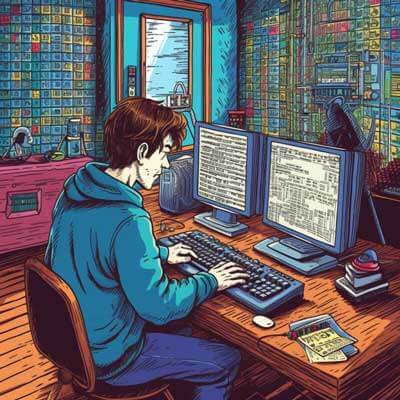
Table of Contents
Introduction
Beego is a useful and flexible web framework for building web applications and APIs using the Go programming language. It provides a solid foundation for developing scalable and efficient applications. In this article, we will explore how to implement payment and communication features in Beego using popular services and libraries.
Related Article: Enterprise Functionalities in Golang: SSO, RBAC and Audit Trails in Gin
Implementing Payment Features in Beego
Implementing payment features in Beego is essential for e-commerce applications and other systems that require online transactions. By integrating payment gateways such as Stripe and PayPal, you can securely process payments and handle transactions. Let's see how to integrate these payment gateways in Beego applications.
Integration of Payment Gateways (Stripe, PayPal) in Beego Applications
To integrate payment gateways like Stripe and PayPal in Beego applications, you need to follow these steps:
1. Register an account with the respective payment gateway provider (Stripe or PayPal).
2. Obtain the necessary API keys to authenticate your Beego application with the payment gateway.
3. Install the required Go packages for Stripe and PayPal integration. For example, you can use the following commands to install the packages:
go get github.com/stripe/stripe-go go get github.com/paypal/checkout-sdk-go
4. Import the installed packages in your Beego application's code:
import ( "github.com/stripe/stripe-go" "github.com/stripe/stripe-go/charge" "github.com/paypal/checkout-sdk-go" )
5. Implement the necessary handlers and routes in Beego to handle payment requests and callbacks from the payment gateway.
6. Use the appropriate methods from the Stripe and PayPal packages to create charges and process payments. For example, to create a charge using Stripe, you can use the following code:
params := &stripe.ChargeParams{ Amount: 2000, Currency: "usd", Source: "tok_visa", Description: "Test Charge", } ch, err := charge.New(params) if err != nil { // handle error } // Process the charge
7. Handle the response from the payment gateway and update your application's database or perform any other necessary actions based on the payment status.
E-commerce Integration in Beego for Payment Features
Beego provides a robust framework for building e-commerce applications. By integrating payment features into your Beego e-commerce application, you can enable secure and seamless online transactions. Here's an example of how to integrate payment features in a Beego e-commerce application:
1. Set up your e-commerce application with Beego, including models, controllers, and views for products, orders, and carts.
2. Create a payment gateway configuration page in your Beego application's admin panel to allow users to enter their API credentials for payment gateways like Stripe or PayPal.
3. Implement the necessary routes and handlers in Beego to handle the checkout process. This includes adding items to the cart, calculating the total amount, and initiating the payment process.
4. Use the Stripe or PayPal Go packages to create charges or initiate payments based on the user's selected payment method.
5. Handle the payment response and update the order status in your database.
6. Notify the user about the payment status and provide a confirmation page with the order details.
Related Article: Building Gin Backends for React.js and Vue.js
Payment Processing in Beego Applications
Payment processing is a critical part of any application that involves financial transactions. Beego provides a flexible framework to handle payment processing in your applications. Here are some key considerations for implementing payment processing in Beego applications:
1. Ensure that you secure your application by implementing proper authentication and authorization mechanisms.
2. Use HTTPS to encrypt the communication between your Beego application and the payment gateway, ensuring the security of sensitive customer data.
3. Implement error handling and proper logging to troubleshoot any issues that may arise during payment processing.
4. Test your payment processing functionality thoroughly to ensure that it works as expected in different scenarios, such as successful payments, failed payments, and refunds.
5. Consider implementing additional security measures, such as tokenization and fraud detection, to protect against fraudulent transactions.
6. Keep your payment processing code modular and maintainable by following best practices for code organization and documentation.
Implementing Communication Features in Beego
Implementing communication features in Beego allows you to enhance your applications with voice and text capabilities. Services like Twilio provide APIs that enable you to send SMS messages, make phone calls, and implement interactive voice response (IVR) systems. Let's explore how to implement voice and text features using services like Twilio in Beego.
Implementing Voice and Text Features in Go Applications with Beego
To implement voice and text features in Go applications with Beego, you can use the Twilio API. Twilio provides a Go package that allows you to interact with their APIs easily. Here's an example of how to send an SMS message using Twilio in a Beego application:
1. Install the Twilio Go package:
go get github.com/twilio/twilio-go
2. Import the Twilio package in your Beego application's code:
import ( "github.com/twilio/twilio-go" "github.com/twilio/twilio-go/rest/api/v2010/account/message" )
3. Set up your Twilio account by obtaining your Account SID and Auth Token from the Twilio dashboard.
4. Initialize a new Twilio client using your Account SID and Auth Token:
client := twilio.NewRestClient("YOUR_ACCOUNT_SID", "YOUR_AUTH_TOKEN")
5. Use the Twilio client to send an SMS message:
params := &message.CreateMessageParams{ Body: "Hello from Twilio!", From: "YOUR_TWILIO_PHONE_NUMBER", To: "RECIPIENT_PHONE_NUMBER", } _, err := client.Messages.CreateMessage(params) if err != nil { // handle error }
6. Handle the response from the Twilio API and take any necessary actions based on the message status.
Building Chatbots with Beego in Go
Chatbots have become increasingly popular for providing automated customer support and handling routine tasks. Beego, combined with natural language processing (NLP) libraries, allows you to build chatbots that can understand and respond to user queries. Here's an example of how to build a chatbot with Beego in Go:
1. Set up your Beego application and define routes and handlers for processing incoming chat messages.
2. Use a natural language processing library like Gophrase to analyze the user's message and extract relevant information. Install the Gophrase package using the following command:
go get github.com/gophrase/gophrase
3. Import the Gophrase package in your Beego application's code:
import ( "github.com/gophrase/gophrase" )
4. Initialize the Gophrase library and load any necessary language models:
phrase := gophrase.New() err := phrase.LoadModel("path/to/language/model") if err != nil { // handle error }
5. Process the user's message using Gophrase to extract intents and entities:
result, err := phrase.Analyze("user message") if err != nil { // handle error } // Extract intents and entities from the result intents := result.GetIntents() entities := result.GetEntities()
6. Based on the extracted intents and entities, implement the logic to respond to the user's query. This could involve querying a database, making API calls, or generating a predefined response.
7. Send the response back to the user via the appropriate communication channel (e.g., SMS, chat interface).
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
API Integration in Beego for Payment and Communication Features
API integration is a common requirement when implementing payment and communication features in Beego applications. Whether you are integrating payment gateways or communication services, Beego provides a straightforward way to interact with external APIs. Here's an example of how to integrate an API in Beego for payment and communication features:
1. Identify the API you want to integrate into your Beego application. This could be a payment gateway API like Stripe or a communication API like Twilio.
2. Obtain the necessary API credentials (e.g., API keys, access tokens) from the API provider.
3. Install the required Go packages for interacting with the API. For example, if you are integrating the Stripe API, you can use the following command to install the Stripe Go package:
go get github.com/stripe/stripe-go
4. Import the installed packages in your Beego application's code:
import ( "github.com/stripe/stripe-go" )
5. Set up the necessary configuration for the API, such as the API endpoint URL and authentication credentials.
6. Implement the required routes and handlers in Beego to handle API requests and responses.
7. Use the appropriate methods from the API package to interact with the API. For example, to create a charge using the Stripe API, you can use the following code:
params := &stripe.ChargeParams{ Amount: 2000, Currency: "usd", Source: "tok_visa", Description: "Test Charge", } ch, err := charge.New(params) if err != nil { // handle error } // Process the charge
8. Handle the API response and update your application's database or perform any other necessary actions based on the response.
Web Development with Payment and Communication Features in Beego
Beego provides a useful framework for web development, allowing you to build feature-rich web applications with payment and communication capabilities. With Beego, you can easily integrate payment gateways, implement chatbots, and enable voice and text features in your web applications. Here's an example of how to develop a web application with payment and communication features using Beego:
1. Set up your Beego application by creating models, controllers, and views for your web application's functionality.
2. Implement the necessary routes and handlers in Beego to handle user requests for payment and communication features.
3. Integrate payment gateways like Stripe or PayPal by following the steps outlined earlier in this article. This will enable users to make secure online payments.
4. Use the Twilio API to implement voice and text features in your web application. For example, you can allow users to send SMS messages or make phone calls directly from your web application.
5. Implement chatbot functionality using natural language processing libraries like Gophrase. This will enable your web application to understand and respond to user queries.
6. Secure your web application by implementing proper authentication and authorization mechanisms. This is crucial when handling sensitive user data and financial transactions.
7. Test your web application thoroughly to ensure that all payment and communication features work as expected. Consider using automated testing frameworks like Go's built-in testing package or external libraries like Ginkgo and Gomega.
8. Deploy your Beego web application to a production environment, ensuring that it is securely hosted and can handle a high volume of traffic.
9. Monitor your web application's performance and logs to identify any issues or potential improvements. Consider using monitoring tools like Prometheus or New Relic to gain insights into your application's performance and user behavior.
Mobile App Development with Payment and Communication Features in Beego
Beego is not only suitable for web development but also for mobile app development. With Beego, you can build robust and scalable backend APIs for your mobile apps, enabling them to leverage payment and communication features. Here's an example of how to develop a mobile app with payment and communication features using Beego:
1. Set up your Beego application by creating controllers and routes for your mobile app's API endpoints.
2. Implement the necessary API endpoints to handle payment and communication requests from your mobile app. This could include endpoints for processing payments, sending SMS messages, or making phone calls.
3. Integrate payment gateways like Stripe or PayPal into your Beego application, following the steps outlined earlier in this article. This will allow your mobile app to securely process payments.
4. Use the Twilio API to implement voice and text features in your mobile app. For example, you can allow users to send SMS messages or make phone calls directly from your mobile app.
5. Implement push notification functionality in your Beego application to communicate with your mobile app. This will enable you to send important updates or notifications to your mobile app users.
6. Secure your Beego API by implementing proper authentication and authorization mechanisms. This is crucial when handling sensitive user data and financial transactions.
7. Test your mobile app thoroughly to ensure that all payment and communication features work as expected. Consider using automated testing frameworks like XCTest for iOS or Espresso for Android.
8. Deploy your Beego application to a production environment, ensuring that it is securely hosted and can handle a high volume of API requests from your mobile app.
9. Monitor your Beego application's performance and logs to identify any issues or potential improvements. Consider using monitoring tools like Prometheus or New Relic to gain insights into your application's performance and user behavior.
Software Integration with Payment and Communication Features in Beego
Integrating payment and communication features into your existing software systems can enhance their functionality and provide new capabilities. Beego offers a flexible framework for integrating payment gateways, implementing chatbots, and enabling voice and text features in your software applications. Here's an example of how to integrate payment and communication features into your software using Beego:
1. Identify the software systems or components that require payment and communication features. This could be an e-commerce system, a customer support application, or any other software that involves financial transactions or communication with users.
2. Set up a new Beego application or add the necessary routes and handlers to your existing Beego application to handle payment and communication requests.
3. Integrate payment gateways like Stripe or PayPal into your Beego application, following the steps outlined earlier in this article. This will enable your software to securely process payments.
4. Use the Twilio API to implement voice and text features in your Beego application. For example, you can allow your software to send automated SMS notifications or make phone calls to users.
5. Implement chatbot functionality using natural language processing libraries like Gophrase. This will enable your software to understand and respond to user queries or automate routine tasks.
6. Secure your Beego application by implementing proper authentication and authorization mechanisms. This is crucial when handling sensitive user data and financial transactions.
7. Test your software thoroughly to ensure that all payment and communication features work as expected. Consider using automated testing frameworks like Go's built-in testing package or external libraries like Ginkgo and Gomega.
8. Deploy your Beego application to a production environment, ensuring that it is securely hosted and can handle a high volume of requests from your software systems.
9. Monitor your Beego application's performance and logs to identify any issues or potential improvements. Consider using monitoring tools like Prometheus or New Relic to gain insights into your application's performance and user behavior.
Related Article: Exploring Advanced Features of Gin in Golang
Voice Recognition and Natural Language Processing in Beego
Voice recognition and natural language processing (NLP) are useful technologies that can enhance the functionality of your Beego applications. By integrating voice recognition and NLP capabilities, you can enable voice commands, automate tasks, and provide personalized user experiences. Here's an example of how to implement voice recognition and NLP in Beego using external libraries:
1. Identify the voice recognition and NLP libraries that best suit your requirements. Some popular options include Google Cloud Speech-to-Text, IBM Watson Speech to Text, and Wit.ai.
2. Set up your Beego application and install the necessary Go packages for interacting with the chosen voice recognition and NLP libraries.
3. Import the required packages in your Beego application's code:
import ( "github.com/your-voice-recognition-library" )
4. Configure the voice recognition and NLP libraries with the necessary credentials and parameters. This typically involves obtaining API keys or access tokens from the library provider.
5. Implement the necessary routes and handlers in Beego to capture voice input from users and send it to the voice recognition library for processing.
6. Process the voice input using the voice recognition library to convert it into text. For example, you can use the following code to process voice input using Google Cloud Speech-to-Text:
result, err := yourVoiceRecognitionLibrary.RecognizeSpeech("voice_input.wav") if err != nil { // handle error } transcript := result.GetTranscript()
7. Use natural language processing libraries like Gophrase to analyze the text and extract intents and entities. This will enable your Beego application to understand the user's command or query.
8. Implement the necessary logic in your Beego application to respond to the user's voice command or query. This could involve querying a database, making API calls, or generating a predefined response.
9. Send the response back to the user via the appropriate communication channel (e.g., voice, text).
Text Messaging Features in Beego with Services like Twilio
Text messaging features are a common requirement in many applications, allowing you to send SMS notifications, alerts, or verification codes to users. Beego, combined with services like Twilio, enables easy integration of text messaging capabilities. Here's an example of how to implement text messaging features in Beego using Twilio:
1. Set up your Beego application and install the Twilio Go package:
go get github.com/twilio/twilio-go
2. Import the Twilio package in your Beego application's code:
import ( "github.com/twilio/twilio-go" "github.com/twilio/twilio-go/rest/api/v2010/account/message" )
3. Set up your Twilio account by obtaining your Account SID and Auth Token from the Twilio dashboard.
4. Initialize a new Twilio client using your Account SID and Auth Token:
client := twilio.NewRestClient("YOUR_ACCOUNT_SID", "YOUR_AUTH_TOKEN")
5. Use the Twilio client to send an SMS message:
params := &message.CreateMessageParams{ Body: "Hello from Twilio!", From: "YOUR_TWILIO_PHONE_NUMBER", To: "RECIPIENT_PHONE_NUMBER", } _, err := client.Messages.CreateMessage(params) if err != nil { // handle error }
6. Handle the response from the Twilio API and take any necessary actions based on the message status.
Integrating Payment Gateways in Go Applications with Beego
Integrating payment gateways in Go applications with Beego allows you to securely process payments and handle transactions. Payment gateways like Stripe and PayPal provide APIs that enable you to integrate their services into your applications. Here's an example of how to integrate payment gateways in Go applications with Beego:
1. Register an account with the desired payment gateway provider (e.g., Stripe, PayPal).
2. Obtain the necessary API keys or access tokens from the payment gateway provider.
3. Install the required Go packages for the payment gateway integration. For example, you can use the following commands to install the Stripe and PayPal Go packages:
go get github.com/stripe/stripe-go go get github.com/paypal/checkout-sdk-go
4. Import the installed packages in your Go application's code:
import ( "github.com/stripe/stripe-go" "github.com/paypal/checkout-sdk-go" )
5. Implement the necessary logic in your Go application to handle payment requests and callbacks from the payment gateway.
6. Use the appropriate methods from the payment gateway packages to create charges and process payments. For example, to create a charge using Stripe, you can use the following code:
params := &stripe.ChargeParams{ Amount: 2000, Currency: "usd", Source: "tok_visa", Description: "Test Charge", } ch, err := charge.New(params) if err != nil { // handle error } // Process the charge
7. Handle the response from the payment gateway and update your application's database or perform any other necessary actions based on the payment status.
Popular Payment Gateway Options for Go Applications with Beego
There are several popular payment gateway options available for Go applications with Beego. These payment gateways provide APIs and libraries that make it easy to integrate their services into your applications. Some popular payment gateway options for Go applications with Beego include:
1. Stripe: Stripe is a widely used payment gateway that provides a useful API for processing online payments. It supports various payment methods, including credit cards, digital wallets, and cryptocurrencies.
2. PayPal: PayPal is another popular payment gateway that offers a range of payment solutions, including PayPal Checkout, PayPal Payments Standard, and PayPal Payments Pro.
3. Braintree: Braintree is a payment gateway owned by PayPal that provides a flexible API for accepting payments in your applications. It supports various payment methods and features advanced fraud detection capabilities.
4. Square: Square is a payment gateway and point-of-sale system that offers a range of payment solutions, including in-person and online payments. It provides a robust API for integrating its services into your applications.
5. Authorize.Net: Authorize.Net is a payment gateway that offers a suite of payment solutions for businesses. It provides a comprehensive API for accepting payments and managing transactions.
When choosing a payment gateway for your Go applications with Beego, consider factors such as pricing, ease of integration, available features, and supported payment methods.
Related Article: Internationalization in Gin with Go Libraries
Building Chatbots with Beego in Go
Chatbots have become increasingly popular for automating customer support, handling routine tasks, and providing personalized user experiences. Beego, combined with natural language processing (NLP) libraries, allows you to build chatbots in Go that can understand and respond to user queries. Here's an example of how to build chatbots with Beego in Go:
1. Set up your Beego application and define routes and handlers for processing incoming chat messages.
2. Use a natural language processing library like Gophrase to analyze the user's message and extract relevant information. Install the Gophrase package using the following command:
go get github.com/gophrase/gophrase
3. Import the Gophrase package in your Beego application's code:
import ( "github.com/gophrase/gophrase" )
4. Initialize the Gophrase library and load any necessary language models:
phrase := gophrase.New() err := phrase.LoadModel("path/to/language/model") if err != nil { // handle error }
5. Process the user's message using Gophrase to extract intents and entities:
result, err := phrase.Analyze("user message") if err != nil { // handle error } // Extract intents and entities from the result intents := result.GetIntents() entities := result.GetEntities()
6. Based on the extracted intents and entities, implement the logic to respond to the user's query. This could involve querying a database, making API calls, or generating a predefined response.
7. Send the response back to the user via the appropriate communication channel (e.g., chat interface).
Creating IVRs with Beego
Interactive voice response (IVR) systems are commonly used in telephony applications to interact with callers and route calls to the appropriate destination. Beego, combined with services like Twilio, allows you to create IVRs in Go that can handle phone calls and provide automated responses. Here's an example of how to create IVRs with Beego:
1. Set up your Beego application and define routes and handlers for processing incoming calls.
2. Use the Twilio API to receive incoming calls and handle the IVR flow. Twilio provides a Go package that makes it easy to interact with their APIs.
3. Configure your Twilio phone number to forward incoming calls to your Beego application's IVR endpoint.
4. Implement the necessary logic in your Beego application to handle the IVR flow. This could involve playing pre-recorded messages, collecting user input, and making decisions based on the user's input.
5. Use the Twilio API to generate dynamic voice responses based on the IVR flow. For example, you can use the TwiML (Twilio Markup Language) to generate XML responses that control the call flow.
6. Handle user input by collecting DTMF (dual-tone multi-frequency) tones or speech recognition using the Twilio API.
7. Implement the necessary logic to route the call to the appropriate destination based on the IVR flow. This could involve transferring the call to another phone number, connecting the caller to a specific department, or playing recorded messages.
8. Test your IVR system thoroughly to ensure that it works as expected in different scenarios. Consider using tools like ngrok to expose your local Beego application to the internet for testing purposes.
Advantages of Integrating Voice and Text Interfaces with Beego
Integrating voice and text interfaces with Beego can provide several advantages for your applications:
1. Enhanced user experiences: Voice and text interfaces enable natural and intuitive interactions with your applications. Users can speak or type commands, making the interaction more conversational and user-friendly.
2. Accessibility: Voice interfaces can make your applications more accessible to users with disabilities or impairments. Users with visual impairments can interact with your application using voice commands, while users with mobility impairments can use speech recognition instead of manual input.
3. Automation and efficiency: Voice and text interfaces allow you to automate routine tasks and processes, reducing the need for manual intervention. This can improve the efficiency of your applications and free up resources for more complex tasks.
4. Personalization: Voice and text interfaces enable personalized interactions with your applications. By understanding user preferences and context, you can tailor the responses and actions of your applications to individual users.
5. Multimodal interactions: Integrating voice and text interfaces with Beego allows you to support multimodal interactions. Users can switch between voice and text input based on their preferences or the context of the interaction.
6. Scalability: Beego's scalability and performance make it well-suited for handling voice and text interfaces. You can handle a high volume of simultaneous interactions without compromising the responsiveness of your applications.
7. Integration with external services: Voice and text interfaces can be integrated with external services like payment gateways, communication APIs, and natural language processing libraries. This allows you to leverage the capabilities of these services and enhance the functionality of your applications.
How Beego Handles Payment Processing
Beego does not have built-in payment processing capabilities. However, Beego provides a flexible framework for integrating payment gateways and handling payment processing in your applications. By leveraging third-party payment gateway APIs and libraries, you can securely process payments and handle transactions in your Beego applications.
To handle payment processing in Beego, you typically follow these steps:
1. Choose a payment gateway provider that suits your requirements. Popular options include Stripe, PayPal, Braintree, Square, and Authorize.Net.
2. Register an account with the chosen payment gateway provider and obtain the necessary API keys or access tokens.
3. Install the required Go packages for the chosen payment gateway integration. For example, if you are integrating Stripe, you can use the following command to install the Stripe Go package:
go get github.com/stripe/stripe-go
4. Import the installed package in your Beego application's code:
import ( "github.com/stripe/stripe-go" )
5. Implement the necessary routes and handlers in Beego to handle payment requests and callbacks from the payment gateway.
6. Use the appropriate methods from the payment gateway package to create charges and process payments. For example, to create a charge using Stripe, you can use the following code:
params := &stripe.ChargeParams{ Amount: 2000, Currency: "usd", Source: "tok_visa", Description: "Test Charge", } ch, err := charge.New(params) if err != nil { // handle error } // Process the charge
7. Handle the response from the payment gateway and update your application's database or perform any other necessary actions based on the payment status.
Beego's flexibility and extensibility make it a suitable choice for handling payment processing in your applications. By integrating payment gateways and following best practices for security and reliability, you can ensure that your Beego applications handle payment processing effectively and securely.
Related Article: Golang Tutorial for Backend Development
Best Practices for Integrating Payment and Communication Features in Go Applications
When integrating payment and communication features in Go applications, it's important to follow best practices to ensure the security, reliability, and maintainability of your applications. Here are some best practices to consider when integrating payment and communication features in Go applications:
1. Use secure connections: Ensure that all communication between your Go application and payment gateways or communication services is encrypted using HTTPS. This helps protect sensitive user data and prevents unauthorized access.
2. Implement authentication and authorization: Secure your Go application by implementing proper authentication and authorization mechanisms. Use strong password hashing algorithms, enforce password complexity requirements, and implement role-based access control to restrict access to sensitive functionality.
3. Validate user input: Validate and sanitize all user input to prevent common security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks. Use appropriate input validation libraries or frameworks to handle input validation and sanitation.
4. Implement error handling and logging: Proper error handling and logging are essential for diagnosing and troubleshooting issues in your Go applications. Implement robust error handling mechanisms and log errors and exceptions to a centralized logging system for analysis and monitoring.
5. Follow PCI DSS compliance guidelines: If your Go application handles credit card information or other sensitive payment data, ensure that you follow the Payment Card Industry Data Security Standard (PCI DSS) guidelines. This includes securely storing cardholder data, implementing access controls, and regularly monitoring and testing your systems for vulnerabilities.
6. Test thoroughly: Test your payment and communication features thoroughly to ensure that they work as expected in different scenarios. This includes testing successful payments, failed payments, refunds, and various edge cases. Consider using automated testing frameworks like Go's built-in testing package or external libraries like Ginkgo and Gomega.
7. Keep dependencies up to date: Regularly update the dependencies of your Go application, including payment gateway SDKs, communication service SDKs, and any other external libraries you use. This helps ensure that you have the latest security patches and bug fixes.
8. Monitor and analyze your application: Monitor the performance and usage of your Go application, including payment and communication features. Use monitoring tools like Prometheus or New Relic to gain insights into your application's performance, identify bottlenecks, and detect any abnormalities or issues.
Alternative Solutions to Stripe and PayPal for Payment Integration in Go
While Stripe and PayPal are popular choices for payment integration in Go applications, there are alternative solutions available that you can consider based on your specific requirements. These alternative payment gateways provide similar functionality and may offer different features or pricing structures. Here are some alternative solutions to Stripe and PayPal for payment integration in Go:
1. Braintree: Braintree is a payment gateway owned by PayPal that provides a flexible API for accepting payments in your applications. It supports various payment methods, including credit cards, digital wallets, and cryptocurrencies.
2. Square: Square is a payment gateway and point-of-sale system that offers a range of payment solutions, including in-person and online payments. It provides a robust API for integrating its services into your applications.
3. Authorize.Net: Authorize.Net is a payment gateway that offers a suite of payment solutions for businesses. It provides a comprehensive API for accepting payments and managing transactions.
4. Adyen: Adyen is a global payment platform that offers a wide range of payment methods and features. It provides a unified API that simplifies payment integration across different regions and currencies.
5. 2Checkout: 2Checkout is a payment gateway that supports multiple payment methods and provides features like recurring billing and fraud protection. It offers a flexible API for integrating its services into your applications.
When evaluating alternative payment solutions, consider factors such as pricing, supported payment methods, available features, and ease of integration with Go applications. Choose a payment gateway that aligns with your business requirements and provides the necessary functionality for your Go applications.
Building Mobile Apps with Payment and Communication Features using Beego
Beego is not only suitable for web development but also for mobile app development. By building mobile apps with payment and communication features using Beego, you can leverage its useful framework and integrate features like payment gateways, SMS messaging, and voice recognition. Here's an example of how to build mobile apps with payment and communication features using Beego:
1. Set up your Beego application and define routes and handlers for your mobile app's API endpoints.
2. Implement the necessary API endpoints to handle payment and communication requests from your mobile app. This could include endpoints for processing payments, sending SMS messages, or making phone calls.
3. Integrate payment gateways like Stripe or PayPal by following the steps outlined earlier in this article. This will allow your mobile app to securely process payments.
4. Use the Twilio API to implement SMS messaging features in your mobile app. For example, you can allow users to send SMS notifications or alerts directly from your mobile app.
5. Implement voice recognition and natural language processing (NLP) features using libraries like Gophrase. This will enable your mobile app to understand and respond to voice commands or queries.
6. Secure your Beego API by implementing proper authentication and authorization mechanisms. This is crucial when handling sensitive user data and financial transactions.
7. Test your mobile app thoroughly to ensure that all payment and communication features work as expected. Consider using automated testing frameworks like XCTest for iOS or Espresso for Android.
8. Deploy your Beego application to a production environment, ensuring that it is securely hosted and can handle a high volume of API requests from your mobile app.
9. Monitor your Beego application's performance and logs to identify any issues or potential improvements. Consider using monitoring tools like Prometheus or New Relic to gain insights into your application's performance and user behavior.