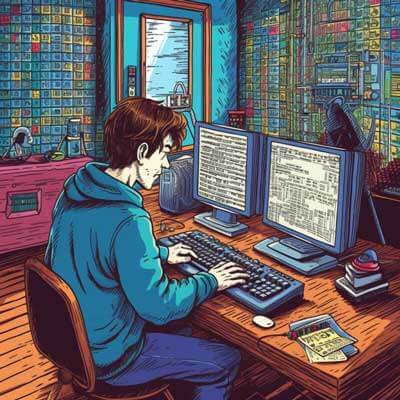
Table of Contents
When executing a query in PostgreSQL, the column size determines how much data is displayed for each column. By default, PostgreSQL will display the entire contents of each column in the query result. However, you can adjust the column size to limit the amount of data displayed.
To set the column size in a PostgreSQL query, you can use the LEFT
function to extract a specific number of characters from a column. The LEFT
function takes two arguments: the column you want to extract characters from and the number of characters to extract. Here's an example:
SELECT LEFT(column_name, 10) FROM table_name;
This query will extract the first 10 characters from the column_name
column in the table_name
table.
You can also use the SUBSTRING
function to extract a substring from a column. The SUBSTRING
function takes three arguments: the column you want to extract characters from, the starting position, and the number of characters to extract. Here's an example:
SELECT SUBSTRING(column_name FROM 2 FOR 5) FROM table_name;
This query will extract a substring starting from the second character and containing 5 characters from the column_name
column in the table_name
table.
What is the Default Column Size in Postgres Queries?
The default column size in PostgreSQL queries is to display the entire contents of each column in the query result. This means that if a column contains a large amount of data, the entire contents of that column will be displayed. This default behavior is useful when you need to see the complete data in a column.
For example, if you have a column that stores paragraphs of text, the default column size will display the entire paragraph in the query result. This can be helpful when you need to analyze the text or perform operations on it.
Related Article: How to Extract Data from PostgreSQL Databases: PSQL ETL
Can I Change the Column Size in Postgres Queries?
Yes, you can change the column size in PostgreSQL queries by using functions like LEFT
or SUBSTRING
to extract a specific number of characters from a column. This allows you to limit the amount of data displayed for each column in the query result.
Changing the column size can be useful in various scenarios. For example, if you have a column that stores long URLs and you only need to display the domain name, you can use the SUBSTRING
function to extract the domain name from the URL. This will reduce the amount of data displayed and make the query result more readable.
It's important to note that changing the column size in a query does not affect the actual data stored in the table. The original data remains unchanged, and only the displayed output is adjusted.
How to Adjust the Column Size in Postgres Queries?
To adjust the column size in PostgreSQL queries, you can use the LEFT
or SUBSTRING
functions to extract a specific number of characters from a column. Here are some examples of how to adjust the column size:
Example 1: Using the LEFT function to extract a specific number of characters from a column
SELECT LEFT(column_name, 10) FROM table_name;
This query will extract the first 10 characters from the column_name
column in the table_name
table.
Example 2: Using the SUBSTRING function to extract a substring from a column
SELECT SUBSTRING(column_name FROM 2 FOR 5) FROM table_name;
This query will extract a substring starting from the second character and containing 5 characters from the column_name
column in the table_name
table.
Adjusting the column size allows you to limit the amount of data displayed for each column in the query result, making it more manageable and readable.
Limitations of Column Size in Postgres Queries
While adjusting the column size in PostgreSQL queries can be useful, there are some limitations to be aware of.
Firstly, adjusting the column size does not affect the actual data stored in the table. The original data remains unchanged, and only the displayed output is adjusted. This means that if you need to retrieve the full data for a column, you will still need to retrieve it separately.
Secondly, adjusting the column size can affect the readability of the data. If you extract a small portion of a column, you may lose important context or information that is present in the full data. It's important to carefully consider the amount of data to extract to ensure that the query result remains meaningful.
Lastly, adjusting the column size can impact the performance of your queries. If you extract a large amount of data from a column, it may require additional resources and slow down the query execution. It's important to test and optimize your queries to ensure that they run efficiently.
Related Article: Tutorial: PostgreSQL Array Literals
Is there a Maximum Column Size in Postgres Queries?
In PostgreSQL, there is no maximum column size for queries. You can extract as many characters as you need from a column using functions like LEFT
or SUBSTRING
. However, it's important to consider the performance implications of extracting large amounts of data from a column, as it may slow down the query execution.
It's worth noting that PostgreSQL has a maximum column size for storing data in tables. The maximum column size depends on the data type of the column and can vary. For example, the text
data type can store up to 1 GB of data, while the varchar
data type has a maximum size of 1 GB.
When working with large amounts of data, it's important to use appropriate data types and optimize your queries to ensure efficient storage and retrieval.
The Impact of Changing Column Size on Performance in Postgres Queries
Changing the column size in PostgreSQL queries can have an impact on performance. When you extract a large amount of data from a column, it may require additional resources and slow down the query execution.
The performance impact of changing the column size depends on various factors, including the size of the data being extracted, the number of rows in the table, and the overall complexity of the query.
Extracting a small portion of a column, such as the first few characters, usually has minimal impact on performance. However, if you extract a large portion of a column or extract data from multiple columns, it can significantly increase the execution time of the query.
To mitigate the performance impact, you can consider the following best practices:
1. Only extract the necessary amount of data: Carefully consider the amount of data to extract from a column. Extracting more data than necessary can unnecessarily slow down the query execution.
2. Use appropriate data types: Choose the appropriate data types for your columns to ensure efficient storage and retrieval of data. Using data types with a smaller storage size can help improve performance.
3. Optimize your queries: Review and optimize your queries to ensure they are efficient. This includes using appropriate indexes, avoiding unnecessary joins, and optimizing the query execution plan.
Best Practices for Setting Column Size in Postgres Queries
When setting the column size in PostgreSQL queries, it's important to follow best practices to ensure the readability and performance of your queries. Here are some best practices to consider:
1. Limit the amount of data displayed: Extract only the necessary amount of data from a column. This helps to keep the query result readable and manageable.
2. Consider the context and information needed: When adjusting the column size, consider the context and information needed from the column. Extracting too little data may result in loss of important information, while extracting too much data may impact performance.
3. Use appropriate data types: Choose the appropriate data types for your columns to ensure efficient storage and retrieval of data. This helps to optimize performance and storage space.
4. Optimize your queries: Review and optimize your queries to ensure they are efficient. This includes using appropriate indexes, avoiding unnecessary joins, and optimizing the query execution plan.
Handling Column Size Exceeding Values in Postgres Queries
In PostgreSQL queries, it's common to encounter situations where the column size exceeds the desired limit. This can happen when extracting data from columns that contain more characters than expected.
To handle column size exceeding values, you can use the CASE
statement in your queries. The CASE
statement allows you to perform conditional logic and specify different actions based on the column size.
Here's an example of how to handle column size exceeding values using the CASE
statement:
SELECT CASE WHEN LENGTH(column_name) > 10 THEN LEFT(column_name, 10) || '...' ELSE column_name END FROM table_name;
In this example, if the length of the column_name
exceeds 10 characters, the CASE
statement will extract the first 10 characters and append ellipsis (...) to indicate that the data has been truncated. If the length is within the desired limit, the original data will be displayed.
Related Article: Step-by-Step Process to Uninstall PostgreSQL on Ubuntu
Setting Different Column Sizes for Different Columns in Postgres Queries
In PostgreSQL queries, you can set different column sizes for different columns by using the LEFT
or SUBSTRING
functions individually for each column. This allows you to adjust the column size based on the specific requirements of each column.
Here's an example of how to set different column sizes for different columns in a PostgreSQL query:
SELECT LEFT(column1, 10), LEFT(column2, 15), LEFT(column3, 20) FROM table_name;
In this example, the LEFT
function is used to extract the first 10 characters from column1
, the first 15 characters from column2
, and the first 20 characters from column3
. This allows you to set different column sizes for different columns in the query result.
Additional Resources
- PostgreSQL Documentation: Numeric Types