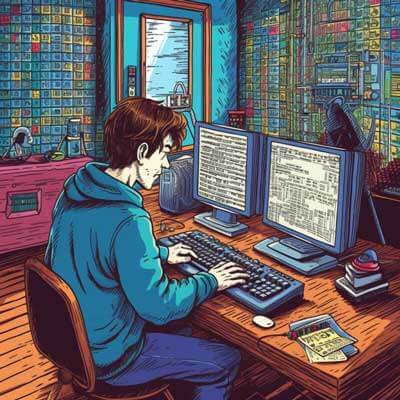
Table of Contents
Assigning Select Query Results to Variables
In PostgreSQL, you can assign the results of a SELECT query to variables for further processing. This can be useful when you want to store specific values from a query result and use them later in your code.
To assign the results of a SELECT query to a variable, you can use the SELECT INTO statement. Here's the syntax:
SELECT column_name INTO variable_name FROM table_name WHERE condition;
Let's say we have a table called "employees" with columns "id" and "name". We want to assign the name of an employee with a specific ID to a variable. Here's an example:
DECLARE employee_name employees.name%TYPE; BEGIN SELECT name INTO employee_name FROM employees WHERE id = 1; RAISE NOTICE 'Employee name: %', employee_name; END;
In this example, we declare a variable called "employee_name" with the same data type as the "name" column in the "employees" table. We then use the SELECT INTO statement to assign the name of an employee with ID 1 to the "employee_name" variable. Finally, we use the RAISE NOTICE statement to display the value of the variable.
You can use this technique to assign any column value to a variable. Just make sure the data type of the variable matches the data type of the column.
Related Article: How to Drop All Tables in a PostgreSQL Database
Saving Select Query Results to Variables
In addition to assigning the results of a SELECT query to variables, you can also save the entire result set into a variable for further processing. This can be useful when you want to perform multiple operations on the result set without executing the query multiple times.
To save the results of a SELECT query to a variable, you can use the SELECT INTO statement with the ARRAY type. Here's the syntax:
SELECT ARRAY(SELECT column_name FROM table_name WHERE condition) INTO variable_name;
Let's say we have a table called "products" with a column called "price". We want to save all the prices of products with a specific category into an array variable. Here's an example:
DECLARE product_prices numeric[]; BEGIN SELECT ARRAY(SELECT price FROM products WHERE category = 'Electronics') INTO product_prices; RAISE NOTICE 'Product prices: %', product_prices; END;
In this example, we declare an array variable called "product_prices" of type numeric[]. We then use the SELECT INTO statement with the ARRAY type to save all the prices of products with the category 'Electronics' into the "product_prices" variable. Finally, we use the RAISE NOTICE statement to display the values stored in the variable.
You can use this technique to save any column values to an array variable. Just make sure the data type of the array variable matches the data type of the column.
Capturing Select Query Results in Variables
Apart from assigning or saving the results of a SELECT query to variables, you can also capture the query output in variables using the FETCH statement. This can be useful when you want to fetch the query results row by row and perform operations on each row individually.
To capture the results of a SELECT query in variables, you can use the FETCH statement with the INTO clause. Here's the syntax:
FETCH [direction] FROM cursor_name INTO variable_name1 [, variable_name2, ...];
Let's say we have a cursor called "employee_cursor" that fetches the employee IDs and names from the "employees" table. We want to capture the employee IDs and names in separate variables. Here's an example:
DECLARE employee_id integer; employee_name text; employee_cursor CURSOR FOR SELECT id, name FROM employees; BEGIN OPEN employee_cursor; LOOP FETCH NEXT FROM employee_cursor INTO employee_id, employee_name; EXIT WHEN NOT FOUND; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_id, employee_name; END LOOP; CLOSE employee_cursor; END;
In this example, we declare two variables, "employee_id" of type integer and "employee_name" of type text. We then open the "employee_cursor" cursor, which fetches the employee IDs and names from the "employees" table. Inside the loop, we use the FETCH statement to capture each row of the query result in the variables "employee_id" and "employee_name". We then use the RAISE NOTICE statement to display the values of the variables. The loop continues until there are no more rows to fetch. Finally, we close the cursor.
You can use this technique to capture any column values in variables row by row. Just make sure the data types of the variables match the data types of the columns.
Storing Output of Select Query in Variables
To store the output of a SELECT query in variables in PostgreSQL, you can use various techniques such as SELECT INTO, ARRAY, and FETCH. These techniques allow you to assign specific column values, save the entire result set, or capture the query output row by row into variables for further processing.
Let's summarize the syntax for each technique:
- Assigning Select Query Results to Variables:
SELECT column_name INTO variable_name FROM table_name WHERE condition;
- Saving Select Query Results to Variables:
SELECT ARRAY(SELECT column_name FROM table_name WHERE condition) INTO variable_name;
- Capturing Select Query Results in Variables:
FETCH [direction] FROM cursor_name INTO variable_name1 [, variable_name2, ...];
Using these techniques, you can easily store the output of a SELECT query in variables and manipulate the data as needed. Whether you need to assign specific values, save the entire result set, or process the output row by row, PostgreSQL provides the necessary tools to handle these scenarios.
Related Article: Using Select Query as a Stored Procedure in PostgreSQL
Syntax for Assigning Select Query Results to Variables
To assign the results of a SELECT query to variables in PostgreSQL, you can use the SELECT INTO statement. This statement allows you to retrieve specific column values from a query result and store them in variables.
Here's the syntax for assigning select query results to variables:
SELECT column_name INTO variable_name FROM table_name WHERE condition;
Let's break down the syntax:
- SELECT: This keyword is used to specify the columns to retrieve from the table.
- column_name: This is the name of the column you want to assign to the variable.
- INTO: This keyword is used to indicate that the query result should be assigned to a variable.
- variable_name: This is the name of the variable you want to assign the query result to.
- FROM: This keyword is used to specify the table from which to retrieve the data.
- table_name: This is the name of the table from which you want to retrieve the data.
- WHERE: This keyword is used to specify any conditions for filtering the data.
- condition: This is the condition or criteria that the data must meet to be included in the result.
Here's an example that demonstrates the syntax:
DECLARE employee_name employees.name%TYPE; BEGIN SELECT name INTO employee_name FROM employees WHERE id = 1; RAISE NOTICE 'Employee name: %', employee_name; END;
In this example, we declare a variable called "employee_name" with the same data type as the "name" column in the "employees" table. We then use the SELECT INTO statement to assign the name of an employee with ID 1 to the "employee_name" variable. Finally, we use the RAISE NOTICE statement to display the value of the variable.
You can use this syntax to assign any column value to a variable. Just make sure the data type of the variable matches the data type of the column.
Saving Output of Select Query to Variables
In PostgreSQL, you can save the output of a SELECT query to variables for further processing. This can be useful when you want to perform multiple operations on the result set without executing the query multiple times.
To save the output of a SELECT query to variables, you can use the SELECT INTO statement with the ARRAY type. This allows you to store the entire result set in a variable as an array.
Here's the syntax for saving the output of a SELECT query to variables:
SELECT ARRAY(SELECT column_name FROM table_name WHERE condition) INTO variable_name;
Let's break down the syntax:
- SELECT: This keyword is used to specify the columns to retrieve from the table.
- column_name: This is the name of the column you want to include in the array.
- INTO: This keyword is used to indicate that the query result should be saved to a variable.
- ARRAY: This is the keyword that specifies the data type of the variable as an array.
- table_name: This is the name of the table from which you want to retrieve the data.
- WHERE: This keyword is used to specify any conditions for filtering the data.
- condition: This is the condition or criteria that the data must meet to be included in the result.
Here's an example that demonstrates the syntax:
DECLARE product_prices numeric[]; BEGIN SELECT ARRAY(SELECT price FROM products WHERE category = 'Electronics') INTO product_prices; RAISE NOTICE 'Product prices: %', product_prices; END;
In this example, we declare an array variable called "product_prices" of type numeric[]. We then use the SELECT INTO statement with the ARRAY type to save all the prices of products with the category 'Electronics' into the "product_prices" variable. Finally, we use the RAISE NOTICE statement to display the values stored in the variable.
You can use this syntax to save any column values to an array variable. Just make sure the data type of the array variable matches the data type of the column.
Capturing Output of Select Query in Variables
In PostgreSQL, you can capture the output of a SELECT query in variables using the FETCH statement. This allows you to fetch the query results row by row and perform operations on each row individually.
To capture the output of a SELECT query in variables, you can use the FETCH statement with the INTO clause. This allows you to specify the variables in which you want to store the query result.
Here's the syntax for capturing the output of a SELECT query in variables:
FETCH [direction] FROM cursor_name INTO variable_name1 [, variable_name2, ...];
Let's break down the syntax:
- FETCH: This keyword is used to retrieve rows from a cursor.
- direction: This is an optional parameter that specifies the direction in which to fetch the rows. It can be NEXT, PRIOR, FIRST, LAST, ABSOLUTE n, or RELATIVE n.
- FROM: This keyword is used to specify the cursor from which to fetch the rows.
- cursor_name: This is the name of the cursor from which you want to fetch the rows.
- INTO: This keyword is used to indicate that the fetched rows should be stored in variables.
- variable_name1, variable_name2, ...: These are the variables in which you want to store the fetched rows.
Here's an example that demonstrates the syntax:
DECLARE employee_id integer; employee_name text; employee_cursor CURSOR FOR SELECT id, name FROM employees; BEGIN OPEN employee_cursor; LOOP FETCH NEXT FROM employee_cursor INTO employee_id, employee_name; EXIT WHEN NOT FOUND; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_id, employee_name; END LOOP; CLOSE employee_cursor; END;
In this example, we declare two variables, "employee_id" of type integer and "employee_name" of type text. We then open the "employee_cursor" cursor, which fetches the employee IDs and names from the "employees" table. Inside the loop, we use the FETCH statement to capture each row of the query result in the variables "employee_id" and "employee_name". We then use the RAISE NOTICE statement to display the values of the variables. The loop continues until there are no more rows to fetch. Finally, we close the cursor.
You can use this syntax to capture any column values in variables row by row. Just make sure the data types of the variables match the data types of the columns.
Assigning Select Results to Variables in PostgreSQL
In PostgreSQL, you can assign the results of a SELECT query to variables for further processing. This can be useful when you want to store specific values from a query result and use them later in your code.
To assign the results of a SELECT query to variables, you can use the SELECT INTO statement. This statement allows you to retrieve specific column values from a query result and store them in variables.
Here's the syntax for assigning select results to variables:
SELECT column_name INTO variable_name FROM table_name WHERE condition;
Let's say we have a table called "employees" with columns "id" and "name". We want to assign the name of an employee with a specific ID to a variable. Here's an example:
DECLARE employee_name employees.name%TYPE; BEGIN SELECT name INTO employee_name FROM employees WHERE id = 1; RAISE NOTICE 'Employee name: %', employee_name; END;
In this example, we declare a variable called "employee_name" with the same data type as the "name" column in the "employees" table. We then use the SELECT INTO statement to assign the name of an employee with ID 1 to the "employee_name" variable. Finally, we use the RAISE NOTICE statement to display the value of the variable.
You can use this syntax to assign any column value to a variable. Just make sure the data type of the variable matches the data type of the column.
Here's another example that demonstrates assigning multiple column values to variables:
DECLARE employee_id employees.id%TYPE; employee_name employees.name%TYPE; BEGIN SELECT id, name INTO employee_id, employee_name FROM employees WHERE id = 1; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_id, employee_name; END;
In this example, we declare two variables, "employee_id" and "employee_name", with the same data types as the corresponding columns in the "employees" table. We then use the SELECT INTO statement to assign the ID and name of an employee with ID 1 to the variables. Finally, we use the RAISE NOTICE statement to display the values of the variables.
You can use this syntax to assign multiple column values to multiple variables in a single query.
Related Article: How to Disable IPv6 in PostgreSQL Databases
Syntax for Saving Select Query Output to Variables in PostgreSQL
To save the output of a SELECT query to variables in PostgreSQL, you can use the SELECT INTO statement with the ARRAY type. This allows you to store the entire result set in a variable as an array.
Here's the syntax for saving select query output to variables:
SELECT ARRAY(SELECT column_name FROM table_name WHERE condition) INTO variable_name;
Let's say we have a table called "products" with a column called "price". We want to save all the prices of products with a specific category into an array variable. Here's an example:
DECLARE product_prices numeric[]; BEGIN SELECT ARRAY(SELECT price FROM products WHERE category = 'Electronics') INTO product_prices; RAISE NOTICE 'Product prices: %', product_prices; END;
In this example, we declare an array variable called "product_prices" of type numeric[]. We then use the SELECT INTO statement with the ARRAY type to save all the prices of products with the category 'Electronics' into the "product_prices" variable. Finally, we use the RAISE NOTICE statement to display the values stored in the variable.
You can use this syntax to save any column values to an array variable. Just make sure the data type of the array variable matches the data type of the column.
Here's another example that demonstrates saving multiple column values to multiple variables:
DECLARE employee_ids integer[]; employee_names text[]; BEGIN SELECT ARRAY(SELECT id FROM employees) INTO employee_ids; SELECT ARRAY(SELECT name FROM employees) INTO employee_names; RAISE NOTICE 'Employee IDs: %', employee_ids; RAISE NOTICE 'Employee Names: %', employee_names; END;
In this example, we declare two array variables, "employee_ids" of type integer[] and "employee_names" of type text[]. We then use the SELECT INTO statement with the ARRAY type to save all the IDs and names of employees into the respective variables. Finally, we use the RAISE NOTICE statement to display the values stored in the variables.
You can use this syntax to save multiple column values to multiple variables in a single query.
Storing Select Query Results in Variables: A How-To Guide
In PostgreSQL, storing select query results in variables can be a useful technique for manipulating and processing data. Whether you need to assign specific column values, save the entire result set, or capture the query output row by row, PostgreSQL provides a variety of methods to accomplish these tasks. In this guide, we will explore different techniques for storing select query results in variables and provide step-by-step examples to illustrate their usage.
Assigning Select Query Results to Variables in PostgreSQL
To assign the results of a SELECT query to variables in PostgreSQL, you can use the SELECT INTO statement. This statement allows you to retrieve specific column values from a query result and store them in variables.
Here's an example that demonstrates how to assign the name of an employee with a specific ID to a variable:
DECLARE employee_name employees.name%TYPE; BEGIN SELECT name INTO employee_name FROM employees WHERE id = 1; RAISE NOTICE 'Employee name: %', employee_name; END;
In this example, we declare a variable called "employee_name" with the same data type as the "name" column in the "employees" table. We then use the SELECT INTO statement to assign the name of an employee with ID 1 to the "employee_name" variable. Finally, we use the RAISE NOTICE statement to display the value of the variable.
You can use this technique to assign any column value to a variable. Just make sure the data type of the variable matches the data type of the column.
Here's another example that demonstrates assigning multiple column values to variables:
DECLARE employee_id employees.id%TYPE; employee_name employees.name%TYPE; BEGIN SELECT id, name INTO employee_id, employee_name FROM employees WHERE id = 1; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_id, employee_name; END;
In this example, we declare two variables, "employee_id" and "employee_name", with the same data types as the corresponding columns in the "employees" table. We then use the SELECT INTO statement to assign the ID and name of an employee with ID 1 to the variables. Finally, we use the RAISE NOTICE statement to display the values of the variables.
You can use this technique to assign multiple column values to multiple variables in a single query.
Saving Select Query Results to Variables: Step-by-Step
To save the output of a SELECT query to variables in PostgreSQL, you can use the SELECT INTO statement with the ARRAY type. This allows you to store the entire result set in a variable as an array.
Here's an example that demonstrates how to save all the prices of products with a specific category into an array variable:
DECLARE product_prices numeric[]; BEGIN SELECT ARRAY(SELECT price FROM products WHERE category = 'Electronics') INTO product_prices; RAISE NOTICE 'Product prices: %', product_prices; END;
In this example, we declare an array variable called "product_prices" of type numeric[]. We then use the SELECT INTO statement with the ARRAY type to save all the prices of products with the category 'Electronics' into the "product_prices" variable. Finally, we use the RAISE NOTICE statement to display the values stored in the variable.
You can use this technique to save any column values to an array variable. Just make sure the data type of the array variable matches the data type of the column.
Here's another example that demonstrates saving multiple column values to multiple variables:
DECLARE employee_ids integer[]; employee_names text[]; BEGIN SELECT ARRAY(SELECT id FROM employees) INTO employee_ids; SELECT ARRAY(SELECT name FROM employees) INTO employee_names; RAISE NOTICE 'Employee IDs: %', employee_ids; RAISE NOTICE 'Employee Names: %', employee_names; END;
In this example, we declare two array variables, "employee_ids" of type integer[] and "employee_names" of type text[]. We then use the SELECT INTO statement with the ARRAY type to save all the IDs and names of employees into the respective variables. Finally, we use the RAISE NOTICE statement to display the values stored in the variables.
You can use this technique to save multiple column values to multiple variables in a single query.
Related Article: How To Change a PostgreSQL User Password
Capturing Select Query Results in Variables: A Practical Approach
Apart from assigning or saving the results of a SELECT query to variables, you can also capture the query output in variables using the FETCH statement. This allows you to fetch the query results row by row and perform operations on each row individually.
Here's an example that demonstrates how to capture the employee IDs and names from the "employees" table in variables:
DECLARE employee_id integer; employee_name text; employee_cursor CURSOR FOR SELECT id, name FROM employees; BEGIN OPEN employee_cursor; LOOP FETCH NEXT FROM employee_cursor INTO employee_id, employee_name; EXIT WHEN NOT FOUND; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_id, employee_name; END LOOP; CLOSE employee_cursor; END;
In this example, we declare two variables, "employee_id" of type integer and "employee_name" of type text. We then open the "employee_cursor" cursor, which fetches the employee IDs and names from the "employees" table. Inside the loop, we use the FETCH statement to capture each row of the query result in the variables "employee_id" and "employee_name". We then use the RAISE NOTICE statement to display the values of the variables. The loop continues until there are no more rows to fetch. Finally, we close the cursor.
You can use this technique to capture any column values in variables row by row. Just make sure the data types of the variables match the data types of the columns.
Assigning Select Results to Variables in PostgreSQL: Best Practices
When assigning select results to variables in PostgreSQL, it's important to follow best practices to ensure efficient and reliable code. Here are some best practices to consider:
1. Use the correct data types: Make sure the data types of the variables match the data types of the columns you are assigning or capturing. This helps prevent data conversion errors and ensures the variables can hold the values properly.
2. Use descriptive variable names: Choose meaningful names for your variables that accurately reflect their purpose. This improves code readability and makes it easier for other developers to understand your code.
3. Handle exceptions: When assigning or capturing select results in variables, be prepared to handle exceptions that may occur. Use try-catch blocks or error handling mechanisms to gracefully handle any errors that may arise during the process.
4. Limit the number of variables: Avoid declaring unnecessary variables. Only declare variables that are necessary for storing the select results. This helps keep your code clean and reduces the risk of confusion or errors.
5. Close cursors after use: If you are using cursors to capture select results in variables, make sure to close the cursor after you have finished processing the data. This helps release system resources and improves performance.
Storing Select Query Results in Variables: Tips and Tricks
Storing select query results in variables in PostgreSQL can be a useful technique for manipulating and processing data. Here are some tips and tricks to help you effectively use this feature:
1. Use the correct data types: When declaring variables to store select query results, make sure the data types of the variables match the data types of the columns you are assigning or capturing. This helps prevent data conversion errors and ensures the variables can hold the values properly.
2. Consider performance implications: Storing select query results in variables can have performance implications, especially if you are dealing with large result sets. Be mindful of the amount of data you are storing in variables and consider whether it is necessary to store the entire result set or just specific values.
3. Use cursors for large result sets: If you are dealing with a large result set, consider using cursors to fetch the data row by row instead of storing the entire result set in variables. This can help improve performance and reduce memory usage.
4. Handle NULL values: When assigning select query results to variables, be aware that NULL values can be returned. Make sure to handle NULL values appropriately in your code to avoid any unexpected behavior.
5. Use descriptive variable names: Choose meaningful names for your variables that accurately reflect their purpose. This improves code readability and makes it easier for other developers to understand your code.
6. Consider using arrays: If you need to store multiple values from a select query, consider using arrays to store the values in a single variable. This can help simplify your code and make it more efficient.
7. Be mindful of scope: When declaring variables to store select query results, make sure to define their scope appropriately. Variables declared within a block or a function are only accessible within that block or function.
8. Test and debug your code: Always test your code thoroughly and make use of debugging tools to ensure that your variables are storing the correct values. This can help identify any issues or unexpected behavior early on.
Capturing Select Query Results in Variables: Common Pitfalls
When capturing select query results in variables in PostgreSQL, it's important to be aware of common pitfalls that can lead to errors or unexpected behavior. Here are some common pitfalls to watch out for:
1. Forgetting to open the cursor: If you are using a cursor to capture select query results in variables, make sure to open the cursor before fetching data from it. Failure to open the cursor will result in an error.
2. Forgetting to close the cursor: After you have finished processing the data, make sure to close the cursor to release system resources. Failure to close the cursor can lead to resource leaks and performance issues.
3. Not checking for the end of the result set: When using a cursor to fetch data row by row, make sure to check for the end of the result set using the NOT FOUND condition. Failure to do so can result in an infinite loop or incorrect processing of data.
4. Incorrect variable data types: Ensure that the data types of the variables you are capturing the select query results in match the data types of the columns you are fetching. Failure to do so can result in data conversion errors or unexpected behavior.
5. Handling NULL values: When capturing select query results in variables, be aware that NULL values can be returned. Make sure to handle NULL values appropriately in your code to avoid any unexpected behavior.
6. Scope of variables: Make sure to define the scope of your variables appropriately. Variables declared within a block or a function are only accessible within that block or function.
7. Performance considerations: Be mindful of the performance implications of capturing select query results in variables, especially if you are dealing with large result sets. Consider whether it is necessary to store the entire result set or just specific values.
8. Testing and debugging: Always test your code thoroughly and make use of debugging tools to ensure that your variables are capturing the correct values. This can help identify any issues or unexpected behavior early on.
Related Article: Tutorial: Modulo Operator in PostgreSQL Databases
Assigning Select Query Results to Variables: Dos and Don'ts
When assigning select query results to variables in PostgreSQL, it's important to follow best practices and avoid common pitfalls. Here are some dos and don'ts to keep in mind:
Dos:
1. Do use the correct data types: Make sure the data types of the variables match the data types of the columns you are assigning. This helps prevent data conversion errors and ensures the variables can hold the values properly.
2. Do use descriptive variable names: Choose meaningful names for your variables that accurately reflect their purpose. This improves code readability and makes it easier for other developers to understand your code.
3. Do handle exceptions: Be prepared to handle exceptions that may occur when assigning select query results to variables. Use try-catch blocks or error handling mechanisms to gracefully handle any errors that may arise during the process.
4. Do limit the number of variables: Avoid declaring unnecessary variables. Only declare variables that are necessary for storing the select results. This helps keep your code clean and reduces the risk of confusion or errors.
5. Do test your code: Always test your code thoroughly to ensure that your variables are storing the correct values. Use test cases that cover different scenarios and edge cases to validate the behavior of your code.
Don'ts:
1. Don't forget to check for NULL values: When assigning select query results to variables, be aware that NULL values can be returned. Make sure to handle NULL values appropriately in your code to avoid any unexpected behavior.
2. Don't use ambiguous variable names: Avoid using generic or ambiguous variable names that do not provide any meaningful information about the data they store. This can make your code harder to read and understand.
3. Don't forget to close cursors: If you are using cursors to assign select query results to variables, make sure to close the cursor after you have finished processing the data. Failure to close the cursor can lead to resource leaks and performance issues.
4. Don't ignore performance considerations: Be mindful of the performance implications of assigning select query results to variables, especially if you are dealing with large result sets. Consider whether it is necessary to store the entire result set or just specific values.
5. Don't skip error handling: Always handle errors that may occur when assigning select query results to variables. Ignoring error handling can result in unexpected behavior and make it difficult to debug issues in your code.
Saving Select Query Results to Variables in PostgreSQL: In-Depth
When working with select query results in PostgreSQL, you may need to save them to variables for further processing. This can be useful when you want to perform multiple operations on the result set without executing the query multiple times. PostgreSQL provides several ways to save select query results to variables, giving you flexibility in how you handle and manipulate the data. In this section, we will explore these techniques in-depth and provide examples to illustrate their usage.
Using the SELECT INTO Statement
One way to save select query results to variables in PostgreSQL is to use the SELECT INTO statement. This statement allows you to assign specific column values from a query result to variables.
Here's an example that demonstrates how to save the name of an employee with a specific ID to a variable:
DECLARE employee_name employees.name%TYPE; BEGIN SELECT name INTO employee_name FROM employees WHERE id = 1; RAISE NOTICE 'Employee name: %', employee_name; END;
In this example, we declare a variable called "employee_name" with the same data type as the "name" column in the "employees" table. We then use the SELECT INTO statement to assign the name of an employee with ID 1 to the "employee_name" variable. Finally, we use the RAISE NOTICE statement to display the value of the variable.
You can use this technique to save any column value to a variable. Just make sure the data type of the variable matches the data type of the column.
Using the ARRAY Type
Another way to save select query results to variables in PostgreSQL is to use the ARRAY type. This allows you to save the entire result set as an array variable.
Here's an example that demonstrates how to save all the prices of products with a specific category into an array variable:
DECLARE product_prices numeric[]; BEGIN SELECT ARRAY(SELECT price FROM products WHERE category = 'Electronics') INTO product_prices; RAISE NOTICE 'Product prices: %', product_prices; END;
In this example, we declare an array variable called "product_prices" of type numeric[]. We then use the SELECT INTO statement with the ARRAY type to save all the prices of products with the category 'Electronics' into the "product_prices" variable. Finally, we use the RAISE NOTICE statement to display the values stored in the variable.
You can use this technique to save any column values to an array variable. Just make sure the data type of the array variable matches the data type of the column.
Using Cursors
Cursors provide another way to save select query results to variables in PostgreSQL. Cursors allow you to fetch the query output row by row and store the values in variables.
Here's an example that demonstrates how to save the employee IDs and names from the "employees" table in variables using a cursor:
DECLARE employee_id integer; employee_name text; employee_cursor CURSOR FOR SELECT id, name FROM employees; BEGIN OPEN employee_cursor; LOOP FETCH NEXT FROM employee_cursor INTO employee_id, employee_name; EXIT WHEN NOT FOUND; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_id, employee_name; END LOOP; CLOSE employee_cursor; END;
In this example, we declare two variables, "employee_id" of type integer and "employee_name" of type text. We then open the "employee_cursor" cursor, which fetches the employee IDs and names from the "employees" table. Inside the loop, we use the FETCH statement to capture each row of the query result in the variables "employee_id" and "employee_name". We then use the RAISE NOTICE statement to display the values of the variables. The loop continues until there are no more rows to fetch. Finally, we close the cursor.
You can use this technique to save any column values to variables row by row. Just make sure the data types of the variables match the data types of the columns.
Capturing Output of Select Query in Variables: Advanced Techniques
Capturing the output of a select query in variables in PostgreSQL opens up a world of possibilities for advanced data manipulation and processing. In addition to the basic techniques we have covered, there are several advanced techniques you can use to harness the full power of this feature. In this section, we will explore some of these techniques and provide examples to illustrate their usage.
Using Record Variables
Record variables in PostgreSQL allow you to capture the output of a select query that returns multiple columns into a single variable. This can be useful when you want to perform complex operations on the query result without the need to declare separate variables for each column.
Here's an example that demonstrates how to capture the employee ID and name in a record variable:
DECLARE employee_record employees%ROWTYPE; BEGIN SELECT * INTO employee_record FROM employees WHERE id = 1; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_record.id, employee_record.name; END;
In this example, we declare a record variable called "employee_record" of type "employees%ROWTYPE". We then use the SELECT INTO statement to capture the output of the query that selects all columns from the "employees" table into the "employee_record" variable. Finally, we use the RAISE NOTICE statement to display the values of the individual columns within the record variable.
You can use this technique to capture the output of a select query with any number of columns into a record variable. Just make sure the record variable is defined with the same structure as the query result.
Using Composite Types
Composite types in PostgreSQL allow you to define custom data types that can be used to capture the output of select queries. This can be useful when you want to encapsulate related columns into a single variable and pass it around as a single unit.
Here's an example that demonstrates how to define a composite type and capture the employee ID and name in a variable of that type:
CREATE TYPE employee_info AS ( id integer, name text ); DECLARE employee_data employee_info; BEGIN SELECT id, name INTO employee_data FROM employees WHERE id = 1; RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_data.id, employee_data.name; END;
In this example, we first create a composite type called "employee_info" that consists of an "id" column of type integer and a "name" column of type text. We then declare a variable called "employee_data" of type "employee_info". We use the SELECT INTO statement to capture the employee ID and name from the "employees" table into the "employee_data" variable. Finally, we use the RAISE NOTICE statement to display the values of the individual fields within the composite variable.
You can use this technique to define custom composite types for capturing the output of select queries with any number of columns. Just make sure the composite variable is defined with the same structure as the query result.
Using Arrays of Composite Types
Building on the previous technique, you can also use arrays of composite types to capture the output of select queries that return multiple rows. This can be useful when you want to store a collection of related rows in a single variable.
Here's an example that demonstrates how to define an array of a composite type and capture the employee ID and name for multiple employees:
CREATE TYPE employee_info AS ( id integer, name text ); DECLARE employee_data employee_info[]; BEGIN SELECT ARRAY(SELECT ROW(id, name) FROM employees) INTO employee_data; FOR i IN 1..array_length(employee_data, 1) LOOP RAISE NOTICE 'Employee ID: %, Employee Name: %', employee_data[i].id, employee_data[i].name; END LOOP; END;
In this example, we first create a composite type called "employee_info" that consists of an "id" column of type integer and a "name" column of type text. We then declare a variable called "employee_data" of type "employee_info[]", which is an array of the composite type. We use the SELECT INTO statement with the ARRAY type to capture the employee ID and name for all employees from the "employees" table into the "employee_data" variable. Finally, we use a loop to iterate over the elements of the array and use the RAISE NOTICE statement to display the values of the individual fields within each composite variable.
You can use this technique to capture the output of select queries that return multiple rows into arrays of composite types. Just make sure the composite type and the array variable are defined with the same structure as the query result.