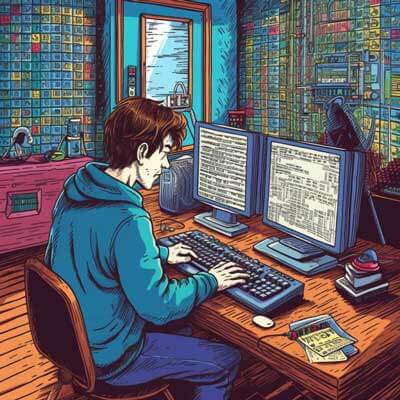
The ‘ValueError: Setting an Array Element with a Sequence’ error occurs in Python when you try to assign a sequence (such as a list or another array) to a single element of a NumPy array. This error typically arises when the shapes of the assigned sequence and the target element do not match. In this answer, we will explore two possible solutions to fix this error.
Possible Solution 1: Reshape the Sequence
One way to fix the ‘ValueError: Setting an Array Element with a Sequence’ error is to reshape the sequence so that its shape matches the shape of the target element. This can be done using the NumPy reshape() function. Here’s an example:
import numpy as np # Create a NumPy array arr = np.array([[1, 2], [3, 4]]) # Reshape the sequence sequence = np.array([5, 6]).reshape((1, 2)) # Assign the reshaped sequence to the target element arr[0] = sequence print(arr)
In this example, we have a 2D NumPy array called ‘arr’. We want to assign the sequence [5, 6] to the first row of ‘arr’. However, the shape of the sequence is (2,) while the shape of the target element is (1, 2). By using the reshape() function, we reshape the sequence to have the same shape as the target element. The resulting array will be:
[[5 6] [3 4]]
By reshaping the sequence to match the shape of the target element, we avoid the ‘ValueError: Setting an Array Element with a Sequence’ error.
Related Article: How To Fix ValueError: Invalid Literal For Int With Base 10
Possible Solution 2: Use a Different Assignment Method
Another way to fix the ‘ValueError: Setting an Array Element with a Sequence’ error is to use a different assignment method that is compatible with sequences. One such method is the np.put() function. Here’s an example:
import numpy as np # Create a NumPy array arr = np.array([[1, 2], [3, 4]]) # Create a sequence sequence = np.array([5, 6]) # Assign the sequence to the target element using np.put() np.put(arr, [0], sequence) print(arr)
In this example, we use the np.put() function to assign the sequence [5, 6] to the first element of the ‘arr’ array. The resulting array will be:
[[5 6] [3 4]]
By using the np.put() function, we can avoid the ‘ValueError: Setting an Array Element with a Sequence’ error and assign the sequence to the target element successfully.
Why was the question asked?
The question “How to fix ‘ValueError: Setting an Array Element with a Sequence’ in Python” is commonly asked by Python developers who encounter this error while working with NumPy arrays. This error often arises when developers try to assign a sequence to a single element of a NumPy array without considering the shape compatibility between the sequence and the target element. Understanding the cause of this error and how to fix it is essential for Python developers who work with NumPy arrays.
Potential Reasons for the Error
Here are a few potential reasons why the ‘ValueError: Setting an Array Element with a Sequence’ error may occur:
1. Shape Mismatch: The shape of the assigned sequence does not match the shape of the target element in the NumPy array.
2. Incorrect Assignment Method: The wrong method is being used to assign the sequence to the target element. For example, using simple assignment (e.g., arr[0] = sequence) instead of a method like np.put().
3. Invalid Data Type: The sequence contains elements of an incompatible data type for the target element in the NumPy array.
Suggestions and Alternative Ideas
To fix the ‘ValueError: Setting an Array Element with a Sequence’ error, consider the following suggestions:
1. Check Shape Compatibility: Ensure that the shape of the assigned sequence matches the shape of the target element in the NumPy array. If the shapes do not match, reshape the sequence using the reshape() function.
2. Use Appropriate Assignment Method: If the simple assignment (e.g., arr[0] = sequence) is causing the error, try using a different assignment method such as np.put().
3. Verify Data Types: Make sure that the data type of the elements in the sequence is compatible with the data type of the target element in the NumPy array. If necessary, convert the data types using functions like np.astype().
Example of Best Practice
Here’s an example of a best practice for avoiding the ‘ValueError: Setting an Array Element with a Sequence’ error:
import numpy as np # Create a NumPy array arr = np.zeros((2, 3)) # Create a sequence with correct shape and data type sequence = np.array([[1, 2, 3], [4, 5, 6]]) # Assign the sequence to the target element arr[0] = sequence print(arr)
In this example, we follow the best practice of ensuring that the shape and data type of the sequence match the target element. By creating the sequence with the correct shape and data type, we can assign it to the target element without encountering the ‘ValueError: Setting an Array Element with a Sequence’ error.