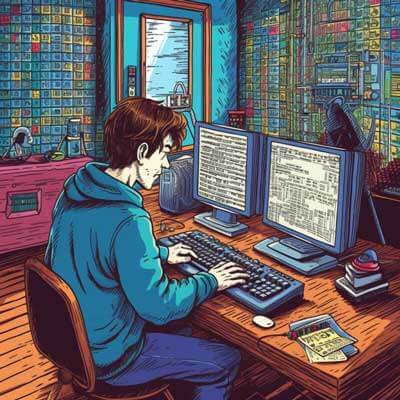
Table of Contents
Schema Stitching in GraphQL
Schema stitching is a useful technique in GraphQL that allows you to combine multiple GraphQL schemas into a single unified schema. This can be useful when working with microservices or modularizing your GraphQL implementation. With schema stitching, you can create a cohesive GraphQL API without having to expose the underlying services or databases.
To illustrate schema stitching, let's consider an example where we have two separate schemas: one for a user service and another for a product service. We want to combine these schemas into a single schema that exposes both user and product data.
First, we define the individual schemas for the user and product services:
# User schema type User { id: ID! name: String! email: String! } type Query { user(id: ID!): User } # Product schema type Product { id: ID! name: String! price: Float! } type Query { product(id: ID!): Product }
Next, we use the schema stitching library to merge these schemas into a single schema:
import { stitchSchemas } from 'graphql-tools'; import { makeRemoteExecutableSchema, introspectSchema } from 'graphql-tools'; import { HttpLink } from 'apollo-link-http'; import fetch from 'node-fetch'; const userSchema = makeRemoteExecutableSchema({ schema: await introspectSchema(fetch('http://user-service/graphql')), link: new HttpLink({ uri: 'http://user-service/graphql', fetch }), }); const productSchema = makeRemoteExecutableSchema({ schema: await introspectSchema(fetch('http://product-service/graphql')), link: new HttpLink({ uri: 'http://product-service/graphql', fetch }), }); const mergedSchema = stitchSchemas({ subschemas: [ { schema: userSchema }, { schema: productSchema }, ], });
Now, we have a single schema that combines both user and product data. We can query this schema as if it were a single GraphQL API:
query { user(id: "123") { id name email } product(id: "456") { id name price } }
Related Article: Managing Data Queries with GraphQL Count
How Resolvers Work in GraphQL
Resolvers are the core components of a GraphQL API that determine how the data is retrieved and returned in response to a GraphQL query. Resolvers are responsible for mapping GraphQL queries to the corresponding data sources and returning the requested data in the expected format.
In GraphQL, each field in a schema corresponds to a resolver function. When a query is executed, the resolver function for each field is called to retrieve the data. Resolvers can be implemented in various programming languages, such as JavaScript, Python, or Java, depending on the backend implementation of the GraphQL API.
Let's take a look at an example of how resolvers work in a JavaScript-based GraphQL API. Suppose we have a schema with a "user" field that returns user data:
type User { id: ID! name: String! email: String! } type Query { user(id: ID!): User }
To implement the resolver for the "user" field, we define a resolver function that takes the arguments provided in the query (in this case, the "id" argument) and returns the corresponding user data:
const resolvers = { Query: { user: (parent, { id }, context) => { // Logic to fetch user data from the database or another data source const userData = getUserById(id); return userData; }, }, };
In this example, the resolver function for the "user" field takes three arguments: "parent", "id", and "context". The "parent" argument represents the result of the parent resolver, if any. The "id" argument is the value passed in the query. The "context" argument provides access to shared data or services that can be used within the resolver.
Inside the resolver function, we can implement the logic to fetch the user data from a database or another data source. In this case, we call the "getUserById" function to retrieve the user data based on the provided ID.
Once the resolver function returns the user data, it will be automatically mapped to the corresponding fields in the GraphQL response. The GraphQL engine takes care of resolving nested fields and merging the data into the final response.
Subscriptions in GraphQL
Subscriptions are a useful feature in GraphQL that allows clients to receive real-time updates from the server. While queries and mutations are request-response operations, subscriptions enable a persistent connection between the client and the server, enabling real-time data streaming.
To implement subscriptions in GraphQL, you need to define the subscription type in your schema and implement the corresponding resolver functions. The subscription type includes fields that define the events or data streams that clients can subscribe to.
Let's consider an example where we want to implement real-time notifications for new messages in a chat application. We start by defining the subscription type in the schema:
type Subscription { newMessage: Message } type Message { id: ID! content: String! sender: User! timestamp: String! }
In this example, we have a "newMessage" field in the subscription type, which represents the event that clients can subscribe to receive new messages.
Next, we implement the resolver for the "newMessage" field. The resolver function needs to return an async iterator that emits the new messages as they occur:
const resolvers = { Subscription: { newMessage: { subscribe: async (parent, args, { pubsub }) => { return pubsub.asyncIterator('NEW_MESSAGE'); }, }, }, };
In this example, we assume that we have a "pubsub" instance that provides the functionality to publish and subscribe to events. The "subscribe" method of the "pubsub" instance returns an async iterator that emits new messages whenever they occur. The argument passed to the "asyncIterator" method ('NEW_MESSAGE') represents the channel or topic to subscribe to.
To send a new message, we use the "publish" method of the "pubsub" instance:
pubsub.publish('NEW_MESSAGE', { newMessage: newMessageData });
When a client subscribes to the "newMessage" subscription, they will receive the new messages as they are published. The client can use a WebSocket connection to maintain the persistent connection and handle the real-time updates.
Subscriptions in GraphQL provide a useful mechanism for building real-time applications, such as chat applications, live dashboards, or collaborative editing tools.
Implementing Authentication in a GraphQL API
Authentication is a critical aspect of building secure GraphQL APIs. It ensures that only authorized users can access certain resources or perform specific actions. There are various authentication mechanisms that can be implemented in a GraphQL API, such as token-based authentication, session-based authentication, or OAuth.
Let's take a look at an example of implementing token-based authentication in a GraphQL API. In this example, we use JSON Web Tokens (JWT) as the authentication mechanism.
First, we define a mutation in the schema to handle the authentication process:
type Mutation { login(email: String!, password: String!): AuthPayload } type AuthPayload { token: String user: User }
In this example, the "login" mutation takes an email and password as arguments and returns an "AuthPayload" object, which includes a token and the user information.
Next, we implement the resolver for the "login" mutation. The resolver function validates the user credentials and generates a JWT token:
const resolvers = { Mutation: { login: (parent, { email, password }, { secret }) => { const user = getUserByEmail(email); if (!user || user.password !== password) { throw new Error('Invalid email or password'); } const token = jwt.sign({ userId: user.id }, secret); return { token, user, }; }, }, };
In this example, the resolver function validates the user credentials by checking if the provided email and password match a user in the database. If the credentials are valid, the resolver generates a JWT token using the "jwt.sign" method, which signs a payload (in this case, the user ID) with a secret key.
Once the resolver returns the "AuthPayload" object with the token and user information, the client can use the token to authenticate subsequent requests by including it in the request headers.
To protect certain parts of the API that require authentication, you can use middleware or middleware-like functions to verify the token and extract the authenticated user from the request context.
Implementing authentication in a GraphQL API is crucial for ensuring the security and integrity of your application.
Related Article: Exploring Directus GraphQL
The Role of Authorization in GraphQL
Authorization is an important aspect of building secure GraphQL APIs. While authentication verifies the identity of a user, authorization determines what actions a user is allowed to perform and what resources they can access.
There are various authorization mechanisms that can be implemented in a GraphQL API, such as role-based access control (RBAC), attribute-based access control (ABAC), or custom authorization logic.
Let's consider an example where we want to implement authorization based on user roles in a GraphQL API. In this example, we assume that the user object has a "role" field that represents the user's role.
First, we define a custom directive in the schema to handle the authorization logic:
directive @hasRole(role: String!) on FIELD_DEFINITION type Query { user(id: ID!): User @hasRole(role: "admin") } type User { id: ID! name: String! email: String! role: String! }
In this example, we define the "@hasRole" directive that can be applied to fields in the schema. The directive takes a "role" argument, which represents the required role for accessing the field.
Next, we implement the directive resolver that performs the authorization logic:
const resolvers = { Query: { user: (parent, { id }, context) => { const user = getUserById(id); // Check if the user has the required role if (user.role !== context.currentUser.role) { throw new Error('Unauthorized'); } return user; }, }, };
In this example, the resolver for the "user" field checks if the user has the required role to access the field. The required role is obtained from the directive argument, and the user's role is obtained from the context object.
If the user does not have the required role, the resolver throws an error indicating that the user is unauthorized to access the field.
Caching in GraphQL APIs
Caching is an essential technique for improving the performance and scalability of GraphQL APIs. Caching can help reduce the number of requests made to underlying data sources and improve the response time for frequently accessed data.
In GraphQL, caching can be implemented at various levels, such as query-level caching, field-level caching, or result-level caching. Depending on your requirements and the caching strategy, you can choose the appropriate level of caching for your API.
Let's consider an example where we want to implement field-level caching in a GraphQL API. In this example, we assume that we have a "product" field that retrieves product data from a remote service.
type Product { id: ID! name: String! price: Float! } type Query { product(id: ID!): Product }
To implement field-level caching, we can use a caching library or module, such as Apollo Server's built-in caching or a third-party caching solution like Redis.
First, we define a resolver for the "product" field that retrieves the product data and caches the result:
const resolvers = { Query: { product: async (parent, { id }, { cache }) => { const cacheKey = `product:${id}`; const cachedProduct = await cache.get(cacheKey); if (cachedProduct) { return cachedProduct; } const product = await fetchProductFromRemoteService(id); await cache.set(cacheKey, product); return product; }, }, };
In this example, the resolver function checks if the product data is already cached using the cache key, which includes the ID of the product. If the product is found in the cache, it is returned directly.
If the product is not found in the cache, the resolver fetches the product data from the remote service and stores it in the cache using the cache key. The next time the same product is requested, it will be served from the cache, reducing the load on the remote service.
Field-level caching can significantly improve the performance of GraphQL APIs by reducing the number of requests made to external services and improving response times for frequently accessed data.
Strategies for Pagination in GraphQL
Pagination is a common requirement in GraphQL APIs when dealing with large datasets. GraphQL provides various strategies for implementing pagination, depending on the specific use case and requirements.
Let's explore two common strategies for pagination in GraphQL: offset-based pagination and cursor-based pagination.
Offset-based pagination involves using the "skip" and "limit" arguments to specify the number of items to skip and the maximum number of items to return. This strategy is straightforward but can be inefficient for large datasets, as offsets can become expensive to compute.
type Query { products(offset: Int, limit: Int): [Product] }
In this example, the "products" query takes "offset" and "limit" arguments to specify the pagination parameters. The "offset" argument indicates the number of items to skip, while the "limit" argument indicates the maximum number of items to return.
To implement offset-based pagination, we can use the "slice" method or equivalent in our resolver:
const resolvers = { Query: { products: (parent, { offset, limit }) => { // Fetch all products from the database or another data source const allProducts = getAllProducts(); // Apply pagination using the offset and limit arguments const paginatedProducts = allProducts.slice(offset, offset + limit); return paginatedProducts; }, }, };
In this example, the resolver fetches all products from the database or another data source. It then applies pagination using the "offset" and "limit" arguments to slice the array of products and return the paginated subset.
While offset-based pagination is simple to implement, it can be inefficient for large datasets, as the database needs to skip a large number of items before returning the requested subset.
Cursor-based pagination provides a more efficient alternative by using opaque cursors to represent the position in the dataset. Instead of relying on offsets, cursor-based pagination uses the "after" and "first" arguments to specify the cursor and the maximum number of items to return.
type Query { products(after: String, first: Int): ProductConnection } type ProductConnection { edges: [ProductEdge] pageInfo: PageInfo! } type ProductEdge { cursor: String! node: Product! } type PageInfo { hasNextPage: Boolean! endCursor: String }
In this example, the "products" query takes "after" and "first" arguments to specify the pagination parameters. The "after" argument represents the cursor indicating the position in the dataset, while the "first" argument represents the maximum number of items to return.
To implement cursor-based pagination, we need to modify our resolver to return the paginated results, along with the cursor and page information:
const resolvers = { Query: { products: (parent, { after, first }) => { // Fetch products from the database or another data source const products = getAllProducts(); // Apply pagination using the after and first arguments const startIndex = after ? products.findIndex((product) => product.id === after) + 1 : 0; const endIndex = startIndex + first; const paginatedProducts = products.slice(startIndex, endIndex); // Create the edges and pageInfo objects const edges = paginatedProducts.map((product) => ({ cursor: product.id, node: product, })); const pageInfo = { hasNextPage: endIndex < products.length, endCursor: paginatedProducts.length > 0 ? paginatedProducts[paginatedProducts.length - 1].id : null, }; return { edges, pageInfo, }; }, }, };
In this example, the resolver finds the index of the cursor in the array of products and uses it to determine the start index for pagination. It then slices the array to return the paginated subset.
The resolver also creates the "edges" array, which includes the cursor and the corresponding product node. The "pageInfo" object indicates whether there is a next page and provides the cursor of the last item in the current page.
Cursor-based pagination provides efficient navigation through large datasets and avoids the need for expensive offset computations. It also allows for more flexible pagination strategies, such as backward pagination or bidirectional pagination.
Effective Error Handling in GraphQL APIs
Error handling is a critical aspect of building robust GraphQL APIs. GraphQL provides a structured approach to handling errors by allowing you to define specific error types and return detailed error messages to clients.
To handle errors in a GraphQL API, you need to consider two types of errors: client errors and server errors.
Client errors occur when the client provides invalid input or sends a malformed query. GraphQL provides a built-in "ValidationError" type that can be used to represent client errors.
type Query { user(id: ID!): User } type User { id: ID! name: String! email: String! }
In this example, the "user" query expects an "id" argument of type ID. If the client provides an invalid ID, we can throw a "ValidationError" with a custom error message:
const resolvers = { Query: { user: (parent, { id }) => { if (!isValidId(id)) { throw new ValidationError('Invalid ID'); } // Fetch user data from the database or another data source const userData = getUserById(id); return userData; }, }, };
In this example, the resolver checks if the provided ID is valid. If it's not, a "ValidationError" is thrown with the error message "Invalid ID". The GraphQL engine will catch the error and return it to the client, along with the corresponding error type.
Server errors occur when there are issues with the server-side implementation, such as database errors or network failures. GraphQL provides a built-in "GraphQLError" type that can be used to represent server errors.
To handle server errors, we can use try-catch blocks or error handling middleware in our resolver functions. We can then throw a "GraphQLError" with a custom error message:
const resolvers = { Query: { user: (parent, { id }) => { try { // Fetch user data from the database or another data source const userData = getUserById(id); return userData; } catch (error) { throw new GraphQLError('Internal server error'); } }, }, };
In this example, the resolver wraps the database query in a try-catch block. If an error occurs, a "GraphQLError" is thrown with the error message "Internal server error". The GraphQL engine will catch the error and return it to the client, indicating that there was an issue on the server side.
Related Article: Sorting Data by Date in GraphQL: A Technical Overview
Performance Optimization Techniques for GraphQL APIs
Performance optimization is crucial for ensuring the responsiveness and scalability of GraphQL APIs. There are various techniques and best practices that can be applied to optimize the performance of your API.
One important technique is to minimize the number of round trips to the server by using batched requests or batched mutations. Instead of making multiple individual requests, you can combine multiple queries or mutations into a single request to reduce latency and network overhead.
# Batched query example query { user(id: "1") { name } product(id: "1") { name } }
In this example, we combine two queries into a single request to fetch the user and product data in a single round trip.
To handle batched requests or mutations, you need to modify your resolver functions to accept an array of requests instead of a single request. You can then process the batched requests in parallel or use batch processing techniques to optimize performance.
Another technique for performance optimization is to implement data loaders, which help reduce the number of database or data source queries. Data loaders allow you to batch and cache requests to external services, avoiding unnecessary duplicate requests.
const DataLoader = require('dataloader'); const userLoader = new DataLoader(async (ids) => { const users = await fetchUsersByIds(ids); return ids.map((id) => users.find((user) => user.id === id)); }); const resolvers = { Query: { user: (parent, { id }) => userLoader.load(id), }, };
In this example, we use the "dataloader" library to implement a user data loader. The data loader accepts an array of user IDs and fetches the corresponding users from the data source. It then returns the users in the same order as the input IDs.
Caching is another important technique for performance optimization in GraphQL APIs. By caching frequently accessed data, you can reduce the load on underlying data sources and improve response times.
You can implement caching at various levels, such as query-level caching, field-level caching, or result-level caching. Depending on your requirements and the caching strategy, you can choose the appropriate level of caching for your API.
Implementing efficient database queries is also crucial for optimizing performance. By using appropriate indexes, query optimization techniques, and efficient data fetching strategies, you can minimize the time spent on executing database queries and improve overall response times.
Lastly, it's important to monitor and analyze the performance of your GraphQL API to identify performance bottlenecks and optimize accordingly. Use tools like performance monitoring and profiling tools to gain insights into query execution times, resolver performance, and overall system performance.
Implementing Versioning in a GraphQL API
Versioning is a common practice in API development to manage changes and ensure backward compatibility. In GraphQL, versioning can be achieved by introducing new types, fields, or arguments while keeping the existing types and fields unchanged.
Let's consider an example where we want to introduce a new field in the "User" type without breaking existing clients. We can achieve this by introducing a new version of the type and providing a resolver for the new field.
type User { id: ID! name: String! email: String! } type UserV2 { id: ID! name: String! email: String! age: Int! } type Query { user(id: ID!): User userV2(id: ID!): UserV2 }
In this example, we introduce a new version of the "User" type called "UserV2" that includes an additional "age" field. The existing "User" type remains unchanged to maintain backward compatibility.
To implement the resolver for the new field, we can define a resolver function for the "userV2" field:
const resolvers = { Query: { userV2: (parent, { id }) => { // Fetch user data from the database or another data source const userData = getUserById(id); // Extend the user data with the age field const userV2Data = { ...userData, age: calculateUserAge(userData) }; return userV2Data; }, }, };
In this example, the resolver function fetches the user data from the database or another data source, just like the resolver for the "user" field. However, it also calculates the user's age using a custom logic and adds it to the user data before returning it.
Versioning in GraphQL allows API developers to introduce changes without breaking existing clients, providing flexibility and backward compatibility.
Additional Resources
- Using GraphQL Subscriptions in Production