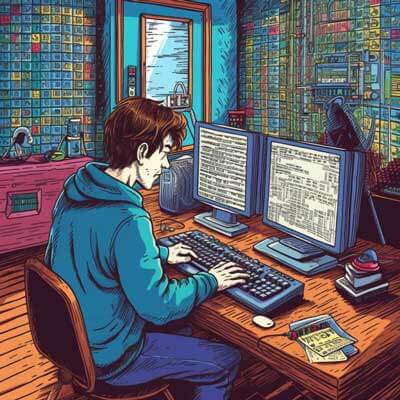
- Understanding the SWAPI REST API
- Exploring SWAPI HTTP Requests
- Querying SWAPI with GraphQL
- Making GraphQL Queries to SWAPI
- Advantages of SWAPI GraphQL over REST API
- SWAPI GraphQL with Different Programming Languages
- Limitations of SWAPI GraphQL
- Performing Mutations with SWAPI GraphQL
- Schema of SWAPI GraphQL
- Pagination in SWAPI GraphQL
- SWAPI GraphQL with Apollo Client
- Real-Time Data Updates with SWAPI GraphQL
- Additional Resources
Understanding the SWAPI REST API
The SWAPI (Star Wars API) is a RESTful API that provides a wealth of information about the Star Wars universe. It allows developers to retrieve information about various entities such as films, characters, starships, vehicles, and more.
To interact with the SWAPI REST API, developers make HTTP requests to specific endpoints. These endpoints correspond to different types of resources available in the API. For example, to retrieve information about a specific film, you would make a GET request to the /films/{id}
endpoint, where {id}
is the ID of the film.
Let’s take a look at an example of making a GET request to the SWAPI REST API using the requests
library in Python:
import requests response = requests.get('https://swapi.dev/api/films/1/') data = response.json() print(data)
In this example, we make a GET request to retrieve information about the first film in the Star Wars saga. The response is returned in JSON format, which we can then parse and use in our application.
Related Article: Working with GraphQL Enums: Values Explained
Exploring SWAPI HTTP Requests
To interact with the SWAPI REST API, developers can make various types of HTTP requests, such as GET, POST, PUT, and DELETE. Each request type serves a different purpose and is used to perform specific actions on the API resources.
Let’s explore some of the common HTTP requests used with the SWAPI REST API:
GET Request: GET requests are used to retrieve information from the API. For example, to get information about a specific character, you would make a GET request to the /people/{id}
endpoint.
response = requests.get('https://swapi.dev/api/people/1/') data = response.json() print(data)
POST Request: POST requests are used to create new resources on the API. For example, to create a new starship, you would make a POST request to the /starships/
endpoint.
new_starship = { "name": "Death Star", "model": "DS-1 Orbital Battle Station", "manufacturer": "Imperial Department of Military Research", "crew": "342,953", "passengers": "843,342", "starship_class": "Deep Space Mobile Battlestation" } response = requests.post('https://swapi.dev/api/starships/', json=new_starship) data = response.json() print(data)
PUT Request: PUT requests are used to update existing resources on the API. For example, to update the details of a specific starship, you would make a PUT request to the /starships/{id}
endpoint.
updated_starship = { "name": "Death Star II", "model": "DS-2 Orbital Battle Station", "manufacturer": "Imperial Department of Military Research", "crew": "1,000,000", "passengers": "1,000,000", "starship_class": "Deep Space Mobile Battlestation" } response = requests.put('https://swapi.dev/api/starships/9/', json=updated_starship) data = response.json() print(data)
DELETE Request: DELETE requests are used to delete existing resources from the API. For example, to delete a specific starship, you would make a DELETE request to the /starships/{id}
endpoint.
response = requests.delete('https://swapi.dev/api/starships/9/') if response.status_code == 204: print("Starship deleted successfully") else: print("Failed to delete starship")
These are just a few examples of how you can use different HTTP requests to interact with the SWAPI REST API. The choice of request type depends on the action you want to perform on the API resources.
Querying SWAPI with GraphQL
GraphQL is a query language and runtime for APIs that allows developers to request specific data and shape the response according to their needs. Unlike REST APIs, where you often receive a fixed set of data, GraphQL allows you to fetch only the data you need, reducing network overhead and improving performance.
With GraphQL, instead of making multiple requests to different endpoints to retrieve related data, you can make a single request and specify the exact data you need using a query.
SWAPI also provides a GraphQL API that allows developers to query the data in a more flexible and efficient way. Let’s explore how we can query SWAPI using GraphQL.
Making GraphQL Queries to SWAPI
To make GraphQL queries to the SWAPI, you need to send a POST request to the GraphQL endpoint (https://swapi-graphql.netlify.app/.netlify/functions/index
).
Here’s an example of a GraphQL query to retrieve information about a specific film:
query { film(id: 1) { title director releaseDate openingCrawl } }
In this query, we’re requesting the title
, director
, releaseDate
, and openingCrawl
fields for the film with an ID of 1.
To make this query, you can use any GraphQL client library or tool. For example, in JavaScript, you can use the graphql-request
library:
import { request, gql } from 'graphql-request'; const query = gql` query { film(id: 1) { title director releaseDate openingCrawl } } `; request('https://swapi-graphql.netlify.app/.netlify/functions/index', query) .then(data => console.log(data)) .catch(error => console.error(error));
This code sends the GraphQL query to the SWAPI GraphQL endpoint and logs the response data to the console.
Related Article: Exploring GraphQL Integration with Snowflake
Advantages of SWAPI GraphQL over REST API
SWAPI GraphQL offers several advantages over the REST API:
1. Flexible Queries: With GraphQL, you can retrieve only the data you need, reducing over-fetching and under-fetching of data. This can lead to improved performance and reduced bandwidth usage.
2. Reduced Network Requests: Instead of making multiple requests to different endpoints, you can make a single GraphQL query to fetch related data. This reduces the number of network requests and simplifies client-side code.
3. Schema Introspection: GraphQL provides built-in schema introspection, allowing you to explore the available types, fields, and relationships in the API. This makes it easier to understand the data structure and write accurate queries.
4. Strong Typing: GraphQL supports type checking, which helps catch errors at compile-time rather than runtime. This can improve code quality and reduce bugs.
5. Versioning: With REST APIs, versioning can be challenging when introducing breaking changes. GraphQL, on the other hand, allows you to evolve the API without impacting existing clients. You can add new fields and types without breaking existing queries.
Overall, SWAPI GraphQL provides a more flexible and efficient way to query the Star Wars API, making it easier for developers to work with the data.
SWAPI GraphQL with Different Programming Languages
SWAPI GraphQL can be used with various programming languages and frameworks. Here are examples of how you can make GraphQL queries to SWAPI using different programming languages:
Python (with graphql-python
library):
from graphqlclient import GraphQLClient client = GraphQLClient('https://swapi-graphql.netlify.app/.netlify/functions/index') query = ''' query { film(id: 1) { title director releaseDate openingCrawl } } ''' result = client.execute(query) print(result)
JavaScript (with graphql-request
library):
import { request, gql } from 'graphql-request'; const query = gql` query { film(id: 1) { title director releaseDate openingCrawl } } `; request('https://swapi-graphql.netlify.app/.netlify/functions/index', query) .then(data => console.log(data)) .catch(error => console.error(error));
Java (with graphql-java
library):
import com.graphqljava.okhttp.GraphQLOkHttp; String query = "{ film(id: 1) { title director releaseDate openingCrawl } }"; GraphQLOkHttp client = new GraphQLOkHttp("https://swapi-graphql.netlify.app/.netlify/functions/index"); String result = client.execute(query); System.out.println(result);
These examples demonstrate how you can make GraphQL queries to SWAPI using different programming languages. The specific libraries and tools may vary, but the overall approach remains the same.
Limitations of SWAPI GraphQL
While SWAPI GraphQL offers many advantages, it also has some limitations:
1. Limited Functionality: SWAPI GraphQL is a community-driven project and may not provide the same level of functionality as the original SWAPI REST API. Some endpoints or features may be missing or not fully implemented.
2. Performance Concerns: GraphQL queries can be more complex and require additional processing compared to REST API requests. This can potentially impact performance, especially when dealing with large datasets or complex queries.
3. Lack of Standardization: GraphQL allows developers to define their own schemas, which can lead to inconsistencies and lack of standardization across different GraphQL APIs. This may require additional effort to understand and work with each API.
It’s important to consider these limitations when deciding whether to use SWAPI GraphQL or the REST API. Evaluate your specific requirements and consider the trade-offs before making a decision.
Related Article: Working with FormData in GraphQL Programming
Performing Mutations with SWAPI GraphQL
In addition to querying data, you can also perform mutations with SWAPI GraphQL. Mutations allow you to modify data on the server, such as creating, updating, or deleting resources.
Here’s an example of a mutation to create a new starship:
mutation { createStarship( input: { name: "Death Star" model: "DS-1 Orbital Battle Station" manufacturer: "Imperial Department of Military Research" crew: "342,953" passengers: "843,342" starshipClass: "Deep Space Mobile Battlestation" } ) { starship { id name model manufacturer crew passengers starshipClass } } }
This mutation creates a new starship with the specified details and returns the ID, name, model, manufacturer, crew, passengers, and starship class of the created starship.
To perform this mutation, you can use the same GraphQL client libraries or tools mentioned earlier.
Schema of SWAPI GraphQL
The schema of SWAPI GraphQL defines the available types, fields, and relationships in the API. It serves as a contract between the server and the client, specifying what data can be queried and how it should be structured.
You can explore the schema of SWAPI GraphQL by using tools like GraphQL Playground or GraphiQL. These tools provide an interactive environment where you can browse the available types, fields, and perform queries.
Here’s an example of how to explore the schema using GraphQL Playground:
1. Open GraphQL Playground in your web browser.
2. Set the endpoint to https://swapi-graphql.netlify.app/.netlify/functions/index
.
3. Click on the “SCHEMA” tab to view the schema.
4. Expand the types and fields to explore the available data.
The schema provides a comprehensive overview of the data available in SWAPI GraphQL, making it easier to understand and work with the API.
Pagination in SWAPI GraphQL
Pagination is a common technique used in APIs to handle large datasets by returning data in smaller chunks or pages. SWAPI GraphQL also supports pagination, allowing you to retrieve data in batches.
To paginate through the results in SWAPI GraphQL, you can use the first
and after
arguments in your queries. The first
argument specifies the number of items to retrieve, and the after
argument specifies the cursor indicating the starting point for the query.
Here’s an example of a query with pagination:
query { allFilms(first: 5, after: "YXJyYXljb25uZWN0aW9uOjA=") { edges { node { title director releaseDate } } pageInfo { endCursor hasNextPage } } }
In this example, we’re retrieving the first 5 films after the specified cursor. The edges
field contains the film nodes, and the pageInfo
field provides information about the pagination, including the endCursor
and hasNextPage
values.
Related Article: Tutorial: Functions of a GraphQL Formatter
SWAPI GraphQL with Apollo Client
Apollo Client is a useful GraphQL client library that makes it easy to work with GraphQL APIs in various programming languages and frameworks. It provides features such as caching, optimistic UI, and real-time data updates.
To use Apollo Client with SWAPI GraphQL, you need to configure the client with the GraphQL endpoint and define your queries and mutations. Here’s an example of using Apollo Client in a React application:
import { ApolloClient, InMemoryCache, gql } from '@apollo/client'; const client = new ApolloClient({ uri: 'https://swapi-graphql.netlify.app/.netlify/functions/index', cache: new InMemoryCache() }); const GET_FILM = gql` query GetFilm($id: ID!) { film(id: $id) { title director releaseDate openingCrawl } } `; client.query({ query: GET_FILM, variables: { id: "1" } }) .then(response => console.log(response.data)) .catch(error => console.error(error));
In this example, we define a GraphQL query using the gql
template literal tag and pass it to the query
method of Apollo Client. We also specify the variables required by the query, such as the film ID.
Apollo Client handles the network request, caching, and response handling, making it easier to work with SWAPI GraphQL in your application.
Real-Time Data Updates with SWAPI GraphQL
Real-time data updates, also known as subscriptions, allow you to receive real-time updates from the server when specific events occur. While SWAPI GraphQL does not support subscriptions out of the box, you can use additional tools and libraries to implement real-time functionality.
One popular library for adding real-time capabilities to GraphQL APIs is Apollo Server. Apollo Server allows you to define subscriptions in your GraphQL schema and handle the real-time communication between the server and the clients.
Here’s an example of how you can implement real-time data updates with Apollo Server and SWAPI GraphQL:
// Import necessary modules and define the schema const typeDefs = ` type Film { id: ID! title: String! director: String! releaseDate: String! openingCrawl: String! } type Query { film(id: ID!): Film } type Subscription { filmUpdated: Film } `; // Implement the resolvers for the queries and subscriptions const resolvers = { Query: { film: (parent, args) => { // Implement the logic to retrieve a film }, }, Subscription: { filmUpdated: { subscribe: () => { // Implement the logic to subscribe to film updates }, }, }, }; // Create an Apollo Server instance and start it const server = new ApolloServer({ typeDefs, resolvers }); server.listen().then(({ url }) => { console.log(`Server ready at ${url}`); });
In this example, we define a Subscription
type in the schema and implement a filmUpdated
subscription resolver. The subscription resolver handles the logic to subscribe to film updates and sends the updated film data to the clients.
Overall, SWAPI GraphQL provides a more flexible and efficient way to query and manipulate data from the Star Wars API. By leveraging the power of GraphQL, developers can retrieve and shape the data according to their needs, making it easier to build applications that consume and interact with SWAPI.
Additional Resources
– What is the purpose of a schema in GraphQL?
– How do you write a query in GraphQL?
– What is a mutation in GraphQL?