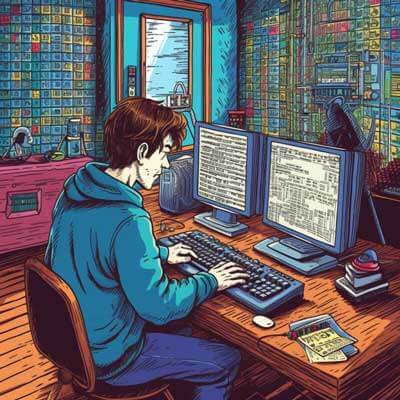
Table of Contents
What is void(0)?
The void operator in Javascript is used to evaluate an expression and return undefined. When used with the value 0, as in void(0), it essentially means "do nothing" or "return undefined". The void(0) expression is commonly used in event handlers or anchor tags to prevent the page from refreshing or navigating to a new URL.
It's important to note that void(0) is not a function, but an operator. The parentheses are used to group the expression and indicate that it should be evaluated. The value inside the parentheses can be any valid Javascript expression, but using 0 is a common convention.
Related Article: How To Add Days To a Date In Javascript
Why is the question asked?
The question about understanding void(0) in Javascript is often asked by developers who are new to the language or are unfamiliar with this particular expression. It is natural to wonder about its purpose and how it can be used effectively in code. By understanding void(0), developers can utilize it in their applications to achieve specific functionalities or behaviors.
Potential Uses of void(0)
Although void(0) may seem unusual at first, it has several practical applications in Javascript. Here are a few examples:
Preventing Page Refresh
One of the most common uses of void(0) is to prevent a page from refreshing when clicking on a link or submitting a form. By using void(0) as the value of the href attribute in an anchor tag, the browser will not navigate to a new page. This is often seen in scenarios where the link is used for executing a JavaScript function or displaying a modal window.
Here's an example of how void(0) can be used to prevent page refresh:
<a href="void(0);">Click Me</a>
In this example, the void(0) expression is used as the value of the href attribute, ensuring that the link does not trigger a page refresh. The onclick attribute is used to call the doSomething()
function when the link is clicked.
Returning Undefined
Another use of void(0) is to explicitly return undefined from a JavaScript function. In some cases, a function may need to perform certain actions without returning any specific value. By using void(0) as the return value, the function can indicate that it does not produce a meaningful result.
Here's an example of how void(0) can be used to return undefined from a function:
function doSomething() { // Perform some actions return void(0); }
In this example, the doSomething()
function performs some actions but does not return a specific value. By using void(0) as the return statement, it explicitly indicates that the function's result is undefined.
Alternative Ideas and Best Practices
While void(0) can be useful in certain situations, it is important to consider alternative approaches and best practices when writing Javascript code. Here are a few suggestions:
Using event.preventDefault()
Instead of relying on void(0) to prevent page refresh, you can use the event.preventDefault()
method. This method is available on event objects and can be called within event handlers to prevent the default action associated with the event.
function handleClick(event) { event.preventDefault(); // Perform some actions }
In this example, the event.preventDefault()
method is called within the click event handler to prevent the default action, which is typically page navigation. This approach provides more control and flexibility compared to using void(0) in anchor tags.
Returning false in event handlers
Another alternative to void(0) in event handlers is to return false at the end of the handler function. Returning false has a similar effect to void(0) and prevents the default behavior associated with the event.
function handleClick() { // Perform some actions return false; }
In this example, returning false from the event handler will prevent the default action, such as form submission or anchor navigation. However, it's important to note that returning false not only prevents the default action but also stops the event propagation. If event propagation is desired, using event.preventDefault()
is a better option.
Using null or undefined
In some cases, using null or undefined may be more appropriate than void(0). For example, if a function needs to return a falsy value, such as null or undefined, it can simply return null or undefined directly.
function getDefaultValue() { return null; }
In this example, the getDefaultValue()
function returns null as the default value. This approach is more explicit and easier to understand compared to using void(0) for the same purpose.