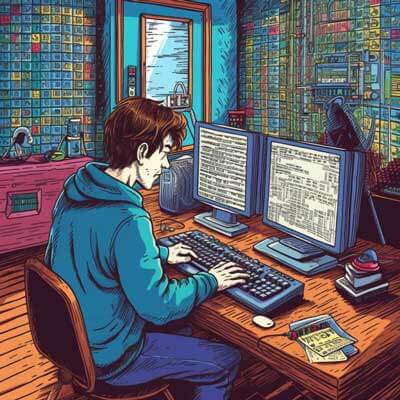
- Real-time data processing
- Data streaming
- Data ingestion
- Distributed data processing
- Data integration
- Data pipeline
- Data scalability
- Data analytics
- Data transformation
- Data storage
- How to process real-time data using Node.js
- Difference between batch processing and stream processing
- Efficiently handling large datasets in Node.js
- Integration of Node.js with other big data processing tools
- Benefits of using Hadoop with Node.js for big data processing
- Storing and managing large datasets in Node.js
- Techniques for transforming and aggregating data in Node.js
Real-time data processing
Real-time data processing refers to the ability to process and analyze data as it is generated, providing immediate insights and actions based on the incoming data. In the context of big data applications, real-time data processing becomes crucial for handling large volumes of data in a timely manner.
Node.js provides a useful platform for real-time data processing. Its event-driven, non-blocking I/O model allows for efficient handling of concurrent requests, making it a suitable choice for processing real-time data streams. By leveraging the event-driven nature of Node.js, developers can build applications that can handle large data streams and process them in real-time.
Here’s an example of how you can process real-time data using Node.js:
const http = require('http'); const server = http.createServer((req, res) => { // Process incoming request data req.on('data', (chunk) => { // Perform data processing operations console.log(chunk.toString()); }); // Send response to the client res.end('Data processed successfully'); }); server.listen(3000, () => { console.log('Server listening on port 3000'); });
In this example, we create an HTTP server using Node.js and process incoming request data by attaching an event listener to the ‘data’ event. As data is received, it is processed in real-time. This allows for efficient handling of large volumes of incoming data.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Data streaming
Data streaming is a technique used to process and transmit data in a continuous flow rather than in discrete chunks. It enables the processing of large datasets by breaking them down into smaller, more manageable chunks. Streaming data allows for real-time analytics, as the data is processed as it is received.
Node.js provides various libraries and frameworks that facilitate data streaming. One such library is the ‘stream’ module, which provides a set of API for creating and working with streams in Node.js.
Here’s an example of how you can create a readable stream in Node.js:
const fs = require('fs'); const readableStream = fs.createReadStream('data.txt'); readableStream.on('data', (chunk) => { // Process the data chunk console.log(chunk.toString()); }); readableStream.on('end', () => { console.log('Stream ended'); });
In this example, we create a readable stream using the ‘createReadStream’ method from the ‘fs’ module. As data is read from the file ‘data.txt’, the ‘data’ event is triggered, allowing us to process the data chunk by attaching an event listener. Once all the data has been read, the ‘end’ event is triggered, indicating that the stream has ended.
Data ingestion
Data ingestion refers to the process of collecting and importing data from various sources into a system for further processing and analysis. In the context of big data applications, data ingestion is a critical step in handling large datasets efficiently.
Node.js provides several libraries and frameworks that facilitate data ingestion. One such library is ‘kafka-node’, which allows for easy integration with Apache Kafka, a widely used distributed streaming platform.
Here’s an example of how you can ingest data into Apache Kafka using Node.js:
const kafka = require('kafka-node'); const Producer = kafka.Producer; const client = new kafka.KafkaClient({ kafkaHost: 'localhost:9092' }); const producer = new Producer(client); producer.on('ready', () => { const payloads = [ { topic: 'my-topic', messages: 'Hello, Kafka!', }, ]; producer.send(payloads, (err, data) => { if (err) { console.error('Error sending message:', err); } else { console.log('Message sent:', data); } }); }); producer.on('error', (err) => { console.error('Error:', err); });
In this example, we create a Kafka producer using the ‘kafka-node’ library. The producer is configured to connect to a Kafka broker running on ‘localhost:9092’. We then send a message to the ‘my-topic’ topic using the ‘send’ method. The ‘ready’ and ‘error’ events are used to handle the readiness and error states of the producer.
Distributed data processing
Distributed data processing refers to the ability to process large datasets across multiple machines or nodes in a distributed system. It allows for parallel processing and improved performance by leveraging the computing power of multiple machines.
Node.js provides several libraries and frameworks that facilitate distributed data processing. One such framework is ‘Apache Hadoop’, an open-source framework that allows for the distributed processing of large datasets across clusters of computers using a simple programming model.
Here’s an example of how you can use Node.js with Apache Hadoop for distributed data processing:
const hadoop = require('hadoop-client'); const job = hadoop.job('wordcount') job.input('hdfs://localhost:9000/input') .output('hdfs://localhost:9000/output') .mapper('wordcount-mapper.js') .reducer('wordcount-reducer.js') .run((err, result) => { if (err) { console.error('Error running job:', err); } else { console.log('Job completed successfully:', result); } });
In this example, we use the ‘hadoop-client’ library to create a Hadoop job for word count. We specify the input and output paths in HDFS (Hadoop Distributed File System) and provide the mapper and reducer scripts. The ‘run’ method is used to execute the job, and the result is logged to the console.
Related Article: How To Use Loop Inside React JSX
Data integration
Data integration refers to the process of combining data from different sources into a unified format for analysis and processing. In the context of big data applications, data integration becomes crucial for handling large volumes of data from diverse sources.
Node.js provides several libraries and frameworks that facilitate data integration. One such library is ‘Apache Kafka’, a distributed streaming platform that allows for the ingestion, storage, and processing of large volumes of data in real-time.
Here’s an example of how you can integrate Node.js with Apache Kafka for data streaming:
const kafka = require('kafka-node'); const Consumer = kafka.Consumer; const client = new kafka.KafkaClient({ kafkaHost: 'localhost:9092' }); const consumer = new Consumer(client, [{ topic: 'my-topic' }]); consumer.on('message', (message) => { console.log('Received message:', message); }); consumer.on('error', (err) => { console.error('Error:', err); });
In this example, we create a Kafka consumer using the ‘kafka-node’ library. The consumer is configured to connect to a Kafka broker running on ‘localhost:9092’ and subscribe to the ‘my-topic’ topic. As messages are received from the topic, the ‘message’ event is triggered, allowing us to process the messages. The ‘error’ event is used to handle any errors that may occur during the consumption process.
Data pipeline
A data pipeline refers to a series of processes and operations that transform and move data from one system to another. In the context of big data applications, data pipelines are used to handle large volumes of data efficiently and ensure the smooth flow of data between different stages of processing.
Node.js provides several libraries and frameworks that facilitate the creation of data pipelines. One such library is ‘Node-RED’, a visual tool for wiring together hardware devices, APIs, and online services in new and interesting ways.
Here’s an example of how you can create a data pipeline using Node-RED:
// Example code snippet for a Node-RED flow [ { "id": "1d9d9f8a.e20a1c", "type": "http in", "z": "a7a0b1c2.3f4d5e", "name": "HTTP Input", "url": "/data", "method": "post", "swaggerDoc": "", "x": 110, "y": 140, "wires": [["770b8e3d.6f7efc"]] }, { "id": "770b8e3d.6f7efc", "type": "function", "z": "a7a0b1c2.3f4d5e", "name": "Data Transformation", "func": "msg.payload = msg.payload.toUpperCase();\nreturn msg;", "outputs": 1, "noerr": 0, "x": 330, "y": 140, "wires": [["4f3f5fd6.7a9d3"]] }, { "id": "4f3f5fd6.7a9d3", "type": "http response", "z": "a7a0b1c2.3f4d5e", "name": "HTTP Response", "statusCode": "", "headers": {}, "x": 540, "y": 140, "wires": [] } ]
In this example, we use Node-RED to create a simple data pipeline. The flow consists of an HTTP input node that receives data via an HTTP POST request. The data is then passed to a function node, where it is transformed (in this case, converted to uppercase). Finally, the transformed data is sent back as an HTTP response.
Data scalability
Data scalability refers to the ability to handle increasing amounts of data without sacrificing performance or quality. In the context of big data applications, data scalability becomes crucial for handling large volumes of data efficiently and ensuring that the system can scale to meet the growing demands of data processing.
Node.js provides several techniques and best practices for achieving data scalability. One such technique is the use of a distributed data storage system, such as Apache Cassandra, which allows for the distributed storage and retrieval of large volumes of data across multiple nodes.
Here’s an example of how you can use Node.js with Apache Cassandra for data storage:
const cassandra = require('cassandra-driver'); const client = new cassandra.Client({ contactPoints: ['localhost'], localDataCenter: 'datacenter1' }); const query = 'SELECT * FROM my_table WHERE id = ?'; const params = ['123']; client.execute(query, params, { prepare: true }) .then(result => { console.log('Data retrieved:', result.rows); }) .catch(err => { console.error('Error retrieving data:', err); });
In this example, we create a Cassandra client using the ‘cassandra-driver’ library. The client is configured to connect to a Cassandra cluster running on ‘localhost’ and uses the ‘datacenter1’ data center for local data distribution. We then execute a SELECT query to retrieve data from the ‘my_table’ table, passing the query parameters and options as needed. The result is logged to the console.
Related Article: How To Upgrade Node.js To The Latest Version
Data analytics
Data analytics refers to the process of analyzing and extracting meaningful insights from data. In the context of big data applications, data analytics becomes crucial for understanding patterns, trends, and correlations within large volumes of data.
Node.js provides several libraries and frameworks that facilitate data analytics. One such library is ‘Pandas’, a useful data manipulation and analysis library for Python. While not native to Node.js, it can be used alongside Node.js through child processes or API calls.
Here’s an example of how you can use Node.js with Pandas for data analytics:
const { spawn } = require('child_process'); const scriptPath = 'analytics.py'; const data = [1, 2, 3, 4, 5]; const process = spawn('python', [scriptPath, JSON.stringify(data)]); process.stdout.on('data', (data) => { const result = JSON.parse(data.toString()); console.log('Analytics result:', result); }); process.stderr.on('data', (data) => { console.error('Error:', data.toString()); });
In this example, we spawn a child process to execute a Python script (‘analytics.py’) that performs data analytics using Pandas. We pass the data as a command-line argument, which is serialized as a JSON string. The script’s output is captured from the stdout stream and logged to the console. Any errors encountered during the execution are captured from the stderr stream and logged as well.
Data transformation
Data transformation refers to the process of converting data from one format or structure to another. In the context of big data applications, data transformation becomes crucial for preparing data for analysis, storage, or integration with other systems.
Node.js provides several libraries and frameworks that facilitate data transformation. One such library is ‘lodash’, a utility library that provides a wide range of functions for manipulating and transforming data.
Here’s an example of how you can use Node.js with lodash for data transformation:
const _ = require('lodash'); const data = [ { id: 1, name: 'John Doe', age: 30 }, { id: 2, name: 'Jane Smith', age: 25 }, { id: 3, name: 'Bob Johnson', age: 35 }, ]; const transformedData = _.map(data, (item) => ({ ...item, fullName: `${item.name} (${item.age})`, })); console.log('Transformed data:', transformedData);
In this example, we use lodash’s ‘map’ function to transform an array of objects. Each object in the array is transformed by adding a ‘fullName’ property, which combines the ‘name’ and ‘age’ properties. The transformed data is then logged to the console.
Data storage
Data storage refers to the process of storing and managing data in a structured manner for future use. In the context of big data applications, data storage becomes crucial for handling large volumes of data efficiently and ensuring data durability and availability.
Node.js provides several libraries and frameworks that facilitate data storage. One such library is ‘MongoDB’, a popular NoSQL database that allows for the storage and retrieval of large volumes of data in a flexible, schema-less format.
Here’s an example of how you can use Node.js with MongoDB for data storage:
const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true }); const schema = new mongoose.Schema({ name: String, age: Number, }); const Model = mongoose.model('MyModel', schema); const data = [ { name: 'John Doe', age: 30 }, { name: 'Jane Smith', age: 25 }, { name: 'Bob Johnson', age: 35 }, ]; Model.insertMany(data) .then(() => { console.log('Data stored successfully'); }) .catch((err) => { console.error('Error storing data:', err); });
In this example, we use the ‘mongoose’ library to connect to a MongoDB database running on ‘localhost:27017’. We define a schema for our data using the ‘Schema’ class and create a model using the ‘model’ method. We then insert an array of data into the database using the ‘insertMany’ method. The success or failure of the operation is logged to the console.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
How to process real-time data using Node.js
Processing real-time data using Node.js involves handling incoming data streams and performing operations on the data as it is received. Node.js provides an event-driven, non-blocking I/O model that is well-suited for processing real-time data efficiently.
Here’s an example of how you can process real-time data using Node.js:
const http = require('http'); const server = http.createServer((req, res) => { // Process incoming request data req.on('data', (chunk) => { // Perform data processing operations console.log(chunk.toString()); }); // Send response to the client res.end('Data processed successfully'); }); server.listen(3000, () => { console.log('Server listening on port 3000'); });
In this example, we create an HTTP server using Node.js and attach an event listener to the ‘data’ event of the incoming request. As data is received, it is processed in real-time. The processed data can then be used to perform further operations, such as storing it in a database or sending it to another system. Finally, a response is sent back to the client to acknowledge the successful processing of the data.
Difference between batch processing and stream processing
Batch processing and stream processing are two different approaches to data processing, each with its own advantages and use cases.
Batch processing involves processing data in fixed-sized batches or chunks. The data is collected over a period of time and processed together as a batch. Batch processing is usually performed on static or historical data and is well-suited for tasks that require complex computations or data analysis.
Stream processing, on the other hand, involves processing data in real-time as it is received. The data is processed as a continuous stream, allowing for immediate insights and actions based on the incoming data. Stream processing is well-suited for tasks that require real-time analytics, monitoring, or alerting.
Here’s an example to illustrate the difference between batch processing and stream processing:
Batch processing example:
const data = [1, 2, 3, 4, 5]; // Process the data as a batch const result = data.map((item) => item * 2); console.log('Batch processing result:', result);
In this example, we have an array of data that we want to process. We apply a transformation to each item in the array using the ‘map’ method and store the result in a new array. The processing is performed on the entire array at once, treating it as a batch.
Stream processing example:
const dataStream = [1, 2, 3, 4, 5]; // Process the data as a stream dataStream.forEach((item) => { const result = item * 2; console.log('Stream processing result:', result); });
In this example, we have a data stream represented as an array. We iterate over each item in the array using the ‘forEach’ method and apply a transformation to each item. The processing is performed in real-time as each item is received, treating it as a stream.
The choice between batch processing and stream processing depends on the specific requirements of the application. Batch processing is suitable for tasks that can tolerate some delay in processing, while stream processing is suitable for tasks that require immediate insights or actions based on real-time data.
Efficiently handling large datasets in Node.js
Handling large datasets efficiently in Node.js involves adopting techniques and best practices that optimize memory usage and processing speed. By leveraging the asynchronous, non-blocking nature of Node.js, developers can design applications that can efficiently handle large volumes of data.
Here are some techniques for efficiently handling large datasets in Node.js:
1. Use streams: Stream processing allows for the efficient handling of large datasets by breaking them down into smaller, more manageable chunks. Node.js provides a built-in ‘stream’ module that allows for the creation and manipulation of streams.
Example:
const fs = require('fs'); const readableStream = fs.createReadStream('large-file.txt'); const writableStream = fs.createWriteStream('output-file.txt'); readableStream.pipe(writableStream);
In this example, we create a readable stream from a large file and a writable stream to an output file. We then use the ‘pipe’ method to connect the two streams, allowing for efficient data transfer without buffering the entire file in memory.
2. Use batching: When processing large datasets, it’s often more efficient to process the data in smaller batches rather than all at once. Batching allows for better memory management and reduces the risk of running out of memory.
Example:
const batchSize = 100; const data = [/* large array of data */]; for (let i = 0; i { console.log('Result:', result); }); worker.on('error', (error) => { console.error('Error:', error); }); worker.on('exit', (code) => { console.log('Worker exited with code:', code); });
In this example, we create a worker thread using the ‘Worker’ class from the ‘worker_threads’ module. The worker thread executes a separate JavaScript file (‘compute.js’) that performs computationally intensive tasks. The main thread can continue processing other tasks while the worker thread is busy. Communication between the main thread and the worker thread is done via message passing.
Related Article: How To Fix Javascript: $ Is Not Defined
Integration of Node.js with other big data processing tools
Node.js can be integrated with other big data processing tools to leverage their capabilities and enhance the functionality of the application. By combining the strengths of different tools, developers can build useful and scalable big data applications.
One such tool is Apache Kafka, a distributed streaming platform that allows for the ingestion, storage, and processing of large volumes of data in real-time. Node.js provides several libraries for integrating with Apache Kafka, such as ‘kafka-node’ and ‘node-rdkafka’.
Here’s an example of how you can integrate Node.js with Apache Kafka using the ‘kafka-node’ library:
const kafka = require('kafka-node'); const Consumer = kafka.Consumer; const client = new kafka.KafkaClient({ kafkaHost: 'localhost:9092' }); const consumer = new Consumer(client, [{ topic: 'my-topic' }]); consumer.on('message', (message) => { console.log('Received message:', message); }); consumer.on('error', (err) => { console.error('Error:', err); });
In this example, we create a Kafka consumer using the ‘kafka-node’ library. The consumer is configured to connect to a Kafka broker running on ‘localhost:9092’ and subscribe to the ‘my-topic’ topic. As messages are received from the topic, the ‘message’ event is triggered, allowing us to process the messages. The ‘error’ event is used to handle any errors that may occur during the consumption process.
Benefits of using Hadoop with Node.js for big data processing
Using Hadoop with Node.js for big data processing offers several benefits, including:
1. Scalability: Hadoop is designed to scale horizontally, allowing for the distributed processing of large datasets across multiple machines or nodes. By leveraging the scalability of Hadoop, developers can handle ever-increasing volumes of data without sacrificing performance.
2. Fault tolerance: Hadoop provides built-in fault tolerance mechanisms, such as data replication and automatic failover. This ensures that data processing can continue uninterrupted even in the presence of hardware or software failures.
3. Data locality: Hadoop optimizes data processing by moving computation closer to the data. This reduces network overhead and improves performance by minimizing data transfer across the network.
4. Ecosystem integration: Hadoop has a rich ecosystem of tools and frameworks that can be used in conjunction with Node.js for various big data processing tasks. For example, tools like Apache Hive and Apache Pig provide high-level query languages and data transformation capabilities, while Apache Spark offers in-memory processing and real-time analytics.
5. Flexibility: Node.js provides a lightweight and flexible runtime environment for running JavaScript applications. By combining the scalability and fault tolerance of Hadoop with the flexibility of Node.js, developers can build useful and adaptable big data processing pipelines.
6. Developer productivity: Node.js has a large and vibrant community, with a wide range of libraries and frameworks available for various tasks. This ecosystem makes it easier for developers to build, test, and deploy big data applications, reducing development time and improving productivity.
Storing and managing large datasets in Node.js
Storing and managing large datasets in Node.js requires efficient and scalable data storage solutions. Node.js provides several libraries and frameworks that facilitate data storage, such as MongoDB, PostgreSQL, and Redis.
Here’s an example of how you can use Node.js with MongoDB for storing and managing large datasets:
const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true }); const schema = new mongoose.Schema({ name: String, age: Number, }); const Model = mongoose.model('MyModel', schema); const data = [ { name: 'John Doe', age: 30 }, { name: 'Jane Smith', age: 25 }, { name: 'Bob Johnson', age: 35 }, ]; Model.insertMany(data) .then(() => { console.log('Data stored successfully'); }) .catch((err) => { console.error('Error storing data:', err); });
In this example, we use the ‘mongoose’ library to connect to a MongoDB database running on ‘localhost:27017’. We define a schema for our data using the ‘Schema’ class and create a model using the ‘model’ method. We then insert an array of data into the database using the ‘insertMany’ method. The success or failure of the operation is logged to the console.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
Techniques for transforming and aggregating data in Node.js
Transforming and aggregating data in Node.js involves manipulating and combining data to derive meaningful insights or create new data structures. Node.js provides several techniques and libraries that facilitate data transformation and aggregation, such as ‘lodash’, ‘Ramda’, and the built-in ‘Array’ methods.
Here’s an example of how you can use the ‘lodash’ library to transform and aggregate data in Node.js:
const _ = require('lodash'); const data = [ { id: 1, name: 'John', age: 30 }, { id: 2, name: 'Jane', age: 25 }, { id: 3, name: 'Bob', age: 35 }, ]; // Transform data const transformedData = _.map(data, (item) => ({ ...item, fullName: `${item.name} Doe`, })); console.log('Transformed data:', transformedData); // Aggregate data const totalAge = _.sumBy(data, 'age'); console.log('Total age:', totalAge);
In this example, we use the ‘lodash’ library to transform and aggregate data. We use the ‘map’ function to transform each object in the ‘data’ array by adding a ‘fullName’ property. We then use the ‘sumBy’ function to aggregate the ‘age’ property of each object in the ‘data’ array.