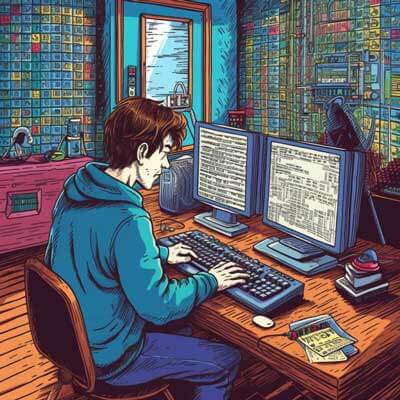
- GraphQL Input Types and Their Functionality
- Understanding GraphQL Input Objects
- Exploring GraphQL Input Fields
- Comparing GraphQL Input Interface and Type
- Example of Using GraphQL Input Interface
- Implementing GraphQL Input Interface
- Differences Between GraphQL Input Interface and Input Object
- Differences Between GraphQL Input Interface and Input Type
- Passing Arguments to a GraphQL Input Interface
- Using GraphQL Input Interface in a Mutation
- Additional Resources
GraphQL Input Types and Their Functionality
GraphQL is a useful query language for APIs that allows clients to request specific data structures and minimize over-fetching or under-fetching of data. In GraphQL, input types are used to define the shape and structure of the data that clients can send as arguments to queries and mutations.
Input types in GraphQL serve a similar purpose as regular types, but they are specifically designed to represent input values. They are defined using the input
keyword in the GraphQL schema and can contain fields with scalar or complex types.
Input types are useful when you want to pass a complex set of arguments to a field or mutation. For example, consider a scenario where you need to create a new user in a GraphQL API. Instead of passing individual arguments like name
, email
, and password
, you can define an input type called UserInput
that encapsulates all these fields. This makes the API more organized and allows for better reusability.
Let’s take a look at an example of defining an input type in GraphQL schema:
input UserInput { name: String! email: String! password: String! }
In the above example, we have defined an input type called UserInput
with three fields: name
, email
, and password
. The exclamation mark after the type indicates that the field is non-null, meaning it must always have a value.
Related Article: Managing Data Queries with GraphQL Count
Understanding GraphQL Input Objects
In GraphQL, an input object is a special type of object that represents a set of input values. It is similar to an input type but allows you to define more complex objects with nested fields.
Input objects are useful when you need to pass a structured set of input values to a field or mutation. For example, consider a scenario where you want to create a new blog post and associate it with multiple tags. Instead of passing an array of tag names as individual arguments, you can define an input object called BlogPostInput
that contains a title
field and a tags
field, where tags
is an array of tag names.
Let’s take a look at an example of defining an input object in GraphQL schema:
input BlogPostInput { title: String! tags: [String!]! }
In the above example, we have defined an input object called BlogPostInput
with two fields: title
and tags
. The tags
field is an array of non-null strings, indicated by the square brackets and exclamation mark.
Input objects can also be nested within other input objects to represent more complex data structures. This allows you to create hierarchical input structures that mirror the structure of the data being passed.
Exploring GraphQL Input Fields
In GraphQL, input fields are used to define the structure and types of the individual fields within an input type or input object.
Input fields can have scalar types like String
, Int
, or Boolean
, as well as complex types like other input types or input objects. They can also have validation rules and default values.
Let’s take a look at an example of defining input fields in GraphQL schema:
input UserInput { name: String! email: String! age: Int isActive: Boolean! }
In the above example, we have defined four input fields within the UserInput
input type: name
, email
, age
, and isActive
. The name
and email
fields are non-null strings, meaning they must always have a value. The age
field is an optional integer, indicated by the absence of the exclamation mark. The isActive
field is a non-null boolean.
Input fields can also have default values, which are used when the field is not provided by the client. This can be useful when you want to provide a default value for an optional field or when you want to enforce a specific value if the field is not explicitly provided.
Comparing GraphQL Input Interface and Type
In GraphQL, both input types and input objects are used to define the structure of input values. While they serve similar purposes, there are some differences between them.
Input types are used to define simple input values with scalar or complex types. They are defined using the input
keyword in the GraphQL schema and can contain fields with validation rules.
Input objects, on the other hand, are used to define more complex input values with nested fields. They allow you to create hierarchical input structures that mirror the structure of the data being passed.
The main difference between input types and input objects is their usage. Input types are typically used when you want to pass a set of input values to a field or mutation, while input objects are used when you need to pass a structured set of input values with nested fields.
Another difference is the way they are defined in the schema. Input types are defined using the input
keyword, followed by the type name and a set of fields. Input objects, on the other hand, are defined using the regular object type syntax, but with the input
keyword instead of the type
keyword.
Here’s an example to illustrate the difference:
input UserInput { name: String! email: String! age: Int } input BlogPostInput { title: String! tags: [String!]! } type Mutation { createUser(input: UserInput!): User createBlogPost(input: BlogPostInput!): BlogPost }
In the above example, we have defined two input types: UserInput
and BlogPostInput
. The UserInput
input type represents the input values for creating a user, while the BlogPostInput
input type represents the input values for creating a blog post.
In the Mutation
type, we have two mutations: createUser
and createBlogPost
. Both mutations accept an input argument, which corresponds to the respective input type.
Overall, input types and input objects provide flexibility and control over the shape and structure of input values in GraphQL. Depending on the complexity of the input data, you can choose between using input types or input objects to define the input values for your fields and mutations.
Related Article: Step by Step Process: Passing Enum in GraphQL Query
Example of Using GraphQL Input Interface
The GraphQL input interface is a useful feature that allows you to define reusable input types and input objects. It provides a way to define a common set of fields and validation rules that can be used across multiple fields or mutations.
Let’s take a look at an example of using the GraphQL input interface to define a common set of input fields for creating users and blog posts:
input CommonInput { createdBy: String! createdAt: String! } input UserInput implements CommonInput { name: String! email: String! } input BlogPostInput implements CommonInput { title: String! content: String! }
In the above example, we have defined an input interface called CommonInput
with two common fields: createdBy
and createdAt
. The UserInput
and BlogPostInput
input types implement this interface, which means they inherit the common fields.
Here’s an example of using the input types in a mutation:
type Mutation { createUser(input: UserInput!): User createBlogPost(input: BlogPostInput!): BlogPost }
In the above example, we have two mutations: createUser
and createBlogPost
. Both mutations accept an input argument, which corresponds to the respective input type. Since both input types implement the CommonInput
interface, the createdBy
and createdAt
fields are automatically included in the mutation arguments.
Implementing GraphQL Input Interface
Implementing the GraphQL input interface involves defining an input interface in the GraphQL schema and having input types or input objects implement that interface.
Let’s take a look at an example of implementing the GraphQL input interface:
input CommonInput { createdBy: String! createdAt: String! } input UserInput implements CommonInput { name: String! email: String! } input BlogPostInput implements CommonInput { title: String! content: String! }
In the above example, we have defined an input interface called CommonInput
with two common fields: createdBy
and createdAt
. The UserInput
and BlogPostInput
input types implement this interface by using the implements
keyword followed by the interface name.
To use the input types in mutations or fields, you can simply reference them as arguments with the appropriate input type:
type Mutation { createUser(input: UserInput!): User createBlogPost(input: BlogPostInput!): BlogPost }
In the above example, we have two mutations: createUser
and createBlogPost
. Both mutations accept an input argument, which corresponds to the respective input type. Since both input types implement the CommonInput
interface, the createdBy
and createdAt
fields are automatically included in the mutation arguments.
Differences Between GraphQL Input Interface and Input Object
GraphQL provides two ways to define input values: input interfaces and input objects. While they serve similar purposes, there are some differences between them.
An input interface is a contract that defines a common set of fields that can be implemented by input types. It allows you to define a reusable set of fields and validation rules that can be shared across multiple input types.
On the other hand, an input object is a specific type that represents a set of input values. It allows you to define more complex objects with nested fields.
The main difference between the input interface and input object is their usage. Input interfaces are typically used when you want to define a common set of fields that can be shared across multiple input types. This promotes code reuse and consistency.
Input objects, on the other hand, are used when you need to define a specific set of input values with nested fields. They allow you to create hierarchical input structures that mirror the structure of the data being passed.
Another difference is the way they are defined in the GraphQL schema. Input interfaces are defined using the interface
keyword, followed by the interface name and a set of fields. Input objects, on the other hand, are defined using the input
keyword, followed by the type name and a set of fields.
Here’s an example to illustrate the difference:
interface CommonInput { createdBy: String! createdAt: String! } input UserInput implements CommonInput { name: String! email: String! } input BlogPostInput { title: String! content: String! }
In the above example, we have defined an input interface called CommonInput
with two common fields: createdBy
and createdAt
. The UserInput
input type implements this interface, which means it inherits the common fields. The BlogPostInput
input type, on the other hand, does not implement any interface.
Related Article: Achieving Production-Ready GraphQL
Differences Between GraphQL Input Interface and Input Type
In GraphQL, input interfaces and input types are similar in function, but there are some differences between them.
An input interface is a contract that defines a common set of fields that can be implemented by input types. It allows you to define a reusable set of fields and validation rules that can be shared across multiple input types.
On the other hand, an input type is a specific type that represents a set of input values. It is typically used to define simple input values with scalar or complex types.
The main difference between the input interface and input type is their usage. Input interfaces are typically used when you want to define a common set of fields that can be shared across multiple input types. This promotes code reuse and consistency.
Input types, on the other hand, are used when you need to define a specific set of input values. They can contain fields with scalar or complex types and can have validation rules and default values.
Another difference is the way they are defined in the GraphQL schema. Input interfaces are defined using the interface
keyword, followed by the interface name and a set of fields. Input types, on the other hand, are defined using the input
keyword, followed by the type name and a set of fields.
Here’s an example to illustrate the difference:
interface CommonInput { createdBy: String! createdAt: String! } input UserInput implements CommonInput { name: String! email: String! } input BlogPostInput { title: String! content: String! }
In the above example, we have defined an input interface called CommonInput
with two common fields: createdBy
and createdAt
. The UserInput
input type implements this interface, which means it inherits the common fields. The BlogPostInput
input type, on the other hand, does not implement any interface.
Passing Arguments to a GraphQL Input Interface
In GraphQL, passing arguments to a GraphQL input interface is similar to passing arguments to input types or input objects. The main difference is that the input interface itself cannot be used as an argument. Instead, you need to use an input type or input object that implements the input interface.
Let’s take a look at an example of passing arguments to a GraphQL input interface:
interface CommonInput { createdBy: String! createdAt: String! } input UserInput implements CommonInput { name: String! email: String! } type Mutation { createUser(input: UserInput!): User }
In the above example, we have defined an input interface called CommonInput
with two common fields: createdBy
and createdAt
. The UserInput
input type implements this interface, which means it inherits the common fields.
To pass arguments to the createUser
mutation, we need to use the UserInput
input type, which implements the CommonInput
interface. Here’s an example of passing arguments to the createUser
mutation:
mutation { createUser(input: { name: "John Doe" email: "john.doe@example.com" createdBy: "admin" createdAt: "2022-01-01" }) { id name email } }
In the above example, we are passing the input
argument to the createUser
mutation. The input
argument is an object that contains the name
, email
, createdBy
, and createdAt
fields. The createdBy
and createdAt
fields are inherited from the CommonInput
interface.
Using GraphQL Input Interface in a Mutation
In GraphQL, you can use a GraphQL input interface in a mutation by defining an input type or input object that implements the input interface. This allows you to define a common set of fields that can be shared across multiple mutations.
Let’s take a look at an example of using a GraphQL input interface in a mutation:
interface CommonInput { createdBy: String! createdAt: String! } input UserInput implements CommonInput { name: String! email: String! } type Mutation { createUser(input: UserInput!): User }
In the above example, we have defined an input interface called CommonInput
with two common fields: createdBy
and createdAt
. The UserInput
input type implements this interface, which means it inherits the common fields.
To use the UserInput
input type in the createUser
mutation, we simply pass it as an argument with the appropriate input type. Here’s an example of using the UserInput
input type in the createUser
mutation:
mutation { createUser(input: { name: "John Doe" email: "john.doe@example.com" createdBy: "admin" createdAt: "2022-01-01" }) { id name email } }
In the above example, we are using the UserInput
input type as the argument to the createUser
mutation. The input
argument is an object that contains the name
, email
, createdBy
, and createdAt
fields. The createdBy
and createdAt
fields are inherited from the CommonInput
interface.
Related Article: Implementing TypeORM with GraphQL and NestJS
Additional Resources
– GraphQL Schema and Types
– GraphQL Mutations
– Understanding Resolvers in GraphQL