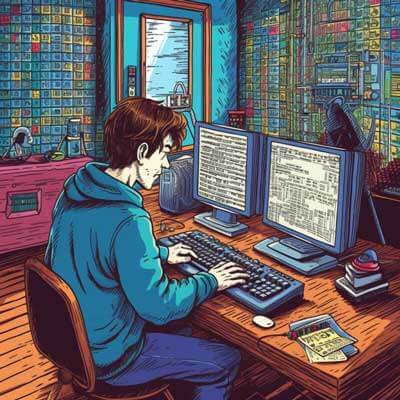
Table of Contents
Merging arrays and removing duplicates is a common task in JavaScript programming. This operation is often required when working with data sets or when combining multiple arrays into a single array. In this guide, we will explore different approaches to merge arrays and remove duplicates in JavaScript.
Why is this question asked?
The question of how to merge arrays and remove duplicates in JavaScript arises because JavaScript provides various methods and techniques to accomplish this task. Depending on the specific requirements and constraints of the project, different approaches may be more suitable. Therefore, it is important for developers to understand the available options and choose the most appropriate solution.
Related Article: How To Replace All Occurrences Of A String In Javascript
Potential reasons for merging arrays and removing duplicates
There are several potential reasons why one might need to merge arrays and remove duplicates in JavaScript:
1. Combining data from multiple sources: When working with data from different sources, it is often necessary to merge the arrays containing the data into a single array. This can be useful, for example, when consolidating data from multiple API responses or when merging data from different database queries.
2. Removing duplicates: Duplicates in an array can cause issues when performing operations such as searching or iterating over the array. By removing duplicates, we can ensure that each element in the array is unique and avoid any unintended side effects.
Possible approaches to merging arrays and removing duplicates
There are multiple approaches to merge arrays and remove duplicates in JavaScript. Let's explore a few of them:
1. Using the spread operator and Set:
One simple approach is to use the spread operator (...) to merge the arrays, and then convert the merged array into a Set to remove duplicates. Finally, convert the Set back into an array using the spread operator.
const array1 = [1, 2, 3]; const array2 = [2, 3, 4]; const mergedArray = [...array1, ...array2]; const uniqueArray = [...new Set(mergedArray)]; console.log(uniqueArray);
Output:
[1, 2, 3, 4]
This approach leverages the Set data structure, which only allows unique values. By converting the merged array into a Set and then back into an array, we effectively remove duplicates.
2. Using the concat method and filter:
Another approach is to use the concat method to merge the arrays, and then use the filter method to remove duplicates by checking for the index of each element.
const array1 = [1, 2, 3]; const array2 = [2, 3, 4]; const mergedArray = array1.concat(array2); const uniqueArray = mergedArray.filter((value, index) => mergedArray.indexOf(value) === index); console.log(uniqueArray);
Output:
[1, 2, 3, 4]
In this approach, the concat method is used to merge the arrays, while the filter method is used to create a new array that only contains elements whose index matches their first occurrence in the merged array. This effectively removes duplicates.
Best practices and considerations
When merging arrays and removing duplicates in JavaScript, it is important to consider the following best practices:
1. Use the most appropriate approach for your specific use case. Depending on the size of the arrays and the desired performance, different approaches may have varying efficiency. For example, using the Set data structure may have better performance for larger arrays, while using the concat and filter approach may be more suitable for smaller arrays.
2. Consider the order of elements. The order of elements in the merged array may differ depending on the approach used. For example, the spread operator approach maintains the order of elements, while the filter approach may rearrange the elements to remove duplicates. Be aware of this potential difference and choose the approach accordingly.
3. Consider the data types of the elements. The approaches mentioned above work well for arrays of primitive values. However, if you are working with arrays of objects or complex data types, you may need to implement custom logic to compare and remove duplicates based on specific object properties or criteria.
4. Consider performance implications. Depending on the size of the arrays and the frequency of the operation, the performance of the chosen approach may vary. If performance is a critical factor, consider benchmarking different approaches to determine the most efficient solution for your specific scenario.
Related Article: How to Play Audio Files Using JavaScript
Alternative ideas
While the approaches mentioned above are commonly used to merge arrays and remove duplicates in JavaScript, there are alternative ideas worth exploring:
1. Using the reduce method: The reduce method can be used to iterate over the arrays and build a new array with unique elements. This approach requires custom logic to check for duplicates and add elements to the new array.
2. Using third-party libraries: There are several popular JavaScript libraries, such as Lodash or Underscore.js, that provide utility functions for working with arrays. These libraries often include methods specifically designed for merging arrays and removing duplicates. Consider using these libraries if they align with your project's requirements and dependencies.