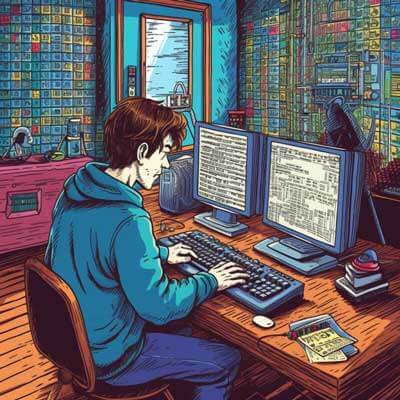
Table of Contents
What is Golang?
Golang, also known as Go, is an open-source programming language developed by Google. It is designed to be efficient, concise, and secure. Go combines the speed of compiled languages like C++ with the simplicity and ease of use of interpreted languages like Python. Go is widely used for building scalable and high-performance applications, especially in the field of web development and cloud infrastructure.
Related Article: Handling Large Data Volume with Golang & Beego
What is the Gin framework?
Gin is a web framework written in Go. It is lightweight, fast, and flexible, making it a popular choice for building web applications in Go. Gin follows the HTTP router model, allowing developers to define routes and handlers easily. It also provides a rich set of middleware that can be used for various purposes, such as authentication, logging, and request validation.
How to set up Single Sign-On (SSO) in Gin using OAuth2.0?
Single Sign-On (SSO) is a mechanism that allows users to authenticate once and gain access to multiple applications or services without the need to log in again. In Gin, we can set up SSO using OAuth2.0, which is an industry-standard protocol for authorization. Here's a step-by-step guide on how to implement SSO in Gin using OAuth2.0:
1. Install the necessary packages:
go get golang.org/x/oauth2go get golang.org/x/oauth2/googlego get github.com/gin-gonic/gin
2. Create a configuration file that contains your OAuth2.0 client credentials, such as the client ID and client secret. You can obtain these credentials by creating a project in the Google Cloud Console.
3. Initialize the OAuth2.0 configuration:
import ( "golang.org/x/oauth2" "golang.org/x/oauth2/google")func main() { // Load the client credentials from the configuration file config, err := google.ConfigFromJSON([]byte(clientCredentials), "https://www.googleapis.com/auth/userinfo.email") if err != nil { log.Fatal("Failed to load client credentials:", err) }}
4. Set up the OAuth2.0 callback route:
import ( "github.com/gin-gonic/gin")func main() { router := gin.Default() // Handle the OAuth2.0 callback router.GET("/oauth2/callback", func(c *gin.Context) { // Get the authorization code from the query parameters code := c.Query("code") // Exchange the authorization code for an access token token, err := config.Exchange(oauth2.NoContext, code) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to exchange authorization code"}) return } // Use the access token to retrieve user information client := config.Client(oauth2.NoContext, token) resp, err := client.Get("https://www.googleapis.com/oauth2/v2/userinfo") if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to retrieve user information"}) return } defer resp.Body.Close() // Parse the user information from the response body var userInfo struct { Email string `json:"email"` } err = json.NewDecoder(resp.Body).Decode(&userInfo) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to parse user information"}) return } // Do something with the user information // ... c.JSON(http.StatusOK, gin.H{"message": "Successfully authenticated"}) }) // Start the server router.Run(":8080")}
5. Redirect the user to the OAuth2.0 authorization endpoint:
import ( "net/http")func main() { router := gin.Default() // Redirect the user to the OAuth2.0 authorization endpoint router.GET("/login", func(c *gin.Context) { url := config.AuthCodeURL("state", oauth2.AccessTypeOffline) c.Redirect(http.StatusTemporaryRedirect, url) }) // ...}
This is a basic example of setting up SSO in Gin using OAuth2.0. The actual implementation may vary depending on the specific OAuth2.0 provider you are using and the requirements of your application.
What is Role-based access control?
Role-based access control (RBAC) is a security model that provides fine-grained access control based on the roles assigned to users. In RBAC, each user is assigned one or more roles, and each role is associated with a set of permissions. This allows administrators to define and manage access control policies at a higher level of abstraction, making it easier to enforce security policies and manage user privileges.
Related Article: Exploring Advanced Features of Gin in Golang
How can Gin integrate with enterprise IAM solutions?
Gin can integrate with enterprise Identity and Access Management (IAM) solutions to provide seamless authentication and authorization functionalities. By integrating with IAM solutions, Gin can leverage existing user management systems, access control policies, and authentication mechanisms. Here's an example of how Gin can integrate with an enterprise IAM solution:
1. Install the necessary packages:
go get github.com/gin-gonic/gingo get github.com/casbin/casbin
2. Set up the Casbin RBAC model and policy:
import ( "github.com/casbin/casbin" "github.com/casbin/gorm-adapter")func main() { // Connect to the database adapter, err := gormadapter.NewAdapter("mysql", "root:password@tcp(127.0.0.1:3306)/") if err != nil { log.Fatal("Failed to connect to the database:", err) } // Initialize the Casbin enforcer with the RBAC model and policy enforcer, err := casbin.NewEnforcer("path/to/model.conf", adapter) if err != nil { log.Fatal("Failed to initialize Casbin enforcer:", err) }}
3. Define middleware to enforce access control policies:
import ( "github.com/gin-gonic/gin")func main() { router := gin.Default() // Define a middleware to enforce access control policies router.Use(func(c *gin.Context) { // Get the current user's ID from the session or token userID := getCurrentUserID(c) // Get the current request's URL and HTTP method requestURL := c.Request.URL.Path requestMethod := c.Request.Method // Check if the current user is allowed to access the requested resource allowed, err := enforcer.Enforce(userID, requestURL, requestMethod) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to enforce access control policies"}) return } if !allowed { c.JSON(http.StatusForbidden, gin.H{"error": "Access denied"}) return } // Continue to the next middleware or handler c.Next() }) // ...}
4. Protect routes using the access control middleware:
import ( "github.com/gin-gonic/gin")func main() { router := gin.Default() // Protect a route using the access control middleware router.GET("/protected", func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{"message": "Access granted"}) }, enforceAccessControl) // ...}
What are audit trails and why are they important in a Gin application?
Audit trails, also known as audit logs or activity logs, are records of events or actions that occur within a system. In the context of a Gin application, audit trails can be used to track and monitor user activities, system events, and data changes. Audit trails are important for several reasons:
1. Compliance: Audit trails help organizations meet regulatory compliance requirements by providing an auditable record of user actions and system events. This is particularly important in industries such as finance, healthcare, and government where data privacy and security regulations are stringent.
2. Security: Audit trails can help detect and investigate security incidents, unauthorized access attempts, or suspicious activities. By monitoring and analyzing audit trails, organizations can identify potential security vulnerabilities and take necessary actions to mitigate risks.
3. Accountability: Audit trails create a sense of accountability and responsibility among users and system administrators. Users are aware that their actions are being logged and can be traced back, which discourages unauthorized activities or misuse of privileges.
4. Forensics: In the event of a system failure, data breach, or legal dispute, audit trails provide valuable evidence for forensic analysis and investigation. They can help reconstruct the sequence of events leading up to an incident, identify the root cause, and establish liability.
To implement audit trails in a Gin application, you can use middleware to intercept and log relevant events. Here's an example of how to implement audit trails in Gin:
1. Install the necessary packages:
go get github.com/gin-gonic/gingo get github.com/sirupsen/logrus
2. Define a middleware to log requests and responses:
import ( "github.com/gin-gonic/gin" log "github.com/sirupsen/logrus")func main() { router := gin.Default() // Define a middleware to log requests and responses router.Use(func(c *gin.Context) { // Log the request log.WithFields(log.Fields{ "method": c.Request.Method, "path": c.Request.URL.Path, "ip": c.ClientIP(), }).Info("Request received") // Process the request c.Next() // Log the response log.WithFields(log.Fields{ "status": c.Writer.Status(), "size": c.Writer.Size(), }).Info("Response sent") }) // ...}
3. Customize the log format and destination:
import ( "os" "github.com/gin-gonic/gin" log "github.com/sirupsen/logrus")func main() { // Set the log format log.SetFormatter(&log.JSONFormatter{}) // Set the log output log.SetOutput(os.Stdout) // ... // Define a middleware to log requests and responses router.Use(func(c *gin.Context) { // Log the request log.WithFields(log.Fields{ "method": c.Request.Method, "path": c.Request.URL.Path, "ip": c.ClientIP(), }).Info("Request received") // ... }) // ...}
What logging options are available in Gin?
Gin provides various options for logging, allowing developers to customize the log format, destination, and level of verbosity. Here are some logging options available in Gin:
1. Default Logger: Gin comes with a built-in logger that logs request details, such as the HTTP method, URL path, and response status. The default logger can be enabled by calling the gin.Default()
function when creating the Gin router. The default logger outputs logs to the standard output (STDOUT) using a predefined log format.
2. Custom Logger: Developers can replace the default logger with a custom logger by calling the gin.New()
function and providing a custom implementation of the gin.Logger()
middleware. This allows developers to define their own log format, log destination, and additional log fields.
3. Third-party Loggers: Gin can also be integrated with third-party logging libraries, such as Logrus, Zap, or Sirupsen/Logrus. These libraries provide more advanced logging features, such as structured logging, log rotation, and log level configuration. To use a third-party logger with Gin, developers need to replace the default logger with the desired logger implementation.
Here's an example of how to use the Logrus logging library with Gin:
1. Install the necessary packages:
go get github.com/gin-gonic/gingo get github.com/sirupsen/logrus
2. Initialize the Logrus logger:
import ( "github.com/gin-gonic/gin" log "github.com/sirupsen/logrus")func main() { // Initialize the Logrus logger log.SetFormatter(&log.JSONFormatter{}) log.SetLevel(log.InfoLevel) router := gin.Default() // Use the Logrus logger middleware router.Use(func(c *gin.Context) { // Log the request log.WithFields(log.Fields{ "method": c.Request.Method, "path": c.Request.URL.Path, "ip": c.ClientIP(), }).Info("Request received") c.Next() }) // ... router.Run(":8080")}
What are the considerations for data compliance in a Gin application?
Data compliance refers to the adherence of an application to relevant data protection and privacy regulations. When developing a Gin application, there are several considerations to keep in mind to ensure data compliance:
1. Data Encryption: sensitive data should be encrypted both at rest and in transit. In a Gin application, you can use TLS/SSL to encrypt data in transit and encryption algorithms like AES to encrypt data at rest.
2. Access Controls: implement Role-Based Access Control (RBAC) or Attribute-Based Access Control (ABAC) to control access to sensitive data. Ensure that only authorized users or roles have access to sensitive data and that access is logged and audited.
3. User Consent and Permissions: obtain explicit user consent for data collection and processing, and ensure that user permissions are respected. Provide mechanisms for users to view, modify, or delete their data, as required by data protection regulations.
4. Data Retention and Deletion: define data retention policies to determine how long data should be stored and when it should be deleted. Implement mechanisms to automatically delete expired or unnecessary data.
5. Logging and Audit Trails: log and audit user activities, system events, and data changes to ensure accountability and traceability. Store logs securely and ensure that they can be easily accessed and reviewed in case of an audit or investigation.
6. Data Minimization: only collect and store the minimum amount of data necessary for the application's functionality. Avoid collecting sensitive or unnecessary data that could increase the risk of data breaches or non-compliance.
7. Data Transfer: ensure that data transfers comply with data protection regulations by using secure protocols and encryption. Avoid transferring data to jurisdictions with inadequate data protection laws.
8. Third-Party Services: carefully review and assess the security and privacy practices of third-party services or APIs used in the Gin application. Ensure that they comply with relevant data protection regulations.
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
Code snippet: Implementing SSO in Gin using OAuth2.0
// main.gopackage mainimport ( "encoding/json" "net/http" "github.com/gin-gonic/gin" "golang.org/x/oauth2" "golang.org/x/oauth2/google")var config *oauth2.Configfunc main() { router := gin.Default() // Set up the OAuth2.0 configuration config = &oauth2.Config{ ClientID: "YOUR_CLIENT_ID", ClientSecret: "YOUR_CLIENT_SECRET", RedirectURL: "http://localhost:8080/oauth2/callback", Scopes: []string{"email"}, Endpoint: google.Endpoint, } // Redirect the user to the OAuth2.0 login page router.GET("/login", func(c *gin.Context) { url := config.AuthCodeURL("state") c.Redirect(http.StatusTemporaryRedirect, url) }) // Handle the OAuth2.0 callback router.GET("/oauth2/callback", func(c *gin.Context) { code := c.Query("code") // Exchange the authorization code for an access token token, err := config.Exchange(oauth2.NoContext, code) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to exchange authorization code"}) return } // Use the access token to retrieve user information client := config.Client(oauth2.NoContext, token) resp, err := client.Get("https://www.googleapis.com/oauth2/v2/userinfo") if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to retrieve user information"}) return } defer resp.Body.Close() // Parse the user information from the response body var userInfo struct { Email string `json:"email"` } err = json.NewDecoder(resp.Body).Decode(&userInfo) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to parse user information"}) return } // Do something with the user information // ... c.JSON(http.StatusOK, gin.H{"message": "Successfully authenticated"}) }) router.Run(":8080")}
This code snippet demonstrates how to implement Single Sign-On (SSO) in Gin using OAuth2.0. It sets up an OAuth2.0 configuration using the Google OAuth2.0 provider and defines two routes: /login
for redirecting the user to the OAuth2.0 login page, and /oauth2/callback
for handling the OAuth2.0 callback and exchanging the authorization code for an access token. The user's email is then retrieved using the access token and can be used for further processing in the application.
Code snippet: Implementing RBAC in Gin
// main.gopackage mainimport ( "github.com/casbin/casbin" "github.com/casbin/gorm-adapter" "github.com/gin-gonic/gin")var enforcer *casbin.Enforcerfunc main() { // Connect to the database adapter, err := gormadapter.NewAdapter("mysql", "root:password@tcp(127.0.0.1:3306)/") if err != nil { panic("Failed to connect to the database") } // Initialize the Casbin enforcer with the RBAC model and policy enforcer, err = casbin.NewEnforcer("path/to/model.conf", adapter) if err != nil { panic("Failed to initialize Casbin enforcer") } router := gin.Default() // Define a middleware to enforce RBAC router.Use(func(c *gin.Context) { // Get the current user's role from the session or token role := getCurrentUserRole(c) // Get the current request's URL and HTTP method path := c.Request.URL.Path method := c.Request.Method // Check if the current user has permission to access the requested resource allowed, err := enforcer.Enforce(role, path, method) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to enforce RBAC"}) return } if !allowed { c.JSON(http.StatusForbidden, gin.H{"error": "Access denied"}) return } // Continue to the next middleware or handler c.Next() }) // ...}
This code snippet demonstrates how to implement Role-Based Access Control (RBAC) in Gin using the Casbin library. It initializes the Casbin enforcer with an RBAC model and policy stored in a database using the Gorm adapter. A middleware is defined to enforce RBAC by checking if the user's role has permission to access the requested resource. The getCurrentUserRole
function should be implemented to retrieve the current user's role from the session or token.
Code snippet: Integrating Gin with enterprise IAM solutions
// main.gopackage mainimport ( "github.com/gin-gonic/gin")func main() { router := gin.Default() // Define a middleware to authenticate and authorize users using enterprise IAM solutions router.Use(func(c *gin.Context) { // Authenticate the user using the IAM solution user, err := authenticateUser(c) if err != nil { c.JSON(http.StatusUnauthorized, gin.H{"error": "Failed to authenticate user"}) return } // Authorize the user based on their roles and permissions allowed, err := authorizeUser(user, c.Request.URL.Path, c.Request.Method) if err != nil { c.JSON(http.StatusInternalServerError, gin.H{"error": "Failed to authorize user"}) return } if !allowed { c.JSON(http.StatusForbidden, gin.H{"error": "Access denied"}) return } // Continue to the next middleware or handler c.Next() }) // ...}
This code snippet demonstrates how to integrate Gin with an enterprise Identity and Access Management (IAM) solution. A middleware is defined to authenticate and authorize users using the IAM solution. The authenticateUser
function should be implemented to authenticate the user and return their information, and the authorizeUser
function should be implemented to authorize the user based on their roles and permissions.
Code snippet: Implementing audit trails in Gin
// main.gopackage mainimport ( "github.com/gin-gonic/gin" "github.com/sirupsen/logrus")func main() { router := gin.Default() // Define a middleware to log requests and responses router.Use(func(c *gin.Context) { // Log the request logrus.WithFields(logrus.Fields{ "method": c.Request.Method, "path": c.Request.URL.Path, "ip": c.ClientIP(), }).Info("Request received") // Process the request c.Next() // Log the response logrus.WithFields(logrus.Fields{ "status": c.Writer.Status(), "size": c.Writer.Size(), }).Info("Response sent") }) // ...}
This code snippet demonstrates how to implement audit trails in a Gin application. A middleware is defined to log requests and responses using the Logrus logging library. The request details, such as the HTTP method, URL path, and client IP address, are logged when a request is received, and the response details, such as the status code and response size, are logged when a response is sent.
Related Article: Integrating Payment, Voice and Text with Beego & Golang
Code snippet: Implementing logging in Gin
// main.gopackage mainimport ( "os" "github.com/gin-gonic/gin" "github.com/sirupsen/logrus")func main() { // Set the log format logrus.SetFormatter(&logrus.JSONFormatter{}) // Set the log output logrus.SetOutput(os.Stdout) router := gin.Default() // Use the Logrus logger middleware router.Use(func(c *gin.Context) { // Log the request logrus.WithFields(logrus.Fields{ "method": c.Request.Method, "path": c.Request.URL.Path, "ip": c.ClientIP(), }).Info("Request received") c.Next() }) // ...}
This code snippet demonstrates how to implement logging in a Gin application using the Logrus logging library. The Logrus logger is initialized with a JSON formatter and set to output logs to the standard output (STDOUT). A middleware is defined to log requests using the Logrus logger. The request details, such as the HTTP method, URL path, and client IP address, are logged when a request is received.
Code snippet: Addressing data compliance in a Gin application
// main.gopackage mainimport ( "github.com/gin-gonic/gin")func main() { router := gin.Default() // Define a middleware to handle data compliance router.Use(func(c *gin.Context) { // Check if the request contains sensitive data if containsSensitiveData(c) { // Encrypt the sensitive data encryptData(c) } // Continue to the next middleware or handler c.Next() }) // ...}
This code snippet demonstrates how to address data compliance in a Gin application. A middleware is defined to handle data compliance. The containsSensitiveData
function should be implemented to check if the request contains sensitive data, and the encryptData
function should be implemented to encrypt the sensitive data. This middleware can be customized to handle other data compliance requirements, such as data retention, data deletion, or data anonymization.