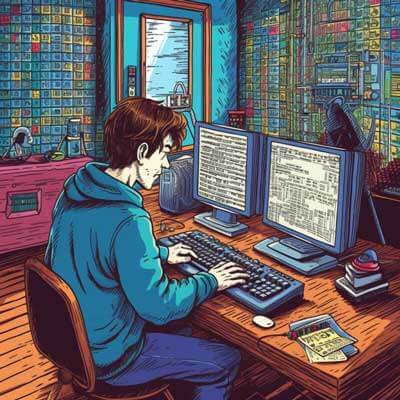
Table of Contents
Project Structuring in Gin
When developing a Gin application, it's crucial to have a well-structured project layout that promotes maintainability and scalability. Here, we'll explore some best practices for structuring a Gin project.
Related Article: Optimizing and Benchmarking Beego ORM in Golang
Separation of Concerns
To keep your codebase organized and maintainable, it's important to separate concerns and follow the Single Responsibility Principle (SRP). This means dividing your code into logical components such as routers, controllers, models, and middleware.
Here's an example project structure for a Gin application:
. ├── cmd │ └── main.go ├── internal │ ├── controllers │ │ └── user_controller.go │ ├── middleware │ │ └── auth_middleware.go │ ├── models │ │ └── user.go │ └── routers │ └── router.go ├── pkg │ └── utils │ └── validation.go └── config.go
In this structure, the cmd
directory contains the main entry point of the application. The internal
directory holds the core implementation of the application, including controllers, models, middleware, and routers. The pkg
directory contains reusable packages or utilities that can be shared across different projects.
Organizing Routes
Gin provides a flexible router that allows you to define routes and handle different HTTP methods. To organize your routes effectively, consider creating a dedicated router file where you define all the routes for your application.
Here's an example of how you can organize your routes in a Gin application:
// router.go package routers import ( "github.com/gin-gonic/gin" "github.com/myapp/controllers" ) func SetupRouter() *gin.Engine { router := gin.Default() // Public routes public := router.Group("/api") { public.POST("/register", controllers.Register) public.POST("/login", controllers.Login) } // Protected routes protected := router.Group("/api") protected.Use(middleware.AuthMiddleware()) { protected.GET("/users", controllers.GetUsers) protected.POST("/users", controllers.CreateUser) protected.GET("/users/:id", controllers.GetUserByID) protected.PUT("/users/:id", controllers.UpdateUser) protected.DELETE("/users/:id", controllers.DeleteUser) } return router }
In this example, we define two groups of routes: public and protected. The public routes are accessible without authentication, while the protected routes require authentication using the AuthMiddleware
we defined.
Organizing your routes in a separate file not only makes it easier to manage and understand the routing logic but also allows for better code reuse and modularity.
Error Handling in Gin
Error handling is an essential aspect of any application, and Gin provides robust mechanisms for handling errors gracefully. Let's explore some best practices for error handling in Gin.
Related Article: Building Gin Backends for React.js and Vue.js
Custom Error Responses
When an error occurs in your application, it's important to provide meaningful error responses to the client. Gin allows you to define custom error responses using middleware and the AbortWithStatusJSON
function.
Here's an example of how you can define custom error responses in Gin:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.Use(ErrorHandlerMiddleware()) router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") // Simulating an error if id == "0" { c.AbortWithStatusJSON(http.StatusBadRequest, gin.H{"error": "Invalid user ID"}) return } // Process user request c.JSON(http.StatusOK, gin.H{"message": "User found"}) }) router.Run(":8080") } func ErrorHandlerMiddleware() gin.HandlerFunc { return func(c *gin.Context) { c.Next() if len(c.Errors) > 0 { err := c.Errors.Last() c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) } } }
In this example, we define a custom error handler middleware that checks for errors after each request. If an error is present, we abort the request and return a JSON response with the error message.
Panic Recovery
Gin provides built-in middleware for recovering from panics, ensuring that your application remains stable even in the face of unexpected errors. By using the gin.Recovery
middleware, you can recover from panics and return a proper error response to the client.
Here's an example of how to use the panic recovery middleware in Gin:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.Use(gin.Recovery()) router.GET("/panic", func(c *gin.Context) { panic("Something went wrong!") }) router.Run(":8080") }
In this example, we intentionally induce a panic by calling the panic
function inside a route handler. However, thanks to the recovery middleware, the panic is caught, and Gin returns a proper error response instead of crashing the application.
Testing in Gin
Testing is a critical aspect of software development, ensuring that your application behaves as expected and minimizing the risk of introducing bugs. In this section, we'll explore strategies for testing Gin applications effectively.
Unit Testing Controllers
When testing a Gin application, it's important to focus on the individual components that make up your application, such as controllers. Unit testing controllers allows you to verify that they handle requests correctly and produce the expected responses.
Here's an example of how you can unit test a controller in Gin:
// user_controller_test.go package controllers_test import ( "net/http" "net/http/httptest" "testing" "github.com/gin-gonic/gin" "github.com/myapp/controllers" "github.com/stretchr/testify/assert" ) func TestGetUserByID(t *testing.T) { // Setup router := gin.Default() router.GET("/users/:id", controllers.GetUserByID) // Create a test request req, err := http.NewRequest("GET", "/users/123", nil) assert.NoError(t, err) // Perform the request rec := httptest.NewRecorder() router.ServeHTTP(rec, req) // Assert the response assert.Equal(t, http.StatusOK, rec.Code) assert.Equal(t, "{\"message\":\"User found\"}", rec.Body.String()) }
In this example, we create a test request using http.NewRequest
and pass it to the router using router.ServeHTTP
. We then assert the response code and body to ensure that the controller produces the expected output.
Related Article: Best Practices for Building Web Apps with Beego & Golang
Integration Testing with HTTP Client
In addition to unit testing individual components, it's important to perform integration testing to verify that different parts of your application work together correctly. Integration testing allows you to test the entire request-response cycle, including middleware and routing.
Here's an example of how you can perform integration testing in Gin using an HTTP client:
// main_test.go package main_test import ( "net/http" "testing" "github.com/gin-gonic/gin" "github.com/myapp/controllers" "github.com/stretchr/testify/assert" ) func TestGetUserByID(t *testing.T) { // Setup router := gin.Default() router.GET("/users/:id", controllers.GetUserByID) // Start the server in a separate goroutine go func() { err := router.Run(":8080") assert.NoError(t, err) }() // Perform the request resp, err := http.Get("http://localhost:8080/users/123") assert.NoError(t, err) defer resp.Body.Close() // Assert the response assert.Equal(t, http.StatusOK, resp.StatusCode) // Additional assertions... }
In this example, we start the Gin server in a separate goroutine using router.Run
and then perform an HTTP request to the running server. We can then assert the response status code, body, and any other relevant data.
Middleware in Gin
Middleware plays a crucial role in Gin applications, allowing you to add functionality that runs before or after request handlers. In this section, we'll explore some common use cases for middleware in Gin and how to implement them effectively.
Authentication Middleware
Authentication is a common requirement for many web applications, and Gin makes it easy to implement authentication middleware. With authentication middleware, you can protect certain routes from unauthorized access and ensure that only authenticated users can access them.
Here's an example of how you can implement authentication middleware in Gin:
// auth_middleware.go package middleware import ( "net/http" "github.com/gin-gonic/gin" ) func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Check if the user is authenticated if isAuthenticated(c) { c.Next() return } // User is not authenticated, return an error response c.AbortWithStatusJSON(http.StatusUnauthorized, gin.H{"error": "Unauthorized"}) } } func isAuthenticated(c *gin.Context) bool { // Check if the user is authenticated based on a JWT token, session, or any other mechanism // Return true if the user is authenticated, false otherwise }
In this example, we define an authentication middleware function that checks if the user is authenticated. If the user is authenticated, the middleware calls c.Next()
to pass control to the next middleware or route handler. Otherwise, it aborts the request and returns an unauthorized error response.
Logging Middleware
Logging is an essential aspect of any application, providing valuable insights into the behavior and performance of your code. Gin allows you to add logging middleware to log requests and responses, making it easier to debug issues and monitor your application.
Here's an example of how you can implement logging middleware in Gin:
// logging_middleware.go package middleware import ( "log" "time" "github.com/gin-gonic/gin" ) func LoggingMiddleware() gin.HandlerFunc { return func(c *gin.Context) { start := time.Now() c.Next() end := time.Now() latency := end.Sub(start) log.Printf("[%s] %s %s %v", c.Request.Method, c.Request.URL.Path, c.ClientIP(), latency) } }
In this example, we define a logging middleware that measures the time it takes to process a request and logs the request method, URL path, client IP, and request latency.
Related Article: Handling Large Volumes of Data with Golang & Gin
RESTful API Development in Gin
Gin is well-suited for developing RESTful APIs, providing a simple and efficient way to define routes, handle requests, and return JSON responses. In this section, we'll explore some best practices for developing RESTful APIs using Gin.
Resource Naming
When designing a RESTful API, it's important to choose appropriate resource names that accurately represent the entities in your application. Resource names should be nouns and use lowercase letters with hyphens separating multiple words.
Here are some examples of well-named resources in a RESTful API:
- /users
- /products
- /orders
HTTP Methods and Routes
RESTful APIs use HTTP methods to perform different actions on resources. When designing your API, it's important to map HTTP methods to appropriate routes that correspond to the desired actions.
Here's an example of how you can define routes for different HTTP methods in a Gin application:
// main.go package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users", getUsers) router.POST("/users", createUser) router.GET("/users/:id", getUserByID) router.PUT("/users/:id", updateUser) router.DELETE("/users/:id", deleteUser) router.Run(":8080") } func getUsers(c *gin.Context) { // Retrieve and return all users } func createUser(c *gin.Context) { // Create a new user } func getUserByID(c *gin.Context) { // Retrieve and return a user by ID } func updateUser(c *gin.Context) { // Update a user by ID } func deleteUser(c *gin.Context) { // Delete a user by ID }
In this example, we define routes for different HTTP methods (GET, POST, PUT, DELETE) that correspond to different actions on the /users
resource.
Concurrency in Gin
Concurrency is an important consideration in modern web applications, allowing you to handle multiple requests simultaneously and improve performance. In this section, we'll explore how Gin handles concurrency and some best practices for concurrent programming in Gin.
Related Article: Golang Tutorial for Backend Development
Goroutines and Concurrency
Gin is built on top of the Go programming language, which has excellent support for concurrency through goroutines. Goroutines are lightweight threads of execution that can run concurrently, allowing you to handle multiple requests simultaneously.
Here's an example of how you can use goroutines to handle concurrent requests in Gin:
// main.go package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users", handleUsersRequest) router.Run(":8080") } func handleUsersRequest(c *gin.Context) { // Retrieve users from the database concurrently usersCh := make(chan []User) errorsCh := make(chan error) go func() { users, err := getUsersFromDB() if err != nil { errorsCh <- err return } usersCh <- users }() select { case users := <-usersCh: c.JSON(http.StatusOK, users) case err := <-errorsCh: c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) } } func getUsersFromDB() ([]User, error) { // Simulate fetching users from the database }
In this example, we use a goroutine to fetch users from the database concurrently. We create two channels, usersCh
and errorsCh
, to receive the results and errors from the goroutine. We then use a select
statement to wait for either the users or an error, depending on which finishes first.
Managing Shared State
When working with concurrent code, it's important to handle shared state correctly to avoid race conditions and ensure data integrity. In Gin applications, shared state can include databases, caches, or other external resources.
Here are some best practices for managing shared state in Gin:
- Use appropriate synchronization mechanisms such as locks or mutexes to protect shared resources from concurrent access.
- Avoid relying on global variables or shared mutable state whenever possible, as they can introduce hard-to-debug race conditions.
- Consider using connection pooling or connection limits to manage database connections and prevent resource exhaustion.
- Use atomic operations or synchronization primitives provided by the Go standard library to perform thread-safe operations on shared variables.
Database Integration with Gin
Gin provides a flexible and extensible framework for integrating databases into your application. In this section, we'll explore how to integrate databases with Gin and some best practices for working with databases effectively.
Connecting to a Database
To connect to a database in a Gin application, you can use a database driver or ORM of your choice. Gin doesn't provide a built-in database integration, but it works seamlessly with popular libraries like GORM, SQLx, or the standard library's database/sql
package.
Here's an example of how you can connect to a PostgreSQL database using the database/sql
package in a Gin application:
// main.go package main import ( "database/sql" "log" "github.com/gin-gonic/gin" _ "github.com/lib/pq" ) func main() { db, err := sql.Open("postgres", "user=postgres password=postgres dbname=mydb sslmode=disable") if err != nil { log.Fatal(err) } router := gin.Default() router.GET("/users", func(c *gin.Context) { // Query users from the database rows, err := db.Query("SELECT * FROM users") if err != nil { c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } defer rows.Close() // Process the rows and return the result // ... }) router.Run(":8080") }
In this example, we use the database/sql
package to connect to a PostgreSQL database and query users from the users
table. The sql.Open
function establishes a connection to the database, and the db.Query
function executes the SQL query.
Related Article: Integrating Beego & Golang Backends with React.js
Database Migrations
As your application evolves, you may need to make changes to your database schema. Database migrations allow you to manage these changes in a structured and version-controlled manner. There are several migration tools available for Go, such as Goose, Golang-migrate, or GORM's built-in migration functionality.
Here's an example of how you can use GORM's migration functionality to perform database migrations in a Gin application:
// main.go package main import ( "log" "github.com/gin-gonic/gin" "gorm.io/driver/postgres" "gorm.io/gorm" ) func main() { db, err := gorm.Open(postgres.Open("user=postgres password=postgres dbname=mydb sslmode=disable"), &gorm.Config{}) if err != nil { log.Fatal(err) } // Perform database migrations err = db.AutoMigrate(&User{}) if err != nil { log.Fatal(err) } router := gin.Default() router.GET("/users", func(c *gin.Context) { // Query users from the database var users []User result := db.Find(&users) if result.Error != nil { c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": result.Error.Error()}) return } // Process the users and return the result // ... }) router.Run(":8080") } type User struct { gorm.Model Name string Email string }
In this example, we use GORM's AutoMigrate
function to automatically perform database migrations based on the defined model structs. We define a User
struct that represents a user entity in the database, and GORM creates the corresponding table and columns.
Logging in Gin
Logging is an essential aspect of any application, providing valuable insights into the behavior and performance of your code. In this section, we'll explore how to implement logging in Gin applications effectively.
Using the Default Logger
Gin provides a default logger that logs information about each request, including the request method, URL path, response status code, and request duration. By default, the logger outputs to the standard output (stdout).
Here's an example of how you can use the default logger in a Gin application:
// main.go package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users", func(c *gin.Context) { // Process the request c.JSON(http.StatusOK, gin.H{"message": "Hello, World!"}) }) router.Run(":8080") }
When you run this application, you should see log output similar to the following:
[GIN] 2022/01/01 - 01:23:45 | 200 | 2.345µs | 127.0.0.1 | GET "/users"
The default logger provides basic information about each request, allowing you to keep track of incoming requests and monitor the performance of your application.
Customizing the Logger
While the default logger is sufficient for many cases, you may need more control over the logging behavior or want to log additional information. Gin allows you to customize the logger by creating a new instance of gin.Logger
and configuring it to your needs.
Here's an example of how you can customize the logger in a Gin application:
// main.go package main import ( "log" "os" "time" "github.com/gin-gonic/gin" ) func main() { // Create a new instance of the logger logger := gin.LoggerWithConfig(gin.LoggerConfig{ Formatter: func(param gin.LogFormatterParams) string { return fmt.Sprintf("[%s] %s %s %d %s\n", param.TimeStamp.Format(time.RFC3339), param.Method, param.Path, param.StatusCode, param.ErrorMessage, ) }, Output: os.Stdout, }) router := gin.New() // Use the custom logger router.Use(logger) router.GET("/users", func(c *gin.Context) { // Process the request c.JSON(http.StatusOK, gin.H{"message": "Hello, World!"}) }) router.Run(":8080") }
In this example, we create a new instance of the logger using gin.LoggerWithConfig
and configure it with a custom log formatter and output destination. The custom log formatter formats the log message to include the timestamp, request method, URL path, response status code, and error message.
Related Article: Implementing Real-time Features with Gin & Golang
Authentication in Gin
Authentication is a fundamental aspect of web applications, ensuring that only authorized users can access certain resources or perform specific actions. In this section, we'll explore how to implement authentication in Gin applications effectively.
Token-based Authentication
Token-based authentication is a popular authentication mechanism used in modern web applications. With token-based authentication, the server generates a token (e.g., JSON Web Token or JWT) after successful authentication, and the client includes this token in subsequent requests to access protected resources.
Here's an example of how you can implement token-based authentication in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" "github.com/dgrijalva/jwt-go" ) func main() { router := gin.Default() router.POST("/login", handleLogin) router.GET("/protected", authenticateMiddleware(), handleProtected) router.Run(":8080") } func handleLogin(c *gin.Context) { // Perform authentication // ... // Generate a JWT token token := jwt.New(jwt.SigningMethodHS256) // Set claims claims := token.Claims.(jwt.MapClaims) claims["sub"] = "example@example.com" claims["exp"] = time.Now().Add(time.Hour * 24).Unix() // Generate encoded token and send it as a response t, err := token.SignedString([]byte("secret")) if err != nil { c.AbortWithStatus(http.StatusInternalServerError) return } c.JSON(http.StatusOK, gin.H{"token": t}) } func authenticateMiddleware() gin.HandlerFunc { return func(c *gin.Context) { tokenString := c.GetHeader("Authorization") // Parse and validate the token token, err := jwt.Parse(tokenString, func(token *jwt.Token) (interface{}, error) { if _, ok := token.Method.(*jwt.SigningMethodHMAC); !ok { return nil, fmt.Errorf("unexpected signing method") } return []byte("secret"), nil }) if err != nil { c.AbortWithStatus(http.StatusUnauthorized) return } // Verify the token if _, ok := token.Claims.(jwt.MapClaims); !ok || !token.Valid { c.AbortWithStatus(http.StatusUnauthorized) return } c.Next() } } func handleProtected(c *gin.Context) { // Protected resource handler // ... }
In this example, we define a /login
route that handles the authentication process. After successful authentication, we generate a JWT token containing the user's claims (e.g., email) and send it as a response to the client.
We also define an authenticateMiddleware
that acts as a middleware for routes that require authentication. This middleware parses and validates the JWT token included in the Authorization
header of the request.
Session-based Authentication
Session-based authentication is another commonly used authentication mechanism. With session-based authentication, the server creates a session for each user after successful authentication and maintains the session state on the server-side. The client includes a session identifier (e.g., a cookie) in subsequent requests to establish their identity.
Here's an example of how you can implement session-based authentication in a Gin application using the github.com/gin-contrib/sessions
middleware:
// main.go package main import ( "net/http" "github.com/gin-contrib/sessions" "github.com/gin-contrib/sessions/cookie" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() store := cookie.NewStore([]byte("secret")) router.Use(sessions.Sessions("mysession", store)) router.POST("/login", handleLogin) router.GET("/protected", authenticateMiddleware(), handleProtected) router.Run(":8080") } func handleLogin(c *gin.Context) { // Perform authentication // ... // Create a session session := sessions.Default(c) session.Set("user", "example@example.com") session.Save() c.Status(http.StatusOK) } func authenticateMiddleware() gin.HandlerFunc { return func(c *gin.Context) { session := sessions.Default(c) user := session.Get("user") if user == nil { c.AbortWithStatus(http.StatusUnauthorized) return } c.Next() } } func handleProtected(c *gin.Context) { // Protected resource handler // ... }
In this example, we use the github.com/gin-contrib/sessions
middleware to handle session-based authentication. We create a session store using the cookie
store implementation and configure it with a secret key. We then use the sessions.Default
function to retrieve the session for each request.
We define a /login
route that handles the authentication process. After successful authentication, we create a session, set a user identifier in the session, and save it.
We also define an authenticateMiddleware
that acts as a middleware for routes that require authentication. This middleware checks if a user identifier exists in the session and aborts the request if it doesn't.
Deployment Strategies for Gin Applications
Deploying a Gin application requires careful consideration of various factors, such as scalability, reliability, and ease of maintenance. In this section, we'll explore different deployment strategies for Gin applications and some best practices to follow.
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
Containerization with Docker
Containerization using Docker is a popular deployment strategy for modern web applications, providing a consistent and reproducible environment that can be easily deployed across different platforms.
Here's an example of how you can containerize a Gin application with Docker:
1. Create a Dockerfile
in the root directory of your Gin application:
# Dockerfile FROM golang:1.17 as build WORKDIR /app COPY go.mod go.sum ./ RUN go mod download COPY . . RUN go build -o main . FROM gcr.io/distroless/base-debian10 COPY --from=build /app/main / EXPOSE 8080 CMD ["/main"]
2. Build the Docker image:
docker build -t myapp .
3. Run the Docker container:
docker run -p 8080:8080 myapp
Orchestration with Kubernetes
Kubernetes is an open-source container orchestration platform that provides advanced features for deploying, scaling, and managing containerized applications. Using Kubernetes, you can easily deploy and manage your Gin application in a highly scalable and resilient manner.
Here's an example of how you can deploy a Gin application on Kubernetes:
1. Create a deployment YAML file (deployment.yaml
) to define the deployment:
# deployment.yaml apiVersion: apps/v1 kind: Deployment metadata: name: myapp spec: replicas: 3 selector: matchLabels: app: myapp template: metadata: labels: app: myapp spec: containers: - name: myapp image: myapp:latest ports: - containerPort: 8080
2. Create a service YAML file (service.yaml
) to expose the deployment:
# service.yaml apiVersion: v1 kind: Service metadata: name: myapp spec: selector: app: myapp ports: - protocol: TCP port: 80 targetPort: 8080 type: LoadBalancer
3. Apply the deployment and service configurations:
kubectl apply -f deployment.yaml kubectl apply -f service.yaml
Performance Optimization in Gin
Performance optimization is crucial for web applications to provide a fast and responsive user experience. In this section, we'll explore some best practices for optimizing the performance of Gin applications.
Minimizing Response Size
Reducing the size of the response payload can significantly improve the performance of your Gin application, especially for bandwidth-constrained clients or mobile devices. Here are some strategies to minimize the response size:
- Use pagination or limit the number of items returned in a single response.
- Use compression (e.g., gzip) to reduce the size of the response during transmission.
- Minify or compress JSON responses by removing unnecessary white spaces and line breaks.
- Optimize image assets by compressing them or using appropriate image formats (e.g., WebP).
Related Article: Golang & Gin Security: JWT Auth, Middleware, and Cryptography
Caching Responses
Caching responses can significantly improve the performance of your Gin application by reducing the load on your server and improving response times for subsequent requests. Here are some caching strategies you can employ:
- Use HTTP caching headers (e.g., Cache-Control
, ETag
, Last-Modified
) to instruct client and intermediate caches to cache the response.
- Implement server-side caching using in-memory caches (e.g., Redis) or distributed caches (e.g., Memcached) for frequently accessed resources or expensive computations.
- Consider using a reverse proxy (e.g., Nginx) with caching capabilities to serve static assets or cache dynamic responses.
Optimizing Database Access
Efficient database access is crucial for the performance of your Gin application. Here are some strategies to optimize database access:
- Use appropriate indexes on frequently queried columns to improve query performance.
- Minimize the number of database roundtrips by using eager loading or batching techniques.
- Implement database connection pooling to reuse database connections and minimize connection overhead.
- Use connection timeouts and query timeouts to prevent long-running queries from impacting the performance of your application.
File Uploads in Gin
Handling file uploads is a common requirement for web applications, allowing users to upload files such as images, documents, or media files. In this section, we'll explore how to handle file uploads in Gin applications effectively.
Uploading Files
To handle file uploads in a Gin application, you can use the c.SaveUploadedFile
function provided by Gin. This function saves the uploaded file to a specified destination on the server's filesystem.
Here's an example of how you can handle file uploads in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.POST("/upload", handleUpload) router.Run(":8080") } func handleUpload(c *gin.Context) { // Retrieve the uploaded file file, err := c.FormFile("file") if err != nil { c.AbortWithStatusJSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Save the file to a specified destination err = c.SaveUploadedFile(file, "uploads/"+file.Filename) if err != nil { c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } c.JSON(http.StatusOK, gin.H{"message": "File uploaded successfully"}) }
In this example, we define a /upload
route that handles file uploads. We retrieve the uploaded file using c.FormFile
and save it to the uploads
directory using c.SaveUploadedFile
.
Related Article: Handling Large Data Volume with Golang & Beego
Limiting File Size
To prevent abuse or resource exhaustion, it's important to limit the size of the uploaded files in your Gin application. You can use the c.MaxMultipartMemory
function provided by Gin to set the maximum memory allowed for multipart forms, including file uploads.
Here's an example of how you can limit the file size in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.MaxMultipartMemory = 8 << 20 // Limit file size to 8MB router.POST("/upload", handleUpload) router.Run(":8080") } func handleUpload(c *gin.Context) { // Retrieve the uploaded file file, err := c.FormFile("file") if err != nil { c.AbortWithStatusJSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Check the file size if file.Size > 8<<20 { c.AbortWithStatusJSON(http.StatusBadRequest, gin.H{"error": "File size exceeds the limit"}) return } // Save the file to a specified destination err = c.SaveUploadedFile(file, "uploads/"+file.Filename) if err != nil { c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } c.JSON(http.StatusOK, gin.H{"message": "File uploaded successfully"}) }
In this example, we set the router.MaxMultipartMemory
field to limit the file size to 8MB. If the uploaded file exceeds this limit, we return an error response.
Best Practices for Error Handling in Gin
Effective error handling is crucial for building robust and reliable applications. In this section, we'll explore some best practices for error handling in Gin applications.
Centralized Error Handling
To ensure consistent error handling across your application, it's beneficial to centralize error handling logic. By centralizing error handling, you can avoid code duplication and easily apply error handling strategies, such as logging or custom error responses, in one place.
Here's an example of how you can centralize error handling in a Gin application using middleware:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.Use(ErrorHandlerMiddleware()) router.GET("/users/:id", func(c *gin.Context) { // Process the request // ... }) router.Run(":8080") } func ErrorHandlerMiddleware() gin.HandlerFunc { return func(c *gin.Context) { c.Next() if len(c.Errors) > 0 { err := c.Errors.Last() // Handle the error (e.g., log, return custom response) c.JSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) } } }
In this example, we define an error handler middleware that wraps all route handlers. After the route handler completes, the middleware checks if any errors occurred during the request. If an error is present, we handle it by returning a custom error response.
Returning Custom Error Responses
When an error occurs in your application, it's important to provide meaningful error responses to the client. Custom error responses can help clients understand and resolve any issues that may occur.
Here's an example of how you can return custom error responses in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") // Simulating an error if id == "0" { c.JSON(http.StatusBadRequest, gin.H{"error": "Invalid user ID"}) return } // Process user request c.JSON(http.StatusOK, gin.H{"message": "User found"}) }) router.Run(":8080") }
In this example, we simulate an error by checking if the user ID is invalid. If the user ID is invalid, we return a custom error response with an appropriate status code and error message. Otherwise, we return a success response.
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
Pagination in Gin Applications
Pagination is a common requirement for APIs that return large result sets. It allows clients to retrieve a subset of data at a time, improving performance and reducing bandwidth consumption. In this section, we'll explore how to implement pagination in Gin applications effectively.
Limit and Offset Pagination
One common approach to pagination is limit and offset pagination, where the client specifies the number of items to retrieve (limit
) and the starting point (offset
) in the result set. This approach is simple and widely supported by databases.
Here's an example of how you can implement limit and offset pagination in a Gin application:
// main.go package main import ( "net/http" "strconv" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users", handleUsers) router.Run(":8080") } func handleUsers(c *gin.Context) { // Retrieve the limit and offset from the query parameters limitStr := c.Query("limit") offsetStr := c.Query("offset") // Parse the limit and offset values limit, err := strconv.Atoi(limitStr) if err != nil { limit = 10 // Default limit } offset, err := strconv.Atoi(offsetStr) if err != nil { offset = 0 // Default offset } // Query users from the database based on the limit and offset users := queryUsers(limit, offset) c.JSON(http.StatusOK, gin.H{"users": users}) } func queryUsers(limit, offset int) []User { // Query users from the database using the limit and offset // ... }
In this example, we define a /users
route that handles retrieving users with limit and offset pagination. We retrieve the limit
and offset
values from the query parameters and parse them using strconv.Atoi
. If the values are not provided or cannot be parsed, we use default values.
We then use the limit
and offset
values to query users from the database using the queryUsers
function. Finally, we return the queried users as a JSON response.
Cursor-based Pagination
Another approach to pagination is cursor-based pagination, where the client specifies a cursor that represents the position in the result set. This approach is more flexible and suitable for scenarios where the result set can change dynamically.
Here's an example of how you can implement cursor-based pagination in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users", handleUsers) router.Run(":8080") } func handleUsers(c *gin.Context) { // Retrieve the cursor from the query parameters cursor := c.Query("cursor") // Query users from the database based on the cursor users := queryUsers(cursor) c.JSON(http.StatusOK, gin.H{"users": users}) } func queryUsers(cursor string) []User { // Query users from the database using the cursor // ... }
In this example, we define a /users
route that handles retrieving users with cursor-based pagination. We retrieve the cursor
value from the query parameters.
We then use the cursor
value to query users from the database using the queryUsers
function. The cursor
value represents the position in the result set, allowing the client to retrieve the next page of results.
Recommended Testing Libraries for Unit Testing in Gin
Unit testing is an essential part of software development, allowing you to verify the correctness of individual components in your application. In this section, we'll explore some recommended testing libraries for unit testing in Gin applications.
Related Article: Real-Time Communication with Beego and WebSockets
Testify
Testify is a popular testing toolkit for Go that provides a set of utilities and assertions to simplify unit testing. It includes a rich set of assertion methods and mocking capabilities, making it a great choice for unit testing in Gin applications.
Here's an example of how you can use Testify to write unit tests for a Gin application:
// user_controller_test.go package controllers_test import ( "net/http" "net/http/httptest" "testing" "github.com/gin-gonic/gin" "github.com/stretchr/testify/assert" "github.com/stretchr/testify/mock" ) type MockUserService struct { mock.Mock } func (m *MockUserService) GetUserByID(id int) (*User, error) { args := m.Called(id) return args.Get(0).(*User), args.Error(1) } func TestGetUserByID(t *testing.T) { // Setup router := gin.Default() userService := new(MockUserService) router.GET("/users/:id", controllers.GetUserByID(userService)) // Mock the user service expectedUser := &User{ID: 123, Name: "John Doe"} userService.On("GetUserByID", 123).Return(expectedUser, nil) // Create a test request req, err := http.NewRequest("GET", "/users/123", nil) assert.NoError(t, err) // Perform the request rec := httptest.NewRecorder() router.ServeHTTP(rec, req) // Assert the response assert.Equal(t, http.StatusOK, rec.Code) assert.Equal(t, "{\"id\":123,\"name\":\"John Doe\"}", rec.Body.String()) } func TestGetUserByID_Error(t *testing.T) { // Setup router := gin.Default() userService := new(MockUserService) router.GET("/users/:id", controllers.GetUserByID(userService)) // Mock the user service to return an error userService.On("GetUserByID", 123).Return(nil, errors.New("User not found")) // Create a test request req, err := http.NewRequest("GET", "/users/123", nil) assert.NoError(t, err) // Perform the request rec := httptest.NewRecorder() router.ServeHTTP(rec, req) // Assert the response assert.Equal(t, http.StatusNotFound, rec.Code) assert.Equal(t, "{\"error\":\"User not found\"}", rec.Body.String()) }
In this example, we use Testify to write unit tests for the GetUserByID
controller. We create a mock user service using Testify's mocking capabilities and define the expected behavior of the mock service.
We then create a test request using http.NewRequest
and perform the request using router.ServeHTTP
. Finally, we assert the response status code and body to validate the behavior of the controller.
Ginkgo and Gomega
Ginkgo and Gomega are popular testing frameworks for Go that provide a rich set of features for writing readable and expressive tests. Ginkgo allows you to write tests in a behavior-driven development (BDD) style, while Gomega provides a set of useful matchers for asserting test expectations.
Here's an example of how you can use Ginkgo and Gomega to write unit tests for a Gin application:
// user_controller_test.go package controllers_test import ( "net/http" "net/http/httptest" "github.com/gin-gonic/gin" . "github.com/onsi/ginkgo" . "github.com/onsi/gomega" ) var _ = Describe("UserController", func() { var ( router *gin.Engine ) BeforeEach(func() { router = gin.Default() }) Describe("GET /users/:id", func() { Context("when the user exists", func() { It("should return the user", func() { // Setup router.GET("/users/:id", controllers.GetUserByID) // Create a test request req, err := http.NewRequest("GET", "/users/123", nil) Expect(err).NotTo(HaveOccurred()) // Perform the request rec := httptest.NewRecorder() router.ServeHTTP(rec, req) // Assert the response Expect(rec.Code).To(Equal(http.StatusOK)) Expect(rec.Body.String()).To(MatchJSON(`{"id": 123, "name": "John Doe"}`)) }) }) Context("when the user does not exist", func() { It("should return a 404 error", func() { // Setup router.GET("/users/:id", controllers.GetUserByID) // Create a test request req, err := http.NewRequest("GET", "/users/123", nil) Expect(err).NotTo(HaveOccurred()) // Perform the request rec := httptest.NewRecorder() router.ServeHTTP(rec, req) // Assert the response Expect(rec.Code).To(Equal(http.StatusNotFound)) Expect(rec.Body.String()).To(MatchJSON(`{"error": "User not found"}`)) }) }) }) })
In this example, we use Ginkgo and Gomega to write unit tests for the GetUserByID
controller in a BDD style. We use Ginkgo's Describe
and Context
functions to structure the tests and Gomega's matchers to assert the desired behavior.
Handling CORS in Gin
Cross-Origin Resource Sharing (CORS) is an important aspect of modern web applications that enables secure communication between different domains. In this section, we'll explore how to handle CORS in Gin applications effectively.
Enabling CORS
To enable CORS in a Gin application, you can use the github.com/gin-contrib/cors
middleware. This middleware adds the necessary headers to the response to allow cross-origin requests.
Here's an example of how you can enable CORS in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-contrib/cors" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() // Enable CORS config := cors.DefaultConfig() config.AllowOrigins = []string{"http://example.com"} config.AllowMethods = []string{"GET", "POST", "PUT", "DELETE"} router.Use(cors.New(config)) router.GET("/users", func(c *gin.Context) { // Handle the request }) router.Run(":8080") }
In this example, we enable CORS by using the cors.DefaultConfig
function to create a default CORS configuration. We then customize the allowed origins and methods to fit your specific requirements. Finally, we use cors.New
to create a new CORS middleware with the custom configuration and apply it to the router using router.Use
.
Related Article: Internationalization in Gin with Go Libraries
Common Security Vulnerabilities in Gin Applications
Building secure web applications is crucial to protect sensitive user data and prevent unauthorized access. In this section, we'll explore some common security vulnerabilities in Gin applications and how to mitigate them.
Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. To mitigate XSS vulnerabilities in Gin applications, you can:
- Sanitize user input by escaping or removing potentially malicious characters.
- Use context-aware output encoding functions, such as html/template
's template.HTMLEscapeString
or html.EscapeString
, to safely output user-generated content.
- Enable Content Security Policy (CSP) headers to restrict the sources of executable scripts and other resources.
SQL Injection
SQL Injection is a vulnerability that allows attackers to execute arbitrary SQL commands by inserting malicious input into SQL queries. To prevent SQL Injection vulnerabilities in Gin applications, you can:
- Use parameterized queries or prepared statements to separate SQL code from user input.
- Avoid constructing SQL queries using string concatenation or interpolation.
- Use an ORM or query builder that automatically handles parameterization and sanitization of user input.
Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is a vulnerability that allows attackers to trick authenticated users into performing unintended actions on a website. To mitigate CSRF vulnerabilities in Gin applications, you can:
- Implement CSRF protection mechanisms such as CSRF tokens or same-site cookies.
- Validate the CSRF token for each sensitive action or request that modifies server-side state.
- Use the github.com/gin-contrib/csrf
middleware to handle CSRF protection automatically.
Related Article: Enterprise Functionalities in Golang: SSO, RBAC and Audit Trails in Gin
Authentication and Authorization
Inadequate authentication and authorization mechanisms can lead to unauthorized access and data breaches. To ensure secure authentication and authorization in Gin applications, you can:
- Use strong and properly hashed passwords for user authentication.
- Implement multi-factor authentication (MFA) to enhance security.
- Use appropriate session management techniques to prevent session hijacking or fixation.
- Enforce access control mechanisms to restrict unauthorized access to sensitive resources.
Database Transactions in Gin
Database transactions are essential for maintaining data consistency and integrity in web applications. In this section, we'll explore how to use database transactions effectively in Gin applications.
Using Database Transactions
To use database transactions in a Gin application, you can leverage the transaction capabilities provided by your chosen database library or ORM. Database transactions allow you to perform a series of database operations as an atomic unit, ensuring that all operations succeed or fail together.
Here's an example of how you can use database transactions in a Gin application using the database/sql
package:
// main.go package main import ( "database/sql" "net/http" "github.com/gin-gonic/gin" ) func main() { db, err := sql.Open("postgres", "user=postgres password=postgres dbname=mydb sslmode=disable") if err != nil { log.Fatal(err) } router := gin.Default() router.POST("/users", func(c *gin.Context) { // Start a database transaction tx, err := db.Begin() if err != nil { c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } defer tx.Rollback() // Create a new user err = createUser(tx, "John Doe") if err != nil { c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } // Commit the transaction err = tx.Commit() if err != nil { c.AbortWithStatusJSON(http.StatusInternalServerError, gin.H{"error": err.Error()}) return } c.Status(http.StatusOK) }) router.Run(":8080") } func createUser(tx *sql.Tx, name string) error { // Insert a new user into the database _, err := tx.Exec("INSERT INTO users (name) VALUES ($1)", name) return err }
In this example, we define a /users
route that creates a new user in the database using a database transaction. We use the db.Begin
function to start a new transaction and the tx.Commit
function to commit the transaction. If an error occurs during the transaction, we use tx.Rollback
to abort the transaction.
Caching Options in Gin
Caching is a useful technique for improving the performance and scalability of web applications. In this section, we'll explore different caching options for Gin applications.
Related Article: Deployment and Monitoring Strategies for Gin Apps
In-Memory Caching
In-memory caching stores frequently accessed data in memory, reducing the need to query the database or perform expensive computations. In a Gin application, you can use an in-memory cache library such as github.com/patrickmn/go-cache
or the sync.Map
from the Go standard library.
Here's an example of how you can use the go-cache
library for in-memory caching in a Gin application:
// main.go package main import ( "net/http" "time" "github.com/gin-gonic/gin" "github.com/patrickmn/go-cache" ) func main() { c := cache.New(5*time.Minute, 10*time.Minute) router := gin.Default() router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") // Check if the user is in the cache if user, found := c.Get(id); found { c.JSON(http.StatusOK, user) return } // Retrieve the user from the database user := getUserFromDatabase(id) // Cache the user c.Set(id, user, cache.DefaultExpiration) c.JSON(http.StatusOK, user) }) router.Run(":8080") } func getUserFromDatabase(id string) User { // Retrieve the user from the database // ... }
In this example, we use the go-cache
library to implement in-memory caching in a Gin application. We create a new cache with a default expiration time of 5 minutes and a cleanup interval of 10 minutes.
When handling a request for a specific user, we check if the user is in the cache using c.Get
. If the user is found, we return it directly from the cache. Otherwise, we retrieve the user from the database, cache it using c.Set
, and return it.
Distributed Caching
Distributed caching allows you to store frequently accessed data in a distributed cache, such as Redis or Memcached. In a Gin application, you can use a distributed cache library such as github.com/go-redis/redis
to leverage the caching capabilities of Redis.
Here's an example of how you can use Redis for distributed caching in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-gonic/gin" "github.com/go-redis/redis/v8" ) func main() { rdb := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", // no password set DB: 0, // use default DB }) router := gin.Default() router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") // Check if the user is in Redis val, err := rdb.Get(c, id).Result() if err == nil { c.JSON(http.StatusOK, val) return } // Retrieve the user from the database user := getUserFromDatabase(id) // Cache the user in Redis rdb.Set(c, id, user, 0) c.JSON(http.StatusOK, user) }) router.Run(":8080") } func getUserFromDatabase(id string) User { // Retrieve the user from the database // ... }
In this example, we use the github.com/go-redis/redis
library to implement distributed caching in a Gin application. We create a new Redis client and configure it to connect to a Redis server running on localhost:6379
.
When handling a request for a specific user, we check if the user is in Redis using rdb.Get
. If the user is found, we return it directly from Redis. Otherwise, we retrieve the user from the database, cache it in Redis using rdb.Set
, and return it.
Rate Limiting in Gin
Rate limiting is an important technique for preventing abuse and ensuring fair usage of web APIs. In this section, we'll explore how to implement rate limiting in Gin applications effectively.
Using Middleware
To implement rate limiting in a Gin application, you can use the github.com/gin-contrib/limit
middleware. This middleware allows you to limit the number of requests per minute, hour, or day for a specific route or set of routes.
Here's an example of how you can use the limit
middleware for rate limiting in a Gin application:
// main.go package main import ( "net/http" "github.com/gin-contrib/limit" "github.com/gin-gonic/gin" ) func main() { router := gin.Default() // Apply rate limiting middleware to the /users route router.GET("/users", limit.MaxAllowed(10), func(c *gin.Context) { // Handle the request }) router.Run(":8080") }
In this example, we use the limit.MaxAllowed
function from the limit
middleware to apply rate limiting to the /users
route. We set the maximum allowed requests to 10 per minute.
Related Article: Design Patterns with Beego & Golang
Recommended Logging Libraries for Gin Applications
Logging is a critical aspect of any application, providing valuable insights into the behavior and performance of your code. In this section, we'll explore some recommended logging libraries for Gin applications.
Zap
Zap is a high-performance logging library for Go that focuses on both performance and usability. It provides a simple and efficient API for logging structured logs, making it a great choice for logging in Gin applications.
Here's an example of how you can use Zap for logging in a Gin application:
// main.go package main import ( "go.uber.org/zap" "net/http" "github.com/gin-gonic/gin" ) func main() { logger, _ := zap.NewProduction() defer logger.Sync() router := gin.Default() router.Use(LoggerMiddleware(logger)) router.GET("/users/:id", func(c *gin.Context) { // Handle the request logger.Info("GET /users/:id", zap.String("path", c.Request.URL.Path), zap.String("id", c.Param("id")), ) }) router.Run(":8080") } func LoggerMiddleware(logger *zap.Logger) gin.HandlerFunc { return func(c *gin.Context) { // Log the request logger.Info("Incoming request", zap.String("method", c.Request.Method), zap.String("path", c.Request.URL.Path), ) c.Next() } }
In this example, we use Zap for logging in a Gin application. We create a new Zap logger using zap.NewProduction
, and we defer logger.Sync
to flush any buffered logs before the program exits.
We define a LoggerMiddleware
that logs incoming requests using the Zap logger. We then use this middleware in the Gin application to log each request.
Logrus
Logrus is a popular logging library for Go that provides a flexible and extensible API. It supports structured logging and integrates well with many third-party libraries, making it a versatile choice for logging in Gin applications.
Here's an example of how you can use Logrus for logging in a Gin application:
// main.go package main import ( "net/http" "os" "github.com/gin-gonic/gin" log "github.com/sirupsen/logrus" ) func main() { log.SetFormatter(&log.JSONFormatter{}) log.SetOutput(os.Stdout) router := gin.Default() router.Use(LoggerMiddleware) router.GET("/users/:id", func(c *gin.Context) { // Handle the request log.WithFields(log.Fields{ "method": c.Request.Method, "path": c.Request.URL.Path, "id": c.Param("id"), }).Info("GET /users/:id") }) router.Run(":8080") } func LoggerMiddleware(c *gin.Context) { // Log the request log.WithFields(log.Fields{ "method": c.Request.Method, "path": c.Request.URL.Path, }).Info("Incoming request") c.Next() }
In this example, we use Logrus for logging in a Gin application. We configure Logrus to use the JSON formatter and output logs to the standard output using log.SetFormatter
and log.SetOutput
.
We define a LoggerMiddleware
that logs incoming requests using Logrus. We then use this middleware in the Gin application to log each request.
Additional Resources
- Best practices for error handling and custom error responses in Gin