Date(); const year = date.getFullYear(); const month = String(date.getMonth() + 1).padStart(2, '0'); const day = String(date.getDate()).padStart(2, '0'); const formattedDate = `${year} ${month} ${day}`; console.log(formattedDate);
In this approach, we create a new Date object and store it in the date
variable. We use the getFullYear() method to get the full year, getMonth() method to get the month (adding 1 because months in JavaScript are zero-based), and getDate() method to get the day. The padStart() method is used to ensure that the month and day have leading zeros if necessary. Finally, we concatenate the year, month, and day with spaces to get the desired YYYY MM DD format.
Alternative Ideas
- If you are using a library or framework like Moment.js, you can easily format a JavaScript date using its formatting functions. Moment.js provides a wide range of formatting options, including the ability to format a date as YYYY MM DD. You can refer to the Moment.js documentation for more information on how to achieve this.
Best Practices
- When working with dates in JavaScript, it is important to keep in mind that the getMonth() method returns zero-based values (0 for January, 11 for December). If you need to display the month with the correct numeric value, make sure to add 1 to it.
- It is recommended to always handle time zones properly when working with dates. The JavaScript Date object uses the local time zone by default, so if you need to work with dates in a specific time zone, consider using a library like Moment.js or a dedicated date manipulation library.
Related Article: How to Choose the Correct Json Date Format in Javascript
Code Snippet: Formatting Current Date as YYYY MM DD in Node.js
const date = new Date(); const year = date.getFullYear(); const month = String(date.getMonth() + 1).padStart(2, '0'); const day = String(date.getDate()).padStart(2, '0'); const formattedDate = `${year} ${month} ${day}`; console.log(formattedDate);
Save the above code in a JavaScript file, for example, formatDate.js
, and run it using Node.js:
node formatDate.js
This will output the current date in the YYYY MM DD format.