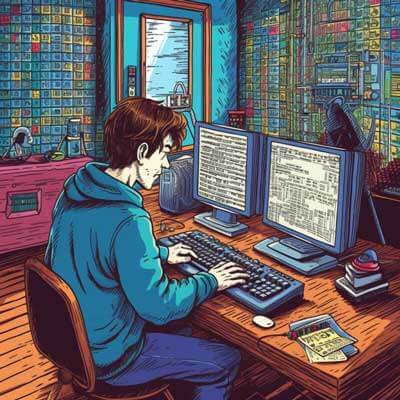
Table of Contents
In PostgreSQL, a stored procedure is a set of SQL statements that are stored in the database server and can be executed as a single unit. Typically, stored procedures are used to perform complex database operations that may require multiple SQL statements to be executed. While stored procedures are commonly used for data manipulation operations, such as inserting, updating, and deleting records, they can also be used to retrieve data using select queries.
Using a select query as a stored procedure in PostgreSQL allows you to encapsulate complex data retrieval logic within a single unit, making it easier to manage and reuse code. This can be particularly useful in scenarios where you need to perform the same data retrieval operation multiple times, or when you want to abstract the details of the query from the calling application.
To use a select query as a stored procedure in PostgreSQL, you can define a function that returns a resultset using the RETURNS TABLE
syntax. The function can then be executed like any other stored procedure, and the resultset can be fetched and processed as needed.
Example 1: Using a Select Query as a Stored Procedure
Suppose we have a table called employees
with columns id
, name
, and salary
. We want to create a stored procedure that retrieves all employees with a salary greater than a specified threshold. Here's how we can define the stored procedure using a select query:
CREATE OR REPLACE FUNCTION get_high_salary_employees(threshold INTEGER)RETURNS TABLE (id INTEGER, name VARCHAR, salary DECIMAL)AS $$BEGIN RETURN QUERY SELECT id, name, salary FROM employees WHERE salary > threshold;END;$$ LANGUAGE plpgsql;
In this example, the get_high_salary_employees
function takes an INTEGER
parameter called threshold
and returns a resultset with three columns: id
, name
, and salary
. The function uses a select query to retrieve employees with a salary greater than the specified threshold, and the resultset is returned using the RETURN QUERY
statement.
To execute the stored procedure and fetch the resultset, you can use the SELECT
statement like this:
SELECT * FROM get_high_salary_employees(5000);
This will return all employees with a salary greater than 5000.
Related Article: Resolving Access Issues with Query Pg Node in PostgreSQL
Example 2: Using a Select Query with Parameters as a Stored Procedure
In some cases, you may want to pass additional parameters to the select query in the stored procedure. This can be done by defining input parameters in the function signature and using them in the select query. Here's an example:
CREATE OR REPLACE FUNCTION get_employees_by_department(department_id INTEGER)RETURNS TABLE (id INTEGER, name VARCHAR, salary DECIMAL)AS $$BEGIN RETURN QUERY SELECT id, name, salary FROM employees WHERE department_id = get_employees_by_department.department_id;END;$$ LANGUAGE plpgsql;
In this example, the get_employees_by_department
function takes an INTEGER
parameter called department_id
and returns a resultset with three columns: id
, name
, and salary
. The function uses a select query to retrieve employees with the specified department ID, and the resultset is returned using the RETURN QUERY
statement.
To execute the stored procedure and fetch the resultset, you can use the SELECT
statement like this:
SELECT * FROM get_employees_by_department(1);
This will return all employees in the department with ID 1.
Using select queries as stored procedures in PostgreSQL can greatly simplify complex data retrieval operations and make the code more manageable and reusable. It allows you to encapsulate the logic of the query within a single unit, making it easier to maintain and modify over time.
PostgreSQL Stored Procedure Example
To further illustrate the concept of using select queries as stored procedures in PostgreSQL, let's consider an example scenario. Suppose we have a database with two tables: orders
and customers
. The orders
table contains information about customer orders, such as the order ID, customer ID, and order amount. The customers
table contains information about customers, such as the customer ID, name, and email address.
Our goal is to create a stored procedure that retrieves all orders along with the customer information for a given customer ID. Here's how we can define the stored procedure:
CREATE OR REPLACE FUNCTION get_orders_by_customer(customer_id INTEGER)RETURNS TABLE (order_id INTEGER, customer_name VARCHAR, customer_email VARCHAR, order_amount DECIMAL)AS $$BEGIN RETURN QUERY SELECT o.order_id, c.name, c.email, o.amount FROM orders o JOIN customers c ON o.customer_id = c.customer_id WHERE o.customer_id = get_orders_by_customer.customer_id;END;$$ LANGUAGE plpgsql;
In this example, the get_orders_by_customer
function takes an INTEGER
parameter called customer_id
and returns a resultset with four columns: order_id
, customer_name
, customer_email
, and order_amount
. The function uses a select query to retrieve orders along with the customer information by joining the orders
table with the customers
table based on the customer ID. The resultset is returned using the RETURN QUERY
statement.
To execute the stored procedure and fetch the resultset, you can use the SELECT
statement like this:
SELECT * FROM get_orders_by_customer(1);
This will return all orders along with the customer information for the customer with ID 1.
Using stored procedures in PostgreSQL provides a useful way to encapsulate complex data retrieval logic and make it easier to manage and reuse. By using select queries as stored procedures, you can effectively retrieve data from the database and process it in a more efficient and organized manner.
Creating a Stored Procedure in PostgreSQL
In PostgreSQL, a stored procedure is created using the CREATE OR REPLACE FUNCTION
statement. The syntax for creating a stored procedure in PostgreSQL is as follows:
CREATE OR REPLACE FUNCTION function_name([parameter_list])[RETURNS return_type]AS $$BEGIN -- SQL statementsEND;$$ LANGUAGE plpgsql;
Let's break down the different parts of the syntax:
- CREATE OR REPLACE FUNCTION
: This is the statement used to create a stored procedure in PostgreSQL.
- function_name
: This is the name of the stored procedure you want to create. It should follow the naming conventions for PostgreSQL objects.
- parameter_list
: This is an optional list of input parameters for the stored procedure. Each parameter should be specified with its name and data type.
- RETURNS return_type
: This is an optional clause that specifies the return type of the stored procedure. If the stored procedure does not return a resultset, this clause can be omitted.
- $$
: This is the start and end delimiter for the body of the stored procedure. The SQL statements that make up the stored procedure are placed between these delimiters.
- BEGIN
and END
: These keywords define the beginning and end of the body of the stored procedure.
- -- SQL statements
: These are the SQL statements that make up the body of the stored procedure. They can include any valid SQL statements, such as select queries, insert statements, update statements, etc.
Here's an example that creates a simple stored procedure in PostgreSQL:
CREATE OR REPLACE FUNCTION say_hello()RETURNS VOIDAS $$BEGIN RAISE NOTICE 'Hello, World!';END;$$ LANGUAGE plpgsql;
In this example, the say_hello
function does not take any input parameters and does not return a resultset. It simply raises a notice message saying "Hello, World!". The RAISE NOTICE
statement is used to output a message to the PostgreSQL log.
To execute the stored procedure, you can use the SELECT
statement like this:
SELECT say_hello();
This will execute the stored procedure and print the "Hello, World!" message to the PostgreSQL log.
Creating a stored procedure in PostgreSQL allows you to define and encapsulate complex database operations in a single unit, making it easier to manage and reuse code. The flexibility and power of stored procedures make them a valuable tool in the PostgreSQL developer's toolkit.
Related Article: How to Extract Data from PostgreSQL Databases: PSQL ETL
Stored Procedure vs Select Query in PostgreSQL
In PostgreSQL, both stored procedures and select queries can be used to retrieve data from the database. However, there are some key differences between the two.
A select query is a single SQL statement that is used to retrieve data from one or more database tables. It can be executed directly in the PostgreSQL client or embedded in an application code. Select queries are typically used to retrieve data based on certain criteria or to perform calculations and aggregations on the data.
On the other hand, a stored procedure is a set of SQL statements that are stored in the database server and can be executed as a single unit. Stored procedures can be used to perform complex database operations that may require multiple SQL statements to be executed. They are typically used to encapsulate business logic and data manipulation operations, such as inserting, updating, and deleting records.
Here are some key differences between stored procedures and select queries in PostgreSQL:
1. Complexity: Stored procedures can handle more complex operations than select queries. They can include multiple SQL statements, control structures like loops and conditionals, and exception handling. Select queries, on the other hand, are limited to a single SQL statement.
2. Reusability: Stored procedures are more reusable than select queries. Once a stored procedure is defined, it can be called from multiple parts of an application or by different applications. Select queries, on the other hand, need to be rewritten or copied if they need to be reused in multiple places.
3. Maintainability: Stored procedures are easier to maintain than select queries. Since the logic of a stored procedure is encapsulated in a single unit, it's easier to modify and update the logic without affecting other parts of the application. Select queries, on the other hand, are scattered throughout the application code and can be harder to maintain.
4. Performance: Stored procedures can provide better performance than select queries in some cases. Since stored procedures are precompiled and stored in the database server, they can be executed faster than select queries that need to be sent over the network. Additionally, stored procedures can take advantage of caching and optimization techniques provided by the database server.
While both stored procedures and select queries have their own strengths and use cases, the choice between them depends on the specific requirements of your application. If you need to perform complex data manipulation operations or encapsulate business logic, stored procedures are a better choice. If you only need to retrieve data based on certain criteria or perform simple calculations, select queries are sufficient.
PostgreSQL Stored Procedure Tutorial
This tutorial will guide you through the process of creating and using stored procedures in PostgreSQL. We will cover the following topics:
1. Creating a basic stored procedure
2. Passing parameters to a stored procedure
3. Returning resultsets from a stored procedure
4. Error handling in stored procedures
5. Executing a stored procedure
6. Calling a stored procedure from an application
1. Creating a Basic Stored Procedure
To create a basic stored procedure in PostgreSQL, follow these steps:
Step 1: Open a PostgreSQL client, such as psql
or pgAdmin.
Step 2: Use the CREATE OR REPLACE FUNCTION
statement to create a stored procedure. Here's an example:
CREATE OR REPLACE FUNCTION say_hello()RETURNS VOIDAS $$BEGIN RAISE NOTICE 'Hello, World!';END;$$ LANGUAGE plpgsql;
Step 3: Execute the above statement to create the stored procedure.
Step 4: To execute the stored procedure, use the SELECT
statement with the stored procedure name:
SELECT say_hello();
This will execute the stored procedure and print the "Hello, World!" message to the PostgreSQL log.
2. Passing Parameters to a Stored Procedure
To pass parameters to a stored procedure in PostgreSQL, follow these steps:
Step 1: Modify the stored procedure definition to include input parameters. Here's an example:
CREATE OR REPLACE FUNCTION greet_person(person_name VARCHAR)RETURNS VOIDAS $$BEGIN RAISE NOTICE 'Hello, %!', person_name;END;$$ LANGUAGE plpgsql;
Step 2: Execute the above statement to create the modified stored procedure.
Step 3: To execute the stored procedure with a parameter, use the SELECT
statement with the stored procedure name and the parameter value:
SELECT greet_person('John');
This will execute the stored procedure and print the "Hello, John!" message to the PostgreSQL log.
Related Article: Integrating PostgreSQL While Loop into Database Operations
3. Returning Resultsets from a Stored Procedure
To return resultsets from a stored procedure in PostgreSQL, follow these steps:
Step 1: Modify the stored procedure definition to include the RETURNS TABLE
clause. Here's an example:
CREATE OR REPLACE FUNCTION get_employees()RETURNS TABLE (id INTEGER, name VARCHAR, salary DECIMAL)AS $$BEGIN RETURN QUERY SELECT id, name, salary FROM employees;END;$$ LANGUAGE plpgsql;
Step 2: Execute the above statement to create the modified stored procedure.
Step 3: To execute the stored procedure and fetch the resultset, use the SELECT
statement like this:
SELECT * FROM get_employees();
This will return all employees from the employees
table.
4. Error Handling in Stored Procedures
To handle errors in stored procedures in PostgreSQL, you can use the EXCEPTION
block. Here's an example:
CREATE OR REPLACE FUNCTION divide_numbers(a INTEGER, b INTEGER)RETURNS DECIMALAS $$DECLARE result DECIMAL;BEGIN BEGIN result := a / b; EXCEPTION WHEN division_by_zero THEN RAISE EXCEPTION 'Cannot divide by zero'; END; RETURN result;END;$$ LANGUAGE plpgsql;
In this example, the stored procedure divide_numbers
divides two numbers and returns the result. If the division by zero error occurs, the EXCEPTION
block is executed and an exception is raised with the message "Cannot divide by zero".
To execute the stored procedure and handle errors, use the BEGIN...END
block and the EXCEPTION
block like this:
BEGIN SELECT divide_numbers(10, 0);EXCEPTION WHEN OTHERS THEN RAISE NOTICE 'An error occurred: %', SQLERRM;END;
This will execute the stored procedure and handle any errors that occur. If a division by zero error occurs, the error message will be raised to the PostgreSQL log.
5. Executing a Stored Procedure
To execute a stored procedure in PostgreSQL, use the SELECT
statement with the stored procedure name. Here's an example:
SELECT stored_procedure_name([parameter_list]);
Replace stored_procedure_name
with the name of the stored procedure you want to execute, and parameter_list
with the list of input parameters, if any.
For example, to execute the say_hello
stored procedure:
SELECT say_hello();
This will execute the stored procedure and print the "Hello, World!" message to the PostgreSQL log.
6. Calling a Stored Procedure from an Application
To call a stored procedure from an application, you need to use the appropriate database driver and execute the stored procedure using the provided API or query language.
Here's an example using the psycopg2 Python library:
import psycopg2# Connect to the PostgreSQL databaseconn = psycopg2.connect( host="localhost", port=5432, database="mydatabase", user="myuser", password="mypassword")# Create a cursor objectcur = conn.cursor()# Call the stored procedurecur.callproc('say_hello')# Commit the changesconn.commit()# Close the cursor and connectioncur.close()conn.close()
In this example, we use the psycopg2
library to connect to the PostgreSQL database and call the say_hello
stored procedure. The results are committed to the database, and the cursor and connection are closed.
Note that the exact syntax for calling a stored procedure may vary depending on the database driver and programming language you are using. Refer to the documentation of your specific database driver for more information.
Related Article: Executing Efficient Spatial Queries in PostgreSQL
Stored Procedure vs Function in PostgreSQL
In PostgreSQL, both stored procedures and functions are database objects that encapsulate a set of SQL statements. While they are similar in many ways, there are some key differences between the two.
A stored procedure is a set of SQL statements that are stored in the database server and can be executed as a single unit. Stored procedures are typically used to perform complex database operations that may require multiple SQL statements to be executed. They can also be used to encapsulate business logic and data manipulation operations, such as inserting, updating, and deleting records. Stored procedures can have input and output parameters, and they can return resultsets.
On the other hand, a function is a database object that takes input parameters and returns a single value or a resultset. Functions are typically used to perform calculations and transformations on data and can be embedded in SQL statements. Functions can have input and output parameters, and they always return a value or a resultset.
Here are some key differences between stored procedures and functions in PostgreSQL:
1. Return Type: Stored procedures do not have a mandatory return type and can return resultsets or no value (VOID
). Functions, on the other hand, always have a return type and must return a value or a resultset.
2. Usage: Stored procedures are typically used for performing complex database operations and encapsulating business logic. They are often called from application code or other stored procedures. Functions, on the other hand, are used for performing calculations and transformations on data. They are often embedded in SQL statements and can be used in expressions.
3. Resultset Handling: Stored procedures can return multiple resultsets, whereas functions can only return a single resultset or a single value.
4. Transaction Handling: Stored procedures can be executed within a transaction and can participate in the transaction management of the calling code. Functions, on the other hand, are always executed within the context of a transaction and cannot participate in transaction management.
Here's an example that illustrates the difference between a stored procedure and a function in PostgreSQL:
-- Stored ProcedureCREATE OR REPLACE PROCEDURE get_employee_count()AS $$DECLARE count INTEGER;BEGIN SELECT COUNT(*) INTO count FROM employees; RAISE NOTICE 'Total employees: %', count;END;$$ LANGUAGE plpgsql;-- FunctionCREATE OR REPLACE FUNCTION calculate_bonus(salary DECIMAL)RETURNS DECIMALAS $$BEGIN RETURN salary * 0.1;END;$$ LANGUAGE plpgsql;
In this example, the get_employee_count
stored procedure retrieves the total number of employees from the employees
table and raises a notice message with the count. The calculate_bonus
function takes an employee's salary as input and returns the bonus amount, which is calculated as 10% of the salary.
Stored procedures and functions are both useful tools in PostgreSQL that allow you to encapsulate and reuse SQL logic. The choice between them depends on the specific requirements of your application and the nature of the SQL logic you need to encapsulate.
Executing a Stored Procedure in PostgreSQL
To execute a stored procedure in PostgreSQL, you can use the SELECT
statement with the stored procedure name. Here's an example:
SELECT stored_procedure_name([parameter_list]);
Replace stored_procedure_name
with the name of the stored procedure you want to execute, and parameter_list
with the list of input parameters, if any.
For example, to execute the say_hello
stored procedure:
SELECT say_hello();
This will execute the stored procedure and print the "Hello, World!" message to the PostgreSQL log.
If the stored procedure has input parameters, you need to pass the values for those parameters in the SELECT
statement. Here's an example:
SELECT stored_procedure_name(parameter1, parameter2, ...);
Replace parameter1
, parameter2
, etc. with the values for the input parameters.
For example, to execute the greet_person
stored procedure with the name "John":
SELECT greet_person('John');
This will execute the stored procedure and print the "Hello, John!" message to the PostgreSQL log.
Executing a stored procedure in PostgreSQL allows you to run complex database operations and perform business logic encapsulated within the stored procedure. The result of the stored procedure execution can be fetched and processed as needed.
Returning Resultset from Stored Procedure in PostgreSQL
To return a resultset from a stored procedure in PostgreSQL, you can use the RETURNS TABLE
syntax when defining the stored procedure. Here's an example:
CREATE OR REPLACE FUNCTION get_employees()RETURNS TABLE (id INTEGER, name VARCHAR, salary DECIMAL)AS $$BEGIN RETURN QUERY SELECT id, name, salary FROM employees;END;$$ LANGUAGE plpgsql;
In this example, the get_employees
function returns a resultset with three columns: id
, name
, and salary
. The function uses a select query to retrieve the data from the employees
table, and the resultset is returned using the RETURN QUERY
statement.
To execute the stored procedure and fetch the resultset, you can use the SELECT
statement like this:
SELECT * FROM get_employees();
This will return all employees from the employees
table.
If the stored procedure has input parameters, you need to pass the values for those parameters in the SELECT
statement. Here's an example:
SELECT stored_procedure_name(parameter1, parameter2, ...);
Replace stored_procedure_name
with the name of the stored procedure, parameter1
, parameter2
, etc. with the values for the input parameters.
For example, to execute the get_high_salary_employees
stored procedure with a threshold of 5000:
SELECT * FROM get_high_salary_employees(5000);
This will return all employees with a salary greater than 5000.
Returning a resultset from a stored procedure in PostgreSQL allows you to encapsulate complex data retrieval logic within a single unit. The resultset can be fetched and processed as needed, providing a flexible and efficient way to retrieve data from the database.
Best Practices for Using Stored Procedures in PostgreSQL
Using stored procedures in PostgreSQL can greatly simplify complex data retrieval and manipulation operations, and make the code more manageable and reusable. Here are some best practices to consider when using stored procedures in PostgreSQL:
1. Plan for Reusability: When designing stored procedures, think about how they can be reused in different parts of your application or by different applications. Consider the input parameters and return types carefully to make the stored procedures as flexible and reusable as possible.
2. Keep It Simple: Try to keep the logic of your stored procedures as simple as possible. Avoid unnecessary complexity and favor clarity and readability. This will make the stored procedures easier to understand, modify, and maintain over time.
3. Use Transactions: If your stored procedures perform data manipulation operations, consider using transactions to ensure data integrity. Use the BEGIN
, COMMIT
, and ROLLBACK
statements to define transaction boundaries and handle exceptions.
4. Handle Errors Gracefully: Always include error handling logic in your stored procedures to handle exceptions and errors that may occur during execution. Use the EXCEPTION
block to catch and handle specific exceptions, and consider logging or reporting the errors for debugging purposes.
5. Test Thoroughly: Before deploying your stored procedures to production, make sure to test them thoroughly in a controlled environment. Test different scenarios, edge cases, and input values to ensure that the stored procedures perform as expected and return the correct results.
6. Document Your Stored Procedures: Document each stored procedure with a clear description, input parameters, return types, and any special considerations. This will make it easier for other developers to understand and use your stored procedures.
7. Monitor Performance: Keep an eye on the performance of your stored procedures and optimize them as needed. Use the PostgreSQL query planner and execution statistics to identify bottlenecks and areas for improvement.
8. Consider Security: When using stored procedures, be mindful of potential security risks. Make sure to apply appropriate access controls and permissions to prevent unauthorized access or modification of data.
Related Article: How to Truncate Tables in PostgreSQL
Additional Resources
- PostgreSQL: Documentation: 11: 41.3. Using Stored Procedures