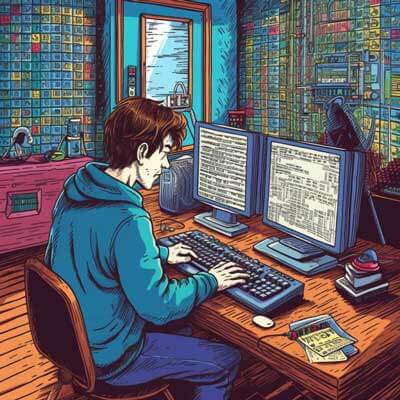
- The Importance of Patching in Linux
- Writing a Bash Script to Apply Patches
- Best Practices for Patch Management
- Scripting Languages for Patching in Linux
- Popular Tools for Automating Patching
- Frequency of Applying Security Patches in Linux
- Steps Involved in the Patching Process
- Automatically Installing Linux Updates with Shell Scripts
- Considerations when Patching Linux Systems
- Benefits of Using Automated Patching Tools
The Importance of Patching in Linux
Patching is a critical process in maintaining the security and stability of Linux systems. As the Linux operating system and its associated software evolve, vulnerabilities and bugs are discovered, which can expose systems to potential security threats or cause software to malfunction. Patching involves applying updates or fixes to address these issues and ensure that the system remains secure and reliable.
Related Article: Adding Color to Bash Scripts in Linux
Writing a Bash Script to Apply Patches
Bash scripting is a useful tool for automating tasks in Linux, including the patching process. By writing a bash script, you can streamline the patching workflow and save time and effort. Let’s take a look at an example of a bash script that applies patches to Linux systems:
#!/bin/bash # Check if the system is running as root if [[ $(id -u) -ne 0 ]]; then echo "This script must be run as root" exit 1 fi # Update the package manager apt update # Install available updates apt upgrade -y # Verify if the updates were successfully installed if [[ $? -eq 0 ]]; then echo "Updates applied successfully" else echo "Failed to apply updates" fi
In this example, the bash script checks if the user running the script has root privileges. It then uses the apt
package manager to update the system and install any available updates. Finally, it provides feedback on the success or failure of the patching process.
Best Practices for Patch Management
Effective patch management is crucial for maintaining the security and stability of Linux systems. Here are some best practices to consider when managing patches:
1. Regularly update your systems: It is important to keep your Linux systems up to date by regularly applying security patches and updates. This helps to protect against known vulnerabilities and ensures that your systems are running the latest software versions.
2. Test patches before deployment: Before applying patches to production systems, it is recommended to test them in a controlled environment. This helps to identify any compatibility issues or unintended consequences that may arise from the patching process.
3. Prioritize critical patches: Not all patches are created equal. It is essential to prioritize critical patches that address known security vulnerabilities or critical bugs. This helps to minimize the risk of exploitation and ensures that your systems remain secure.
4. Maintain an inventory of software and dependencies: Keeping track of the software and dependencies running on your Linux systems is essential for effective patch management. This allows you to identify which components are affected by specific patches and ensure that all relevant updates are applied.
5. Monitor vendor notifications and security advisories: Stay informed about security vulnerabilities and patches released by software vendors and the Linux community. Monitor security advisories and subscribe to relevant mailing lists or RSS feeds to receive timely notifications about new patches.
Scripting Languages for Patching in Linux
While bash scripting is a popular choice for automating patching tasks in Linux, there are other scripting languages that can be used as well. Let’s explore a few alternatives:
1. Python: Python is a versatile scripting language that is widely used in the Linux ecosystem. It has a rich set of libraries and frameworks that make it easy to write scripts for patch management. For example, the apt
module in Python allows you to interact with the package manager and apply updates to Debian-based systems.
import apt cache = apt.Cache() cache.update() cache.upgrade()
2. Perl: Perl is another scripting language commonly used in Linux environments. It has a long history of being used for system administration tasks, including patch management. The CPAN module Sys::Patch
provides a convenient interface for applying patches to Linux systems.
use Sys::Patch; my $patcher = Sys::Patch->new(); $patcher->update(); $patcher->upgrade();
3. Ruby: Ruby is a dynamic, object-oriented scripting language that is gaining popularity in the Linux community. It has a wide range of libraries and tools that make it suitable for automating patch management tasks. The apt
gem in Ruby provides a simple way to interact with the package manager and apply updates.
require 'apt' Apt.update Apt.upgrade
Related Article: How to Calculate the Sum of Inputs in Bash Scripts
Popular Tools for Automating Patching
In addition to scripting languages, there are several popular tools available for automating the patching process in Linux. These tools provide a more comprehensive and centralized approach to patch management. Let’s explore a few of them:
1. Ansible: Ansible is a useful automation tool that allows you to define and manage infrastructure as code. It provides a wide range of modules for managing Linux systems, including patch management. With Ansible, you can define playbooks that specify the desired state of your systems and apply patches accordingly.
- name: Update and upgrade packages apt: update_cache: yes upgrade: dist
2. Puppet: Puppet is a configuration management tool that allows you to define and enforce the desired state of your systems. It provides a declarative language for managing configurations, including patch management. With Puppet, you can define manifests that specify the required patches for your systems.
package { 'openssh-server': ensure => 'latest', }
3. Chef: Chef is another popular configuration management tool that follows a similar approach to Puppet. It allows you to define recipes that describe the desired state of your systems, including patch management. With Chef, you can ensure that the required patches are applied to your Linux systems.
package 'openssh-server' do action :upgrade end
Frequency of Applying Security Patches in Linux
The frequency of applying security patches in Linux depends on various factors, including the criticality of the vulnerabilities being addressed, the risk tolerance of the organization, and the availability of resources for testing and deployment. In general, it is recommended to apply security patches as soon as possible to minimize the exposure to potential threats.
For critical security vulnerabilities, patches should be applied immediately, especially if there is an active exploit in the wild. Organizations may also consider prioritizing patches based on the severity of the vulnerability, the potential impact on the system, and the likelihood of exploitation.
In some cases, patches may need to be tested in a non-production environment before being applied to production systems. This testing phase helps to ensure that the patches do not introduce compatibility issues or unintended consequences. However, organizations should strive to minimize the time between patch availability and deployment to reduce the risk of exploitation.
Steps Involved in the Patching Process
The patching process in Linux typically involves several steps to ensure the successful application of updates. Let’s take a look at the common steps involved in the patching process:
1. Assessment: The first step in the patching process is to assess the available patches and determine their relevance to your systems. This involves reviewing security advisories, vendor notifications, and other sources of patch information to identify patches that address vulnerabilities or bugs affecting your systems.
2. Testing: Before applying patches to production systems, it is important to test them in a controlled environment. This helps to identify any compatibility issues or unintended consequences that may arise from the patching process. Testing can be performed on a separate test environment or using virtualization technologies.
3. Planning: Once patches have been assessed and tested, a patching plan should be developed. This plan includes determining the order of patch deployment, scheduling maintenance windows, and coordinating with stakeholders to minimize disruption to services or downtime.
4. Deployment: The next step is to deploy the patches to the target systems. This can be done manually or using automated patch management tools. During deployment, it is important to monitor the progress and verify that patches are applied successfully.
5. Verification: After patches have been deployed, it is crucial to verify that they have been applied correctly and that the systems are functioning as expected. This can involve running tests, checking system logs, and performing a post-patching assessment to ensure that the vulnerabilities or bugs have been addressed.
6. Monitoring: Patch management is an ongoing process, and it is important to monitor systems for new vulnerabilities and patches. This involves staying informed about security advisories, vendor notifications, and other sources of patch information to ensure that systems remain up to date and protected against emerging threats.
Related Article: Locating Largest Memory in Bash Script on Linux
Automatically Installing Linux Updates with Shell Scripts
Shell scripts provide a convenient way to automate the installation of Linux updates. By writing a shell script, you can streamline the process of checking for updates, applying patches, and verifying the installation. Let’s take a look at an example of a shell script that automatically installs Linux updates:
#!/bin/bash # Check if the system is running as root if [[ $(id -u) -ne 0 ]]; then echo "This script must be run as root" exit 1 fi # Update the package manager apt update # Install available updates apt upgrade -y # Verify if the updates were successfully installed if [[ $? -eq 0 ]]; then echo "Updates installed successfully" else echo "Failed to install updates" fi
In this example, the shell script first checks if the user running the script has root privileges. It then uses the apt
package manager to update the system and install any available updates. Finally, it provides feedback on the success or failure of the installation process.
Considerations when Patching Linux Systems
When patching Linux systems, there are several considerations that should be taken into account to ensure a smooth and successful patching process. Let’s explore some important considerations:
1. Backup: Before applying patches, it is recommended to perform a backup of critical data and configurations. This helps to protect against any unforeseen issues that may arise during the patching process. In the event of a problem, a backup allows you to restore the system to its previous state.
2. Dependency Management: Linux systems often have complex dependency chains, where updates to one package may require updates to other packages. It is important to carefully manage dependencies to ensure that all relevant updates are applied and that the system remains in a consistent state.
3. System Compatibility: When applying patches, it is crucial to consider the compatibility of the patches with the existing system configurations and software stack. Compatibility issues can arise if patches are applied to systems running outdated software versions or if patches conflict with custom configurations. It is recommended to test patches in a non-production environment before applying them to production systems.
4. Rollback Plan: Despite thorough testing and planning, issues can still occur during the patching process. It is important to have a rollback plan in place to revert the system to its previous state in case of any problems. This includes having backups, documenting the patching process, and ensuring that the necessary tools and resources are available to roll back the changes.
Benefits of Using Automated Patching Tools
Automated patching tools provide several benefits for managing the patching process in Linux systems. Let’s explore some of the key advantages:
1. Time-saving: Automated patching tools streamline the patching process by automating repetitive tasks. This saves time and effort for system administrators, allowing them to focus on other critical tasks.
2. Consistency: Automated patching tools ensure that patches are applied consistently across multiple systems. This helps to maintain a consistent security posture and reduces the risk of vulnerabilities caused by human error or oversight.
3. Centralized Management: Patch management tools provide a centralized platform for managing patches across multiple systems. This allows administrators to easily track the status of patches, schedule deployments, and monitor the patching process from a single interface.
4. Compliance: Automated patching tools can help organizations meet compliance requirements by ensuring that systems are up to date with the latest security patches. This is particularly important in regulated industries where security and compliance are top priorities.
5. Reporting and Auditing: Patch management tools often provide reporting and auditing capabilities, allowing administrators to generate reports on patch status, track patch deployment history, and demonstrate compliance with security policies and regulations.