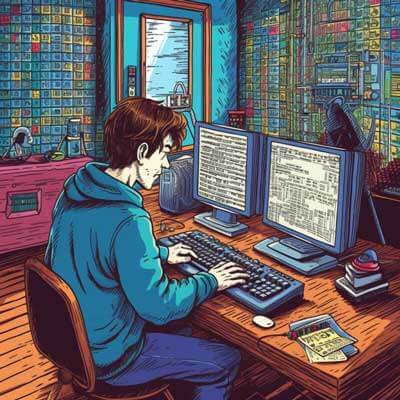
Table of Contents
The Purpose of a GraphQL Mutation
A mutation in GraphQL is used to modify or change data on the server. It allows clients to send requests to the server to create, update, or delete data. Mutations are similar to queries in GraphQL, but while queries are used for retrieving data, mutations are used for modifying data.
In order to implement an upsert operation in GraphQL, we need to understand the purpose of a mutation. The upsert operation combines the functionalities of both updating and inserting data. It checks if the data already exists, and if it does, it updates it. If the data does not exist, it inserts it as a new record.
Let's take a look at an example of how to implement an upsert operation in GraphQL using a mutation. Suppose we have a schema with a "User" type and we want to implement an upsert operation for creating or updating a user.
First, we define the mutation in our schema:
type Mutation { upsertUser(id: ID!, name: String!, email: String!): User! }
In this example, the upsertUser
mutation takes three arguments: id
, name
, and email
. The id
argument is used to identify the user, while the name
and email
arguments are used to update or insert the user's name and email.
Next, we implement the resolver function for the upsertUser
mutation. The resolver function is responsible for handling the logic of the mutation and interacting with the data source. Here's an example of how the resolver function might look like in JavaScript:
const upsertUser = async (_, { id, name, email }, { dataSources }) => { // Check if the user already exists const existingUser = await dataSources.userAPI.getUserById(id); if (existingUser) { // Update the user const updatedUser = await dataSources.userAPI.updateUser(id, name, email); return updatedUser; } else { // Insert a new user const newUser = await dataSources.userAPI.createUser(id, name, email); return newUser; } }; module.exports = { Mutation: { upsertUser, }, };
In this example, we first check if the user already exists by calling the getUserById
method of the userAPI
data source. If the user exists, we update the user by calling the updateUser
method. If the user does not exist, we insert a new user by calling the createUser
method.
Related Article: Exploring Default Values in GraphQL Programming
Defining Data Structure with a GraphQL Schema
In GraphQL, the schema defines the structure of the data that can be queried or mutated. It acts as a contract between the client and the server, specifying what types of data can be requested and what operations can be performed on that data.
To implement an upsert operation in GraphQL, we first need to define the data structure in the schema. This involves defining the types, fields, and relationships between the types.
Let's continue with the previous example of a "User" type and define its structure in the schema:
type User { id: ID! name: String! email: String! } type Query { getUserById(id: ID!): User } type Mutation { upsertUser(id: ID!, name: String!, email: String!): User! }
In this example, we define a "User" type with three fields: id
, name
, and email
. The id
field is of type ID
, which represents a unique identifier. The name
and email
fields are of type String
.
We also define a Query
type with a single field getUserById
, which takes an id
argument and returns a User
. This allows clients to query for a user by their ID.
Finally, we define a Mutation
type with a single field upsertUser
, which takes the id
, name
, and email
arguments and returns a User
. This allows clients to upsert a user by providing their ID, name, and email.
The Role of a Resolver in GraphQL
In GraphQL, resolvers are functions that are responsible for resolving the value of a field in a type. They define how the data is fetched or computed for a particular field.
To implement an upsert operation in GraphQL, we need to understand the role of a resolver. Resolvers are essential for handling the logic of mutations and querying data from a data source.
Let's continue with the previous example and implement the resolvers for the getUserById
and upsertUser
fields.
First, we define the resolvers object with the resolver functions for the Query
and Mutation
types:
const resolvers = { Query: { getUserById: async (_, { id }, { dataSources }) => { return await dataSources.userAPI.getUserById(id); }, }, Mutation: { upsertUser: async (_, { id, name, email }, { dataSources }) => { // Implementation of the upsertUser resolver }, }, }; module.exports = resolvers;
In this example, we define the resolver function for the getUserById
field in the Query
type. The resolver function takes the id
argument and the dataSources
object, which provides access to the data source. Inside the resolver function, we call the getUserById
method of the userAPI
data source to fetch the user by their ID.
For the upsertUser
field in the Mutation
type, we leave the implementation of the resolver function empty for now.
Resolvers are the heart of a GraphQL API as they define how the data is fetched or computed for each field. They provide a flexible and customizable way to handle the logic of mutations and querying data from a data source.
Creating a GraphQL API
Creating a GraphQL API involves setting up a GraphQL server that handles incoming queries and mutations from clients. There are several ways to create a GraphQL API, depending on the programming language and frameworks you are using.
To implement an upsert operation in GraphQL, we need to create a GraphQL API that supports mutations. This involves setting up a server, defining the schema, and implementing the resolvers.
Let's continue with the previous example and create a GraphQL API using Apollo Server, a popular GraphQL server implementation for JavaScript.
First, we install the required dependencies:
npm install apollo-server graphql
Next, we create a file named server.js
and set up the Apollo Server:
const { ApolloServer } = require('apollo-server'); const typeDefs = require('./schema'); const resolvers = require('./resolvers'); const server = new ApolloServer({ typeDefs, resolvers, }); server.listen().then(({ url }) => { console.log(`Server running at ${url}`); });
In this example, we import the ApolloServer
class from the apollo-server
package. We also import the typeDefs
and resolvers
from separate files (schema.js
and resolvers.js
).
We then create a new instance of ApolloServer
and pass in the typeDefs
and resolvers
to configure the server.
Finally, we call the listen
method of the server to start the GraphQL API. The server listens on a port (by default, port 4000) and logs the URL where it is running.
With this setup, we have created a GraphQL API that supports mutations. Clients can send queries and mutations to the server, and the server will handle them using the defined schema and resolvers.
Related Article: Sorting Data by Date in GraphQL: A Technical Overview
Understanding the Functionality of a GraphQL Server
A GraphQL server is responsible for receiving and handling GraphQL requests from clients. It acts as a mediator between the client and the data source, resolving queries and mutations based on the defined schema and resolvers.
To implement an upsert operation in GraphQL, we need to understand the functionality of a GraphQL server. The server is responsible for parsing and validating GraphQL requests, executing the requested operations, and returning the results to the client.
Let's continue with the previous example and explore the functionality of a GraphQL server using Apollo Server.
When the server receives a GraphQL request, it goes through the following steps:
1. Parsing: The server parses the request and extracts the query or mutation operation, variables, and operation name from the request payload.
2. Validation: The server validates the parsed request against the defined schema to ensure that the requested fields, arguments, and types are valid.
3. Execution: The server executes the requested operation by calling the appropriate resolver functions. Resolvers are called in a specific order, following the structure of the requested operation.
4. Resolution: Resolvers fetch or compute the data for each field in the requested operation. They can interact with data sources, perform calculations, or fetch data from external APIs.
5. Result formatting: The server formats the resolved data into the response payload, following the structure defined in the schema. It also includes any errors or validation messages if applicable.
6. Sending the response: The server sends the formatted response payload back to the client.
Differences Between a GraphQL Query and a Mutation
In GraphQL, queries and mutations are used to retrieve and modify data, respectively. While both queries and mutations are used to interact with the server, there are some key differences between them.
To implement an upsert operation in GraphQL, we need to understand the differences between queries and mutations. This will allow us to use the appropriate operation for retrieving or modifying data.
Queries are used to retrieve data from the server. They are read-only operations and do not modify any data on the server. Queries are executed in parallel, allowing clients to request multiple fields and related data in a single request.
Here's an example of a query that retrieves a user by their ID:
query { getUserById(id: "123") { id name email } }
In this example, the getUserById
query takes an id
argument and returns the id
, name
, and email
fields of the user.
On the other hand, mutations are used to modify or change data on the server. They can create, update, or delete data. Mutations are executed sequentially, meaning that each mutation is executed one after another. This ensures that mutations are applied in the expected order.
Here's an example of a mutation that upserts a user by their ID:
mutation { upsertUser(id: "123", name: "John Doe", email: "john@example.com") { id name email } }
In this example, the upsertUser
mutation takes the id
, name
, and email
arguments and returns the id
, name
, and email
fields of the upserted user.
Exploring GraphQL Types and Their Definitions
In GraphQL, types define the structure of the data that can be queried or mutated. They represent the shape of the data and specify the fields and their types.
To implement an upsert operation in GraphQL, we need to explore the types and their definitions. This will allow us to define the data structure and specify the fields and their types.
Let's continue with the previous example and explore the types and their definitions.
In GraphQL, types can be scalar or object types. Scalar types represent primitive values, such as strings, numbers, booleans, or dates. Object types represent complex data structures and can have fields of scalar or other object types.
In our example, we have a scalar type ID
and an object type User
. Here's how we define them in the schema:
scalar ID type User { id: ID! name: String! email: String! }
In this example, we define the scalar type ID
using the scalar
keyword. The ID
type represents a unique identifier.
We also define the object type User
with three fields: id
, name
, and email
. The id
field is of type ID
and is marked with an exclamation mark (!
) to indicate that it is non-nullable. The name
and email
fields are of type String
.
An Introduction to GraphQL Directives and Their Functionality
In GraphQL, directives are used to provide additional instructions or metadata to the server about how to process a field or fragment. They allow clients to control the execution and behavior of queries and mutations.
To implement an upsert operation in GraphQL, we need to introduce directives and understand their functionality. This will allow us to apply custom logic or behavior to specific fields or fragments.
Let's explore some common directives and their functionality:
1. @include: The @include
directive is used to conditionally include a field or fragment based on a Boolean argument. It takes a Boolean value as an argument and includes the field or fragment if the argument is true
.
query($includeUser: Boolean!) { user { id name @include(if: $includeUser) } }
In this example, the name
field is conditionally included based on the value of the $includeUser
variable.
2. @skip: The @skip
directive is used to conditionally skip a field or fragment based on a Boolean argument. It takes a Boolean value as an argument and skips the field or fragment if the argument is true
.
query($skipUser: Boolean!) { user { id name @skip(if: $skipUser) } }
In this example, the name
field is conditionally skipped based on the value of the $skipUser
variable.
3. @deprecated: The @deprecated
directive is used to mark a field or enum value as deprecated. It takes an optional reason argument to provide additional information about the deprecation.
type User { id: ID! name: String! @deprecated(reason: "Use 'fullName' instead.") fullName: String! }
In this example, the name
field is marked as deprecated and the reason is provided as "Use 'fullName' instead".
Related Article: Exploring Directus GraphQL
How GraphQL Subscriptions Work
In GraphQL, subscriptions are a way to enable real-time communication between the server and the client. They allow clients to subscribe to specific events or data changes on the server and receive updates in real-time.
To implement an upsert operation in GraphQL, we need to understand how subscriptions work. This will allow us to provide real-time updates to clients when data is inserted or updated.
Let's explore how subscriptions work in GraphQL:
1. Define the subscription type in the schema: We start by defining a subscription type in the schema, similar to how we define query and mutation types. The subscription type represents the events or data changes that clients can subscribe to.
type Subscription { newUser: User! }
In this example, we define a newUser
subscription that returns a User
object when a new user is created.
2. Implement the resolver for the subscription: Next, we implement the resolver function for the subscription. The resolver function is responsible for handling the subscription logic and pushing updates to the subscribed clients.
const { PubSub } = require('apollo-server'); const pubsub = new PubSub(); const resolvers = { Subscription: { newUser: { subscribe: () => pubsub.asyncIterator(['NEW_USER']), }, }, }; // Publish a new user const newUser = { id: '123', name: 'John Doe', email: 'john@example.com', }; pubsub.publish('NEW_USER', { newUser }); module.exports = resolvers;
In this example, we create a PubSub
instance from the apollo-server
package. We define the resolver function for the newUser
subscription, which returns an async iterator that listens for events with the NEW_USER
topic. Whenever a new user is published, we use the pubsub.publish
method to push the new user data to the subscribed clients.
3. Subscribe to the subscription from the client: Finally, clients can subscribe to the subscription from their GraphQL client or WebSocket connection. They can receive real-time updates whenever a new user is created.
subscription { newUser { id name email } }
In this example, the client subscribes to the newUser
subscription and receives the id
, name
, and email
fields of the new user whenever a new user is created.
The Usefulness of GraphQL Introspection
GraphQL introspection is a useful feature that allows clients to query the GraphQL schema itself. It provides a way to discover and explore the capabilities of a GraphQL API without relying on external documentation or knowledge of the implementation details.
To implement an upsert operation in GraphQL, we need to understand the usefulness of GraphQL introspection. This will allow us to discover and explore the schema, understand the available types and fields, and make informed queries and mutations.
Let's explore some common use cases and examples of GraphQL introspection:
1. Discovering available types and fields: Clients can use introspection to discover the available types and fields in the schema. This allows them to understand the structure of the data and make informed queries or mutations.
query IntrospectionQuery { __schema { types { name kind } } }
In this example, the client queries the __schema
field and retrieves the list of types in the schema along with their names and kinds (scalar, object, enum, etc.).
2. Retrieving details of a specific type: Clients can use introspection to retrieve the details of a specific type in the schema, such as its fields, arguments, and descriptions.
query IntrospectionQuery { __type(name: "User") { name kind description fields { name type { name kind } } } }
In this example, the client queries the __type
field with the name of the type (User
) and retrieves its name, kind, description, and fields.
3. Validating and documenting the schema: Introspection can be used to validate and document the schema by checking if the schema adheres to certain rules or conventions, and generating documentation based on the introspected schema.
query IntrospectionQuery { __schema { queryType { name } mutationType { name } subscriptionType { name } } }
In this example, the client queries the __schema
field and retrieves the names of the query, mutation, and subscription types in the schema. This can be used to validate that the schema has the required top-level types and to generate documentation based on the introspected schema.
Additional Resources
- GraphQL Schema Definition Language