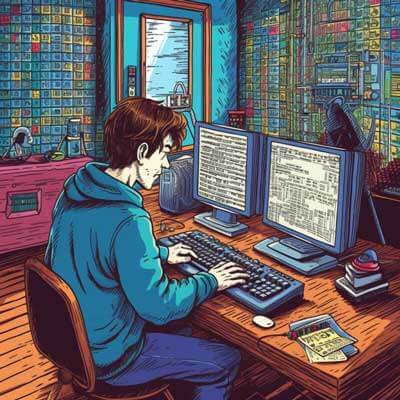
Table of Contents
When working with MySQL databases, it is common to encounter boolean values in query results. By default, MySQL represents boolean true as 1 and boolean false as 0. However, in certain scenarios, it may be necessary to convert these boolean values to a different representation, such as "Yes" and "No". This can be particularly useful when presenting query results to end-users or integrating with other systems that expect different representations for boolean values.
Converting MySQL query result from true to yes not only enhances the readability and usability of the data but also ensures compatibility and interoperability with external systems. It allows for a more intuitive understanding of the data and avoids confusion that may arise from using numerical representations for boolean values.
Exploring the Syntax for Converting MySQL Query Result from True to Yes
MySQL provides various functions and operators that can be used to convert boolean values to different representations. One of the most commonly used functions is the IF() function, which allows you to conditionally return a value based on a specified condition.
The syntax for the IF() function is as follows:
IF(condition, value_if_true, value_if_false)
Here, the condition is the expression that evaluates to a boolean value, value_if_true is the value that will be returned if the condition is true, and value_if_false is the value that will be returned if the condition is false.
To convert a boolean true value to "Yes" and a boolean false value to "No", you can use the IF() function as follows:
SELECT IF(boolean_column, 'Yes', 'No') AS converted_value FROM table_name;
This query will return the converted_value column, where the boolean_column values are converted to "Yes" if true and "No" if false.
Related Article: Securing MySQL Access through Bash Scripts in Linux
Understanding the Boolean Values in MySQL Query Results
In MySQL, boolean values are represented using the TINYINT data type. By default, boolean true is represented as 1 and boolean false is represented as 0. However, it is important to note that MySQL is flexible in interpreting boolean values and can also accept other representations such as 'true' and 'false', as well as non-zero and zero values.
When working with MySQL query results, it is crucial to understand how boolean values are stored and interpreted to ensure accurate conversions. By default, MySQL will display boolean values as 1 and 0 in query results. However, you can use the IF() function or other conversion techniques to display boolean values as different representations, such as "Yes" and "No".
Converting MySQL Query Result from True to Yes: Step-by-Step Guide
To convert MySQL query result from true to yes, follow these step-by-step instructions:
Step 1: Identify the boolean column in your table that you want to convert.
Step 2: Construct a SELECT query that retrieves the boolean column and uses the IF() function to convert the values to "Yes" and "No".
Step 3: Execute the query and observe the result set.
Let's illustrate this with an example. Suppose we have a table named "users" with a boolean column named "is_active". We want to convert the values of the "is_active" column to "Yes" if true and "No" if false.
Here's the step-by-step guide:
Step 1: Identify the boolean column "is_active" in the "users" table.
Step 2: Construct the SELECT query:
SELECT IF(is_active, 'Yes', 'No') AS active_status FROM users;
This query will retrieve the "is_active" column and convert the boolean values to "Yes" if true and "No" if false. The result set will include a column named "active_status" with the converted values.
Step 3: Execute the query and observe the result set:
+--------------+ | active_status | +--------------+ | Yes | | No | | No | | Yes | +--------------+
The result set shows the converted values of the "is_active" column in the "active_status" column.
Techniques for Changing MySQL Query Result from True to Yes
In addition to using the IF() function, there are other techniques that can be used to change MySQL query result from true to yes. Let's explore some of these techniques:
1. CASE statement:
The CASE statement allows you to perform conditional logic in SQL queries. It can be used to convert boolean values to different representations, including "Yes" and "No".
Here's an example of using the CASE statement to convert a boolean column "is_active" to "Yes" if true and "No" if false:
SELECT CASE WHEN is_active THEN 'Yes' ELSE 'No' END AS active_status FROM users;
2. Boolean to string conversion:
Another technique is to explicitly convert the boolean values to strings using the CAST() or CONCAT() functions.
Here's an example of converting a boolean column "is_active" to "Yes" if true and "No" if false using the CAST() function:
SELECT CAST(is_active AS CHAR(3)) AS active_status FROM users;
Or using the CONCAT() function:
SELECT CONCAT('', is_active) AS active_status FROM users;
These techniques provide alternative ways to convert boolean values to different representations and give you flexibility in choosing the method that best suits your needs.
Related Article: How to Connect Java with MySQL
Best Practices for Converting MySQL Query Result from True to Yes
When converting MySQL query result from true to yes, it is important to follow best practices to ensure accurate and efficient conversions. Here are some best practices to consider:
1. Use appropriate data types: Ensure that the boolean values in your table are stored using the correct data type, such as TINYINT, BOOL, or BOOLEAN. Using the correct data type will help avoid inconsistencies and improve query performance.
2. Use explicit conversion functions: When converting boolean values to different representations, use explicit conversion functions like IF(), CASE, CAST(), or CONCAT(). This helps to make the conversion logic clear and avoids any potential confusion.
3. Consider internationalization: If your application supports multiple languages, keep in mind that the representation of boolean values may vary across different locales. Consider using localization techniques to handle different representations based on the user's language preference.
4. Test and validate: Before deploying your code to production, thoroughly test and validate the conversion logic to ensure that it produces the expected results. Consider testing different scenarios, including true, false, and null values, to ensure full coverage.
Common Mistakes to Avoid When Converting MySQL Query Result from True to Yes
When converting MySQL query result from true to yes, certain mistakes can lead to incorrect results or inefficient queries. Here are some common mistakes to avoid:
1. Incorrect syntax: Make sure to use the correct syntax for the conversion functions and operators. Check the documentation for the specific version of MySQL you are using to ensure that you are using the appropriate syntax.
2. Neglecting null values: If your boolean column allows null values, make sure to handle them properly in your conversion logic. Consider using the COALESCE() function or the IS NULL operator to handle null values explicitly.
3. Not considering different representations: Remember that boolean values can be represented in various ways, such as numeric values, string literals ('true' and 'false'), or even non-zero and zero values. Make sure to consider the specific representation used in your database when performing conversions.
4. Overcomplicating the logic: Keep the conversion logic simple and straightforward. Overcomplicating the logic can lead to confusion and errors. If possible, use built-in functions and operators instead of custom logic.
Troubleshooting Tips for Converting MySQL Query Result from True to Yes
While converting MySQL query result from true to yes is a relatively straightforward task, you may encounter some issues along the way. Here are some troubleshooting tips to help you resolve common problems:
1. Check data types: Ensure that the data type of your boolean column matches the expected data type for boolean values. If the data type is incorrect, conversions may not work as expected. Consider altering the column's data type if necessary.
2. Verify column names: Double-check that you are referencing the correct column name in your SELECT query. Typos or referencing the wrong column can lead to incorrect results.
3. Debug your conversion logic: If your conversions are not producing the expected results, consider using debugging techniques to identify the issue. Break down your conversion logic into smaller parts and test each part to isolate the problem.
4. Review documentation and examples: Consult the MySQL documentation and search for examples or tutorials that cover similar conversion scenarios. This can provide valuable insights and help you identify any mistakes in your approach.
5. Seek help from the community: If you are still having trouble, reach out to the MySQL community for assistance. Forums, communities, and online resources can provide guidance and solutions to specific issues.
Using Functions to Convert MySQL Query Result from True to Yes
In addition to the IF() function, the CASE statement, CAST() function, and CONCAT() function, MySQL provides other functions that can be used to convert MySQL query result from true to yes.
One such function is the BOOLEAN function, which can be used to convert boolean values to their string representations.
Here's an example of using the BOOLEAN function to convert a boolean column "is_active" to "Yes" if true and "No" if false:
SELECT BOOLEAN(is_active) AS active_status FROM users;
This query will return the "active_status" column with the converted values of the "is_active" column.
Other functions that can be used for conversion include FORMAT(), REPLACE(), and CONCAT_WS(). These functions offer additional flexibility and customization options for converting MySQL query result from true to yes.
Related Article: How To Import a SQL File With a MySQL Command Line
Strategies for Efficiently Converting MySQL Query Result from True to Yes
To efficiently convert MySQL query result from true to yes, consider the following strategies:
1. Perform the conversion in the database: Whenever possible, perform the conversion directly in the database rather than in application code. This minimizes data transfer and processing overhead, resulting in improved performance.
2. Use appropriate indexing: If you frequently perform conversions on large datasets, consider adding appropriate indexes to the columns involved in the conversion. This can significantly speed up the conversion process by reducing the number of rows that need to be scanned.
3. Limit unnecessary conversions: Only convert boolean values when necessary. If you don't need to present the converted values to end-users or integrate with external systems, it may be more efficient to keep the boolean values as they are.
4. Optimize query execution: Ensure that your queries are optimized for performance. This includes using appropriate indexes, minimizing the number of rows retrieved, and avoiding unnecessary joins or subqueries.
Advanced Techniques for Converting MySQL Query Result from True to Yes
In addition to the basic techniques discussed earlier, there are advanced techniques that can be used to convert MySQL query result from true to yes. These techniques offer additional flexibility and customization options. Let's explore some of these advanced techniques:
1. Custom conversion functions: MySQL allows you to define your own custom functions using the CREATE FUNCTION statement. You can create a custom conversion function that encapsulates your specific conversion logic and use it in your queries.
2. Stored procedures: Another advanced technique is to use stored procedures to perform the conversion. Stored procedures allow you to encapsulate complex logic and reuse it across multiple queries or applications.
3. User-defined variables: User-defined variables can be used to store intermediate values during the conversion process. This can be useful when performing complex conversions that require multiple steps or conditions.
4. Regular expressions: Regular expressions can be used to match and replace specific patterns in the query result. They provide useful pattern-matching capabilities and can be used to perform advanced conversions based on specific criteria.
These advanced techniques provide additional flexibility and customization options for converting MySQL query result from true to yes. They can be particularly useful for complex conversions or scenarios where the standard conversion functions are not sufficient.
Additional Resources
- MySQL - UPDATE Query