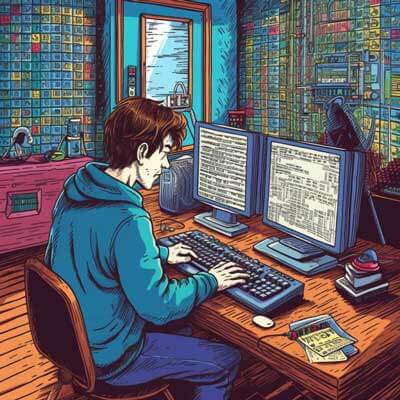
- The Role of JavaScript in AI Implementations
- Supporting AI Development with Node.js
- Introduction to TensorFlow.js and its Use in Machine Learning
- Implementing NLP Functionalities with Open-source Libraries
- Building Recommendation Systems using AI in Node.js
- Examples of Predictive Models in Node.js
- Advantages of Using Open-source Libraries for NLP in AI
- Limitations of TensorFlow.js for Machine Learning in Node.js
- Using JavaScript for Data Preprocessing in AI
- Best Practices for Implementing AI in Node.js
- Additional Resources
The Role of JavaScript in AI Implementations
JavaScript has become one of the most popular programming languages in recent years, primarily due to its versatility and widespread adoption in web development. However, its role is not limited to just client-side scripting or building interactive web applications. JavaScript has also made significant advancements in the field of artificial intelligence (AI) implementations.
Traditionally, AI has been associated with languages like Python and R, which are known for their extensive libraries and frameworks for machine learning and data analysis. However, with the advent of libraries like TensorFlow.js, JavaScript has emerged as a viable option for AI development.
JavaScript’s popularity and ease of use make it an attractive choice for AI implementations. Developers who are already familiar with JavaScript can leverage their existing knowledge and skills to build AI-powered applications without having to learn a new programming language. Additionally, JavaScript’s asynchronous nature and event-driven programming model make it well-suited for handling large datasets and real-time data processing, which are essential components of AI applications.
Moreover, JavaScript’s compatibility with web browsers allows for seamless integration of AI functionality into web applications. This opens up a wide range of possibilities, including chatbots, recommendation systems, sentiment analysis, and more, all powered by AI algorithms running directly in the browser.
Let’s take a look at how JavaScript, specifically in the context of Node.js, supports AI development and explore some of the key libraries and frameworks that enable AI implementations in Node.js.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Supporting AI Development with Node.js
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to run JavaScript code on the server-side, enabling server-side scripting and building scalable network applications. Node.js provides a rich ecosystem of packages and frameworks, making it a popular choice for web and backend development.
When it comes to AI development, Node.js offers several advantages. Firstly, it allows for seamless integration of AI functionality into existing web applications. Developers can leverage the power of AI to enhance user experiences, optimize business processes, and gain insights from data, all within the same codebase.
Secondly, Node.js provides a vast ecosystem of open-source libraries and frameworks that facilitate AI development. These libraries range from general-purpose machine learning frameworks to specialized libraries for natural language processing (NLP), computer vision, and more. One such library is TensorFlow.js.
Introduction to TensorFlow.js and its Use in Machine Learning
TensorFlow.js is an open-source library developed by Google that brings the power of TensorFlow, a popular machine learning framework, to JavaScript and Node.js. It allows developers to build and train machine learning models directly in the browser or on the server-side using JavaScript.
One of the key advantages of TensorFlow.js is its ability to leverage the GPU capabilities of modern web browsers and hardware accelerators like WebGL. This enables high-performance computations for training and running machine learning models, even in the browser. With TensorFlow.js, developers can perform tasks like image classification, object detection, and natural language processing, all without leaving the JavaScript ecosystem.
To get started with TensorFlow.js in Node.js, you first need to install the library using npm, the package manager for Node.js. Open your terminal and run the following command:
npm install @tensorflow/tfjs
Once installed, you can import TensorFlow.js into your Node.js application using the following code snippet:
const tf = require('@tensorflow/tfjs');
Now, let’s explore how to implement NLP functionalities using open-source libraries in Node.js.
Implementing NLP Functionalities with Open-source Libraries
Natural Language Processing (NLP) is a subfield of AI that focuses on the interaction between computers and human language. It enables machines to understand, interpret, and generate human language, opening up possibilities for applications like sentiment analysis, chatbots, language translation, and more.
Node.js provides a range of open-source libraries for NLP that can be seamlessly integrated into your AI implementations. One such library is Natural, a general-purpose NLP library that provides a wide range of functionalities, including tokenization, stemming, part-of-speech tagging, and more.
To use the Natural library in your Node.js application, you first need to install it using npm. Run the following command in your terminal:
npm install natural
Once installed, you can import the Natural library into your Node.js application using the following code snippet:
const natural = require('natural');
Now, let’s look at an example of implementing sentiment analysis using the Natural library in Node.js.
Example 1: Sentiment Analysis with Natural in Node.js
const natural = require('natural'); // Create a new sentiment analyzer const analyzer = new natural.SentimentAnalyzer(); // Analyze the sentiment of a sentence const sentence = "I love this product! It exceeded my expectations."; const sentiment = analyzer.getSentiment(sentence); console.log(`Sentiment: ${sentiment}`);
In this example, we import the Natural library and create a new sentiment analyzer. We then analyze the sentiment of a given sentence using the getSentiment
method and log the result to the console.
Example 2: Tokenization with Natural in Node.js
const natural = require('natural'); // Tokenize a sentence const sentence = "Natural language processing is a fascinating field."; const tokenizer = new natural.WordTokenizer(); const tokens = tokenizer.tokenize(sentence); console.log(tokens);
In this example, we use the Natural library to tokenize a given sentence into individual words. We create a new word tokenizer using the WordTokenizer
class and then use the tokenize
method to tokenize the sentence. The resulting tokens are then logged to the console.
These examples demonstrate how open-source libraries like Natural can be used to implement NLP functionalities in Node.js, enabling developers to build AI-powered applications with ease.
Related Article: How To Use Loop Inside React JSX
Building Recommendation Systems using AI in Node.js
Recommendation systems are a common use case for AI implementations, especially in the context of e-commerce, content platforms, and personalized user experiences. These systems analyze user preferences and behavior to provide personalized recommendations, such as product recommendations, movie recommendations, music recommendations, and more.
In Node.js, several libraries and frameworks can be used to build recommendation systems. One popular library is the LightFM library, which provides a flexible and efficient implementation of recommendation algorithms. LightFM supports both collaborative filtering and content-based filtering, allowing developers to build hybrid recommendation systems.
To use the LightFM library in your Node.js application, you first need to install it using npm. Run the following command in your terminal:
npm install lightfm
Once installed, you can import the LightFM library into your Node.js application using the following code snippet:
const lightfm = require('lightfm');
Now, let’s look at an example of building a movie recommendation system using the LightFM library in Node.js.
Example 1: Movie Recommendation System with LightFM in Node.js
const lightfm = require('lightfm'); // Load the movie recommendation dataset const dataset = lightfm.datasets.MovieLens(); // Build a recommendation model using collaborative filtering const model = lightfm.LightFM({ loss: 'warp', // Weighted Approximate-Rank Pairwise learning_rate: 0.05, no_components: 30, }); // Train the model using the dataset model.fit(dataset, epochs=30, num_threads=2); // Get recommendations for a user const user_id = 1; const n_rec = 5; const recommendations = model.predict(user_id, np.arange(dataset.shape[1]), num_threads=2); console.log(recommendations);
In this example, we import the LightFM library and load the MovieLens dataset, a popular dataset for movie recommendation systems. We then build a recommendation model using collaborative filtering, specify the loss function, learning rate, and number of components. Next, we train the model using the dataset and specify the number of epochs and number of threads. Finally, we generate recommendations for a specific user and log the recommendations to the console.
Example 2: Content-Based Filtering with LightFM in Node.js
const lightfm = require('lightfm'); // Load the movie recommendation dataset const dataset = lightfm.datasets.MovieLens(); // Build a recommendation model using content-based filtering const model = lightfm.LightFM({ loss: 'warp-kos', // Weighted Approximate-Rank Pairwise with content-based features learning_rate: 0.05, no_components: 30, }); // Train the model using the dataset and item features model.fit(dataset, item_features=dataset.item_features, epochs=30, num_threads=2); // Get recommendations for a user const user_id = 1; const n_rec = 5; const recommendations = model.predict(user_id, np.arange(dataset.shape[1]), item_features=dataset.item_features, num_threads=2); console.log(recommendations);
In this example, we build a recommendation model using content-based filtering, which takes into account item features in addition to user preferences. We specify the loss function, learning rate, number of components, and train the model using the dataset and item features. Finally, we generate recommendations for a specific user, taking into account the item features, and log the recommendations to the console.
These examples demonstrate how libraries like LightFM can be used to build recommendation systems in Node.js, empowering developers to create personalized and engaging experiences for their users.
Examples of Predictive Models in Node.js
Predictive modeling is a technique used in machine learning to predict future outcomes based on historical data. In Node.js, several libraries and frameworks can be used to build predictive models, ranging from general-purpose machine learning libraries to specialized libraries for specific tasks.
One such library is the scikit-learn library, which is widely used in Python for machine learning tasks. Although scikit-learn is primarily a Python library, it can be accessed and used in Node.js using the Python shell.
To use scikit-learn in Node.js, you first need to have Python installed on your machine. You can then install the scikit-learn library using pip, the Python package manager. Open your terminal and run the following command:
pip install scikit-learn
Once installed, you can use the Python shell in Node.js to import and use the scikit-learn library. Here’s an example of building a predictive model using scikit-learn in Node.js.
Example 1: Predictive Model with scikit-learn in Node.js
const { PythonShell } = require('python-shell'); // Specify the Python script to execute const scriptPath = 'path/to/your/python_script.py'; // Specify the input data const inputData = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]; const inputFeatures = [[0, 0, 0], [1, 1, 1]]; // Set up the Python shell const options = { mode: 'text', pythonPath: 'python', pythonOptions: ['-u'], scriptPath: scriptPath, args: [JSON.stringify(inputData), JSON.stringify(inputFeatures)], }; // Execute the Python script PythonShell.run('your_python_script.py', options, (err, result) => { if (err) throw err; console.log(result); });
In this example, we use the PythonShell
module in Node.js to execute a Python script that builds a predictive model using scikit-learn. We specify the path to the Python script, the input data, and the input features. We then set up the Python shell with the necessary options and execute the Python script. The result is logged to the console.
Example 2: Predictive Model with TensorFlow.js in Node.js
const tf = require('@tensorflow/tfjs'); // Define the model architecture const model = tf.sequential(); model.add(tf.layers.dense({ units: 10, inputShape: [5], activation: 'relu' })); model.add(tf.layers.dense({ units: 1, activation: 'sigmoid' })); // Compile the model model.compile({ optimizer: 'adam', loss: 'binaryCrossentropy', metrics: ['accuracy'] }); // Generate random training data const x = tf.randomNormal([100, 5]); const y = tf.randomUniform([100, 1]); // Train the model model.fit(x, y, { epochs: 10 }).then(() => { // Use the model to make predictions const testInput = tf.randomNormal([10, 5]); const predictions = model.predict(testInput); predictions.print(); });
In this example, we use TensorFlow.js to build a predictive model in Node.js. We define a simple model architecture with two dense layers, compile the model with an optimizer and loss function, and generate random training data. We then train the model for a specified number of epochs and use the trained model to make predictions on test data.
These examples demonstrate how libraries like scikit-learn and TensorFlow.js can be used to build predictive models in Node.js, enabling developers to make accurate predictions and gain insights from their data.
Advantages of Using Open-source Libraries for NLP in AI
When it comes to implementing natural language processing (NLP) functionalities in AI, open-source libraries provide several advantages. These libraries offer pre-built functionality, optimized algorithms, and a community of developers contributing to their improvement and maintenance. Let’s explore some of the key advantages of using open-source libraries for NLP in AI.
1. Time and Effort Savings: Open-source libraries for NLP provide pre-built functionality, such as tokenization, part-of-speech tagging, sentiment analysis, and more. These libraries abstract away the complexities of NLP algorithms, allowing developers to focus on the higher-level implementation details of their AI applications. By leveraging these libraries, developers can save time and effort that would otherwise be spent on implementing NLP functionalities from scratch.
2. Performance and Scalability: Open-source libraries are often developed and optimized by a community of contributors. These libraries undergo rigorous testing and optimization to ensure high performance and scalability. By using open-source libraries for NLP, developers can leverage the expertise and efforts of the community to build high-performance AI applications that can handle large datasets and real-time processing.
3. Flexibility and Customization: Open-source libraries for NLP provide a wide range of functionalities and options, allowing developers to customize and fine-tune their AI models based on their specific requirements. These libraries often provide extensive documentation, tutorials, and examples that guide developers through the process of implementing and customizing NLP functionalities. This flexibility enables developers to build AI applications that are tailored to their unique use cases.
4. Community Support and Collaboration: Open-source libraries foster a community of developers who contribute to their improvement and maintenance. These communities provide support, answer questions, and share best practices, enabling developers to learn from others and collaborate on solving common challenges. By being part of an active community, developers can stay up-to-date with the latest advancements in NLP and benefit from the collective knowledge and experience of the community.
5. Integration and Compatibility: Open-source libraries for NLP are often designed to work seamlessly with other libraries and frameworks, enabling developers to integrate them into their existing AI pipelines and workflows. These libraries provide compatibility with popular programming languages and platforms, ensuring that developers can leverage their existing tools and technologies without having to make significant changes to their stack.
Overall, using open-source libraries for NLP in AI provides developers with a solid foundation to build useful and efficient AI applications. These libraries offer time and effort savings, high performance and scalability, flexibility and customization, community support and collaboration, as well as integration and compatibility with existing tools and technologies.
Related Article: How To Upgrade Node.js To The Latest Version
Limitations of TensorFlow.js for Machine Learning in Node.js
While TensorFlow.js is a useful library that brings the capabilities of TensorFlow to JavaScript and Node.js, it does have some limitations when it comes to machine learning in Node.js. Let’s explore some of the key limitations of TensorFlow.js.
1. Limited Model Support: TensorFlow.js supports a subset of the models and functionalities available in TensorFlow. While it provides support for common tasks like image classification, object detection, and natural language processing, it may not have the same level of support for more specialized models or advanced features. Developers working on complex machine learning tasks may need to consider using the Python version of TensorFlow for access to the full range of models and functionalities.
2. Performance Considerations: Although TensorFlow.js leverages the GPU capabilities of modern web browsers and hardware accelerators, the performance of machine learning models in the browser may not be on par with native implementations. The limited computational power of the browser environment and the overhead of running JavaScript code can impact the performance and scalability of machine learning models. Developers should carefully evaluate the performance requirements of their applications and consider using server-side TensorFlow or other frameworks for computationally intensive tasks.
3. Dependency on Web Technologies: TensorFlow.js relies on web technologies like WebGL and WebAssembly to run machine learning models in the browser. While this enables cross-platform compatibility and seamless integration with web applications, it also introduces dependencies on specific browser versions and support for these technologies. This can limit the deployment options and compatibility of TensorFlow.js models, especially in environments where web technologies are not widely supported or available.
4. Learning Curve and Ecosystem Maturity: TensorFlow.js is relatively new compared to its Python counterpart, TensorFlow. As a result, the ecosystem and community support for TensorFlow.js may not be as mature or extensive. Developers may encounter a steeper learning curve and fewer resources, tutorials, and examples compared to Python-based machine learning frameworks. However, the TensorFlow.js community is growing rapidly, and more resources and support are becoming available over time.
Despite these limitations, TensorFlow.js remains a valuable tool for machine learning in Node.js, especially for web-based applications and scenarios where cross-platform compatibility is essential. Developers should carefully consider their specific use cases, performance requirements, and the available ecosystem before deciding to use TensorFlow.js for their machine learning projects.
Using JavaScript for Data Preprocessing in AI
Data preprocessing is a critical step in AI implementations, as the quality and structure of the input data directly impact the performance and accuracy of machine learning models. JavaScript, with its rich ecosystem of libraries and frameworks, can be effectively used for data preprocessing tasks in AI. Let’s explore how JavaScript can be used for data preprocessing in AI and look at some examples.
1. Data Cleaning: JavaScript provides useful string manipulation and regular expression capabilities, making it well-suited for data cleaning tasks. Using JavaScript, developers can remove or replace unwanted characters, handle missing values, normalize data formats, and perform other data cleaning operations. Here’s an example of data cleaning using JavaScript:
const data = ['1,John,Doe', '2,Jane,Smith', '3,Bob,Johnson']; // Remove commas and split into individual values const cleanedData = data.map((entry) => entry.replace(',', '').split(',')); console.log(cleanedData);
In this example, we have an array of strings representing CSV data. We use JavaScript’s map
function to iterate over each entry, remove the commas using the replace
method, and split the string into individual values using the split
method. The cleaned data is then logged to the console.
2. Data Transformation: JavaScript provides useful array manipulation capabilities, allowing developers to transform data structures easily. Using JavaScript, developers can reshape arrays, apply mathematical operations, aggregate data, and perform other data transformation tasks. Here’s an example of data transformation using JavaScript:
const data = [[1, 2], [3, 4], [5, 6]]; // Transpose the data matrix const transposedData = data[0].map((col, i) => data.map(row => row[i])); console.log(transposedData);
In this example, we have a 2D array representing a data matrix. We use JavaScript’s map
function to iterate over each column index, and for each column, we use another map
function to iterate over each row and extract the corresponding element. The transposed data matrix is then logged to the console.
3. Data Normalization: JavaScript provides mathematical functions and libraries that enable developers to normalize data easily. Using JavaScript, developers can scale data to a specific range, standardize data using mean and standard deviation, and perform other data normalization operations. Here’s an example of data normalization using JavaScript:
const data = [1, 2, 3, 4, 5]; // Scale the data to the range [0, 1] const normalizedData = data.map((value) => (value - Math.min(...data)) / (Math.max(...data) - Math.min(...data))); console.log(normalizedData);
In this example, we have an array of numerical values. We use JavaScript’s map
function to iterate over each value, subtract the minimum value from each value, divide by the range (maximum value minus minimum value), and obtain the normalized value. The normalized data is then logged to the console.
These examples demonstrate how JavaScript can be used for data preprocessing tasks in AI, including data cleaning, transformation, and normalization. JavaScript’s array manipulation capabilities, mathematical functions, and string manipulation capabilities make it a useful tool for preparing data for machine learning models.
Best Practices for Implementing AI in Node.js
Implementing AI in Node.js requires careful consideration of best practices to ensure efficient and effective development. Let’s explore some best practices that developers should follow when implementing AI in Node.js.
1. Choose the Right Libraries and Frameworks: Selecting the right libraries and frameworks is crucial for successful AI implementations in Node.js. Consider factors like community support, documentation, performance, and compatibility when choosing libraries and frameworks. It’s also important to evaluate the specific requirements of your AI project and choose libraries and frameworks that address those requirements effectively.
2. Use Asynchronous Programming: Node.js is well-known for its asynchronous nature and event-driven programming model. When implementing AI in Node.js, leverage asynchronous programming techniques to handle large datasets, real-time processing, and long-running computations. This ensures that your AI applications remain responsive and scalable, even when dealing with computationally intensive tasks.
3. Optimize Performance: Performance optimization is critical for AI implementations, especially when dealing with large datasets and complex models. Utilize techniques like caching, parallel processing, and efficient memory management to optimize the performance of your AI applications. Consider using tools like profilers and performance monitoring systems to identify bottlenecks and fine-tune your code for optimal performance.
4. Ensure Data Quality and Integrity: Data plays a crucial role in AI implementations. Ensure that your data is of high quality, properly labeled, and representative of the problem you are trying to solve. Implement data validation and data cleansing techniques to identify and handle missing values, outliers, and other data quality issues. It’s also important to implement data integrity checks to ensure that your AI models are trained on accurate and reliable data.
5. Implement Model Versioning and Deployment: As your AI models evolve and improve, it’s essential to implement proper versioning and deployment strategies. Use version control systems to track changes to your models and ensure reproducibility. Implement deployment pipelines that automate the process of deploying new versions of your models to production environments, ensuring that your AI applications always use the latest and most accurate models.
6. Regularly Update and Maintain Dependencies: Node.js has a vast ecosystem of libraries and dependencies. Regularly update your dependencies to ensure that you are using the latest versions with bug fixes, security patches, and performance improvements. However, be cautious when updating dependencies, as breaking changes or incompatibilities can occur. Test thoroughly after updating dependencies to ensure that your AI applications continue to function as expected.
7. Monitor and Evaluate Model Performance: Implement monitoring and evaluation mechanisms to assess the performance of your AI models in real-world scenarios. Monitor key metrics like accuracy, precision, recall, and F1 score, and use techniques like A/B testing to compare different versions of your models. Continuously evaluate and fine-tune your models based on real-world feedback to ensure that they remain accurate and effective.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Additional Resources
– Getting Started with TensorFlow.js in Node.js
– TensorFlow.js GitHub Repository
– Natural Language Processing in Node.js with Natural