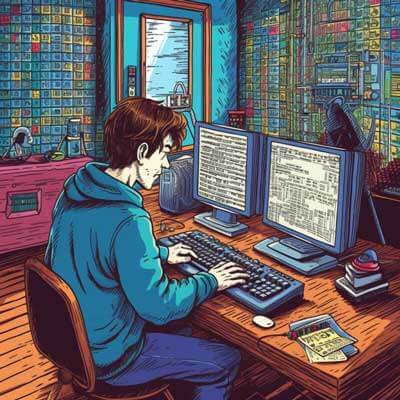
Table of Contents
When working with PHP and MySQL, it's common to encounter situations where queries need to be executed within a loop. However, performing repetitive database queries in a loop can have a negative impact on performance, leading to slower execution times and increased resource usage. In this article, we will explore various techniques and best practices to help you avoid redundant MySQL queries in PHP loops.
Technique 1: Query Caching and its Impact on Performance
One of the most common techniques to prevent redundant MySQL queries in PHP loops is by utilizing query caching. Query caching allows the database server to store the results of a query in memory, making subsequent identical queries faster by retrieving the results from the cache instead of executing the query again.
To enable query caching in MySQL, you can modify the my.cnf
configuration file and set the query_cache_size
parameter to an appropriate value. Additionally, you can use the SQL_CACHE
keyword in your queries to explicitly instruct MySQL to cache the results.
Here's an example of how to enable query caching in MySQL and utilize it in PHP:
// Enable query caching in MySQL SET GLOBAL query_cache_size = 268435456; // Execute a query with query caching in PHP $query = "SELECT * FROM users WHERE active = 1 SQL_CACHE"; $result = mysqli_query($connection, $query);
Related Article: How to Work with PHP Arrays: a Complete Guide
Technique 2: Understanding Database Indexing for Efficient Queries
Another technique to avoid repetitive MySQL queries in PHP loops is to optimize your database by using appropriate indexes. Indexes are data structures that improve the speed of data retrieval operations on database tables. By creating indexes on frequently queried columns, you can speed up the execution of queries and reduce the need for redundant queries within loops.
To create an index in MySQL, you can use the CREATE INDEX
statement. For example, if you frequently query a users
table based on the email
column, you can create an index on that column as follows:
CREATE INDEX idx_email ON users (email);
Technique 3: Leveraging ORM to Avoid Repetitive MySQL Queries in PHP
Object-Relational Mapping (ORM) libraries, such as Doctrine and Eloquent, provide useful abstractions for database operations in PHP. These libraries allow you to map database tables to PHP objects, providing a convenient and intuitive way to interact with the database.
Here's an example of using the Eloquent ORM to avoid redundant MySQL queries in PHP loops:
// Define a User model with a relationship to Posts class User extends \Illuminate\Database\Eloquent\Model { public function posts() { return $this->hasMany('Post'); } } // Retrieve users and their posts using Eloquent $users = User::with('posts')->get(); foreach ($users as $user) { // Access posts without additional queries foreach ($user->posts as $post) { // Process posts } }
Technique 4: Utilizing Stored Procedures to Optimize MySQL Queries
Stored Procedures are precompiled SQL statements stored in the database. They can be called from PHP, reducing the need for repetitive MySQL queries within loops. Stored Procedures offer several benefits, including improved performance, code reusability, and enhanced security.
To create a stored procedure in MySQL, you can use the CREATE PROCEDURE
statement. Here's an example of a stored procedure that retrieves all active users:
DELIMITER // CREATE PROCEDURE GetActiveUsers() BEGIN SELECT * FROM users WHERE active = 1; END // DELIMITER ;
Once the stored procedure is created, you can call it from PHP using the appropriate MySQL extension. Here's an example using the mysqli extension:
// Call the GetActiveUsers stored procedure $query = "CALL GetActiveUsers()"; $result = mysqli_query($connection, $query);
Related Article: How to Use PHP Curl for HTTP Post
Technique 5: Benefits of Prepared Statements in PHP for Reducing Query Redundancy
Prepared statements are a feature of PHP's database extensions that allow you to prepare a query once and execute it multiple times with different parameter values. Prepared statements offer several benefits, including improved performance, increased security, and prevention of SQL injection attacks.
Here's an example of using prepared statements in PHP to avoid redundant MySQL queries in a loop:
// Prepare a statement $query = "SELECT * FROM users WHERE id = ?"; $stmt = mysqli_prepare($connection, $query); // Bind parameters and execute the statement in a loop foreach ($ids as $id) { mysqli_stmt_bind_param($stmt, "i", $id); mysqli_stmt_execute($stmt); // Process the result set $result = mysqli_stmt_get_result($stmt); while ($row = mysqli_fetch_assoc($result)) { // Process the row } } // Clean up the statement mysqli_stmt_close($stmt);
Technique 6: Exploring Database Connection Pooling to Improve Query Efficiency
Database connection pooling is a technique that allows you to reuse existing database connections instead of creating a new connection for each query. This can significantly improve query efficiency by reducing the overhead of establishing and tearing down connections.
There are various connection pooling libraries available for PHP, such as PDO and MySQLi. These libraries provide built-in support for connection pooling, allowing you to configure and manage connection pools efficiently.
Here's an example of using PDO connection pooling to improve query efficiency in PHP:
// Create a PDO connection pool $pdoOptions = [ PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION, PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC, PDO::ATTR_PERSISTENT => true, PDO::ATTR_EMULATE_PREPARES => false, ]; $pdoDsn = 'mysql:host=localhost;dbname=mydatabase'; $pdoUsername = 'myusername'; $pdoPassword = 'mypassword'; $pdoPool = new Pool(function () use ($pdoDsn, $pdoUsername, $pdoPassword, $pdoOptions) { return new PDO($pdoDsn, $pdoUsername, $pdoPassword, $pdoOptions); }); // Execute queries using the connection pool foreach ($ids as $id) { $pdoPool->run(function (PDO $pdo) use ($id) { $stmt = $pdo->prepare("SELECT * FROM users WHERE id = :id"); $stmt->execute(['id' => $id]); // Process the result set while ($row = $stmt->fetch()) { // Process the row } }); }
Technique 7: Lazy Loading and its Role in Reducing Repetitive MySQL Queries
Lazy loading is a technique where related data is loaded on-demand, rather than upfront. This can help reduce the number of redundant MySQL queries in PHP loops by loading only the necessary data when it is actually needed.
ORM libraries often provide lazy loading capabilities, allowing you to specify relationships between objects and load related data as required. By utilizing lazy loading, you can optimize the execution of MySQL queries in PHP loops and avoid unnecessary queries for data that is not needed.
Here's an example of using lazy loading in PHP with the Doctrine ORM:
// Define a User entity with a lazy-loaded relationship to Posts class User { // ... /** * @ORM\OneToMany(targetEntity="Post", mappedBy="user") */ private $posts; public function getPosts() { if ($this->posts === null) { $this->posts = $this->entityManager->getRepository(Post::class)->findBy(['user' => $this]); } return $this->posts; } // ... } // Retrieve users and their posts using Doctrine $users = $entityManager->getRepository(User::class)->findAll(); foreach ($users as $user) { // Lazy load posts only when needed foreach ($user->getPosts() as $post) { // Process posts } }
Technique 8: The Importance of Database Normalization in Preventing Query Redundancy
Database normalization is an essential process in database design that helps reduce data redundancy and improve data integrity. By organizing data into separate tables and establishing relationships between them, you can minimize the need for redundant MySQL queries in PHP loops.
When designing your database schema, you should aim for at least the third normal form (3NF). This involves eliminating repeating groups, breaking down data into smaller tables, and establishing relationships between them using foreign keys.
Related Article: How to Use Laravel Folio: Tutorial with Advanced Examples
Tips for Optimizing MySQL Queries: Best Practices and Recommendations
In addition to the techniques discussed above, here are some general tips and best practices for optimizing MySQL queries in PHP loops:
1. Minimize the number of queries: Try to combine multiple queries into a single query whenever possible, reducing the overhead of executing multiple queries in a loop.
2. Use appropriate indexes: Analyze your query patterns and create indexes on frequently queried columns to speed up query execution.
3. Use LIMIT and OFFSET: If you need to retrieve a subset of data from a large result set, use the LIMIT and OFFSET clauses to limit the number of rows returned.
4. Avoid unnecessary data retrieval: Only retrieve the data that you actually need in your PHP loop, rather than fetching all columns from the database.
5. Use efficient data structures: Consider using associative arrays or objects instead of indexed arrays when processing query results, as they provide faster access to data.
6. Optimize loop logic: Review your loop logic and ensure that it is efficient and optimized. Avoid unnecessary iterations and redundant calculations within the loop.
7. Monitor and analyze query performance: Use tools like the MySQL EXPLAIN statement and query profiling to identify performance bottlenecks and optimize your queries accordingly.
Additional Resources
- How to avoid repetitive MySQL queries in a loop in PHP
- Libraries and frameworks to avoid repetitive MySQL queries in PHP loops
- Using prepared statements in PHP to avoid repetitive MySQL queries in a loop