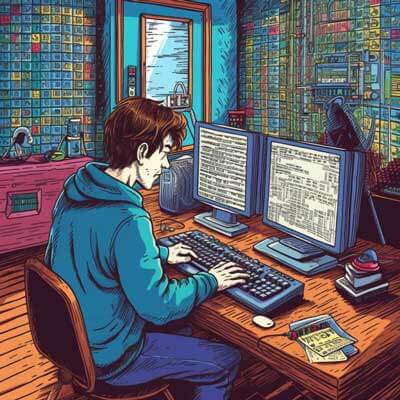
If you encounter the error message “undefined reference to pthread_create” while compiling a program in Linux, it means that the linker is unable to find the required pthread library. This error is commonly caused by a missing or incorrect linker flag. To fix this issue, you can follow the steps below:
1. Check if the pthread library is installed
First, make sure that the pthread library is installed on your system. You can do this by running the following command in your terminal:
sudo apt-get install libpthread-stubs0-dev
If the library is already installed, you will see a message indicating that it is up to date. Otherwise, the installation process will begin.
Related Article: Troubleshooting: Unable to Save Bash Scripts in Vi on Linux
2. Add the -pthread linker flag
To resolve the “undefined reference to pthread_create” error, you need to add the -pthread linker flag when compiling your program. This flag tells the linker to link the pthread library. Here’s an example of how to compile a C program with the -pthread flag:
gcc -pthread your_program.c -o your_program
If you are using C++, you can use the g++ command:
g++ -pthread your_program.cpp -o your_program
Make sure to replace “your_program.c” or “your_program.cpp” with the actual name of your source file.
3. Use the pthread library functions
If you are still encountering the same error after adding the -pthread flag, make sure that you are using the pthread library functions correctly in your code. The pthread_create function, which is causing the undefined reference error, is used to create a new thread. Here’s an example of how to correctly use pthread_create:
#include <pthread.h> void* thread_function(void* arg) { // Thread logic goes here return NULL; } int main() { pthread_t thread; pthread_create(&thread, NULL, thread_function, NULL); pthread_join(thread, NULL); return 0; }
In this example, a new thread is created using pthread_create, and then the pthread_join function is used to wait for the thread to complete before exiting the main function.
4. Check for other potential issues
If the above steps did not solve the issue, there might be other factors causing the “undefined reference to pthread_create” error. Here are some additional things to check:
– Make sure that you are including the pthread.h header file in your source code.
– Verify that your compiler and linker are up to date.
– Check for any typos or syntax errors in your code that might be causing the linker to fail.
Related Article: Fixing the 'Linux Username Not In The Sudoers File' Error