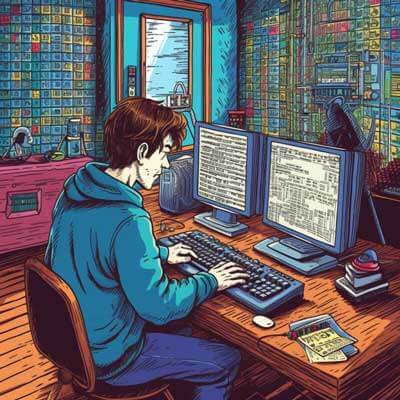
Table of Contents
What is a case statement in PostgreSQL?
In PostgreSQL, a case statement is a conditional expression that allows you to perform different actions based on the result of an expression or a boolean condition. It is a useful tool for data manipulation and can be used in various scenarios to make your queries more dynamic and flexible.
The case statement in PostgreSQL has two forms: simple case and searched case. The simple case form compares an expression to a set of constant values, while the searched case form evaluates multiple boolean expressions to determine the result.
Let's look at an example of a simple case statement in PostgreSQL:
SELECT product_name, CASE category_id WHEN 1 THEN 'Electronics' WHEN 2 THEN 'Clothing' WHEN 3 THEN 'Home Appliances' ELSE 'Other' END AS category FROM products;
In this example, the case statement is used to categorize products based on their category_id. If the category_id is 1, the product belongs to the Electronics category. If the category_id is 2, the product belongs to the Clothing category. If the category_id is 3, the product belongs to the Home Appliances category. Otherwise, the product is categorized as Other. The result of the case statement is aliased as "category" in the query result.
Related Article: Integrating Fluent Bit with PostgreSQL Databases
How do you use a case statement in a query in PostgreSQL?
To use a case statement in a query in PostgreSQL, you need to include the case statement within the SELECT clause. The syntax for a case statement in a query is as follows:
SELECT column1, column2, ..., CASE WHEN condition1 THEN result1 WHEN condition2 THEN result2 ... WHEN conditionN THEN resultN ELSE result END AS alias FROM table;
In this syntax, you can have multiple WHEN conditions and corresponding results. The condition is evaluated for each row in the table, and the result of the first matching condition is returned. If none of the conditions match, the ELSE result is returned. The result can be a literal value, a column value, or an expression.
Let's consider an example where we want to calculate the discounted price for products based on their original price:
SELECT product_name, price, CASE WHEN price > 100 THEN price * 0.9 WHEN price > 50 THEN price * 0.95 ELSE price END AS discounted_price FROM products;
In this example, the case statement is used to calculate the discounted price based on the original price. If the price is greater than 100, a 10% discount is applied. If the price is greater than 50 but less than or equal to 100, a 5% discount is applied. Otherwise, the original price is returned as the discounted price.
Can you put a query inside a case statement in PostgreSQL?
Yes, you can put a query inside a case statement in PostgreSQL. This allows you to perform subqueries or aggregate functions to generate the result of the case statement dynamically.
Let's look at an example where we want to categorize products based on their average price:
SELECT product_name, price, CASE WHEN price > (SELECT AVG(price) FROM products) THEN 'Expensive' ELSE 'Affordable' END AS category FROM products;
In this example, the case statement includes a subquery to calculate the average price of all products. If the price of a product is greater than the average price, it is categorized as "Expensive". Otherwise, it is categorized as "Affordable".
Using a query inside a case statement provides flexibility and allows you to make decisions based on dynamic conditions or calculations.
Advantages of using a case statement in PostgreSQL
The case statement in PostgreSQL offers several advantages that make it a useful tool for data manipulation:
1. Conditional logic: The case statement allows you to perform different actions based on the result of an expression or a boolean condition. This enables you to implement complex business rules and logic in your queries.
2. Flexibility: With the case statement, you can handle multiple conditions and their corresponding results within a single query. This eliminates the need for multiple IF statements or separate queries, making your code more concise and efficient.
3. Readability: By using a case statement, you can express your logic directly in SQL, making it easier for other developers to understand and maintain your code. It provides a clear and structured way to handle conditional operations.
4. Dynamic results: The case statement allows you to generate results dynamically by including subqueries or aggregate functions within the case statement. This enables you to perform calculations or retrieve data from other tables based on conditions.
5. Simplified data manipulation: The case statement can be used in various scenarios, such as data transformation, categorization, conditional aggregation, and more. It simplifies complex data manipulation tasks and reduces the need for multiple queries or procedural code.
Let's consider an example where we want to categorize products based on their price range:
SELECT product_name, price, CASE WHEN price < 50 THEN 'Cheap' WHEN price >= 50 AND price < 100 THEN 'Moderate' ELSE 'Expensive' END AS price_category FROM products;
In this example, the case statement is used to categorize products into three price categories: Cheap, Moderate, and Expensive. This provides a clear and concise way to analyze and present the data.
Related Article: Working With PostgreSQL: Extracting Day of Week
Limitations of using a case statement in PostgreSQL
While the case statement in PostgreSQL is a useful tool, it also has some limitations that you should be aware of:
1. Limited flexibility for complex logic: Although the case statement allows you to handle multiple conditions and results, it may not be suitable for complex business rules or advanced logic. In such cases, it is recommended to use procedural code or user-defined functions.
2. Performance impact: Using a case statement with subqueries or complex expressions can have a performance impact on your queries. It is important to optimize your queries and consider the execution plan to ensure efficient processing.
3. Limited control flow: The case statement in PostgreSQL does not support control flow statements like loops or branching. If you need to perform iterative operations or conditional branching, you should consider using procedural code or user-defined functions.
4. Limited error handling: The case statement does not provide built-in error handling mechanisms. If you need to handle exceptions or errors in your logic, you should use error handling constructs like TRY-CATCH or EXCEPTION blocks.
Despite these limitations, the case statement in PostgreSQL is still a versatile and valuable tool for data manipulation and conditional operations.
Using a case statement in data manipulation queries in PostgreSQL
The case statement can be used in data manipulation queries in PostgreSQL to perform conditional operations, transform data, or generate dynamic results. Let's explore some examples of using a case statement in data manipulation queries.
Example 1: Updating the status of orders based on their total amount
UPDATE orders SET status = CASE WHEN total_amount > 1000 THEN 'High Value' WHEN total_amount > 500 THEN 'Medium Value' ELSE 'Low Value' END;
In this example, the case statement is used in an UPDATE statement to update the status of orders based on their total_amount. If the total_amount is greater than 1000, the status is set to 'High Value'. If the total_amount is greater than 500 but less than or equal to 1000, the status is set to 'Medium Value'. Otherwise, the status is set to 'Low Value'.
Example 2: Calculating the total revenue by category
SELECT category, SUM(CASE WHEN price > 100 THEN price * quantity ELSE 0 END) AS revenue FROM products GROUP BY category;
In this example, the case statement is used in a SELECT statement to calculate the total revenue for each category. If the price of a product is greater than 100, the revenue is calculated by multiplying the price with the quantity. Otherwise, the revenue is set to 0. The result is aggregated using the SUM function and grouped by the category.
These examples demonstrate how the case statement can be used to perform conditional operations and generate dynamic results in data manipulation queries.
Working of a case statement in PostgreSQL
The case statement in PostgreSQL works by evaluating the conditions specified in the WHEN clauses and returning the corresponding result for the first matching condition. If none of the conditions match, the result specified in the ELSE clause is returned.
The case statement is evaluated for each row in the table or result set, allowing you to perform conditional operations or generate dynamic results based on the values of the columns.
Let's consider an example to understand the working of a case statement:
SELECT product_name, price, CASE WHEN price > 100 THEN 'Expensive' WHEN price > 50 THEN 'Moderate' ELSE 'Affordable' END AS price_category FROM products;
In this example, the case statement is used to categorize products based on their price. If the price is greater than 100, the product is categorized as "Expensive". If the price is greater than 50 but less than or equal to 100, the product is categorized as "Moderate". Otherwise, the product is categorized as "Affordable".
For each row in the products table, the case statement evaluates the conditions in the order specified and returns the corresponding result for the first matching condition. The result is aliased as "price_category" in the query result.
This working of the case statement allows you to perform conditional operations and generate dynamic results based on the values of the columns in your data.
Nesting case statements in PostgreSQL
In PostgreSQL, you can nest case statements within other case statements to handle more complex conditional logic. This allows you to perform multiple levels of conditional operations and make decisions based on different sets of conditions.
Let's consider an example where we want to categorize products based on their price and availability:
SELECT product_name, price, CASE WHEN price > 100 THEN CASE WHEN stock_quantity > 0 THEN 'Expensive (In Stock)' ELSE 'Expensive (Out of Stock)' END WHEN price > 50 THEN CASE WHEN stock_quantity > 0 THEN 'Moderate (In Stock)' ELSE 'Moderate (Out of Stock)' END ELSE CASE WHEN stock_quantity > 0 THEN 'Affordable (In Stock)' ELSE 'Affordable (Out of Stock)' END END AS category FROM products;
In this example, the case statement is nested within other case statements to categorize products based on their price and availability. If the price is greater than 100, a nested case statement checks the stock_quantity to determine if the product is in stock or out of stock. Similar nested case statements are used for the price range of 50 to 100 and less than or equal to 50.
Related Article: Tutorial: Dealing with Non-Existent Relations in PostgreSQL
Alternatives to using a case statement in PostgreSQL
While the case statement is a useful tool for conditional operations in PostgreSQL, there are alternative approaches that you can consider depending on your requirements and the complexity of your logic.
1. IF-ELSE statements: PostgreSQL provides an IF-ELSE statement that allows you to perform conditional operations similar to a case statement. The IF-ELSE statement is useful when you have a simple condition and want to execute different blocks of code based on the condition.
2. COALESCE function: The COALESCE function in PostgreSQL can be used as an alternative to a case statement when you want to return the first non-null value from a list of expressions. It simplifies the handling of null values and provides a concise way to handle different scenarios.
3. Procedural code: In some cases, when you have complex business rules or advanced logic, it may be more appropriate to use procedural code in PostgreSQL. This can be done using stored procedures, user-defined functions, or triggers. Procedural code allows you to define custom logic and control flow, making it suitable for complex scenarios.
4. JOINs and subqueries: Instead of using a case statement to generate dynamic results, you can consider using JOINs and subqueries to retrieve data from other tables based on conditions. This allows you to perform more complex calculations and retrieve data from related tables.
The choice of using a case statement or alternative approaches depends on the specific requirements of your application and the complexity of your logic. It is important to evaluate the trade-offs and choose the approach that best suits your needs.
Additional Resources
- PostgreSQL CASE expression