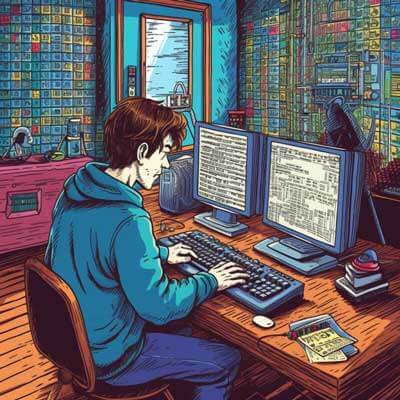
Table of Contents
Removing a specific item from an array in JavaScript is a common task that developers encounter in their day-to-day programming. Whether it's removing duplicate elements, filtering out unwanted values, or deleting a specific item based on a certain condition, there are several approaches you can take to achieve this. In this answer, we will explore two commonly used methods for removing elements from an array in JavaScript.
Method 1: Using the filter() Method
The filter() method is a powerful tool in JavaScript that allows you to create a new array containing only the elements that pass a certain condition. By using this method, you can easily remove a specific item from an array based on a given criteria. Here is an example:
let array = [1, 2, 3, 4, 5]; // Remove the number 3 from the array let newArray = array.filter(item => item !== 3); console.log(newArray); // Output: [1, 2, 4, 5]
In this example, we have an array [1, 2, 3, 4, 5]
and we want to remove the number 3 from it. We use the filter() method along with an arrow function to create a new array newArray
that only contains the elements that are not equal to 3. The resulting array [1, 2, 4, 5]
does not include the number 3.
Using the filter() method to remove elements from an array is a clean and concise approach. It allows you to easily specify the condition for removing elements and creates a new array without modifying the original one.
Related Article: How to Use Map Function on Objects in Javascript
Method 2: Using the splice() Method
The splice() method is another commonly used method for removing elements from an array in JavaScript. Unlike the filter() method, which creates a new array, the splice() method modifies the original array by removing or replacing elements at the specified index. Here is an example:
let array = [1, 2, 3, 4, 5]; // Remove the number 3 from the array array.splice(2, 1); console.log(array); // Output: [1, 2, 4, 5]
In this example, we use the splice() method to remove the element at index 2 (which is the number 3) from the array. The first parameter specifies the starting index from which to remove elements, and the second parameter specifies the number of elements to remove. In this case, we remove only one element.
The splice() method is useful when you want to modify the original array directly without creating a new one. However, it's important to note that the splice() method changes the original array, so be cautious when using it.
Why is the question asked?
The question of how to remove a specific item from an array in JavaScript is commonly asked because arrays are a fundamental data structure in JavaScript, and manipulating arrays is a common task in programming. Removing specific elements from an array can be useful in various scenarios, such as filtering out unwanted values, deleting duplicates, or modifying the array based on a certain condition.
Suggestions and Alternative Ideas
While the filter() and splice() methods are commonly used for removing elements from an array in JavaScript, there are other approaches you can consider depending on your specific use case. Here are a few alternative ideas:
1. Using the indexOf() and splice() methods:
let array = [1, 2, 3, 4, 5];
// Find the index of the number 3 in the array
let index = array.indexOf(3);
// Remove the element at the found index
if (index > -1) {
array.splice(index, 1);
}
console.log(array);
// Output: [1, 2, 4, 5]
2. Using the pop() or shift() methods:
let array = [1, 2, 3, 4, 5];
// Remove the last element from the array
array.pop();
console.log(array);
// Output: [1, 2, 3, 4]
// Remove the first element from the array
array.shift();
console.log(array);
// Output: [2, 3, 4]
3. Using the map() method:
let array = [1, 2, 3, 4, 5];
// Map over the array and return a new array without the number 3
let newArray = array.map(item => item === 3 ? null : item).filter(Boolean);
console.log(newArray);
// Output: [1, 2, 4, 5]
These alternative ideas provide different ways to remove elements from an array in JavaScript. Depending on your specific use case and requirements, you can choose the method that best suits your needs.
Related Article: How to Check a Radio Button Using jQuery
Best Practices
When removing a specific item from an array in JavaScript, it's important to keep the following best practices in mind:
1. Ensure the item you want to remove is present in the array before attempting to remove it. You can use methods like indexOf() or includes() to check if the item exists in the array before proceeding with the removal.
2. If you want to keep the original array intact and create a new array without the specific item, consider using the filter() method. This approach is generally safer and more maintainable, as it avoids modifying the original array.
3. If you need to modify the original array directly, be cautious when using methods like splice(). Make sure you provide the correct index and number of elements to remove, as incorrect usage can lead to unintended modifications.
4. Consider the performance implications of the chosen method, especially when dealing with large arrays. Some methods, such as splice(), can be less efficient for large arrays compared to others like filter().