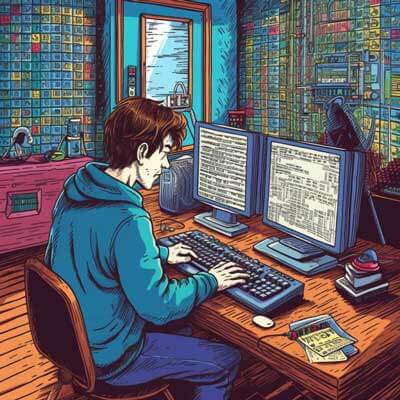
- How can I call an Angular component function from JavaScript?
- What is the syntax to invoke an Angular component function from JavaScript?
- How do I execute an Angular component function from JavaScript?
- Is it possible to trigger an Angular component function from JavaScript?
- What are the different ways to call an Angular component function from JavaScript?
- Can I dispatch an Angular component function from JavaScript?
- How can I emit an Angular component function from JavaScript?
- Is there a way to fire an Angular component function from JavaScript?
- What is the recommended approach to call an Angular component function from JavaScript?
- Are there any limitations or restrictions when calling an Angular component function from JavaScript?
How can I call an Angular component function from JavaScript?
To call an Angular component function from JavaScript, you need to understand the component’s lifecycle and how to access it from JavaScript code. Angular provides several mechanisms to interact with components, such as ViewChild, ElementRef, and EventEmitter.
One common approach is to use the ViewChild decorator to get a reference to the component in your JavaScript code. ViewChild allows you to access a component instance and its public methods and properties. Here’s an example:
import { Component, ViewChild } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @ViewChild('myComponent') myComponent: MyComponent; callComponentFunction() { this.myComponent.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, we have an AppComponent that contains a button. When the button is clicked, it calls the callComponentFunction method, which in turn invokes the componentFunction method of the MyComponent instance.
Here’s the HTML template for the AppComponent:
<h1>Hello, Angular!</h1> <button>Call Component Function</button>
And here’s the code for the MyComponent:
@Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, we use the ViewChild decorator to get a reference to the MyComponent instance in the AppComponent. We then call the componentFunction method on the MyComponent instance to execute the desired functionality.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
What is the syntax to invoke an Angular component function from JavaScript?
The syntax to invoke an Angular component function from JavaScript depends on how you access the component instance. If you use the ViewChild decorator, you can directly call the component’s methods using the instance variable.
Here’s an example:
import { Component, ViewChild } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @ViewChild('myComponent') myComponent: MyComponent; callComponentFunction() { this.myComponent.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent calls the componentFunction method of the MyComponent instance using the syntax this.myComponent.componentFunction()
.
Alternatively, you can also use ElementRef to access the DOM element representing the component and then call the component’s methods using the nativeElement property.
Here’s an example:
import { Component, ElementRef } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { constructor(private elementRef: ElementRef) {} callComponentFunction() { const myComponentElement = this.elementRef.nativeElement.querySelector('#myComponent'); myComponentElement.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent uses the elementRef property to access the DOM element that represents the MyComponent instance. It then calls the componentFunction method using the syntax myComponentElement.componentFunction()
.
How do I execute an Angular component function from JavaScript?
To execute an Angular component function from JavaScript, you can use various approaches depending on how you access the component instance. The most common approach is to use the ViewChild decorator or ElementRef to get a reference to the component and then call its methods.
Here’s an example using ViewChild:
import { Component, ViewChild } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @ViewChild('myComponent') myComponent: MyComponent; callComponentFunction() { this.myComponent.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent calls the componentFunction method of the MyComponent instance by using the syntax this.myComponent.componentFunction()
.
Alternatively, you can use ElementRef to access the DOM element representing the component and then call the component’s methods using the nativeElement property.
Here’s an example using ElementRef:
import { Component, ElementRef } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { constructor(private elementRef: ElementRef) {} callComponentFunction() { const myComponentElement = this.elementRef.nativeElement.querySelector('#myComponent'); myComponentElement.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent uses the elementRef property to access the DOM element that represents the MyComponent instance. It then calls the componentFunction method using the syntax myComponentElement.componentFunction()
.
Is it possible to trigger an Angular component function from JavaScript?
Yes, it is possible to trigger an Angular component function from JavaScript by using various mechanisms provided by Angular. You can trigger a component function by calling it directly, using event binding, or by using custom events.
Here’s an example of triggering a component function by calling it directly:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { callComponentFunction() { this.componentFunction(); } componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent directly calls the componentFunction method to trigger its execution.
You can also trigger a component function using event binding. Here’s an example:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { callComponentFunction() { // Trigger the componentFunction by emitting a custom event this.componentFunction.emit(); } componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent emits a custom event called componentFunction. The componentFunction event is then bound to a method in the component’s template, triggering the execution of the component function.
Related Article: How to Use the forEach Loop with JavaScript
What are the different ways to call an Angular component function from JavaScript?
There are several ways to call an Angular component function from JavaScript, depending on how you access the component instance and the specific requirements of your application. Some common approaches include using ViewChild, ElementRef, EventEmitter, and @Output.
Here’s an example using ViewChild:
import { Component, ViewChild } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @ViewChild('myComponent') myComponent: MyComponent; callComponentFunction() { this.myComponent.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent calls the componentFunction method of the MyComponent instance by using the syntax this.myComponent.componentFunction()
.
You can also use ElementRef to access the DOM element representing the component and then call the component’s methods using the nativeElement property.
Here’s an example using ElementRef:
import { Component, ElementRef } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { constructor(private elementRef: ElementRef) {} callComponentFunction() { const myComponentElement = this.elementRef.nativeElement.querySelector('#myComponent'); myComponentElement.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent uses the elementRef property to access the DOM element that represents the MyComponent instance. It then calls the componentFunction method using the syntax myComponentElement.componentFunction()
.
Another approach is to use EventEmitter and @Output to create a custom event in the component and emit it when you want to trigger the function. Here’s an example:
import { Component, EventEmitter, Output } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @Output() componentFunctionEvent: EventEmitter = new EventEmitter(); callComponentFunction() { this.componentFunctionEvent.emit(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { @Input() componentFunctionEvent: EventEmitter; ngOnInit() { this.componentFunctionEvent.subscribe(() => { this.componentFunction(); }); } componentFunction() { console.log('Component function called'); } }
In this example, the AppComponent emits the componentFunctionEvent when the button is clicked. The MyComponent subscribes to the event in its ngOnInit method and triggers the componentFunction when the event is emitted.
These are just a few examples of different ways to call an Angular component function from JavaScript. The approach you choose depends on your specific requirements and the architecture of your application.
Can I dispatch an Angular component function from JavaScript?
No, you cannot directly dispatch an Angular component function from JavaScript. Angular component functions are typically executed within the Angular framework’s context, and they require the Angular component instance to be properly initialized and managed.
However, you can trigger the execution of an Angular component function from JavaScript by using various mechanisms provided by Angular, such as ViewChild, ElementRef, EventEmitter, and @Output. These mechanisms allow you to access the component instance and call its methods, effectively achieving the same result as dispatching a component function.
Here’s an example using ViewChild:
import { Component, ViewChild } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @ViewChild('myComponent') myComponent: MyComponent; callComponentFunction() { this.myComponent.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent calls the componentFunction method of the MyComponent instance by using the syntax this.myComponent.componentFunction()
.
While you cannot directly dispatch an Angular component function from JavaScript, using these mechanisms allows you to achieve the desired functionality.
How can I emit an Angular component function from JavaScript?
To emit an Angular component function from JavaScript, you can use EventEmitter and @Output in combination with event binding in Angular.
Here’s an example:
import { Component, EventEmitter, Output } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @Output() componentFunctionEvent: EventEmitter = new EventEmitter(); callComponentFunction() { this.componentFunctionEvent.emit(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { @Input() componentFunctionEvent: EventEmitter; ngOnInit() { this.componentFunctionEvent.subscribe(() => { this.componentFunction(); }); } componentFunction() { console.log('Component function called'); } }
In this example, the AppComponent emits the componentFunctionEvent when the button is clicked. The MyComponent subscribes to the event in its ngOnInit method and triggers the componentFunction when the event is emitted.
Related Article: How to Use Javascript Substring, Splice, and Slice
Is there a way to fire an Angular component function from JavaScript?
Yes, there are several ways to fire an Angular component function from JavaScript, depending on how you access the component instance and the specific requirements of your application. Some common approaches include using ViewChild, ElementRef, EventEmitter, and @Output.
Here’s an example using ViewChild:
import { Component, ViewChild } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @ViewChild('myComponent') myComponent: MyComponent; callComponentFunction() { this.myComponent.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent calls the componentFunction method of the MyComponent instance by using the syntax this.myComponent.componentFunction()
.
You can also use ElementRef to access the DOM element representing the component and then call the component’s methods using the nativeElement property.
Here’s an example using ElementRef:
import { Component, ElementRef } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { constructor(private elementRef: ElementRef) {} callComponentFunction() { const myComponentElement = this.elementRef.nativeElement.querySelector('#myComponent'); myComponentElement.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent uses the elementRef property to access the DOM element that represents the MyComponent instance. It then calls the componentFunction method using the syntax myComponentElement.componentFunction()
.
These are just a few examples of different ways to fire an Angular component function from JavaScript. The approach you choose depends on your specific requirements and the architecture of your application.
What is the recommended approach to call an Angular component function from JavaScript?
The recommended approach to call an Angular component function from JavaScript depends on the specific requirements of your application, as well as the architectural patterns and best practices you follow. However, some commonly recommended approaches include using ViewChild, ElementRef, and EventEmitter.
Using ViewChild is a common and straightforward approach to access a component instance and call its methods from JavaScript. By using the ViewChild decorator, you can get a reference to the component and directly call its functions.
Here’s an example:
import { Component, ViewChild } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @ViewChild('myComponent') myComponent: MyComponent; callComponentFunction() { this.myComponent.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent calls the componentFunction method of the MyComponent instance by using the syntax this.myComponent.componentFunction()
.
Another approach is to use ElementRef to access the DOM element representing the component and then call its methods using the nativeElement property. However, this approach is not recommended unless absolutely necessary, as it tightly couples your code to the DOM structure and bypasses the Angular component model.
Here’s an example using ElementRef:
import { Component, ElementRef } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { constructor(private elementRef: ElementRef) {} callComponentFunction() { const myComponentElement = this.elementRef.nativeElement.querySelector('#myComponent'); myComponentElement.componentFunction(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { componentFunction() { console.log('Component function called'); } }
In this example, the callComponentFunction method in the AppComponent uses the elementRef property to access the DOM element that represents the MyComponent instance. It then calls the componentFunction method using the syntax myComponentElement.componentFunction()
.
Additionally, you can use EventEmitter and @Output to create a custom event in the component and emit it when you want to trigger the function. This approach allows for decoupling and better component interaction.
Here’s an example using EventEmitter and @Output:
import { Component, EventEmitter, Output } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello, Angular!</h1> <button>Call Component Function</button> ` }) export class AppComponent { @Output() componentFunctionEvent: EventEmitter = new EventEmitter(); callComponentFunction() { this.componentFunctionEvent.emit(); } } @Component({ selector: 'app-my-component', template: ` <p>This is MyComponent</p> ` }) export class MyComponent { @Input() componentFunctionEvent: EventEmitter; ngOnInit() { this.componentFunctionEvent.subscribe(() => { this.componentFunction(); }); } componentFunction() { console.log('Component function called'); } }
In this example, the AppComponent emits the componentFunctionEvent when the button is clicked. The MyComponent subscribes to the event in its ngOnInit method and triggers the componentFunction when the event is emitted.
Ultimately, the recommended approach to call an Angular component function from JavaScript depends on the specific needs and constraints of your application. It’s important to consider the maintainability, performance, and scalability implications of each approach and choose the one that best fits your requirements.
Are there any limitations or restrictions when calling an Angular component function from JavaScript?
When calling an Angular component function from JavaScript, there are a few limitations and restrictions to be aware of:
1. Accessing component functions directly from JavaScript without following the proper Angular component lifecycle can lead to unexpected behavior or errors. It’s important to ensure that the component is properly initialized and its dependencies are resolved before calling any functions.
2. If you are using ViewChild or ElementRef to access the component instance, you need to make sure that the component is rendered and available in the DOM before attempting to access it. Otherwise, you may encounter null or undefined values.
3. When accessing component functions using ViewChild or ElementRef, you should avoid tightly coupling your code to the component’s template structure. This can make your code more brittle and harder to maintain, as any changes to the template may break the JavaScript code that relies on it.
4. Calling component functions directly from JavaScript bypasses the Angular change detection mechanism. This means that any changes made by the component function may not be reflected in the component’s template or trigger other lifecycle hooks. It’s important to keep this in mind and manually trigger change detection if necessary.
5. When using EventEmitter and @Output to trigger a component function, be aware that the event emission and function execution may be asynchronous. This can lead to potential race conditions or unexpected behavior if not handled properly.
6. Accessing component functions from JavaScript should be done with caution, as it can introduce security risks if not properly validated or sanitized. Make sure to validate any input parameters and sanitize any user-provided data before passing it to the component function to prevent potential security vulnerabilities.
Overall, while it is possible to call an Angular component function from JavaScript, it’s important to follow best practices, properly handle component lifecycle, and be aware of any limitations or restrictions to ensure the stability and reliability of your application.