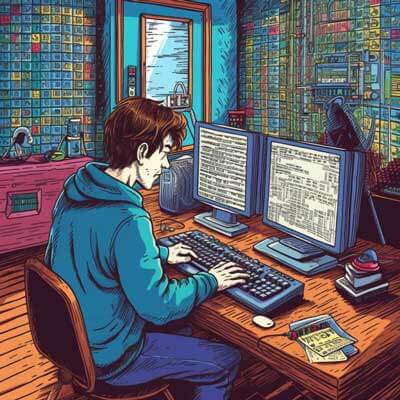
- How to Set a Default Value for a GraphQL Field
- What is the Purpose of Default Values in GraphQL
- How Can I Specify a Default Value for a GraphQL Argument
- Can I Have Multiple Default Values for a Single GraphQL Field
- What Happens if a Default Value is Not Provided for a GraphQL Argument
- How to Define a Default Value for a GraphQL Input Object Field
- Are Default Values Mandatory in GraphQL
- Can I Override a Default Value in GraphQL
- What are the Different Ways to Set Default Values in GraphQL
- How to Handle Default Values in GraphQL Mutations
- Additional Resources
How to Set a Default Value for a GraphQL Field
In GraphQL, fields represent the properties or data that can be requested from a GraphQL API. When defining a field in a GraphQL schema, you can specify a default value for that field. This default value will be used if no explicit value is provided for the field in the GraphQL query.
To set a default value for a GraphQL field, you need to define the field in the schema using the GraphQL schema definition language (SDL). In the SDL, you can use the =
symbol followed by the default value to assign a default value to a field.
Let’s consider an example where we have a User
type with a role
field that has a default value of "user"
:
type User { id: ID! name: String! role: String = "user" }
In this example, if the role
field is not explicitly provided in the GraphQL query, the default value of "user"
will be returned.
Related Article: Exploring GraphQL Integration with Snowflake
What is the Purpose of Default Values in GraphQL
The purpose of default values in GraphQL is to provide a fallback value for fields or arguments when no explicit value is provided. Default values can be useful in several scenarios:
1. Ensuring backward compatibility: If you need to introduce a new field or argument to an existing GraphQL API, setting a default value allows clients to omit the new field or argument in their queries without causing errors.
2. Simplifying client code: Default values can remove the need for clients to always provide a value for a field or argument. This can make client code more concise and reduce the number of required input parameters.
3. Providing sensible defaults: Default values can be used to set reasonable or commonly used values for fields or arguments. This can improve the user experience by reducing the burden on clients to always specify values.
How Can I Specify a Default Value for a GraphQL Argument
In GraphQL, arguments are used to pass parameters to fields or directives in a query. Just like fields, you can also specify default values for arguments in the GraphQL schema.
To specify a default value for a GraphQL argument, you need to define the argument in the schema using the SDL and assign a default value to it using the =
symbol.
Let’s consider an example where we have a users
field that accepts an argument status
, which has a default value of "active"
:
type Query { users(status: String = "active"): [User!]! } type User { id: ID! name: String! status: String! }
In this example, if the status
argument is not explicitly provided in the query, the default value of "active"
will be used.
You can also specify default values for arguments of custom input object types. We will explore this further in the next section.
Can I Have Multiple Default Values for a Single GraphQL Field
No, in GraphQL, you cannot have multiple default values for a single field. Each field can have at most one default value, which is specified in the schema.
If you need different default values for a field based on certain conditions, you can consider using nullable types and conditional resolvers to achieve the desired behavior.
Related Article: Working with FormData in GraphQL Programming
What Happens if a Default Value is Not Provided for a GraphQL Argument
If a default value is not provided for a GraphQL argument and the argument is not explicitly provided in the query, the argument will be considered as null
.
For example, let’s consider a user
field that accepts an argument id
with no default value:
type Query { user(id: ID): User } type User { id: ID! name: String! }
If the id
argument is not provided in the query, the id
argument will be null
and the resolver function for the user
field can handle the null
value accordingly.
How to Define a Default Value for a GraphQL Input Object Field
In GraphQL, you can define input object types that are used to pass complex input data to fields or arguments. These input object types can also have fields with default values.
To define a default value for a field of an input object type, you need to specify the default value in the SDL when defining the input object type.
Let’s consider an example where we have an input object type UserInput
with a role
field that has a default value of "user"
:
input UserInput { name: String! role: String = "user" }
In this example, if the role
field is not explicitly provided when passing an instance of UserInput
as an argument, the default value of "user"
will be used.
Are Default Values Mandatory in GraphQL
No, default values are not mandatory in GraphQL. Fields and arguments can be defined without default values, and it is up to the client to provide explicit values for them.
Default values are optional and can be used to provide fallback values when no explicit value is provided.
Related Article: Tutorial: Functions of a GraphQL Formatter
Can I Override a Default Value in GraphQL
Yes, you can override a default value in GraphQL by explicitly providing a value for the field or argument in the GraphQL query.
When a value is explicitly provided, it will take precedence over the default value specified in the schema.
For example, let’s consider a user
field with a default value of "user"
:
type User { id: ID! name: String! role: String = "user" }
If a client wants to override the default value of "user"
and provide a different value for the role
field, they can do so by including the role
field in their query and specifying a different value.
What are the Different Ways to Set Default Values in GraphQL
There are a few different ways to set default values in GraphQL:
1. Default values for fields: You can set default values for fields by defining them in the schema using the SDL. The default value is assigned using the =
symbol.
2. Default values for arguments: You can set default values for arguments of fields by defining them in the schema using the SDL. The default value is assigned using the =
symbol.
3. Default values for input object fields: You can set default values for fields of input object types by defining them in the schema using the SDL. The default value is assigned using the =
symbol.
4. Default values in resolvers: In addition to setting default values in the schema, you can also set default values in resolver functions. This can be useful when you need more complex default value logic or when the default value depends on runtime information.
How to Handle Default Values in GraphQL Mutations
When handling default values in GraphQL mutations, you can follow a similar approach as with fields and arguments. You can define default values for input object fields used in mutations and handle those default values in your resolver functions.
Let’s consider an example where we have a mutation createUser
that accepts an input object type UserInput
with a default value for the role
field:
type Mutation { createUser(input: UserInput): User! } input UserInput { name: String! role: String = "user" } type User { id: ID! name: String! role: String! }
In this example, if the role
field is not explicitly provided when calling the createUser
mutation, the default value of "user"
will be used. The resolver function for the mutation can handle the default value and create a user with the specified or default role.
Related Article: Exploring OneOf in GraphQL Programming
Additional Resources
– The Purpose of a Schema in GraphQL
– Resolvers in GraphQL
– Understanding Mutations in GraphQL