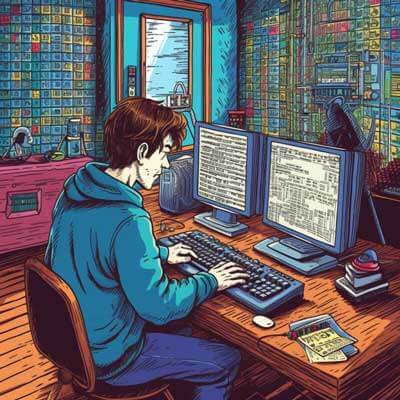
Table of Contents
Converting a string to a number is a common task in PHP programming. There are several scenarios where you might need to convert a string to a number, such as when performing mathematical calculations or when comparing numeric values. In this guide, we will explore different methods to convert a string to a number in PHP.
Method 1: Using the intval() function
The intval()
function is a built-in PHP function that converts a value to an integer. It takes a string as an argument and returns the integer representation of that string.
Here's an example of how to use intval()
to convert a string to an integer:
$string = "123"; $number = intval($string); echo $number; // Output: 123
In the above example, the string "123" is converted to the integer 123
using the intval()
function.
Related Article: How To Fix the “Array to string conversion” Error in PHP
Method 2: Using the (int) cast
Another way to convert a string to an integer in PHP is by using the (int)
cast. This cast explicitly converts a value to an integer.
Here's an example of how to use the (int)
cast to convert a string to an integer:
$string = "456"; $number = (int) $string; echo $number; // Output: 456
In the above example, the string "456" is cast to an integer using the (int)
cast.
Method 3: Using the floatval() function
If you need to convert a string to a floating-point number (decimal number), you can use the floatval()
function in PHP. This function takes a string as an argument and returns the floating-point representation of that string.
Here's an example of how to use floatval()
to convert a string to a floating-point number:
$string = "3.14"; $number = floatval($string); echo $number; // Output: 3.14
In the above example, the string "3.14" is converted to the floating-point number 3.14
using the floatval()
function.
Method 4: Using the (float) cast
Similar to the (int)
cast, you can also use the (float)
cast to convert a string to a floating-point number in PHP.
Here's an example of how to use the (float)
cast to convert a string to a floating-point number:
$string = "2.718"; $number = (float) $string; echo $number; // Output: 2.718
In the above example, the string "2.718" is cast to a floating-point number using the (float)
cast.
Related Article: How To Make A Redirect In PHP
Method 5: Using the number_format() function
If you have a string that represents a number with decimal places and you want to convert it to a formatted string with a specific number of decimal places, you can use the number_format()
function in PHP.
Here's an example of how to use number_format()
to convert a string to a formatted number:
$string = "12345.6789"; $formattedNumber = number_format($string, 2); echo $formattedNumber; // Output: 12,345.68
In the above example, the string "12345.6789" is converted to the formatted number string "12,345.68" using the number_format()
function.
Method 6: Using the filter_var() function
The filter_var()
function in PHP can be used to validate and filter various types of data, including numeric values. By using the FILTER_VALIDATE_INT
or FILTER_VALIDATE_FLOAT
filter with the filter_var()
function, you can convert a string to a numeric value.
Here's an example of how to use filter_var()
to convert a string to a numeric value:
$string = "789"; $number = filter_var($string, FILTER_VALIDATE_INT); echo $number; // Output: 789
In the above example, the string "789" is converted to the integer 789
using the filter_var()
function with the FILTER_VALIDATE_INT
filter.
Why is the conversion from string to number necessary?
The need to convert a string to a number often arises in scenarios where numeric calculations or comparisons are required. For example, when working with user input or data retrieved from a database, the values are typically stored as strings. To perform mathematical operations or compare the values, it is necessary to convert the strings to numeric values.
Additionally, converting strings to numbers can be useful when working with APIs or external services that require specific data types. By converting the strings to the appropriate numeric types, you can ensure that the data is properly interpreted by the receiving system.
Best practices
When converting a string to a number in PHP, it is important to consider the following best practices:
1. Ensure that the string represents a valid numeric value before attempting to convert it. You can use functions like is_numeric()
or ctype_digit()
to check if a string is numeric before performing the conversion.
2. Be aware of potential issues with floating-point precision when converting strings to floating-point numbers. PHP uses floating-point representation for decimal numbers, which can introduce small rounding errors. If precision is critical, consider using a decimal library or performing calculations with integer values instead.
3. Handle conversion errors gracefully. If a string cannot be converted to a number, PHP will return 0
or false
, depending on the conversion function used. It is a good practice to check the return value and handle any conversion errors appropriately.
4. Consider the locale and formatting conventions when working with formatted numbers. The conversion functions may not handle localized number formats correctly. If you need to convert a formatted number string, it may be necessary to remove any non-numeric characters or adjust the formatting before performing the conversion.