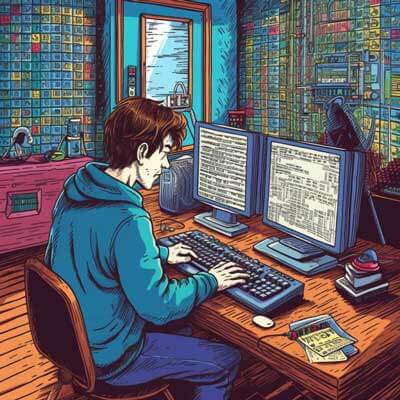
- The Role of Typename in GraphQL
- The GraphQL Type System
- The GraphQL Schema
- GraphQL Introspection
- GraphQL Query
- GraphQL Mutation
- GraphQL Subscription
- GraphQL Scalar Types
- GraphQL Directive
- GraphQL Resolver
- GraphQL Client
- Purpose of Typename in GraphQL
- How Typename Works in GraphQL
- When to Use Typename in GraphQL
- Changing Typename in a GraphQL Query
- Available Scalar Types in GraphQL
- Defining a Custom Scalar Type in GraphQL
- Role of a Resolver in GraphQL
- Implementing a Resolver in GraphQL
- Differences Between Query and Mutation in GraphQL
- Purpose of a Directive in GraphQL
- Additional Resources
The Role of Typename in GraphQL
In GraphQL, the __typename
field is a special field that can be used to retrieve the name of the type of an object. It is automatically added to every GraphQL object type and can be included in the selection set of a query or mutation.
The main role of __typename
is to provide information about the type of an object returned by a GraphQL query. This is particularly useful when dealing with polymorphic types, where an object can be of different types depending on the context. By including __typename
in the selection set, the client can determine the specific type of the returned object and perform appropriate actions based on its type.
For example, let’s say we have a GraphQL schema with two types: Post
and Comment
. Both types have a text
field, but the Post
type also has an additional title
field. If we query for both posts
and comments
, we can use __typename
to differentiate between the two types:
query { posts { __typename title text } comments { __typename text } }
The __typename
field will return either "Post"
or "Comment"
for each object, allowing the client to handle them differently based on their type.
It’s important to note that __typename
is not a reserved keyword in GraphQL and can be used as a regular field name if desired. However, when used as a regular field, it will not provide the type information and behave like any other field.
Related Article: Managing Data Queries with GraphQL Count
The GraphQL Type System
The GraphQL type system is a key component of GraphQL that defines the structure and behavior of the data available in a GraphQL API. It consists of various types that can be used to define the shape of data and relationships between them.
GraphQL provides several built-in scalar types, such as String
, Int
, Float
, Boolean
, and ID
, which represent basic data types. Additionally, GraphQL allows defining custom object types, enum types, interface types, union types, and input types.
Object types represent complex objects with multiple fields. Each field has a name and a type, and can also have arguments. For example, in a blog schema, we might have an Author
object type with fields like name
, email
, and posts
.
Enum types represent a finite set of possible values. For example, we might have an Enum
type called Status
with values like PUBLISHED
and DRAFT
.
Interface types define a common set of fields that other types can implement. They are useful for defining shared behavior across multiple types. For example, we might have an Entity
interface with a createdAt
field that both Post
and Comment
implement.
Union types represent a type that can be one of several other types. They are useful when a field can return different types of objects. For example, we might have a SearchResult
union type that can represent either a Post
or a Comment
object.
Input types represent the input arguments for a field. They are used when defining the arguments for a field in a query or mutation. For example, we might have an Input
type called PostInput
with fields like title
and text
that can be used when creating a new post.
The GraphQL Schema
The GraphQL schema is a central piece of a GraphQL API. It defines the available types, fields, and relationships in the API, and serves as a contract between the client and server.
The schema is usually defined using the GraphQL Schema Definition Language (SDL), which provides a concise and human-readable syntax for defining the schema. It consists of two main parts: the type definitions and the root types.
The type definitions define the types used in the API, including object types, enum types, interface types, union types, and input types. Each type is defined with its fields, arguments, and their types.
Here’s an example of a simple schema that defines a Post
type with fields for title
and text
:
type Post { title: String text: String }
The root types define the entry points for querying, mutating, and subscribing to data in the API. There are three root types: Query
, Mutation
, and Subscription
. Each root type has fields that correspond to the different operations.
Here’s an example of a schema with a Query
root type:
type Query { post(id: ID!): Post posts: [Post] }
This schema defines a Query
type with two fields: post
and posts
. The post
field takes an id
argument of type ID!
and returns a Post
object. The posts
field returns a list of Post
objects.
GraphQL Introspection
GraphQL introspection is a feature that allows clients to query the schema of a GraphQL API at runtime. It provides a way to dynamically discover the available types, fields, and relationships in the API, making it easier to build flexible and adaptable clients.
To perform introspection, clients can send a special GraphQL query called __schema
that retrieves the full schema definition. This query can be executed just like any other query against the API.
Here’s an example of an introspection query:
{ __schema { types { name fields { name type { name kind } } } } }
This query retrieves the name and kind of all types in the schema, as well as the name and type of all fields for each type. The result of the query provides a detailed description of the available types and fields in the API.
Introspection is particularly useful when building GraphQL clients, as it allows them to dynamically adapt to changes in the API without requiring manual updates. Clients can use the introspection query to generate code, validate queries, and provide autocompletion and type checking.
However, it’s important to note that introspection can also pose security risks if not properly controlled. Exposing sensitive information about the API through introspection can potentially be exploited by malicious actors. Therefore, it’s recommended to carefully configure introspection capabilities in production environments.
Related Article: Step by Step Process: Passing Enum in GraphQL Query
GraphQL Query
In GraphQL, a query is a read-only operation that allows clients to fetch data from a GraphQL API. It is the most common type of operation in GraphQL and is used to retrieve data in a structured and efficient manner.
A GraphQL query is a hierarchical structure that specifies the fields to be retrieved and their relationships. It is defined using the GraphQL query language, which provides a flexible and intuitive syntax for constructing queries.
Here’s an example of a GraphQL query that retrieves a list of posts with their titles and authors:
query { posts { title author { name } } }
This query starts with the keyword query
followed by an optional operation name (in this case, it is omitted). Inside the query, we specify the fields we want to retrieve. In this example, we want to retrieve the title
field of each post, as well as the name
field of the author of each post.
The result of a query is a JSON-like structure that matches the shape of the query. In this case, the result would be an array of objects, each containing a title
field and an author
field with a nested name
field.
Queries in GraphQL can also include arguments to filter, sort, or paginate the data. Arguments are specified after the field name and enclosed in parentheses. For example, we could modify the previous query to only retrieve the posts published after a certain date:
query { posts(publishedAfter: "2022-01-01") { title author { name } } }
This query includes the publishedAfter
argument with a value of "2022-01-01"
. The API would then filter the posts based on this argument before returning the result.
GraphQL Mutation
In GraphQL, a mutation is an operation that allows clients to modify data in a GraphQL API. It is used to create, update, or delete data, similar to how POST, PUT, and DELETE HTTP methods are used in RESTful APIs.
A GraphQL mutation is defined using the GraphQL query language, just like a query. However, mutations are distinguished from queries by using the keyword mutation
instead of query
.
Here’s an example of a GraphQL mutation that creates a new post:
mutation { createPost(input: { title: "New Post", text: "Lorem ipsum" }) { id title text } }
This mutation starts with the keyword mutation
followed by an optional operation name (in this case, it is omitted). Inside the mutation, we specify the operation to be performed. In this example, we want to create a new post with a title of “New Post” and text of “Lorem ipsum”.
The result of a mutation is a JSON-like structure that matches the shape of the mutation. In this case, the result would be an object with the id
, title
, and text
fields of the newly created post.
Mutations in GraphQL can also include arguments, just like queries. For example, we could modify the previous mutation to update an existing post:
mutation { updatePost(id: "123", input: { title: "Updated Post", text: "Dolor sit amet" }) { id title text } }
This mutation includes the id
argument to specify which post to update, and the input
argument with the new values for the post. The API would then update the post based on these arguments before returning the result.
GraphQL Subscription
In GraphQL, a subscription is a real-time operation that allows clients to receive updates from a GraphQL API as they happen. It is used to subscribe to changes in the data and receive a stream of events over a persistent connection.
A GraphQL subscription is defined using the GraphQL query language, similar to a query or mutation. However, subscriptions are distinguished from queries and mutations by using the keyword subscription
instead of query
or mutation
.
Here’s an example of a GraphQL subscription that listens for new comments on a post:
subscription { newComment(postId: "123") { id text createdAt } }
This subscription starts with the keyword subscription
followed by an optional operation name (in this case, it is omitted). Inside the subscription, we specify the event to subscribe to. In this example, we want to receive new comments on the post with the ID “123”.
The result of a subscription is a JSON-like structure that matches the shape of the subscription. In this case, the result would be an object with the id
, text
, and createdAt
fields of each new comment.
Subscriptions in GraphQL are typically implemented using WebSockets or other real-time communication protocols. The server maintains a persistent connection with the client and sends updates whenever the subscribed event occurs. This allows clients to receive real-time updates without the need for polling or long-polling.
Related Article: Achieving Production-Ready GraphQL
GraphQL Scalar Types
In GraphQL, scalar types are the basic building blocks for defining the shape of data. They represent atomic values that cannot be further decomposed, such as strings, integers, floats, booleans, and IDs.
GraphQL provides several built-in scalar types that cover common data types:
– String
: Represents textual data, such as names, descriptions, and content.
– Int
: Represents signed 32-bit integers.
– Float
: Represents signed double-precision floating-point values.
– Boolean
: Represents true or false values.
– ID
: Represents a unique identifier, often used to refetch an object or as a key for caching.
Scalar types in GraphQL are used to define the types of fields and arguments in the schema. For example, we might have a Post
object type with fields of scalar types:
type Post { id: ID! title: String! views: Int! isPublished: Boolean! createdAt: String! }
In this example, the Post
type has fields like id
of type ID
, title
of type String
, views
of type Int
, isPublished
of type Boolean
, and createdAt
of type String
. The exclamation mark (!
) denotes that the field is non-nullable, meaning it must always have a value.
Scalar types in GraphQL can also be customized or extended by defining custom scalar types. This allows developers to handle specialized data types or enforce specific validation rules. Custom scalar types are defined in the schema using the scalar
keyword.
GraphQL Directive
In GraphQL, a directive is a way to add additional instructions or metadata to a GraphQL document, such as a query, mutation, or schema definition. Directives allow developers to control the behavior of the execution engine and customize the response based on certain conditions or requirements.
GraphQL provides several built-in directives that can be used in a GraphQL document:
– @include(if: Boolean)
: Conditionally includes a field or fragment based on the value of the if
argument.
– @skip(if: Boolean)
: Conditionally skips a field or fragment based on the value of the if
argument.
– @deprecated(reason: String)
: Marks a field or enum value as deprecated with an optional deprecation reason.
Directives are applied to fields or fragments by adding them before the field or fragment definition using the @
symbol. For example, we can use the @include
directive to conditionally include a field in a query:
query { user { name email @include(if: $includeEmail) } }
In this example, the email
field is conditionally included based on the value of the variable $includeEmail
. If $includeEmail
is true
, the email
field will be included in the response. If $includeEmail
is false
, the email
field will be skipped.
Directives in GraphQL can also be customized or extended by defining custom directives. This allows developers to add domain-specific instructions or metadata to a GraphQL document. Custom directives are defined in the schema using the directive
keyword.
GraphQL Resolver
In GraphQL, a resolver is a function or method that is responsible for fetching the data for a field in a GraphQL schema. Resolvers are a key component of the GraphQL execution engine and play a crucial role in determining how data is retrieved and transformed.
Resolvers are defined for each field in the GraphQL schema and are executed in a specific order during the execution of a query or mutation. They are responsible for retrieving the data from the appropriate data source, such as a database or an API, and transforming it into the shape expected by the client.
Here’s an example of a resolver function for a posts
field in a GraphQL schema:
const resolvers = { Query: { posts: () => { // Retrieve and return the list of posts from the data source return fetchPosts(); } } };
In this example, the resolver function is defined for the posts
field in the Query
root type. When a query is executed that includes the posts
field, this resolver function will be called to fetch the data.
Resolvers can also accept arguments, which are passed from the query or mutation to the resolver function. For example, we could modify the previous resolver to include a limit
argument:
const resolvers = { Query: { posts: (_, { limit }) => { // Retrieve and return a limited number of posts from the data source return fetchPosts(limit); } } };
In this example, the limit
argument is passed from the query to the resolver function, allowing the client to specify the maximum number of posts to retrieve.
Resolvers in GraphQL can also handle nested fields and relationships between types. For example, a resolver for a field that represents a relationship between two types can fetch the related data and return it in the response.
Related Article: Implementing TypeORM with GraphQL and NestJS
GraphQL Client
In GraphQL, a client is a piece of software that consumes a GraphQL API and sends queries, mutations, and subscriptions to retrieve and modify data. GraphQL clients play a crucial role in building applications that interact with a GraphQL API and are responsible for managing the communication with the server.
GraphQL clients can be implemented in various programming languages and frameworks, and provide a range of features and functionalities to simplify the interaction with a GraphQL API. They typically provide tools for generating code from a GraphQL schema, validating queries, caching data, and handling real-time updates.
Here’s an example of a simple GraphQL client written in JavaScript using the apollo-client
library:
import { ApolloClient, InMemoryCache, gql } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://api.example.com/graphql', cache: new InMemoryCache() }); client.query({ query: gql` query { posts { title author { name } } } ` }).then(result => { console.log(result.data); }).catch(error => { console.error(error); });
In this example, we create a new instance of the ApolloClient
class with the URL of the GraphQL API and an in-memory cache. We then use the query
method to send a GraphQL query to retrieve a list of posts with their titles and authors. The result of the query is logged to the console.
GraphQL clients provide abstractions and utilities to simplify the process of sending queries, mutations, and subscriptions to a GraphQL API. They handle the underlying network communication, manage the state of the application, and provide tools for optimizing performance and reducing the amount of data transferred.
Purpose of Typename in GraphQL
The __typename
field in GraphQL serves the purpose of providing type information about objects returned in a query. It allows clients to determine the specific type of an object and perform appropriate actions based on its type.
The main purpose of __typename
is to enable polymorphic queries, where a field can return objects of different types. By including __typename
in the selection set of a query, clients can differentiate between the different types of objects and handle them accordingly.
For example, let’s say we have a GraphQL schema with two types: Post
and Comment
. Both types have a text
field, but the Post
type also has an additional title
field. If we query for both posts
and comments
, we can use __typename
to differentiate between the two types:
query { posts { __typename title text } comments { __typename text } }
The __typename
field will return either "Post"
or "Comment"
for each object, allowing the client to handle them differently based on their type. For example, the client could display the title
field for posts, but not for comments.
The purpose of __typename
goes beyond just differentiating between types in a query. It also enables the client to dynamically adapt to changes in the schema. By including __typename
in the selection set, the client can detect changes in the types returned by a field and adjust its behavior accordingly.
How Typename Works in GraphQL
In GraphQL, the __typename
field is automatically added to every GraphQL object type. It is available for selection in any query, mutation, or subscription and provides the name of the type of the object being returned.
The __typename
field works by introspecting the GraphQL schema at runtime. When a query is executed, the GraphQL execution engine analyzes the query and identifies the fields to be included in the response. If the __typename
field is included in the selection set, the execution engine retrieves the name of the type for each object and includes it in the response.
For example, if we have a GraphQL query that includes the __typename
field:
query { posts { __typename title text } }
The execution engine will retrieve the name of the type for each Post
object returned in the response and include it in the result. The result might look something like this:
{ "data": { "posts": [ { "__typename": "Post", "title": "Hello World", "text": "Lorem ipsum dolor sit amet" }, { "__typename": "Post", "title": "GraphQL 101", "text": "Lorem ipsum dolor sit amet" } ] } }
In this example, the __typename
field returns the value "Post"
for each Post
object in the response. This allows the client to determine the specific type of each object and handle them accordingly.
The __typename
field is also available for selection in nested fields and fragments. For example, we can include __typename
in a nested field to retrieve the type information for related objects:
query { posts { title author { __typename name } } }
In this case, the __typename
field will return the name of the type for each Author
object in the response.
Related Article: Exploring Solid GraphQL
When to Use Typename in GraphQL
The __typename
field in GraphQL should be used when there is a need to differentiate between different types of objects returned in a query. It is particularly useful when dealing with polymorphic types, where a field can return objects of different types.
Here are some scenarios when __typename
can be useful:
– Polymorphic queries: When a field can return objects of different types, including __typename
allows the client to handle each type differently. For example, a field that returns both Post
and Comment
objects can use __typename
to display different information for each type.
– Conditional rendering: When rendering UI components based on the type of an object, including __typename
allows the client to conditionally render different components based on the type. For example, a list of items where each item can have a different component based on its type.
– Dynamic behavior: When the behavior of the client needs to dynamically adapt to changes in the schema, including __typename
allows the client to detect changes in the types returned by a field and adjust its behavior accordingly.
It’s important to note that using __typename
in every query can add overhead to the response size, especially for large queries with many objects. Therefore, it’s recommended to use __typename
selectively when needed and consider the trade-off between flexibility and performance.
Changing Typename in a GraphQL Query
In GraphQL, the __typename
field is automatically added to every GraphQL object type and cannot be changed or customized. It provides the name of the type of the object being returned and is determined by the GraphQL schema.
The __typename
field is not meant to be modified by the client in a query. Its purpose is to provide type information about the objects returned in the response, allowing the client to determine the specific type of each object and handle them accordingly.
For example, let’s say we have a GraphQL query that includes the __typename
field:
query { posts { __typename title text } }
The execution engine will automatically populate the __typename
field with the name of the type for each Post
object returned in the response. The client cannot modify the value of __typename
in the query or change its behavior.
If the client needs to change the behavior based on the type of an object, it can use the value of __typename
in the response to determine the specific type and perform appropriate actions.
Available Scalar Types in GraphQL
GraphQL provides several built-in scalar types that cover common data types. These scalar types represent atomic values that cannot be further decomposed and are used to define the types of fields and arguments in the schema.
Here is a list of the available scalar types in GraphQL:
– String
: Represents textual data, such as names, descriptions, and content.
– Int
: Represents signed 32-bit integers.
– Float
: Represents signed double-precision floating-point values.
– Boolean
: Represents true or false values.
– ID
: Represents a unique identifier, often used to refetch an object or as a key for caching.
Scalar types in GraphQL are used to define the types of fields and arguments in the schema. For example, we might have a Post
object type with fields of scalar types:
type Post { id: ID! title: String! views: Int! isPublished: Boolean! createdAt: String! }
In this example, the Post
type has fields like id
of type ID
, title
of type String
, views
of type Int
, isPublished
of type Boolean
, and createdAt
of type String
. The exclamation mark (!
) denotes that the field is non-nullable, meaning it must always have a value.
Scalar types in GraphQL can also be customized or extended by defining custom scalar types. This allows developers to handle specialized data types or enforce specific validation rules. Custom scalar types are defined in the schema using the scalar
keyword.
Related Article: Implementing Upsert Operation in GraphQL
Defining a Custom Scalar Type in GraphQL
In GraphQL, custom scalar types can be defined to handle specialized data types or enforce specific validation rules. Custom scalar types allow developers to extend the built-in scalar types and define their own atomic values.
To define a custom scalar type in GraphQL, you need to provide a name for the type and specify how the values of the type should be serialized and parsed. This can be done in the schema using the scalar
keyword.
Here’s an example of a custom scalar type called Date
that represents a date value:
scalar Date
In this example, we define a custom scalar type called Date
. The name of the type is Date
, and it does not have any additional configuration.
Once the custom scalar type is defined, you can use it as the type of a field or argument in the schema. For example, we can define a Post
object type with a createdAt
field of type Date
:
type Post { id: ID! title: String! createdAt: Date! }
In this example, the createdAt
field of the Post
type is of type Date
, which is our custom scalar type.
To handle the serialization and parsing of values of the custom scalar type, you need to implement the logic in the resolver functions. The resolver functions are responsible for converting the values between their internal representation and the serialized representation.
For example, in JavaScript, you can define a resolver function for the Date
scalar type that converts the date value to a string:
const resolvers = { Date: { serialize: (value) => { // Convert the date value to a string return value.toString(); }, parseValue: (value) => { // Parse the string value to a date return new Date(value); }, parseLiteral: (ast) => { if (ast.kind === Kind.STRING) { // Parse the string literal to a date return new Date(ast.value); } return null; } } };
In this example, we define resolver functions for the Date
scalar type. The serialize
function converts the date value to a string, the parseValue
function parses a string value to a date, and the parseLiteral
function parses a string literal to a date.
Role of a Resolver in GraphQL
In GraphQL, a resolver is a function or method that is responsible for fetching the data for a field in a GraphQL schema. Resolvers are a key component of the GraphQL execution engine and play a crucial role in determining how data is retrieved and transformed.
The role of a resolver is to resolve the value of a field in the schema. When a query or mutation is executed, the GraphQL execution engine analyzes the query and identifies the fields to be included in the response. For each field, the execution engine invokes the corresponding resolver function to fetch the data.
Resolvers are defined for each field in the GraphQL schema and are executed in a specific order during the execution of a query or mutation. They are responsible for retrieving the data from the appropriate data source, such as a database or an API, and transforming it into the shape expected by the client.
Here’s an example of a resolver function for a posts
field in a GraphQL schema:
const resolvers = { Query: { posts: () => { // Retrieve and return the list of posts from the data source return fetchPosts(); } } };
In this example, the resolver function is defined for the posts
field in the Query
root type. When a query is executed that includes the posts
field, this resolver function will be called to fetch the data.
Resolvers can also accept arguments, which are passed from the query or mutation to the resolver function. For example, we could modify the previous resolver to include a limit
argument:
const resolvers = { Query: { posts: (_, { limit }) => { // Retrieve and return a limited number of posts from the data source return fetchPosts(limit); } } };
In this example, the limit
argument is passed from the query to the resolver function, allowing the client to specify the maximum number of posts to retrieve.
Resolvers in GraphQL can also handle nested fields and relationships between types. For example, a resolver for a field that represents a relationship between two types can fetch the related data and return it in the response.
The role of a resolver is to bridge the gap between the GraphQL schema and the data sources. It allows developers to define the logic for fetching and transforming the data, providing a flexible and efficient way to retrieve data in a GraphQL API.
Implementing a Resolver in GraphQL
In GraphQL, a resolver is a function or method that is responsible for fetching the data for a field in a GraphQL schema. Resolvers are a key component of the GraphQL execution engine and play a crucial role in determining how data is retrieved and transformed.
To implement a resolver in GraphQL, you need to define a resolver function for each field in the schema. The resolver function is responsible for fetching the data from the appropriate data source, such as a database or an API, and transforming it into the shape expected by the client.
Here’s an example of a resolver function for a posts
field in a GraphQL schema:
const resolvers = { Query: { posts: () => { // Retrieve and return the list of posts from the data source return fetchPosts(); } } };
In this example, the resolver function is defined for the posts
field in the Query
root type. When a query is executed that includes the posts
field, this resolver function will be called to fetch the data.
Resolvers can also accept arguments, which are passed from the query or mutation to the resolver function. For example, we could modify the previous resolver to include a limit
argument:
const resolvers = { Query: { posts: (_, { limit }) => { // Retrieve and return a limited number of posts from the data source return fetchPosts(limit); } } };
In this example, the limit
argument is passed from the query to the resolver function, allowing the client to specify the maximum number of posts to retrieve.
Resolvers in GraphQL can also handle nested fields and relationships between types. For example, a resolver for a field that represents a relationship between two types can fetch the related data and return it in the response.
To implement a resolver, you need to determine the appropriate data source for each field and write the logic to fetch and transform the data. This can involve making database queries, calling APIs, or performing any other necessary operations.
Resolvers provide a flexible and efficient way to retrieve data in a GraphQL API. They allow developers to define the logic for fetching and transforming the data, providing a seamless experience for clients interacting with the API.
Related Article: AEM GraphQL: A Critical Component in Modern Programming
Differences Between Query and Mutation in GraphQL
In GraphQL, both queries and mutations are operations that allow clients to interact with a GraphQL API. However, there are some key differences between queries and mutations in terms of their purpose and behavior.
Queries are read-only operations that allow clients to retrieve data from a GraphQL API. They are used to fetch data in a structured and efficient manner and are the most common type of operation in GraphQL.
Queries are defined using the GraphQL query language and are executed against the Query
root type in the schema. They specify the fields to be retrieved and their relationships, allowing the client to request exactly the data it needs.
Here’s an example of a GraphQL query that retrieves a list of posts with their titles and authors:
query { posts { title author { name } } }
In this example, the query starts with the keyword query
followed by an optional operation name. Inside the query, we specify the fields we want to retrieve. In this case, we want to retrieve the title
field of each post, as well as the name
field of the author of each post.
Mutations, on the other hand, are write operations that allow clients to modify data in a GraphQL API. They are used to create, update, or delete data, similar to how POST, PUT, and DELETE HTTP methods are used in RESTful APIs.
Mutations are also defined using the GraphQL query language, but they are executed against the Mutation
root type in the schema. They specify the operation to be performed, such as creating a new object or updating an existing object.
Here’s an example of a GraphQL mutation that creates a new post:
mutation { createPost(input: { title: "New Post", text: "Lorem ipsum" }) { id title text } }
In this example, the mutation starts with the keyword mutation
followed by an optional operation name. Inside the mutation, we specify the operation to be performed. In this case, we want to create a new post with a title of “New Post” and text of “Lorem ipsum”.
The main difference between queries and mutations is that queries are read-only operations, while mutations are write operations. Queries retrieve data from the API without modifying it, while mutations modify data in the API.
Another difference is that mutations can have side effects, such as updating a database or sending notifications, while queries are side-effect free and should not modify any data in the API.
Overall, queries and mutations in GraphQL provide a unified and flexible way to interact with a GraphQL API, allowing clients to retrieve and modify data in a consistent manner.
Purpose of a Directive in GraphQL
In GraphQL, a directive is a way to add additional instructions or metadata to a GraphQL document, such as a query, mutation, or schema definition. Directives allow developers to control the behavior of the execution engine and customize the response based on certain conditions or requirements.
The main purpose of a directive in GraphQL is to modify the execution behavior of a field or fragment. Directives can be used to conditionally include or skip a field, deprecate a field, provide hints for caching, or perform other custom logic.
Directives are defined in the schema using the directive
keyword and can be applied to fields or fragments in a GraphQL document. They are included in the document by adding them before the field or fragment definition using the @
symbol.
Here’s an example of a directive called deprecated
that marks a field as deprecated:
directive @deprecated(reason: String) on FIELD_DEFINITION type Query { posts: [Post] @deprecated(reason: "Use the `feed` field instead") }
In this example, the @deprecated
directive is defined with an argument called reason
. It can be applied to field definitions using the on FIELD_DEFINITION
directive location.
Directives can also be customized or extended by defining custom directives. This allows developers to add domain-specific instructions or metadata to a GraphQL document. Custom directives are defined in the schema using the directive
keyword.
The purpose of a directive in GraphQL is to provide a way to modify the behavior and response of a field or fragment based on certain conditions or requirements. Directives enable developers to customize the execution behavior of a GraphQL document and provide additional instructions or metadata to the execution engine.
Additional Resources
– GraphQL – A query language for APIs
– Defining a Schema – GraphQL