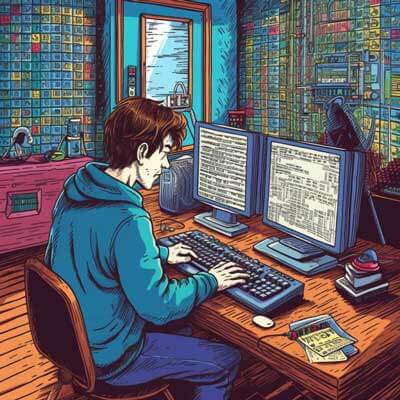
- The Importance of Indexes in Query Optimization
- Understanding Index Scans in PostgreSQL
- Determining if Your Query Uses an Index
- Analyzing the Execution Plan of a PostgreSQL Query
- Different Types of Indexes in PostgreSQL
- Tools for Determining Index Usage in PostgreSQL
- Best Practices for Optimizing Queries with Indexes
- Simultaneous Use of Multiple Indexes in a PostgreSQL Query
- Forcing PostgreSQL to Use a Specific Index for a Query
- Potential Drawbacks of Using Indexes in PostgreSQL
- Additional Resources
The Importance of Indexes in Query Optimization
Indexes play a crucial role in enhancing the performance of database queries. By organizing data in a specific manner, indexes allow the database management system to efficiently locate and retrieve the requested data. Without indexes, the database would have to scan through the entire table to find the relevant rows, resulting in slow and inefficient query execution.
Indexes are particularly useful when dealing with large datasets, as they significantly reduce the amount of data that needs to be examined. By creating indexes on frequently accessed columns, you can speed up query execution time and improve overall system performance.
Let’s consider an example to understand the importance of indexes in query optimization. Suppose we have a table called “employees” with millions of records, and we want to retrieve all employees with a specific job title. Without an index on the job title column, the database would have to scan through every record in the table to find the matching rows. However, if we create an index on the job title column, the database can quickly locate the relevant rows by traversing the index structure instead of scanning the entire table. This can result in a significant reduction in query execution time, especially when dealing with large datasets.
In PostgreSQL, indexes can be created on one or more columns of a table. There are different types of indexes available, such as B-tree, hash, GiST, GIN, and SP-GiST, each suitable for different types of queries and data structures. We will explore these different types of indexes in more detail later in this article.
Related Article: Detecting Optimization Issues in PostgreSQL Query Plans
Understanding Index Scans in PostgreSQL
To determine if a PostgreSQL query is utilizing an index, it is important to understand the different types of index scans that can occur during query execution. PostgreSQL employs various index scan methods based on the query’s conditions and the available indexes.
The most common index scan method is the B-tree index scan, which is used when querying with equality or range conditions on indexed columns. The B-tree index is the default index type in PostgreSQL and is suitable for most use cases. It organizes data in a balanced tree structure, allowing for efficient searching and retrieval of data.
Another index scan method is the bitmap index scan, which is used when multiple conditions are combined using logical operators (AND, OR) in the query. The bitmap index scan creates a bitmap of matching rows for each condition and performs a bitwise operation to determine the final set of rows that satisfy all conditions.
There are also specialized index scan methods like the hash index scan, GiST index scan, GIN index scan, and SP-GiST index scan. These index types are optimized for specific data structures and query patterns and are used in specific scenarios where they provide better query performance compared to B-tree index scans.
Now let’s look at some examples to illustrate the different types of index scans in PostgreSQL.
Example 1: B-tree Index Scan
Suppose we have a table called “customers” with the following schema:
CREATE TABLE customers ( id SERIAL PRIMARY KEY, name VARCHAR(100), age INT, city VARCHAR(100) );
We create a B-tree index on the “name” column:
CREATE INDEX idx_customers_name ON customers (name);
Now, let’s execute a query that retrieves all customers with the name “John”:
SELECT * FROM customers WHERE name = 'John';
During the execution of this query, PostgreSQL will perform a B-tree index scan on the “name” column. It will use the index to quickly locate the rows where the name is “John”, resulting in fast and efficient query execution.
Example 2: Bitmap Index Scan
Suppose we have a table called “orders” with the following schema:
CREATE TABLE orders ( id SERIAL PRIMARY KEY, customer_id INT, product_id INT, quantity INT, price DECIMAL(10, 2) );
We create a B-tree index on the “customer_id” column and another B-tree index on the “product_id” column:
CREATE INDEX idx_orders_customer_id ON orders (customer_id); CREATE INDEX idx_orders_product_id ON orders (product_id);
Now, let’s execute a query that retrieves all orders for a specific customer and a specific product:
SELECT * FROM orders WHERE customer_id = 123 AND product_id = 456;
During the execution of this query, PostgreSQL will perform a bitmap index scan on both the “customer_id” and “product_id” columns. It will create bitmaps for each condition and perform a bitwise AND operation to determine the final set of rows that satisfy both conditions. This allows PostgreSQL to efficiently retrieve the relevant rows without scanning the entire table.
Understanding the different types of index scans in PostgreSQL is essential to determine if a query is utilizing an index effectively. By analyzing the execution plan of a query, we can gain insights into the index scan methods employed and identify potential areas for optimization.
Determining if Your Query Uses an Index
To determine if a PostgreSQL query is utilizing an index, we can analyze the execution plan generated by the query planner. The execution plan describes the steps taken by the database engine to execute the query, including the index scan methods used.
PostgreSQL provides several ways to obtain the execution plan for a query. Let’s explore some of these methods:
1. EXPLAIN command:
The EXPLAIN command allows us to obtain the execution plan for a query without actually executing it. We can use the EXPLAIN command by prefixing our query with it, like this:
EXPLAIN SELECT * FROM customers WHERE name = 'John';
The EXPLAIN command will display the execution plan in a textual format. It provides detailed information about the query’s execution steps, including the index scans used, the estimated cost of each step, and the order in which the steps are executed. By examining the execution plan, we can determine if an index is being utilized effectively.
2. EXPLAIN ANALYZE command:
The EXPLAIN ANALYZE command is similar to the EXPLAIN command but also executes the query and measures the actual execution time. This allows us to see the actual performance of the query and validate if the index is being utilized effectively.
EXPLAIN ANALYZE SELECT * FROM customers WHERE name = 'John';
The EXPLAIN ANALYZE command will display the execution plan along with the actual execution time and other performance-related information. By comparing the execution time with and without the index, we can determine if the index is improving query performance.
3. Query planner functions:
PostgreSQL provides several query planner functions that allow us to obtain the execution plan programmatically. These functions can be used within SQL queries or in programming languages that have PostgreSQL bindings.
For example, we can use the pg_stat_statements extension to get the execution plan for a specific query:
SELECT * FROM pg_stat_statements WHERE query = 'SELECT * FROM customers WHERE name = ''John''';
The pg_stat_statements table stores statistics about executed queries, including the execution plan. By querying this table, we can obtain the execution plan for a specific query and analyze if an index is being utilized effectively.
Analyzing the Execution Plan of a PostgreSQL Query
Analyzing the execution plan of a PostgreSQL query is crucial for understanding how the database engine is executing the query and whether the indexes are being utilized effectively. The execution plan provides valuable insights into the steps taken by the query planner and helps identify potential areas for optimization.
Let’s explore the components of a typical execution plan in PostgreSQL:
1. Node types:
The execution plan consists of various nodes, each representing a specific operation performed by the query planner. Common node types include Seq Scan, Index Scan, Bitmap Index Scan, Hash Join, Nested Loop, and Aggregate.
2. Cost and timing information:
Each node in the execution plan has associated cost and timing information. The cost represents the estimated resource usage for executing the operation, and the timing information includes the startup time, total time, and actual time spent by the query planner.
3. Join types:
If the query involves joining multiple tables, the execution plan will include join nodes representing the different join types used. Common join types include Nested Loop, Hash Join, and Merge Join.
4. Index scans:
The execution plan may include index scan nodes, indicating that an index is being utilized for retrieving data. These nodes provide information about the index being used, the index scan method employed, and the columns being scanned.
To analyze the execution plan of a PostgreSQL query, we can use the EXPLAIN command or its variants, as mentioned in the previous section. Let’s consider an example to understand how to analyze the execution plan:
Example:
Suppose we have a table called “products” with the following schema:
CREATE TABLE products ( id SERIAL PRIMARY KEY, name VARCHAR(100), price DECIMAL(10, 2), category_id INT );
We create a B-tree index on the “category_id” column:
CREATE INDEX idx_products_category_id ON products (category_id);
Now, let’s execute a query that retrieves all products in a specific category, sorted by price:
EXPLAIN SELECT * FROM products WHERE category_id = 123 ORDER BY price;
The execution plan will be displayed, providing information about the nodes, costs, and timing. By examining the execution plan, we can determine if the index on the “category_id” column is being utilized effectively. We can also identify any potential performance bottlenecks in the query execution.
Analyzing the execution plan helps us understand how the query planner is executing our queries and provides insights into the efficiency of our index usage. By optimizing the query based on the execution plan, we can improve query performance and overall system efficiency.
Related Article: Examining Query Execution Speed on Dates in PostgreSQL
Different Types of Indexes in PostgreSQL
PostgreSQL offers several types of indexes, each designed for specific use cases and query patterns. Understanding the different types of indexes available in PostgreSQL is essential for effective query optimization.
Let’s explore the different types of indexes in PostgreSQL:
1. B-tree index:
The B-tree index is the default index type in PostgreSQL and is suitable for most use cases. It organizes data in a balanced tree structure, allowing for efficient searching and retrieval of data. B-tree indexes are useful for equality and range queries on indexed columns.
Example:
To create a B-tree index on the “name” column of a table called “users”:
CREATE INDEX idx_users_name ON users (name);
2. Hash index:
The hash index is suitable for equality queries on indexed columns. It uses a hash function to generate a hash code for each indexed value, allowing for fast lookup of matching rows. Hash indexes are ideal for queries that involve equality checks on indexed columns.
Example:
To create a hash index on the “email” column of a table called “users”:
CREATE INDEX idx_users_email ON users USING hash (email);
3. GiST index:
The GiST (Generalized Search Tree) index is a flexible index type that supports a wide range of data types and query patterns. It is suitable for complex queries involving multidimensional data, spatial data, and full-text search. GiST indexes allow for efficient searching and retrieval of data based on various criteria.
Example:
To create a GiST index on the “location” column of a table called “places”:
CREATE INDEX idx_places_location ON places USING gist (location);
4. GIN index:
The GIN (Generalized Inverted Index) index is designed for full-text search and supports efficient searching and retrieval of data based on specific keywords or phrases. GIN indexes are useful for queries that involve text search operations.
Example:
To create a GIN index on the “content” column of a table called “articles”:
CREATE INDEX idx_articles_content ON articles USING gin (content);
5. SP-GiST index:
The SP-GiST (Space-Partitioned Generalized Search Tree) index is an extension of the GiST index and is suitable for complex data types and query patterns. SP-GiST indexes provide efficient searching and retrieval of data for various data structures, including trees, graphs, and geometric objects.
Example:
To create an SP-GiST index on the “geometry” column of a table called “shapes”:
CREATE INDEX idx_shapes_geometry ON shapes USING spgist (geometry);
These are some of the different types of indexes available in PostgreSQL. Each index type has its own characteristics and is suitable for specific use cases. By understanding the different types of indexes and their usage scenarios, we can choose the appropriate index type to optimize our queries effectively.
Tools for Determining Index Usage in PostgreSQL
PostgreSQL provides several tools and utilities for determining index usage and optimizing queries. These tools help analyze the execution plans, monitor query performance, and identify potential areas for optimization.
Let’s explore some of the key tools available in PostgreSQL:
1. EXPLAIN command:
The EXPLAIN command, as mentioned earlier, allows us to obtain the execution plan for a query without actually executing it. By analyzing the execution plan, we can determine if an index is being utilized effectively and identify potential areas for optimization.
Example:
To obtain the execution plan for a query:
EXPLAIN SELECT * FROM customers WHERE name = 'John';
2. EXPLAIN ANALYZE command:
The EXPLAIN ANALYZE command, also mentioned earlier, provides the execution plan along with the actual execution time and other performance-related information. By comparing the execution time with and without the index, we can determine if the index is improving query performance.
Example:
To obtain the execution plan and analyze the query performance:
EXPLAIN ANALYZE SELECT * FROM customers WHERE name = 'John';
3. pg_stat_statements extension:
The pg_stat_statements extension provides statistics about executed queries, including the execution plans. By querying the pg_stat_statements table, we can obtain the execution plan for a specific query and analyze if an index is being utilized effectively.
Example:
To obtain the execution plan for a specific query using the pg_stat_statements extension:
SELECT * FROM pg_stat_statements WHERE query = 'SELECT * FROM customers WHERE name = ''John''';
4. pg_stat_activity view:
The pg_stat_activity view provides information about the currently running queries and their execution plans. By querying this view, we can monitor the query activity in real-time and identify queries that may benefit from index optimization.
Example:
To view the currently running queries and their execution plans:
SELECT query, plan FROM pg_stat_activity;
5. pgBadger:
pgBadger is a PostgreSQL log analyzer that generates detailed reports from the PostgreSQL log files. It provides insights into query performance, including the usage of indexes and the overall system health. By analyzing the pgBadger reports, we can identify queries that may require index optimization.
These are some of the tools available in PostgreSQL for determining index usage and optimizing queries. By using these tools effectively, we can improve query performance, identify potential bottlenecks, and optimize our database system for better efficiency.
Best Practices for Optimizing Queries with Indexes
Optimizing queries with indexes is essential for improving query performance and overall system efficiency. By following best practices for index usage, we can ensure that our queries execute quickly and efficiently.
Let’s explore some best practices for optimizing queries with indexes in PostgreSQL:
1. Identify the right columns to index:
Carefully analyze the query patterns and identify the columns that are frequently used in the WHERE clause or involved in join conditions. These columns are good candidates for indexing as they can significantly improve query performance.
2. Consider index selectivity:
When choosing columns to index, consider their selectivity, i.e., the number of distinct values in the column compared to the total number of rows. Indexing highly selective columns is more beneficial as it reduces the number of rows to be scanned.
3. Avoid over-indexing:
While indexing is crucial for query optimization, excessive indexing can have a negative impact on write performance and disk space usage. Avoid creating indexes on columns that are rarely used in queries or have low selectivity.
4. Regularly analyze and update statistics:
PostgreSQL maintains statistics about the distribution of data in tables, which helps the query planner in generating efficient execution plans. Regularly analyze and update these statistics to ensure optimal query performance.
5. Use multi-column indexes:
In some cases, creating indexes on multiple columns can provide better query performance compared to individual single-column indexes. Multi-column indexes are useful when queries involve conditions on multiple columns or join conditions.
6. Be cautious with index-only scans:
PostgreSQL supports index-only scans, where data can be retrieved directly from the index without accessing the table. While index-only scans can improve query performance, they require that all columns in the SELECT clause are included in the index.
7. Monitor and tune query performance:
Regularly monitor query performance using tools like EXPLAIN, EXPLAIN ANALYZE, and pg_stat_statements. Analyze the execution plans and identify queries that may benefit from index optimization. Fine-tune the queries and indexes based on the analysis.
8. Regularly maintain and reindex indexes:
Over time, as data changes, indexes may become fragmented and less efficient. Regularly maintain and reindex the indexes to ensure optimal query performance. Use tools like pg_repack or REINDEX to rebuild indexes when necessary.
Related Article: Analyzing Postgres: Maximum Query Handling Capacity
Simultaneous Use of Multiple Indexes in a PostgreSQL Query
PostgreSQL allows the simultaneous use of multiple indexes in a query to improve query performance. By utilizing multiple indexes, the database can efficiently retrieve data from different indexed columns and combine the results to satisfy the query conditions.
Let’s consider an example to understand the simultaneous use of multiple indexes in PostgreSQL:
Example:
Suppose we have a table called “orders” with the following schema:
CREATE TABLE orders ( id SERIAL PRIMARY KEY, customer_id INT, product_id INT, quantity INT, price DECIMAL(10, 2) );
We create two B-tree indexes, one on the “customer_id” column and another on the “product_id” column:
CREATE INDEX idx_orders_customer_id ON orders (customer_id); CREATE INDEX idx_orders_product_id ON orders (product_id);
Now, let’s execute a query that retrieves all orders for a specific customer and a specific product:
SELECT * FROM orders WHERE customer_id = 123 AND product_id = 456;
During the execution of this query, PostgreSQL can utilize both the “idx_orders_customer_id” and “idx_orders_product_id” indexes simultaneously. It can perform index scans on both indexes and combine the results to determine the final set of rows that satisfy both conditions.
The simultaneous use of multiple indexes allows PostgreSQL to efficiently retrieve the relevant rows without scanning the entire table. It speeds up query execution and improves overall system performance.
When using multiple indexes in a query, it is important to consider the selectivity of the indexed columns and the query conditions. Highly selective columns and conditions that involve equality checks are more suitable for index usage.
Forcing PostgreSQL to Use a Specific Index for a Query
In PostgreSQL, the query planner determines the most efficient execution plan for a query based on the available indexes, table statistics, and query conditions. However, there may be situations where we want to force PostgreSQL to use a specific index for a query, even if the query planner suggests a different plan.
To force PostgreSQL to use a specific index for a query, we can employ the following techniques:
1. Index hints:
PostgreSQL allows us to provide hints to the query planner using the INDEX hint. By specifying the index name in the query, we can force PostgreSQL to use that index for executing the query.
Example:
SELECT * FROM customers WHERE name = 'John' AND city = 'New York' -- INDEX (idx_customers_name)
In this example, we want to force PostgreSQL to use the “idx_customers_name” index for the query. By adding the INDEX hint, we instruct PostgreSQL to use that specific index regardless of the query planner’s decision.
2. Index-only scans:
PostgreSQL supports index-only scans, where data can be retrieved directly from the index without accessing the table. By creating an index that includes all the columns required by the query, we can force PostgreSQL to use the index-only scan.
Example:
Suppose we have a table called “products” with the following schema:
CREATE TABLE products ( id SERIAL PRIMARY KEY, name VARCHAR(100), price DECIMAL(10, 2), category_id INT );
We create an index on the “name” and “price” columns:
CREATE INDEX idx_products_name_price ON products (name, price);
Now, let’s execute a query that retrieves only the “name” and “price” columns:
SELECT name, price FROM products WHERE category_id = 123;
It is important to note that forcing PostgreSQL to use a specific index should be done with caution. The query planner is designed to choose the most efficient execution plan based on various factors, including table statistics and available indexes. Forcing the use of a specific index may not always result in improved query performance and can lead to suboptimal execution plans.
Before forcing PostgreSQL to use a specific index, it is recommended to thoroughly analyze the query performance, examine the execution plans, and consider alternative optimization techniques. Only force the use of a specific index when it is absolutely necessary and after careful evaluation of the potential impact on query performance.
Potential Drawbacks of Using Indexes in PostgreSQL
While indexes can significantly improve query performance, they also come with certain drawbacks and considerations that need to be taken into account.
Let’s explore some potential drawbacks of using indexes in PostgreSQL:
1. Increased disk space usage:
Indexes require additional disk space to store the index data structures. When creating multiple indexes on a table, the disk space usage can increase significantly, especially for large tables with millions of rows. This can be a concern if disk space is limited or if the database needs to be replicated across multiple servers.
2. Index maintenance overhead:
Indexes need to be maintained and updated whenever the underlying data changes. This maintenance overhead can impact write performance, as the database needs to update the indexes along with the data modifications. Regular index maintenance and reindexing may be required to ensure optimal query performance.
3. Indexes may become fragmented:
Over time, indexes may become fragmented due to data modifications and updates. Fragmented indexes can result in slower query performance, as the database needs to traverse more index blocks to retrieve the data. Regular index maintenance, such as rebuilding or reorganizing indexes, may be necessary to reduce fragmentation.
4. Choosing the wrong columns to index:
Choosing the wrong columns to index can lead to suboptimal query performance. Indexes on columns that are rarely used in queries or have low selectivity can result in wasted disk space and unnecessary index maintenance overhead. Careful analysis of query patterns and column selectivity is required to choose the right columns to index.
5. Query planner overhead:
The query planner needs to analyze the query, table statistics, and available indexes to determine the most efficient execution plan. When multiple indexes are available, the query planner needs to evaluate various index scan methods and choose the best one. This overhead can impact query planning and optimization time, especially for complex queries involving multiple tables and indexes.
6. Indexes increase query complexity:
Indexes introduce additional complexity to the database schema and query execution. Maintaining and managing indexes requires additional knowledge and expertise. Incorrect or inefficient use of indexes can lead to suboptimal query performance and increased system complexity.
It is important to carefully consider these potential drawbacks and weigh the trade-offs before creating indexes in PostgreSQL. Proper analysis of query patterns, table statistics, and index selectivity is crucial for effective index usage and optimal query performance. Regular monitoring and maintenance of indexes can help mitigate the potential drawbacks and ensure that the database system performs efficiently.
Related Article: Evaluating Active Connections to a PostgreSQL Query
Additional Resources
– How can I determine if my PostgreSQL query is using an index?
– What is the PostgreSQL query planner and how does it relate to index usage?
– How do I use the EXPLAIN command in PostgreSQL to analyze query execution plans?