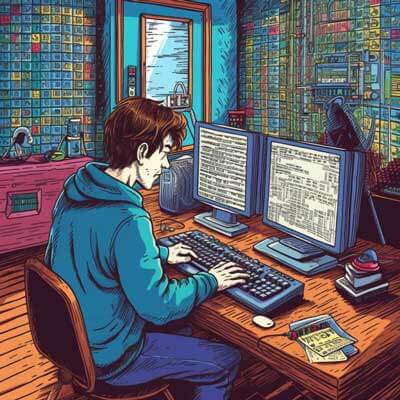
Looping through an array of strings in Bash is a common task when working with Linux command line scripts. In this guide, we will explore several ways to accomplish this.
Method 1: Using a For Loop
One way to loop through an array of strings in Bash is by using a for loop. Here is an example:
#!/bin/bash # Declare an array of strings fruits=("apple" "banana" "orange" "grape") # Loop through the array using a for loop for fruit in "${fruits[@]}"; do echo "I like $fruit" done
In this example, we declare an array of strings named fruits
with four elements. We then use a for loop to iterate over each element in the array. The fruit
variable is assigned the value of each element in turn, and we use echo
to print a message for each fruit.
Related Article: How To Echo a Newline In Bash
Method 2: Using a While Loop
Another way to loop through an array of strings in Bash is by using a while loop. Here is an example:
#!/bin/bash # Declare an array of strings fruits=("apple" "banana" "orange" "grape") # Get the length of the array length=${#fruits[@]} # Initialize a counter variable i=0 # Loop through the array using a while loop while [[ i -lt length ]]; do echo "I like ${fruits[i]}" ((i++)) done
In this example, we declare an array of strings named fruits
with four elements. We use the ${#fruits[@]}
syntax to get the length of the array and store it in the variable length
.
We then initialize a counter variable i
to 0 and use a while loop to iterate over each element in the array. Inside the loop, we use ${fruits[i]}
to access the value of the current element and ((i++))
to increment the counter variable.
Best Practices
When looping through an array of strings in Bash, it is important to keep a few best practices in mind:
1. Use double quotes around the array variable: When referencing the array variable, it is a good practice to enclose it in double quotes ("${array[@]}"
). This ensures that each element is treated as a separate string, even if it contains spaces or special characters.
2. Use the [@]
notation to reference the entire array: When looping through an array, use the [@]
notation ("${array[@]}"
) to reference the entire array. This ensures that each element is treated as a separate item, even if it contains spaces or special characters.
3. Use descriptive variable names: Choose meaningful variable names that describe the purpose of the variable. This makes the code more readable and easier to understand.
4. Consider using printf
instead of echo
: While echo
is commonly used for printing output in Bash scripts, printf
provides more flexibility and control over the output format. Consider using printf
when you need more advanced formatting options.
Alternative Ideas
Apart from using for and while loops, there are a few alternative ideas for looping through an array of strings in Bash:
1. Using a C-style for loop: Bash supports C-style for loops, which can be useful when you need more control over the loop initialization, condition, and iteration. Here is an example:
# Declare an array of strings fruits=("apple" "banana" "orange" "grape") # Loop through the array using a C-style for loop for ((i=0; i<${#fruits[@]}; i++)); do echo "I like ${fruits[i]}" done
2. Using a range-based loop: If you have a continuous range of integers that you want to loop through, you can use a range-based loop. Here is an example:
# Loop through a range of integers from 1 to 5 for i in {1..5}; do echo "Number: $i" done
This can be useful if you don’t have an array defined and just want to iterate over a sequence of numbers.
Related Article: How to Use If-Else Statements in Shell Scripts