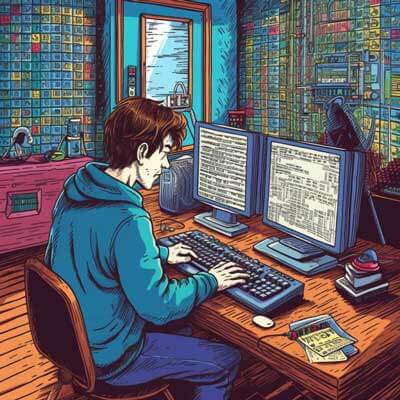
- What is an enum in GraphQL?
- Example
- How can I pass an enum value in a GraphQL query?
- Example
- Can I use an enum as an argument in a GraphQL mutation?
- Example
- How do I define an enum in a GraphQL schema?
- Example
- What is the purpose of a resolver in GraphQL?
- Example
- What are the different types available in GraphQL?
- Example
- What is the role of a directive in a GraphQL query?
- Example
- How can I subscribe to real-time data using GraphQL subscriptions?
- Example
- Can I use a scalar type as an argument in a GraphQL query?
- Example
- What are the best practices for working with enums in GraphQL?
- Additional Resources
What is an enum in GraphQL?
An enum, short for enumeration, is a scalar type in GraphQL that represents a set of predefined values. It allows you to define a specific list of allowed values for a field or an argument. Enums are useful when you want to restrict the input or output of a field or argument to a specific set of options.
In GraphQL, enums can be used to represent a variety of data, such as status codes, categories, or any other type of value that has a fixed set of options. Enums provide a way to define and enforce a specific set of values for specific fields or arguments. They help in maintaining consistency and avoiding invalid inputs or outputs.
Related Article: Working with GraphQL Enums: Values Explained
Example:
Suppose you have a GraphQL schema that defines a “Color” enum:
enum Color { RED BLUE GREEN }
Here, the “Color” enum has three possible values: RED, BLUE, and GREEN. These are the only valid values that can be used in fields or arguments that are of type “Color”. Any other value will result in an error.
How can I pass an enum value in a GraphQL query?
To pass an enum value in a GraphQL query, you simply need to specify the enum value as a string. Enum values are represented as strings in GraphQL queries.
When querying a field that expects an enum value, you can pass the desired enum value as an argument. The argument should be of the same type as the enum defined in the schema.
Example:
Suppose you have a GraphQL schema that defines a “Product” type with a “color” field of type “Color” enum:
type Product { id: ID! name: String! color: Color! } enum Color { RED BLUE GREEN }
To query for products of a specific color, you can pass the enum value as an argument in the query:
query { productsByColor(color: RED) { id name } }
In this example, we are querying for products that have the color RED. The enum value RED is passed as the argument to the “productsByColor” field.
Related Article: Working with FormData in GraphQL Programming
Can I use an enum as an argument in a GraphQL mutation?
Yes, you can use an enum as an argument in a GraphQL mutation. Enums can be used as arguments in mutations just like they can be used in queries.
When defining a mutation that takes an enum argument, you need to specify the argument type as the corresponding enum type defined in the schema. The client can then pass the desired enum value as an argument when executing the mutation.
Example:
Suppose you have a GraphQL schema that defines a mutation to create a new product with a specified color:
type Mutation { createProduct(name: String!, color: Color!): Product! } type Product { id: ID! name: String! color: Color! } enum Color { RED BLUE GREEN }
To create a new product with a specific color, you can pass the enum value as an argument in the mutation:
mutation { createProduct(name: "Example Product", color: BLUE) { id name color } }
In this example, we are executing the “createProduct” mutation and passing the name and color arguments. The enum value BLUE is passed as the color argument.
How do I define an enum in a GraphQL schema?
To define an enum in a GraphQL schema, you need to use the “enum” keyword followed by the name of the enum type. Within the enum block, you define the possible values for the enum.
Each possible value is defined as a separate line, without quotes or commas. The values should be in uppercase by convention, but GraphQL does not enforce this.
Related Article: Tutorial: GraphQL Typename
Example:
Suppose you want to define an enum called “Status” with three possible values: ACTIVE, INACTIVE, and PENDING. The GraphQL schema definition would look like this:
enum Status { ACTIVE INACTIVE PENDING }
In this example, the “Status” enum has three possible values: ACTIVE, INACTIVE, and PENDING.
What is the purpose of a resolver in GraphQL?
A resolver in GraphQL is a function that determines how to fetch the data for a specific field in a GraphQL query. Resolvers are responsible for resolving the value of a field by fetching the data from the appropriate data source.
In a GraphQL schema, each field can have a resolver function associated with it. When a field is requested in a query, the corresponding resolver is invoked to fetch the data for that field.
Resolvers can be written in any programming language and are responsible for handling the business logic of fetching the data. They can retrieve data from a database, perform calculations, call external APIs, or perform any other necessary operations to fulfill the query.
Example:
Suppose you have a GraphQL schema that defines a “Product” type with a “name” field:
type Product { id: ID! name: String! }
To resolve the “name” field, you would need to write a resolver function in your GraphQL server. The resolver function for the “name” field can retrieve the corresponding data from a database or any other data source.
Here’s an example of a resolver function written in JavaScript using the Apollo Server library:
const resolvers = { Product: { name: (parent, args, context, info) => { // Logic to fetch the name of the product return fetchProduct(parent.id).name; } } };
In this example, the resolver function for the “name” field takes four arguments: parent, args, context, and info. The parent argument represents the resolved value of the parent field (if any), args represent the arguments passed to the field, context represents the shared context between all resolvers, and info provides information about the execution state of the query.
The resolver function retrieves the name of the product by calling the fetchProduct function with the parent’s ID and returns the name value.
Related Article: Tutorial: GraphQL Input Interface
What are the different types available in GraphQL?
GraphQL has several built-in scalar types that represent different kinds of data. These scalar types are the fundamental building blocks of GraphQL schemas. The different scalar types available in GraphQL are:
– String: A UTF-8 character sequence.
– Int: A signed 32-bit integer.
– Float: A signed double-precision floating-point value.
– Boolean: True or false.
– ID: A unique identifier, often used to refetch an object or as a key for caching.
In addition to the scalar types, GraphQL also supports complex types such as lists and objects. Lists represent a collection of values of the same type, while objects represent a collection of named fields with their corresponding types.
You can also define custom types in GraphQL by using the “type” keyword followed by the name of the custom type. Custom types can be objects, enums, or scalars.
Example:
Here’s an example of a GraphQL schema that uses different scalar and complex types:
type Query { hello: String! age: Int! price: Float! isTrue: Boolean! productId: ID! products: [Product!]! } type Product { id: ID! name: String! price: Float! }
In this example, the “Query” type has fields that represent different scalar types: “hello” is of type String, “age” is of type Int, “price” is of type Float, “isTrue” is of type Boolean, and “productId” is of type ID.
The “Query” type also has a field “products” that represents a list of “Product” objects. The “Product” object has fields “id” of type ID and “name” of type String.
What is the role of a directive in a GraphQL query?
In GraphQL, a directive is used to modify the behavior of a field or an operation in a query. Directives provide a way to add additional instructions or conditions to the execution of a query.
Directives are specified using the “@” symbol followed by the directive name and any arguments it requires. They can be applied to fields, fragments, or entire query operations.
GraphQL comes with two built-in directives: @include and @skip. The @include directive allows you to conditionally include or exclude a field based on a Boolean value. The @skip directive allows you to conditionally skip a field or fragment based on a Boolean value.
You can also define custom directives in GraphQL to add custom logic or behavior to your queries.
Related Article: Tutorial: Functions of a GraphQL Formatter
Example:
Here’s an example of a GraphQL query that uses the @include and @skip directives:
query { products { id name price @include(if: true) description @skip(if: false) } }
In this example, the “products” field is always included in the query. However, the “price” field is conditionally included based on the value of the “if” argument, which is set to true. The “description” field is conditionally skipped based on the value of the “if” argument, which is set to false.
As a result, the “price” field will be included in the query, while the “description” field will be skipped.
How can I subscribe to real-time data using GraphQL subscriptions?
GraphQL subscriptions allow you to subscribe to real-time data updates from a GraphQL server. Subscriptions are a way to establish a long-lived connection between the client and the server, enabling real-time data streaming.
To use GraphQL subscriptions, you need to define a subscription type in your GraphQL schema. The subscription type defines the available subscription fields and their return types.
The client can then use the subscription fields in a query to subscribe to specific events or data updates. When a subscribed event occurs, the server pushes the updated data to the client, allowing for real-time updates.
Subscriptions are typically implemented using WebSocket protocol, which enables bidirectional communication between the client and the server.
Example:
Here’s an example of a GraphQL schema that includes a subscription type:
type Subscription { newProduct: Product! } type Product { id: ID! name: String! }
In this example, the subscription type includes a field called “newProduct” of type “Product”. This subscription allows clients to subscribe to new product events.
To subscribe to the “newProduct” event, the client needs to send a subscription query to the server:
subscription { newProduct { id name } }
When a new product is created on the server, the server pushes the updated product data to all subscribed clients. The clients receive the updated data and can take appropriate actions, such as updating the UI.
Related Article: Sorting Data by Date in GraphQL: A Technical Overview
Can I use a scalar type as an argument in a GraphQL query?
Yes, you can use a scalar type as an argument in a GraphQL query. Scalar types are the fundamental building blocks of GraphQL schemas, and they can be used as arguments for fields or operations.
When using a scalar type as an argument, you need to specify the argument name and its corresponding type in the query.
Example:
Suppose you have a GraphQL schema that defines a “Product” type with a “price” field of type “Float”:
type Product { id: ID! name: String! price: Float! }
To query for products with a specific price, you can pass the price as an argument in the query:
query { productsByPrice(price: 100.0) { id name } }
In this example, we are querying for products that have a price of 100.0. The price value is passed as the argument to the “productsByPrice” field.
What are the best practices for working with enums in GraphQL?
When working with enums in GraphQL, there are several best practices you can follow to ensure a clean and maintainable schema:
1. Use descriptive and meaningful enum values: Choose enum values that accurately describe the options they represent. This makes the schema more self-explanatory and easier to understand for both clients and developers.
2. Define enums close to the fields that use them: Place enum definitions near the fields or arguments that use them. This improves readability and makes it easier to understand the context in which the enum is used.
3. Use enums for mutually exclusive options: Enums are useful for representing a set of mutually exclusive options. If a field or argument allows multiple options to be selected, consider using a list or another appropriate type instead.
4. Avoid using string literals: Instead of using string literals directly in your queries, use the corresponding enum values. This helps in avoiding typos and ensures that only valid enum values are used.
5. Handle unknown enum values gracefully: When working with enum values, handle unknown or unexpected values gracefully in your resolvers. Instead of returning an error, consider providing a default or fallback value.
6. Document your enums: Provide clear documentation for your enums to explain the possible values and their meanings. This helps clients understand the available options and how they should be used.
7. Consider versioning enums: If you anticipate changes to the enum values in the future, consider versioning your enums to maintain backward compatibility with existing clients. This can be done by introducing new enum values while keeping the old ones intact.
Related Article: Managing Data Queries with GraphQL Count