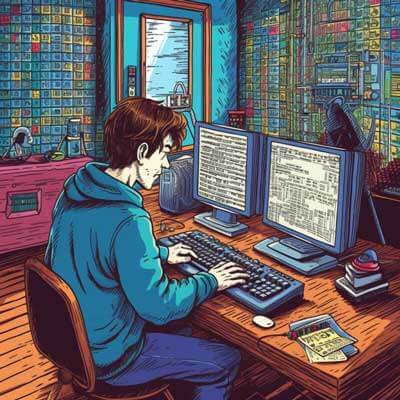
Table of Contents
Adding a class to a given element in JavaScript is a common task when working with DOM manipulation. It allows you to dynamically apply styles or behavior to specific elements on a webpage. In this answer, we will explore two possible ways to add a class to a given element in JavaScript.
Method 1: Using the classList property
One way to add a class to a given element is by using the classList
property. The classList
property provides methods to manipulate the class attribute of an element.
Here's an example of how you can add a class to an element using the classList.add()
method:
const element = document.getElementById('myElement'); element.classList.add('myClass');
In the above example, we first retrieve the element with the ID "myElement" using document.getElementById()
. Then, we use the classList.add()
method to add the class "myClass" to the element.
Related Article: How to Generate a GUID/UUID in JavaScript
Method 2: Using the className property
Another way to add a class to a given element is by using the className
property. The className
property allows you to get or set the value of the class attribute of an element.
Here's an example of how you can add a class to an element using the className
property:
const element = document.getElementById('myElement'); element.className += ' myClass';
In the above example, we first retrieve the element with the ID "myElement" using document.getElementById()
. Then, we concatenate the existing class name with the new class name "myClass" and assign it back to the className
property.
Why the question was asked in the first place and the potential reasons
The question of how to add a class to a given element in JavaScript is commonly asked by developers who are working on web development projects. There are several potential reasons why someone might need to add a class to a specific element:
1. Dynamic styling: By adding or removing classes, you can dynamically change the appearance of elements based on user interactions, events, or other conditions. This allows for more interactive and responsive web interfaces.
2. Behavior modification: Adding or removing classes can also be used to modify the behavior of elements. For example, you can use classes to toggle the visibility of certain elements or enable/disable certain functionality.
3. CSS animations and transitions: Classes are often used in conjunction with CSS animations and transitions to create visually appealing effects on webpages. By adding or removing classes, you can trigger animations or transitions defined in CSS.
Suggestions and alternative ideas
While the above methods provide a straightforward way to add a class to a given element in JavaScript, there are some alternative ideas and suggestions to consider:
1. Using a JavaScript library/framework: If you are working on a complex web application, you might consider using a JavaScript library or framework like React, Vue.js, or Angular. These frameworks provide more advanced ways to manage class names and styles, such as using state-based class toggling or scoped CSS.
2. CSS-in-JS: Another alternative is to use CSS-in-JS libraries like styled-components or Emotion. These libraries allow you to write CSS styles directly in your JavaScript code and dynamically apply them to elements. This can provide more flexibility and maintainability, especially in larger projects.
3. Utilizing a utility library: There are utility libraries like lodash or Underscore.js that provide helper functions for manipulating class names and styles. These libraries can simplify the process of adding, removing, or toggling classes on elements.
Related Article: How To Validate An Email Address In Javascript
Best Practices
When adding a class to a given element in JavaScript, it is important to follow some best practices to ensure clean and maintainable code:
1. Use descriptive class names: Choose class names that clearly describe the purpose or behavior of the element. This makes it easier for other developers to understand and work with your code.
2. Separate concerns: Avoid mixing JavaScript code with CSS styles. Instead, use CSS classes to define styles and use JavaScript to add or remove those classes as needed.
3. Consider performance implications: Adding or removing classes can trigger reflows and repaints in the browser, which can impact performance, especially when dealing with large numbers of elements. If performance is a concern, consider using more optimized approaches like CSS transitions or animations.
4. Use event delegation: If you have multiple elements that need to have a class added or removed based on a certain event, consider using event delegation. Event delegation allows you to attach event listeners to a parent element and handle events for its child elements. This can reduce the number of event listeners and improve performance.