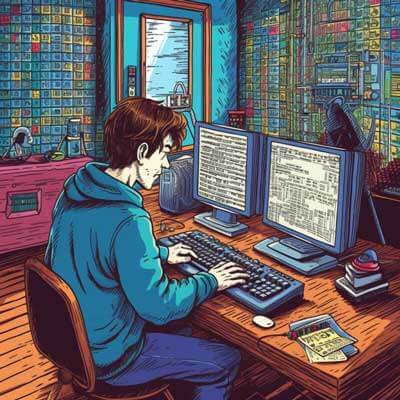
Table of Contents
Server-Side Rendering (SSR) in Next.js
Server-side rendering (SSR) is a crucial aspect of modern web development. It allows web pages to be rendered on the server and sent as fully-formed HTML to the client. This approach offers several benefits, such as improved performance, better search engine optimization (SEO), and enhanced user experience. Next.js, a popular framework built on top of React, provides excellent support for server-side rendering.
Next.js also offers a great developer experience by providing a seamless integration with React. Developers can use familiar React components and concepts, such as state management and lifecycle methods, while benefiting from server-side rendering capabilities. This allows for code reuse and a smoother development process.
Related Article: How to Work with Big Data using JavaScript
Example 1: Server-side rendering with Next.js
To illustrate how Next.js handles server-side rendering, let's consider a simple example. Suppose we have a page component called HomePage
that fetches some data from an API and displays it on the page. In a traditional React setup, this data fetching would happen on the client-side, after the initial page load. However, with Next.js, we can leverage server-side rendering to fetch the data on the server and send it as part of the initial HTML response.
// pages/HomePage.js import React from 'react'; const HomePage = ({ data }) => { return ( <div> <h1>Welcome to my website!</h1> <p>{data}</p> </div> ); }; export async function getServerSideProps() { // Fetch data from an API const response = await fetch('https://api.example.com/data'); const data = await response.json(); // Pass the fetched data as props to the component return { props: { data, }, }; } export default HomePage;
In this example, the getServerSideProps
function is a special function provided by Next.js. It runs on the server and fetches the data from the API before rendering the HomePage
component. The fetched data is then passed as props to the component, ensuring that it is available during the initial render.
Example 2: Client-side rendering fallback
Next.js also provides the flexibility to fallback to client-side rendering for certain parts of the page that don't require server-side rendering. This can be useful for components that rely on user interactions or dynamic data that is not available during the initial server-side render.
// pages/HomePage.js import React, { useState, useEffect } from 'react'; const HomePage = () => { const [dynamicData, setDynamicData] = useState(null); useEffect(() => { // Fetch dynamic data on the client-side fetch('https://api.example.com/dynamic-data') .then(response => response.json()) .then(data => setDynamicData(data)); }, []); return ( <div> <h1>Welcome to my website!</h1> {dynamicData && <p>{dynamicData}</p>} </div> ); }; export default HomePage;
In this example, the dynamicData
state is initialized as null
, and the useEffect
hook is used to fetch the dynamic data on the client-side. Once the data is fetched, it is stored in the dynamicData
state, triggering a re-render of the component. This allows for a smooth user experience, as the dynamic content is loaded after the initial render.
Next.js and Its Benefits for Frontend Development
Next.js is a useful framework that simplifies frontend development by providing built-in support for server-side rendering, static site generation, and other advanced features. It is built on top of React and offers a seamless integration with the popular JavaScript library.
Related Article: How to Autofill a Textarea Element with VueJS
Example 1: Creating a new Next.js project
To get started with Next.js, you can use the create-next-app
command-line tool, which sets up a new Next.js project with all the necessary dependencies and configuration files.
npx create-next-app my-app
This command will create a new directory called my-app
, containing the initial Next.js project structure.
Example 2: Creating a new page in Next.js
Next.js follows a file-based routing system, where each page is represented by a separate file in the pages
directory. To create a new page, simply create a new file with the desired name in the pages
directory.
// pages/About.js import React from 'react'; const About = () => { return ( <div> <h1>About Us</h1> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p> </div> ); }; export default About;
In this example, we create a new page component called About
. This component renders a simple heading and paragraph. When navigating to /about
in the browser, Next.js will automatically render this component and serve it as part of the HTML response.
How Next.js Handles Routing
Next.js provides a simple and intuitive routing system that allows developers to define routes using file-based routing. This means that each page component corresponds to a specific route, based on the file name and location.
Next.js follows a convention where each file inside the pages
directory represents a route. For example, a file named about.js
inside the pages
directory will correspond to the /about
route.
Example 1: Defining routes in Next.js
To define a route in Next.js, simply create a new file in the pages
directory with the desired route name. The file name must be in lowercase and can include dynamic segments denoted by square brackets.
// pages/posts/[slug].js import React from 'react'; import { useRouter } from 'next/router'; const Post = () => { const router = useRouter(); const { slug } = router.query; return ( <div> <h1>Post: {slug}</h1> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p> </div> ); }; export default Post;
In this example, we create a dynamic route for blog posts using the file name [slug].js
. The slug
parameter can be accessed using the useRouter
hook provided by Next.js. When navigating to /posts/hello-world
, the Post
component will be rendered with the slug
parameter set to hello-world
.
Related Article: How To Remove Duplicates From A Javascript Array
Example 2: Nested routes in Next.js
Next.js also supports nested routes, allowing for more complex routing structures. Nested routes can be defined by creating subdirectories under the pages
directory.
// pages/blog/index.js import React from 'react'; const Blog = () => { return ( <div> <h1>Blog</h1> <p>Welcome to our blog!</p> </div> ); }; export default Blog;
In this example, we create a nested route by creating a subdirectory called blog
under the pages
directory. Inside the blog
directory, we create an index.js
file that represents the /blog
route. Navigating to /blog
in the browser will render the Blog
component.
Using Next.js with React
Next.js is built on top of React and provides seamless integration with the popular JavaScript library. This allows developers to leverage the power of React components, state management, and other features while benefiting from the server-side rendering capabilities provided by Next.js.
Example 1: Creating a React component in Next.js
Creating a React component in Next.js is similar to creating a component in a traditional React setup. Simply define a new function or class component and export it.
// components/Hello.js import React from 'react'; const Hello = () => { return <h1>Hello, Next.js!</h1>; }; export default Hello;
In this example, we create a simple functional component called Hello
, which renders a heading with the text "Hello, Next.js!". This component can be imported and used in any Next.js page component.
Example 2: Using state in a Next.js component
Next.js components can leverage the state management capabilities provided by React. This allows for dynamic and interactive user interfaces.
// components/Counter.js import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <h1>Counter: {count}</h1> <button>Increment</button> </div> ); }; export default Counter;
In this example, we create a simple counter component that uses the useState
hook to manage the state. The count
state variable is initialized with a value of 0, and the setCount
function is used to update the count. When the "Increment" button is clicked, the increment
function is called, updating the count and triggering a re-render of the component.
Related Article: How to Get Selected Option From Dropdown in JQuery
Common Technologies Used with Next.js
Next.js is a versatile framework that can be used with a wide range of technologies and tools. Here are some common technologies that are often used in conjunction with Next.js.
Example 1: Styling with CSS-in-JS
Next.js provides built-in support for styling components using various CSS-in-JS solutions such as styled-components and emotion.
npm install styled-components
// components/StyledButton.js import styled from 'styled-components'; const StyledButton = styled.button` background-color: #0070f3; color: white; padding: 10px 20px; border: none; border-radius: 4px; cursor: pointer; `; export default StyledButton;
In this example, we use the styled-components library to create a custom styled button component. The styles are defined using a tagged template literal syntax, allowing us to write CSS directly inside the JavaScript code. The resulting component can be used in any Next.js page component.
Example 2: Data fetching with Axios
Next.js provides flexibility when it comes to fetching data from external APIs or databases. The axios library is a popular choice for making HTTP requests in JavaScript applications.
npm install axios
// components/UserList.js import React, { useState, useEffect } from 'react'; import axios from 'axios'; const UserList = () => { const [users, setUsers] = useState([]); useEffect(() => { axios.get('https://api.example.com/users') .then(response => setUsers(response.data)) .catch(error => console.error(error)); }, []); return ( <div> <h1>User List</h1> <ul> {users.map(user => ( <li>{user.name}</li> ))} </ul> </div> ); }; export default UserList;
In this example, we use the axios library to fetch a list of users from an external API. The useEffect
hook is used to perform the data fetching when the component mounts. The fetched data is stored in the users
state variable and rendered as a list of user names.
Retrieving the URL in Next.js on the Server-Side Component
In some cases, you may need to retrieve the URL of the current page in a Next.js server-side component. This can be useful for various purposes, such as generating dynamic content or handling redirects.
Next.js provides several ways to retrieve the URL in a server-side component, depending on the specific use case.
Related Article: How To Format A Date In Javascript
Example 1: Retrieving the URL using the req
object
When handling server-side rendering with Next.js, you can access the current request object (req
) in the getServerSideProps
function. The req
object contains various properties and methods, including the URL of the current page.
// pages/Example.js const Example = () => { // ... }; export async function getServerSideProps({ req }) { const currentUrl = req.url; // Access the current URL and pass it as props to the component return { props: { currentUrl, }, }; } export default Example;
In this example, we retrieve the current URL by accessing the url
property of the req
object passed as an argument to the getServerSideProps
function. The retrieved URL is then passed as props to the component.
Example 2: Retrieving the URL using the useRouter
hook
Next.js provides the useRouter
hook, which can be used to access the current URL in a server-side component or a client-side component.
// pages/Example.js import { useRouter } from 'next/router'; const Example = () => { const router = useRouter(); const currentUrl = router.asPath; // ... }; export default Example;
In this example, we import the useRouter
hook from the next/router
module and initialize the router
object. We can then access the current URL using the asPath
property of the router
object.
Setting Up Server-Side Rendering with Next.js
Setting up server-side rendering with Next.js is straightforward and requires minimal configuration. Next.js provides a built-in functionality called getServerSideProps
, which allows you to fetch data on the server-side before rendering a page.
Example 1: Using getServerSideProps
to fetch data
To fetch data on the server-side in Next.js, you can define a special function called getServerSideProps
inside your page component. This function runs on the server before rendering the page and can fetch data from an external API or perform any other server-side operations.
// pages/Example.js import axios from 'axios'; const Example = ({ data }) => { // Render the page using the fetched data }; export async function getServerSideProps() { const response = await axios.get('https://api.example.com/data'); const data = response.data; return { props: { data, }, }; } export default Example;
In this example, we define the getServerSideProps
function, which fetches data from an external API using the axios library. The fetched data is then passed as props to the component, allowing it to be accessed during the initial render.
Related Article: How to Check for String Equality in Javascript
Example 2: Using getServerSideProps
to handle redirects
Next.js also allows you to handle redirects on the server-side using the getServerSideProps
function. This can be useful for scenarios where you need to perform authentication or authorization checks before rendering the page.
// pages/Example.js const Example = () => { // Render the page }; export async function getServerSideProps({ req, res }) { const isAuthenticated = // Perform authentication check if (!isAuthenticated) { res.writeHead(302, { Location: '/login', }); res.end(); } return { props: {}, }; } export default Example;
In this example, we perform an authentication check inside the getServerSideProps
function. If the user is not authenticated, we use the res
object to set the response status code to 302 (indicating a temporary redirect) and specify the location of the login page. The user will then be redirected to the login page.
Limitations and Drawbacks of Using Next.js for Server-Side Rendering
While Next.js provides useful server-side rendering capabilities, there are some limitations and drawbacks to consider when using it in your projects.
Example 1: Increased server load
Server-side rendering can put additional load on the server, as each request needs to be processed and rendered on the server before being sent to the client. This can impact the scalability and performance of your application, especially under heavy traffic.
Example 2: Limited client-side interactivity
Server-side rendering is primarily focused on generating and sending fully-rendered HTML to the client. This means that client-side interactivity, such as dynamic updates or user interactions, may be limited compared to client-side rendering frameworks.
Related Article: How to Use 'This' Keyword in jQuery
Optimizing Server-Side Rendering Performance in Next.js
To optimize server-side rendering performance in Next.js, you can follow several best practices and techniques.
Example 1: Caching rendered pages
Next.js provides built-in support for server-side rendering caching, allowing you to cache the rendered HTML output of your pages. This can significantly improve performance by reducing the load on the server and speeding up subsequent page loads.
// pages/Example.js export async function getServerSideProps({ req, res }) { res.setHeader('Cache-Control', 's-maxage=1, stale-while-revalidate'); // ... }
In this example, we set the Cache-Control
header to enable caching with a maximum age of 1 second (s-maxage=1
). This tells the client and any intermediate caches to cache the rendered page for 1 second. During this time, subsequent requests for the same page will be served from the cache, reducing the load on the server.
Example 2: Prefetching data on the client-side
To improve the perceived performance of your Next.js application, you can prefetch data on the client-side. This allows you to fetch data in advance, so it's already available when the user navigates to a new page.
// components/UserList.js import React, { useState, useEffect } from 'react'; import axios from 'axios'; const UserList = () => { const [users, setUsers] = useState([]); useEffect(() => { axios.get('https://api.example.com/users') .then(response => setUsers(response.data)) .catch(error => console.error(error)); }, []); return ( <div> <h1>User List</h1> <ul> {users.map(user => ( <li>{user.name}</li> ))} </ul> </div> ); }; export default UserList;
In this example, we use the useEffect
hook to fetch the list of users from an external API. By fetching the data on the client-side, we can reduce the initial server-side rendering time and improve the perceived performance of the page.