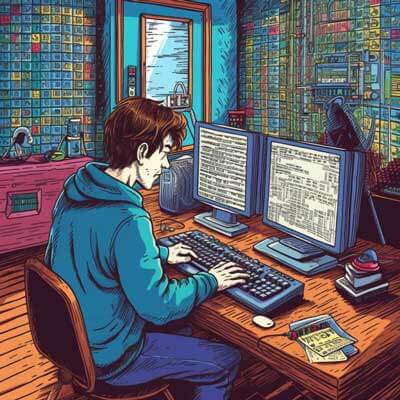
- How does GraphQL work?
- What is the purpose of sorting by date in GraphQL?
- How to sort data in GraphQL by date?
- What are the benefits of using GraphQL for sorting data?
- Can I use GraphQL to sort data in descending order?
- How to implement pagination with sorted data in GraphQL?
- What are the common pagination techniques in GraphQL?
- How to filter data before sorting in GraphQL?
- What are the best practices for sorting data in GraphQL?
- Are there any limitations when sorting data in GraphQL?
- Additional Resources
How does GraphQL work?
GraphQL is an open-source query language for APIs that was developed by Facebook. It provides a more efficient and flexible way to request and manipulate data from server-side APIs. Unlike traditional REST APIs, GraphQL allows clients to specify exactly what data they need for a particular request, reducing over-fetching and under-fetching of data.
At its core, GraphQL operates on a schema that defines the types of data that can be queried and the structure of the queries themselves. Clients can send queries to the GraphQL server specifying the fields they want to retrieve, and the server will respond with the requested data in a JSON format.
For example, let’s say we have a GraphQL schema that defines a “User” type with fields like “id”, “name”, and “email”. A client can send a query like this to retrieve the name and email of a specific user:
query { user(id: 123) { name email } }
The server will then respond with a JSON object containing the requested data:
{ "data": { "user": { "name": "John Doe", "email": "john@example.com" } } }
This way, the client has full control over the data it receives, eliminating the need for multiple round-trips to fetch related data and reducing unnecessary data transfer.
Related Article: Achieving Production-Ready GraphQL
What is the purpose of sorting by date in GraphQL?
Sorting data by date is a common requirement in many applications, especially when dealing with time-sensitive data such as events, blog posts, or social media posts. In GraphQL, sorting data by date allows clients to retrieve data in a specific order based on the date or time of creation or modification.
For example, let’s say we have a GraphQL schema that defines a “Post” type with a “createdAt” field representing the date and time the post was created. Clients can include a sorting argument in their queries to specify the desired order:
query { posts(sortBy: CREATED_AT_DESC) { title createdAt } }
The server will then respond with the posts sorted in descending order based on the “createdAt” field:
{ "data": { "posts": [ { "title": "Post 3", "createdAt": "2022-01-03T15:30:00Z" }, { "title": "Post 2", "createdAt": "2022-01-02T10:45:00Z" }, { "title": "Post 1", "createdAt": "2022-01-01T08:00:00Z" } ] } }
This allows clients to easily display the posts in the desired order, such as in a timeline or news feed.
How to sort data in GraphQL by date?
To sort data in GraphQL by date, you need to define a sorting argument in your GraphQL schema and implement the sorting logic in your GraphQL server.
First, let’s start by defining the sorting argument in the schema. Assuming we have a “Post” type with a “createdAt” field representing the date and time the post was created, we can add a “sortBy” argument to the field that accepts an enum type representing the sorting options:
enum SortBy { CREATED_AT_ASC CREATED_AT_DESC } type Post { title: String! createdAt: DateTime! } type Query { posts(sortBy: SortBy): [Post!]! }
In this example, the “SortBy” enum type defines two sorting options: “CREATED_AT_ASC” for ascending order and “CREATED_AT_DESC” for descending order.
Next, we need to implement the sorting logic in the resolver function for the “posts” field in the “Query” type. The resolver function will receive the “sortBy” argument and use it to sort the posts accordingly. Here’s an example implementation using JavaScript:
const resolvers = { Query: { posts: (_, { sortBy }) => { // Retrieve the posts from the database or any other data source let posts = [ { title: "Post 1", createdAt: new Date("2022-01-01T08:00:00Z") }, { title: "Post 2", createdAt: new Date("2022-01-02T10:45:00Z") }, { title: "Post 3", createdAt: new Date("2022-01-03T15:30:00Z") } ]; // Sort the posts based on the sortBy argument if (sortBy === "CREATED_AT_ASC") { posts.sort((a, b) => a.createdAt - b.createdAt); } else if (sortBy === "CREATED_AT_DESC") { posts.sort((a, b) => b.createdAt - a.createdAt); } return posts; } } };
In this example, the resolver function receives the “sortBy” argument and sorts the posts array based on the sorting option. The sorted posts are then returned to the client.
Clients can now include the “sortBy” argument in their queries to specify the desired sorting order:
query { posts(sortBy: CREATED_AT_DESC) { title createdAt } }
The server will respond with the posts sorted in descending order based on the “createdAt” field.
What are the benefits of using GraphQL for sorting data?
Using GraphQL for sorting data provides several benefits compared to traditional REST APIs:
1. Efficiency: GraphQL allows clients to request only the data they need, reducing over-fetching and under-fetching of data. Clients can specify the sorting criteria in their queries, ensuring that they receive the sorted data without unnecessary fields or additional requests.
2. Flexibility: GraphQL provides clients with the flexibility to sort data based on various criteria, including date, time, or any other custom fields. The sorting options can be defined in the GraphQL schema, making it easy for clients to understand and use.
3. Control: GraphQL gives clients full control over the data they receive. Clients can specify the sorting order, filter criteria, and pagination options in their queries, allowing them to retrieve the exact data they need in the desired format.
4. Simplicity: GraphQL simplifies the process of sorting data by providing a standardized way to specify sorting options in queries. Clients don’t need to construct complex query parameters or handle different sorting conventions for different endpoints.
Overall, using GraphQL for sorting data enhances the developer experience by providing a more efficient and flexible way to retrieve and manipulate data.
Related Article: AEM GraphQL: A Critical Component in Modern Programming
Can I use GraphQL to sort data in descending order?
Yes, you can use GraphQL to sort data in descending order. In GraphQL, sorting options can be defined in the schema and implemented in the resolver functions.
To enable sorting in descending order, you can define a sorting option in the schema that represents descending order. For example, you can add a “CREATED_AT_DESC” option to the “SortBy” enum type:
enum SortBy { CREATED_AT_ASC CREATED_AT_DESC }
In the resolver function for the field that supports sorting, you can check the sorting option and sort the data accordingly. Here’s an example implementation in JavaScript:
const resolvers = { Query: { posts: (_, { sortBy }) => { let posts = [ { title: "Post 1", createdAt: new Date("2022-01-01T08:00:00Z") }, { title: "Post 2", createdAt: new Date("2022-01-02T10:45:00Z") }, { title: "Post 3", createdAt: new Date("2022-01-03T15:30:00Z") } ]; if (sortBy === "CREATED_AT_ASC") { posts.sort((a, b) => a.createdAt - b.createdAt); } else if (sortBy === "CREATED_AT_DESC") { posts.sort((a, b) => b.createdAt - a.createdAt); } return posts; } } };
In this example, the resolver function checks the value of the “sortBy” argument and sorts the “posts” array in descending order if the value is “CREATED_AT_DESC”.
Clients can include the “sortBy” argument in their queries to specify the desired sorting order:
query { posts(sortBy: CREATED_AT_DESC) { title createdAt } }
The server will respond with the posts sorted in descending order based on the “createdAt” field.
How to implement pagination with sorted data in GraphQL?
Pagination is a common technique used to limit the amount of data returned in a single response and provide a way to retrieve additional data in subsequent requests. When implementing pagination with sorted data in GraphQL, you need to consider the following:
1. Cursor-based pagination: Cursor-based pagination is a technique where a unique identifier (cursor) is used to mark the position of the last item in a list. Clients can then provide this cursor in subsequent requests to retrieve the next or previous set of items. When sorting data, the cursor should be based on the sorting criteria to ensure consistency.
2. Limit and offset: Another approach to pagination is to use the limit and offset parameters. The limit parameter specifies the maximum number of items to return, and the offset parameter specifies the number of items to skip. By combining these parameters with sorting options, clients can retrieve specific subsets of the sorted data.
Let’s see an example implementation of cursor-based pagination with sorted data in GraphQL using JavaScript:
const resolvers = { Query: { posts: (_, { sortBy, cursor, limit }) => { let posts = [ { id: 1, title: "Post 1", createdAt: new Date("2022-01-01T08:00:00Z") }, { id: 2, title: "Post 2", createdAt: new Date("2022-01-02T10:45:00Z") }, { id: 3, title: "Post 3", createdAt: new Date("2022-01-03T15:30:00Z") } ]; if (sortBy === "CREATED_AT_ASC") { posts.sort((a, b) => a.createdAt - b.createdAt); } else if (sortBy === "CREATED_AT_DESC") { posts.sort((a, b) => b.createdAt - a.createdAt); } // Find the index of the last item based on the cursor const lastIndex = posts.findIndex(post => post.id === cursor); // Slice the array based on the lastIndex and limit const slicedPosts = lastIndex !== -1 ? posts.slice(lastIndex + 1, lastIndex + 1 + limit) : []; // Create the next cursor const nextCursor = slicedPosts.length > 0 ? slicedPosts[slicedPosts.length - 1].id : null; // Return the sliced posts and the next cursor return { posts: slicedPosts, nextCursor }; } } };
In this example, the resolver function receives the “sortBy”, “cursor”, and “limit” arguments. It sorts the “posts” array based on the sorting option, finds the index of the last item based on the cursor, slices the array to retrieve the desired subset, and creates the next cursor based on the last item in the subset.
The resolver function returns an object that contains the sliced posts and the next cursor:
{ posts: [ { id: 2, title: "Post 2", createdAt: "2022-01-02T10:45:00Z" }, { id: 3, title: "Post 3", createdAt: "2022-01-03T15:30:00Z" } ], nextCursor: 3 }
Clients can include the “sortBy”, “cursor”, and “limit” arguments in their queries to retrieve specific subsets of the sorted data:
query { posts(sortBy: CREATED_AT_DESC, cursor: 1, limit: 2) { posts { id title createdAt } nextCursor } }
The server will respond with the sliced posts and the next cursor.
What are the common pagination techniques in GraphQL?
When implementing pagination in GraphQL, there are several common techniques that can be used:
1. Cursor-based pagination: Cursor-based pagination is a technique where a unique identifier (cursor) is used to mark the position of the last item in a list. Clients can then provide this cursor in subsequent requests to retrieve the next or previous set of items. This technique provides a consistent and efficient way to paginate through sorted data.
2. Limit and offset: Limit and offset pagination is a technique where the client specifies the maximum number of items to return (limit) and the number of items to skip (offset). This allows clients to retrieve specific subsets of the data. However, this technique can suffer from performance issues when dealing with large offsets.
3. Relay-style pagination: Relay-style pagination is a pagination technique inspired by Facebook’s Relay framework. It involves using a combination of cursors, edges, and nodes to represent a paginated list. The edges contain the cursor and the node, while the nodes represent the actual data items. This technique provides a standardized way to paginate data and is commonly used in GraphQL implementations that follow the Relay specification.
4. Offset-based pagination: Offset-based pagination is a technique where the client specifies the starting index (offset) and the number of items to return (limit). This technique is simpler to implement but can suffer from performance issues when dealing with large offsets, similar to the limit and offset technique.
The choice of pagination technique depends on the specific requirements of the application and the characteristics of the data being paginated. Cursor-based pagination is generally recommended for sorting data, as it provides a consistent and efficient way to paginate through the sorted data.
Related Article: Exploring Default Values in GraphQL Programming
How to filter data before sorting in GraphQL?
Filtering data before sorting is a common requirement in many applications. In GraphQL, you can filter data before sorting by including a filtering argument in your queries and implementing the filtering logic in your resolver functions.
Let’s take an example where we have a GraphQL schema that defines a “Post” type with fields like “title”, “createdAt”, and “author”. Clients can include a filtering argument in their queries to specify the criteria for filtering:
type Post { title: String! createdAt: DateTime! author: String! } type Query { posts(filterByAuthor: String): [Post!]! }
In this example, the “posts” field in the “Query” type accepts a “filterByAuthor” argument that specifies the author name for filtering.
In the resolver function for the “posts” field, you can implement the filtering logic by checking the value of the “filterByAuthor” argument. Here’s an example implementation using JavaScript:
const resolvers = { Query: { posts: (_, { filterByAuthor }) => { let posts = [ { title: "Post 1", createdAt: new Date("2022-01-01T08:00:00Z"), author: "John Doe" }, { title: "Post 2", createdAt: new Date("2022-01-02T10:45:00Z"), author: "Jane Smith" }, { title: "Post 3", createdAt: new Date("2022-01-03T15:30:00Z"), author: "John Doe" } ]; // Filter the posts based on the filterByAuthor argument if (filterByAuthor) { posts = posts.filter(post => post.author === filterByAuthor); } // Sort the posts based on the createdAt field posts.sort((a, b) => a.createdAt - b.createdAt); return posts; } } };
In this example, the resolver function checks the value of the “filterByAuthor” argument and filters the “posts” array based on the author name if the argument is provided. The filtered posts are then sorted based on the “createdAt” field.
Clients can include the “filterByAuthor” argument in their queries to specify the desired filtering criteria:
query { posts(filterByAuthor: "John Doe") { title createdAt } }
The server will respond with the posts filtered by the author name and sorted based on the “createdAt” field.
What are the best practices for sorting data in GraphQL?
When sorting data in GraphQL, it’s important to follow best practices to ensure efficient and consistent behavior. Here are some best practices for sorting data in GraphQL:
1. Define sorting options in the schema: To provide a clear and consistent API, define the sorting options in the GraphQL schema using enum types. This allows clients to easily understand and use the available sorting options.
2. Implement the sorting logic in resolver functions: Implement the sorting logic in the resolver functions of the fields that support sorting. This ensures that the sorting is done on the server side and provides a consistent behavior across different clients.
3. Use cursor-based pagination for sorted lists: When paginating through sorted lists, use cursor-based pagination instead of limit and offset. Cursor-based pagination provides a consistent and efficient way to paginate through the sorted data and avoids performance issues with large offsets.
4. Consider performance implications: Sorting large datasets can have performance implications, especially when combined with filtering and pagination. Consider implementing efficient sorting algorithms and optimizing your data retrieval and processing logic to ensure good performance.
5. Document the available sorting options: Document the available sorting options in your GraphQL API documentation to help clients understand and use the sorting functionality. Provide clear examples and explanations of how to use the sorting options in queries.
6. Test the sorting functionality: Test the sorting functionality thoroughly to ensure that it works as expected and provides consistent results. Write unit tests and integration tests to cover different sorting scenarios and edge cases.
Are there any limitations when sorting data in GraphQL?
While GraphQL provides useful capabilities for sorting data, there are some limitations to be aware of:
1. Backend implementation: Sorting data in GraphQL relies on the backend implementation. If the backend data source does not natively support sorting or has limitations on the sorting capabilities, it may impact the sorting functionality in GraphQL.
2. Performance considerations: Sorting large datasets can have performance implications, especially when combined with filtering and pagination. Sorting requires processing and comparing the values of the sorting fields, which can become expensive for large datasets. Consider implementing efficient sorting algorithms and optimizing your data retrieval and processing logic to ensure good performance.
3. Complexity: Sorting data in GraphQL can introduce complexity, especially when dealing with nested fields or relationships. Sorting nested fields or relationships may require additional resolver functions and database queries to retrieve and sort the related data.
4. Over-fetching and under-fetching: When sorting data in GraphQL, it’s important to consider the balance between over-fetching and under-fetching of data. Sorting large datasets can result in over-fetching of data if the client only needs a subset of the sorted data. On the other hand, under-fetching of data can occur if the client needs additional related data for each sorted item.
5. Schema changes: If the sorting options or the sorting fields change in the GraphQL schema, it may impact the client applications that rely on the sorting functionality. It’s important to carefully plan and communicate any changes to the sorting functionality to ensure a smooth transition for client applications.
Related Article: Exploring Directus GraphQL
Additional Resources
– Sorting in GraphQL
– Sorting and Pagination in GraphQL
– Best Practices for Sorting and Filtering in GraphQL