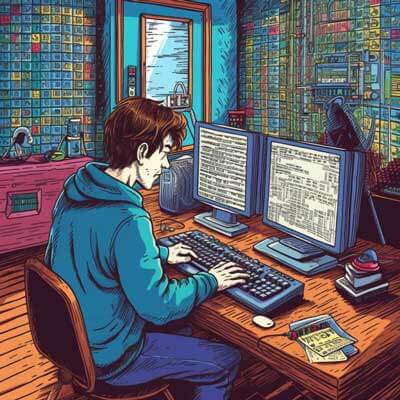
Schema Stitching
Schema stitching is a technique in GraphQL that allows you to combine multiple GraphQL schemas into a single, unified schema. This can be useful when you have multiple services or microservices, each with their own GraphQL schema, and you want to expose a single GraphQL API to your clients.
To demonstrate how schema stitching works, let’s consider a simple example where we have two GraphQL schemas, schemaA
and schemaB
, representing two services:
# schemaA type Query { hello: String } type Mutation { updateHello(newHello: String!): String }
# schemaB type Query { goodbye: String } type Mutation { updateGoodbye(newGoodbye: String!): String }
Now, let’s stitch these two schemas together into a single schema using the mergeSchemas
function from the graphql-tools
library:
const { mergeSchemas } = require('graphql-tools'); const schemaA = require('./schemaA'); const schemaB = require('./schemaB'); const mergedSchema = mergeSchemas({ schemas: [schemaA, schemaB], });
In this example, we import the schemaA
and schemaB
modules, which contain the respective GraphQL schemas. Then, we pass these schemas to the mergeSchemas
function, which returns a new schema that combines the types and fields from both schemas.
Now, clients can make queries and mutations against the merged schema as if it were a single schema:
query { hello goodbye } mutation { updateHello(newHello: "Hello, world!") updateGoodbye(newGoodbye: "Goodbye, world!") }
This will result in a single request being sent to the appropriate underlying services, and the responses will be combined into a single response.
Related Article: Managing Data Queries with GraphQL Count
Subscriptions in GraphQL
Subscriptions in GraphQL allow clients to receive real-time updates from the server. This is particularly useful for applications that require real-time data, such as chat applications or live dashboards.
To implement subscriptions in GraphQL, you need to define a special type called Subscription
in your schema. This type contains fields that represent the events that clients can subscribe to. Each field should have a resolver function that determines how the server should handle the subscription.
Here’s an example of a schema with a subscription field:
type Subscription { newMessage: Message } type Message { id: ID! content: String! }
In this example, the Subscription
type has a field called newMessage
, which represents a subscription for new messages. The newMessage
field returns an object of type Message
, which has an id
and content
.
To implement the subscription resolver, you can use a library like graphql-subscriptions
or subscriptions-transport-ws
. Here’s an example using graphql-subscriptions
:
const { PubSub } = require('graphql-subscriptions'); const pubsub = new PubSub(); const resolvers = { Subscription: { newMessage: { subscribe: () => pubsub.asyncIterator('NEW_MESSAGE'), }, }, }; // Publish a new message pubsub.publish('NEW_MESSAGE', { newMessage: { id: '1', content: 'Hello, world!' } });
In this example, we create an instance of PubSub
from graphql-subscriptions
, which provides the publish-subscribe functionality. Then, we define the resolver for the newMessage
field in the Subscription
type. The subscribe
function returns an AsyncIterator
that represents the stream of events for the subscription.
To send updates to the subscribed clients, we use the publish
method of PubSub
, passing the event name and the payload. This will trigger the execution of the subscription resolver and send the update to the clients.
Clients can subscribe to the newMessage
subscription using a WebSocket connection. When a new message is published, the server will send the update to the clients over the WebSocket connection.
Introspection in GraphQL
Introspection is a useful feature in GraphQL that allows clients to query the GraphQL schema itself. It provides a way for clients to discover the available types, fields, and directives in the schema, and to dynamically generate queries and mutations based on the schema.
To perform introspection in GraphQL, you can use the __schema
field, which is a predefined field that represents the schema itself. By querying this field, clients can retrieve information about the schema.
Here’s an example of a query that performs introspection:
query IntrospectionQuery { __schema { types { name kind } directives { name description args { name description type { name kind } } } } }
In this example, the query requests information about the types in the schema and their kinds (scalar, object, interface, etc.). It also requests information about the directives in the schema, including their names, descriptions, and arguments.
The server will respond with the introspection data, which can be used by clients to generate dynamic queries and mutations. For example, clients can dynamically generate input objects based on the arguments of a directive, or generate queries based on the available types in the schema.
Introspection is particularly useful in client libraries and development tools for auto-generating code, providing autocompletion, and validating queries against the schema.
Relay and its Relation to GraphQL
Relay is a useful framework for building data-driven applications with GraphQL. It was developed by Facebook and is widely used in large-scale GraphQL applications.
Relay provides a set of conventions and tools that help you structure your code and manage data fetching and caching. It introduces the concept of “connections” and “edges” to represent paginated data, and provides a client-side store for caching and managing data.
Relay is tightly integrated with GraphQL, and relies on certain features of the GraphQL specification, such as the use of fragments and the @relay
directive.
To use Relay, you need to define your schema following certain conventions, such as using the @relay
directive to annotate fields that represent connections, and using fragments to specify the data requirements of your components.
Here’s an example of a GraphQL schema that uses Relay conventions:
type Query { node(id: ID!): Node } type User implements Node { id: ID! name: String! } interface Node { id: ID! } type PageInfo { hasNextPage: Boolean! hasPreviousPage: Boolean! startCursor: String! endCursor: String! } type Connection { edges: [Edge!]! pageInfo: PageInfo! } type Edge { node: Node! cursor: String! }
In this example, we have a Query
type with a field called node
, which is used to fetch individual nodes by their ID. We also have a User
type that implements the Node
interface, and a Connection
type that represents a connection of nodes.
To fetch data with Relay, you need to define fragments that specify the data requirements of your components. Fragments are reusable pieces of GraphQL query that can be included in other queries. Here’s an example of a fragment:
fragment UserFragment on User { id name }
In this example, the UserFragment
fragment includes the id
and name
fields of the User
type.
Relay also provides a set of hooks and components for fetching and rendering data in your components. These include the RelayEnvironmentProvider
, useFragment
, and usePagination
hooks, and the Suspense
and Fragment
components.
Overall, Relay is a useful framework that provides a structured approach to building data-driven applications with GraphQL. It simplifies data fetching and caching, and promotes best practices for managing data in your application.
Related Article: Step by Step Process: Passing Enum in GraphQL Query
Resolvers in GraphQL
Resolvers are functions in GraphQL that determine how to fetch the data for a particular field in a GraphQL query. They are a crucial part of the GraphQL execution process, as they provide the data for the fields requested by the client.
Resolvers are defined for each field in the GraphQL schema and can be implemented in any programming language. They receive four arguments: parent
, args
, context
, and info
.
– parent
: The result of the previous resolver in the execution chain. For fields at the root level, this will be the root object itself.
– args
: The arguments passed to the field in the GraphQL query.
– context
: A context object that is shared across all resolvers in a particular execution.
– info
: Contains information about the field being resolved, such as its name and the path to it in the query.
Here’s an example of a resolver function in JavaScript:
const resolvers = { Query: { hello: (parent, args, context, info) => { return 'Hello, world!'; }, }, };
In this example, we define a resolver function for the hello
field in the Query
type. The resolver simply returns the string “Hello, world!”.
Resolvers can also be asynchronous if they need to fetch data from a remote service or a database. In JavaScript, you can use async/await
or return a Promise
to handle asynchronous operations.
const resolvers = { Query: { user: async (parent, args, context, info) => { const { id } = args; const user = await fetchUserById(id); return user; }, }, };
In this example, the resolver fetches a user by ID from a hypothetical fetchUserById
function, which returns a Promise
that resolves to the user object.
Resolvers can also resolve nested fields by accessing properties on the parent object. For example:
const resolvers = { User: { fullName: (parent, args, context, info) => { return `${parent.firstName} ${parent.lastName}`; }, }, };
In this example, the resolver for the fullName
field in the User
type concatenates the firstName
and lastName
properties of the parent object.
Resolvers can also perform complex data fetching operations, such as joining data from multiple sources or caching the results for efficient querying.
Overall, resolvers are a fundamental part of GraphQL and allow you to customize how data is fetched and transformed for each field in your schema. They provide flexibility and control over the data fetching process and enable you to integrate GraphQL with any backend system or data source.
Directives in GraphQL
Directives are a useful feature in GraphQL that allow you to add conditional logic and modify the execution of fields and fragments in a GraphQL query or mutation.
A directive is a special kind of field that is prefixed with the @
symbol and can be applied to any field or fragment in a GraphQL document. Directives can be used to control the visibility of fields, specify the shape of the response, provide default values for arguments, and more.
Here’s an example of a query that uses the @include
directive to conditionally include a field based on a variable:
query { user(id: "123") { name email @include(if: $includeEmail) } }
In this example, the @include
directive is applied to the email
field. The if
argument of the directive specifies a variable $includeEmail
, which controls whether the field should be included in the response. If the value of $includeEmail
is true
, the email
field will be included; otherwise, it will be omitted.
Directives can also be used to modify the execution of fields. For example, the @skip
directive can be used to conditionally skip a field:
query { user(id: "123") { name email @skip(if: $skipEmail) } }
In this example, the @skip
directive is applied to the email
field. The if
argument of the directive specifies a variable $skipEmail
, which controls whether the field should be skipped. If the value of $skipEmail
is true
, the email
field will be skipped; otherwise, it will be executed as usual.
Directives can also be defined in the GraphQL schema to provide additional functionality or behavior. For example, the @deprecated
directive can be used to mark a field or enum value as deprecated:
type User { id: ID! name: String! email: String @deprecated(reason: "Use `contactInfo` instead") contactInfo: String! }
In this example, the @deprecated
directive is applied to the email
field. The reason
argument of the directive specifies the reason for deprecating the field. When clients query the email
field, they will receive a deprecation warning along with the specified reason.
Directives can also be used to create custom functionality in your GraphQL schema. You can define your own directives and implement the custom behavior in the resolver functions of the directive. This allows you to extend the capabilities of GraphQL and add domain-specific functionality to your API.
Overall, directives are a useful feature in GraphQL that allow you to add conditional logic and modify the execution of fields and fragments. They provide flexibility and control over the shape and behavior of your GraphQL API.
Mutations in GraphQL
Mutations in GraphQL allow clients to modify data on the server. They are a fundamental part of the GraphQL specification and provide a consistent and flexible way to perform write operations.
To define a mutation in GraphQL, you need to add a Mutation
type to your schema and define fields that represent the mutations. Each mutation field should have an input type, which represents the input arguments for the mutation, and a return type, which represents the result of the mutation.
Here’s an example of a schema with a mutation field:
type Mutation { createUser(input: CreateUserInput!): CreateUserPayload } input CreateUserInput { name: String! email: String! } type CreateUserPayload { user: User }
In this example, we have a Mutation
type with a field called createUser
. The createUser
field takes an input argument of type CreateUserInput
, which contains the name
and email
of the user to be created. The createUser
field returns a payload of type CreateUserPayload
, which contains the created user.
To execute a mutation, clients can use the mutation
keyword in their GraphQL query:
mutation { createUser(input: { name: "John Doe", email: "john@example.com" }) { user { id name email } } }
In this example, the mutation creates a new user with the name “John Doe” and the email “john@example.com”. The result of the mutation includes the id
, name
, and email
of the created user.
Mutations can also have side effects and return errors. For example, if a user with the same email already exists, the mutation could return an error indicating the conflict:
mutation { createUser(input: { name: "John Doe", email: "john@example.com" }) { user { id name email } error { message } } }
In this example, the createUser
mutation returns an error
field in addition to the user
field. If an error occurs during the mutation, the error
field will be populated with the error message.
Mutations can also be asynchronous and perform complex operations, such as updating multiple resources or sending notifications. They can interact with databases, external services, or any other backend system.
Overall, mutations in GraphQL provide a flexible and consistent way to perform write operations on the server. They allow clients to modify data with fine-grained control and receive detailed feedback about the outcome of the mutation.
Related Article: Achieving Production-Ready GraphQL
Pagination in GraphQL
Pagination is a common requirement in web applications, where data needs to be fetched in chunks or pages to improve performance and reduce network load. GraphQL provides built-in support for pagination through the use of connections, edges, and cursors.
To implement pagination in GraphQL, you need to define a connection type and an edge type in your schema. The connection type represents a paginated list of nodes, and the edge type represents a single node in the connection, along with a cursor that identifies its position in the list.
Here’s an example of a schema with pagination types:
type Query { users(first: Int, after: String): UserConnection! } type UserConnection { edges: [UserEdge!]! pageInfo: PageInfo! } type UserEdge { node: User! cursor: String! } type User { id: ID! name: String! }
In this example, we have a Query
type with a field called users
. The users
field takes two arguments: first
, which specifies the number of nodes to fetch per page, and after
, which specifies the cursor that identifies the starting point of the next page.
The users
field returns a UserConnection
type, which has an edges
field that contains a list of UserEdge
types. Each UserEdge
type represents a user node in the connection, along with its cursor.
To fetch the first page of users, clients can make a query like this:
query { users(first: 10) { edges { node { id name } cursor } pageInfo { hasNextPage hasPreviousPage startCursor endCursor } } }
In this example, the users
field is called with the first
argument set to 10, indicating that the client wants to fetch the first 10 users. The result includes the edges
field, which contains the list of user nodes, and the pageInfo
field, which provides information about the pagination state.
To fetch the next page of users, clients can make a query like this:
query { users(first: 10, after: "eyJpZCI6MX0=") { edges { node { id name } cursor } pageInfo { hasNextPage hasPreviousPage startCursor endCursor } } }
In this example, the users
field is called with the first
argument set to 10 and the after
argument set to the cursor of the last node in the previous page. This will fetch the next 10 users after the specified cursor.
Pagination in GraphQL can also support other features, such as backward pagination, sorting, filtering, and more. It provides a flexible and standardized way to fetch data in chunks and navigate through large result sets.
Caching in GraphQL
Caching is an essential technique for improving the performance of web applications by reducing the amount of redundant data fetching and processing. GraphQL provides built-in support for caching through the use of a client-side cache.
The client-side cache in GraphQL stores the results of previous queries and mutations, allowing subsequent queries to be served from the cache instead of making a network request. This can significantly improve the performance of your application, especially for repeated or similar queries.
To enable caching in GraphQL, you need to use a GraphQL client library that supports caching, such as Apollo Client or Relay. These libraries provide a cache implementation that automatically stores and retrieves data from the cache.
When a query is executed, the client library checks the cache for a matching result. If the result is found, it is returned immediately without making a network request. If the result is not found, the query is sent to the server, and the response is stored in the cache for future use.
Here’s an example of caching in Apollo Client:
import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://api.example.com/graphql', cache: new InMemoryCache(), });
In this example, we create a new instance of Apollo Client with a configured cache. The InMemoryCache
class provided by Apollo Client is a simple and efficient cache implementation that stores data in memory.
Once the client is configured, you can execute queries as usual. Apollo Client will automatically check the cache for matching results and retrieve data from the cache when possible.
Caching in GraphQL can be further optimized by using cache normalization techniques. Cache normalization involves storing data in a normalized form, where each entity is stored only once and referenced by other entities using unique identifiers. This reduces redundancy and improves cache efficiency.
To normalize the cache, you need to define a schema for your data and configure your cache to normalize the data based on the schema. This can be done using the typePolicies
option of the cache configuration.
Here’s an example of cache normalization in Apollo Client:
import { ApolloClient, InMemoryCache } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://api.example.com/graphql', cache: new InMemoryCache({ typePolicies: { User: { keyFields: ['id'], }, }, }), });
In this example, we configure the cache to normalize data of type User
based on their id
field. This ensures that user data is stored only once in the cache, and other entities that reference users can use the id
to retrieve the user data.
Caching in GraphQL is a useful technique for improving the performance and responsiveness of your application. It reduces redundant data fetching and processing, and allows you to serve data from the cache instead of making network requests.
Performance Improvement in GraphQL
Performance is a critical aspect of any web application. With its flexible and efficient nature, GraphQL provides several techniques to improve performance and optimize the execution of queries and mutations.
Here are some performance improvement techniques in GraphQL:
Related Article: Implementing TypeORM with GraphQL and NestJS
Batching
Batching is a technique in GraphQL that allows you to combine multiple queries or mutations into a single network request. This reduces the number of round trips between the client and the server, improving performance.
To implement batching, you can use a GraphQL client library that supports batching, such as Apollo Client or Relay. These libraries automatically batch multiple queries or mutations into a single request, reducing network overhead.
Here’s an example of batching in Apollo Client:
import { ApolloClient, InMemoryCache } from '@apollo/client'; import { BatchHttpLink } from '@apollo/client/link/batch-http'; const client = new ApolloClient({ link: new BatchHttpLink({ uri: 'https://api.example.com/graphql' }), cache: new InMemoryCache(), });
In this example, we use the BatchHttpLink
class provided by Apollo Client to batch multiple queries or mutations into a single HTTP request.
Batching is particularly useful for scenarios where you need to fetch data from multiple sources or execute complex mutations that depend on each other. It reduces network latency and improves overall performance.
Data Loader
Data Loader is a utility library for batching and caching data fetching in GraphQL. It allows you to efficiently load data from a data source, such as a database or an API, by batching multiple requests and caching the results.
To use Data Loader, you need to define a loader function for each data source in your application. The loader function is responsible for fetching data from the data source and returning the result.
Here’s an example of using Data Loader with a hypothetical database:
const { DataLoader } = require('dataloader'); const { fetchUsersByIds } = require('./database'); const userLoader = new DataLoader(async (ids) => { const users = await fetchUsersByIds(ids); return ids.map((id) => users.find((user) => user.id === id)); }); const resolvers = { Query: { user: (parent, args, context, info) => { return userLoader.load(args.id); }, }, };
In this example, we create a DataLoader
instance with a loader function that fetches users by their IDs from a hypothetical fetchUsersByIds
function. The loader function takes an array of IDs and returns an array of users in the same order.
The user
resolver uses the load
method of the userLoader
to fetch a user by its ID. The loader takes care of batching and caching the requests, ensuring that each user is fetched only once.
Data Loader is particularly useful when you have complex data fetching requirements or need to optimize data loading from slow or unreliable data sources. It reduces the number of requests and improves the overall performance of your application.
Defer and Stream
Defer and Stream are two directives in GraphQL that allow you to control the timing and streaming of response data. They are useful for optimizing the rendering and performance of real-time or large result sets.
The @defer
directive allows you to defer the processing and rendering of a field until later in the response. This can be useful for fields that are not immediately needed or have a high computational cost.
The @stream
directive allows you to progressively send response data to the client as it becomes available. This is useful for streaming large result sets or real-time data, allowing the client to start processing the data as it arrives.
Here’s an example of using the @defer
directive:
query { user(id: "123") { name email @defer posts { title } } }
In this example, the email
field is deferred, meaning that it will be processed and sent to the client separately from the other fields. This allows the client to render the name
and posts
fields first, without waiting for the email
field.
Here’s an example of using the @stream
directive:
query { streamPosts { id title content comments @stream } }
In this example, the comments
field is streamed, meaning that the server will send the comments to the client as they become available. This allows the client to start rendering the posts and the comments as they arrive, improving the perceived performance of the application.
Defer and Stream are useful techniques for optimizing the rendering and performance of real-time or large result sets in GraphQL. They allow you to control the timing and streaming of response data, improving the user experience and reducing network load.
Related Article: Implementing Upsert Operation in GraphQL
Additional Resources
– GraphQL Schema
– GraphQL Resolvers
– GraphQL Mutations vs Queries