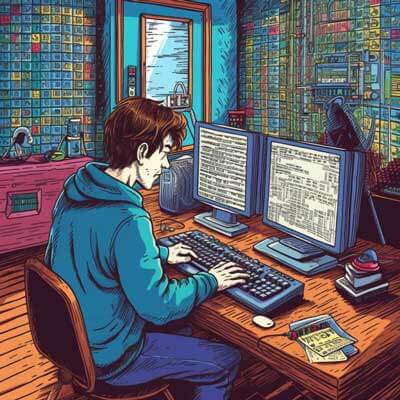
- SQL Between Dates in MySQL
- Introduction
- Example 1: Retrieving Data within a Date Range
- Example 2: Combining BETWEEN and Other Conditions
- SQL Between Dates in PostgreSQL
- Introduction
- Example 1: Retrieving Data within a Date Range
- Example 2: Combining BETWEEN and Other Conditions
- Date Queries in MySQL
- Introduction
- Example 1: Extracting Year, Month, and Day
- Example 2: Calculating Date Differences
- Date Queries in PostgreSQL
- Introduction
- Example 1: Extracting Year, Month, and Day
- Example 2: Calculating Date Differences
- Use Cases: SQL Between Date Ranges
- Introduction
- Example 1: Sales Analysis
- Example 2: Event Attendance
- Best Practices: SQL Between Date Ranges
- Introduction
- Best Practice 1: Indexing Date Columns
- Best Practice 2: Avoid Casting or Converting Dates
- Real World Examples: SQL Between Date Ranges
- Introduction
- Example 1: Hotel Room Availability
- Example 2: Stock Price Analysis
- Performance Considerations: SQL Between Date Ranges
- Introduction
- Consideration 1: Indexing
- Consideration 2: Query Optimization
- Advanced Technique 1: SQL Between Date Ranges
- Introduction
- Example: Retrieving Weekday Data
- Advanced Technique 2: SQL Between Date Ranges
- Introduction
- Example: Retrieving Latest Orders
- Code Snippet 1: SQL Between Date Ranges
- Code Snippet 2: SQL Between Date Ranges
- Code Snippet 3: SQL Between Date Ranges
- Code Snippet 4: SQL Between Date Ranges
- Code Snippet 5: SQL Between Date Ranges
- Error Handling: SQL Between Date Ranges
- Introduction
- Error: Incorrect Date Format
- Error: Invalid Date Range
- Error: Null Date Values
SQL Between Dates in MySQL
Related Article: Processing MySQL Queries in PHP: A Detailed Guide
Introduction
In this chapter, we will explore how to use the SQL BETWEEN operator to query data within a specific date range in MySQL. The BETWEEN operator allows us to retrieve records that fall within a given range, inclusive of the start and end dates.
Example 1: Retrieving Data within a Date Range
To illustrate the usage of the SQL BETWEEN operator, let’s consider a scenario where we have a table named “orders” with the following structure:
CREATE TABLE orders ( id INT, order_date DATE );
Suppose we want to retrieve all orders placed between January 1, 2022, and February 28, 2022. We can use the BETWEEN operator as follows:
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28';
This query will return all the orders that were placed within the specified date range.
Example 2: Combining BETWEEN and Other Conditions
In addition to specifying a date range, we can also include other conditions in our query. For instance, let’s say we want to retrieve orders placed between January 1, 2022, and February 28, 2022, but only for customers with a specific ID. We can modify our query as follows:
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28' AND customer_id = 123;
This query will return orders that meet both the date range condition and the customer ID condition.
Related Article: How to Perform a Full Outer Join in MySQL
SQL Between Dates in PostgreSQL
Introduction
In this chapter, we will explore how to use the SQL BETWEEN operator to query data within a specific date range in PostgreSQL. The BETWEEN operator allows us to retrieve records that fall within a given range, inclusive of the start and end dates.
Example 1: Retrieving Data within a Date Range
To illustrate the usage of the SQL BETWEEN operator, let’s consider a scenario where we have a table named “orders” with the following structure:
CREATE TABLE orders ( id INT, order_date DATE );
Suppose we want to retrieve all orders placed between January 1, 2022, and February 28, 2022. We can use the BETWEEN operator as follows:
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28';
This query will return all the orders that were placed within the specified date range.
Related Article: How to Fix MySQL Error Code 1175 in Safe Update Mode
Example 2: Combining BETWEEN and Other Conditions
In addition to specifying a date range, we can also include other conditions in our query. For instance, let’s say we want to retrieve orders placed between January 1, 2022, and February 28, 2022, but only for customers with a specific ID. We can modify our query as follows:
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28' AND customer_id = 123;
This query will return orders that meet both the date range condition and the customer ID condition.
Date Queries in MySQL
Introduction
In this chapter, we will explore advanced date queries in MySQL. We will cover various techniques to manipulate and extract information from date values stored in MySQL tables.
Related Article: How to Update Records in MySQL with a Select Query
Example 1: Extracting Year, Month, and Day
MySQL provides several date functions that allow us to extract specific components from a date. For example, we can use the YEAR(), MONTH(), and DAY() functions to extract the year, month, and day from a date, respectively.
Consider a table named “orders” with an “order_date” column of type DATE. To extract the year, month, and day from the “order_date” column, we can use the following query:
SELECT YEAR(order_date) AS order_year, MONTH(order_date) AS order_month, DAY(order_date) AS order_day FROM orders;
This query will return the year, month, and day values for each order.
Example 2: Calculating Date Differences
MySQL also provides functions to calculate the difference between two dates. For instance, we can use the DATEDIFF() function to calculate the number of days between two dates.
Suppose we have a table named “employees” with columns “hire_date” and “termination_date”. To calculate the number of days an employee worked, we can use the following query:
SELECT employee_id, DATEDIFF(termination_date, hire_date) AS employment_days FROM employees;
This query will return the employee ID and the number of days between the hire date and termination date, representing their employment duration.
Date Queries in PostgreSQL
Related Article: How to Use MySQL Query String Contains
Introduction
In this chapter, we will explore advanced date queries in PostgreSQL. We will cover various techniques to manipulate and extract information from date values stored in PostgreSQL tables.
Example 1: Extracting Year, Month, and Day
PostgreSQL provides several date functions that allow us to extract specific components from a date. For example, we can use the EXTRACT() function to extract the year, month, and day from a date value.
Consider a table named “orders” with an “order_date” column of type DATE. To extract the year, month, and day from the “order_date” column, we can use the following query:
SELECT EXTRACT(YEAR FROM order_date) AS order_year, EXTRACT(MONTH FROM order_date) AS order_month, EXTRACT(DAY FROM order_date) AS order_day FROM orders;
This query will return the year, month, and day values for each order.
Example 2: Calculating Date Differences
PostgreSQL provides the AGE() function to calculate the difference between two dates. The AGE() function returns an interval representing the difference between two timestamps or dates.
Suppose we have a table named “employees” with columns “hire_date” and “termination_date”. To calculate the number of years an employee worked, we can use the following query:
SELECT employee_id, EXTRACT(YEAR FROM AGE(termination_date, hire_date)) AS employment_years FROM employees;
This query will return the employee ID and the number of years between the hire date and termination date, representing their employment duration.
Related Article: How to Resolve Secure File Priv in MySQL
Use Cases: SQL Between Date Ranges
Introduction
In this chapter, we will explore different use cases for using the SQL BETWEEN operator to query data within date ranges.
Example 1: Sales Analysis
Suppose we have a table named “sales” with columns “order_date” and “amount”. We want to analyze sales data within specific date ranges to gain insights into revenue trends.
To retrieve sales data between January 1, 2022, and February 28, 2022, we can use the following query:
SELECT SUM(amount) AS total_sales FROM sales WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28';
This query will return the total sales amount for the specified date range. We can apply similar queries to analyze sales data for different periods and compare the results.
Related Article: Securing MySQL Access through Bash Scripts in Linux
Example 2: Event Attendance
Consider a table named “events” with columns “event_date” and “attendance_count”. We want to track event attendance within specific date ranges to measure event popularity.
To retrieve the total attendance count for events between January 1, 2022, and February 28, 2022, we can use the following query:
SELECT SUM(attendance_count) AS total_attendance FROM events WHERE event_date BETWEEN '2022-01-01' AND '2022-02-28';
This query will return the total attendance count for the specified date range. By analyzing attendance data for different periods, we can identify patterns and make informed decisions regarding event planning.
Best Practices: SQL Between Date Ranges
Introduction
In this chapter, we will discuss best practices for using the SQL BETWEEN operator to query data within date ranges. Following these best practices can help improve query performance and ensure accurate results.
Related Article: Creating a Bash Script for a MySQL Database Backup
Best Practice 1: Indexing Date Columns
To optimize queries involving date ranges, it is recommended to create an index on the date column used in the BETWEEN operator. Indexing the date column allows the database to quickly locate records within the specified range, resulting in faster query execution.
For example, to create an index on the “order_date” column in the “orders” table, you can use the following SQL statement:
CREATE INDEX idx_order_date ON orders (order_date);
Best Practice 2: Avoid Casting or Converting Dates
When using the BETWEEN operator, it is best to avoid casting or converting dates unnecessarily. Performing operations on dates can impact query performance and introduce potential errors.
Instead, ensure that the date values in your query match the data type of the date column. This approach avoids unnecessary conversions and ensures accurate results.
Real World Examples: SQL Between Date Ranges
Related Article: Converting MySQL Query Results from True to Yes
Introduction
In this chapter, we will explore real-world examples of using the SQL BETWEEN operator to query data within date ranges. These examples demonstrate practical scenarios where date range queries are commonly used.
Example 1: Hotel Room Availability
Consider a hotel management system with a table named “room_availability” that tracks the availability of hotel rooms on specific dates. The table has columns “room_id”, “date”, and “available”.
To check room availability between two dates, we can use the following query:
SELECT room_id FROM room_availability WHERE date BETWEEN '2022-01-01' AND '2022-01-07' AND available = true;
This query will return the room IDs of available rooms between the specified dates. By adjusting the date range, we can retrieve room availability information for different periods.
Example 2: Stock Price Analysis
Suppose we have a table named “stock_prices” that stores daily stock prices for various companies. The table has columns “company_id”, “date”, and “price”.
To analyze the performance of a specific stock between two dates, we can use the following query:
SELECT * FROM stock_prices WHERE company_id = 123 AND date BETWEEN '2022-01-01' AND '2022-02-28';
This query will return the stock prices for the specified company within the specified date range. By analyzing the retrieved data, we can gain insights into the stock’s performance over time.
Related Article: How To Import a SQL File With a MySQL Command Line
Performance Considerations: SQL Between Date Ranges
Introduction
In this chapter, we will discuss performance considerations when using the SQL BETWEEN operator to query data within date ranges. By understanding these considerations, we can optimize our queries and improve overall performance.
Consideration 1: Indexing
As mentioned earlier, indexing the date column used in the BETWEEN operator can significantly improve query performance. By creating an index on the date column, the database can quickly locate records within the specified range.
However, it is important to note that excessive indexing can also impact performance. Only index the date columns that are frequently used in range queries to strike a balance between query performance and storage overhead.
Related Article: Tutorial on Database Sharding in MySQL
Consideration 2: Query Optimization
To further optimize queries involving date ranges, consider the following techniques:
– Limit the number of rows returned by applying additional conditions or filters.
– Use appropriate data types for date columns to ensure efficient storage and comparison.
– Minimize unnecessary calculations or conversions on date values within the query.
Advanced Technique 1: SQL Between Date Ranges
Introduction
In this chapter, we will explore an advanced technique for using the SQL BETWEEN operator to query data within date ranges. This technique involves combining the BETWEEN operator with other SQL functions to achieve more complex date range queries.
Related Article: Using Stored Procedures in MySQL
Example: Retrieving Weekday Data
Suppose we have a table named “sales” with columns “order_date” and “amount”. We want to retrieve sales data for weekdays only, excluding weekends.
To achieve this, we can use the DAYOFWEEK() function along with the BETWEEN operator. The DAYOFWEEK() function returns the weekday index for a given date, where Sunday is represented by 1 and Saturday by 7.
The following query retrieves sales data for weekdays between January 1, 2022, and February 28, 2022:
SELECT * FROM sales WHERE DAYOFWEEK(order_date) BETWEEN 2 AND 6 AND order_date BETWEEN '2022-01-01' AND '2022-02-28';
This query ensures that only weekday sales data within the specified date range is retrieved.
Advanced Technique 2: SQL Between Date Ranges
Introduction
In this chapter, we will explore another advanced technique for using the SQL BETWEEN operator to query data within date ranges. This technique involves using subqueries to further refine our date range queries.
Related Article: Tutorial: Working with SQL Data Types in MySQL
Example: Retrieving Latest Orders
Suppose we have a table named “orders” with columns “order_date” and “amount”. We want to retrieve the latest orders placed within a specific date range.
To achieve this, we can use a subquery to first retrieve the maximum order date within the desired range. We can then use this result to filter the main query and retrieve the latest orders.
The following query retrieves the latest orders placed between January 1, 2022, and February 28, 2022:
SELECT * FROM orders WHERE order_date = ( SELECT MAX(order_date) FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28' );
This query retrieves the orders with the maximum order date within the specified range, effectively giving us the latest orders.
Code Snippet 1: SQL Between Date Ranges
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28';
This code snippet demonstrates how to use the SQL BETWEEN operator to retrieve records within a specific date range in MySQL.
Code Snippet 2: SQL Between Date Ranges
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28' AND customer_id = 123;
This code snippet illustrates how to combine the SQL BETWEEN operator with other conditions to retrieve records within a date range and for a specific customer ID in MySQL.
Related Article: How to Insert Multiple Rows in a MySQL Database
Code Snippet 3: SQL Between Date Ranges
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28';
This code snippet demonstrates how to use the SQL BETWEEN operator to retrieve records within a specific date range in PostgreSQL.
Code Snippet 4: SQL Between Date Ranges
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28' AND customer_id = 123;
This code snippet illustrates how to combine the SQL BETWEEN operator with other conditions to retrieve records within a date range and for a specific customer ID in PostgreSQL.
Code Snippet 5: SQL Between Date Ranges
SELECT EXTRACT(YEAR FROM order_date) AS order_year, EXTRACT(MONTH FROM order_date) AS order_month, EXTRACT(DAY FROM order_date) AS order_day FROM orders;
This code snippet demonstrates how to use the EXTRACT() function in PostgreSQL to extract the year, month, and day from a date column in a table.
Error Handling: SQL Between Date Ranges
Introduction
In this chapter, we will discuss error handling when using the SQL BETWEEN operator to query data within date ranges. Understanding common errors and how to handle them can help ensure the reliability and accuracy of our queries.
Error: Incorrect Date Format
One common error when using the SQL BETWEEN operator is providing dates in an incorrect format. The date format must match the expected format in the database.
For example, if the database expects dates in the format ‘YYYY-MM-DD’, providing dates in a different format will result in an error. To resolve this error, ensure that the provided dates match the expected format.
Error: Invalid Date Range
Another common error is specifying an invalid date range. This can occur when the start date is later than the end date, resulting in an empty or incorrect result set.
To avoid this error, always double-check the specified date range and ensure that the start date is earlier than or equal to the end date.
Error: Null Date Values
When working with date columns, it is important to handle null values appropriately. Null date values can cause unexpected behavior or errors in queries that involve the SQL BETWEEN operator.
To handle null date values, consider adding an additional condition to exclude records with null dates from your queries. For example:
SELECT * FROM orders WHERE order_date BETWEEN '2022-01-01' AND '2022-02-28' AND order_date IS NOT NULL;
This query excludes records with null order dates from the result set.
Overall, it is crucial to validate and handle errors related to date formats, invalid date ranges, and null date values to ensure the correctness and reliability of our queries.