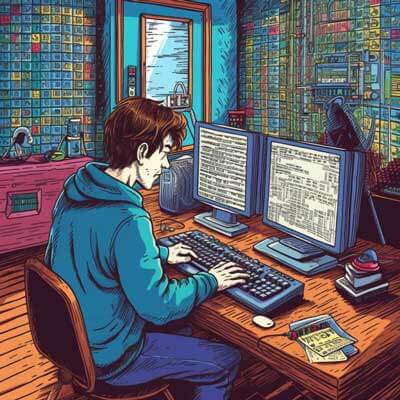
- The Purpose of the Gin Framework
- Handling Complex Route Patterns in Gin
- Understanding the Middleware Mechanism in Gin
- Manipulating the Context in Gin
- State Management in Gin
- Benefits of Using Gin’s Middleware Mechanism
- Examples of Complex Route Patterns in Gin
- Handling Groups in Gin
- Best Practices for Context Manipulation in Gin
- Managing State in Gin
- Additional Resources
The Purpose of the Gin Framework
The Gin framework is a useful web framework written in the Go programming language (Golang). It is designed to be minimalistic and efficient, making it a popular choice for building high-performance web applications and APIs. Gin provides a robust set of features and tools that enable developers to create scalable and reliable applications with ease.
One of the key goals of Gin is to improve developer productivity by offering a simple and intuitive API. It achieves this by leveraging the features of the Go language and providing a clean and expressive syntax. With Gin, developers can focus on building their application logic without getting bogged down by unnecessary complexities.
Gin is also known for its excellent performance. It is built on top of the fasthttp package, which is a high-performance alternative to the standard net/http package in Go. This allows Gin to handle a large number of concurrent requests efficiently, making it suitable for applications that require high throughput.
In addition to its performance and productivity benefits, Gin also offers a range of advanced features that make it a versatile framework for building web applications. These features include a flexible routing system, a middleware mechanism for request processing, custom context manipulation, and state management.
In the following sections, we will explore these advanced features of Gin in more detail, providing examples and code snippets to illustrate their usage and benefits.
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
Handling Complex Route Patterns in Gin
Route patterns are an essential part of any web application framework, as they determine how incoming requests are matched and routed to the appropriate handlers. Gin provides a useful and flexible routing system that allows developers to define complex route patterns with ease.
Gin’s routing system is based on the httprouter package, which is a high-performance router for Go. It supports a wide range of route patterns, including static routes, parameterized routes, and wildcard routes.
Let’s take a look at some examples to understand how complex route patterns can be defined in Gin:
Example 1: Static Route
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/hello", func(c *gin.Context) { c.String(200, "Hello, World!") }) router.Run(":8080") }
In this example, we define a static route using the GET method. When the client sends a GET request to “/hello”, the handler function is executed, and the response “Hello, World!” is returned.
Example 2: Parameterized Route
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "User ID: "+id) }) router.Run(":8080") }
In this example, we define a parameterized route using the colon “:” syntax. When the client sends a GET request to “/users/123”, the handler function is executed, and the response “User ID: 123” is returned. The value of the “id” parameter is extracted using the Param
function.
Example 3: Wildcard Route
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/files/*filepath", func(c *gin.Context) { filepath := c.Param("filepath") c.String(200, "File Path: "+filepath) }) router.Run(":8080") }
In this example, we define a wildcard route using the asterisk “*” syntax. When the client sends a GET request to “/files/path/to/file.txt”, the handler function is executed, and the response “File Path: path/to/file.txt” is returned. The value of the “filepath” parameter is extracted using the Param
function.
Gin’s routing system provides a flexible and expressive way to define complex route patterns. By leveraging the static, parameterized, and wildcard routes, developers can handle a wide range of URL patterns and build useful web applications.
Understanding the Middleware Mechanism in Gin
Middleware is a key concept in web development that allows developers to add logic to the request-response cycle of an application. It provides a way to process requests before they reach the handler functions and modify the response before it is sent back to the client.
Gin’s middleware mechanism is one of its standout features, as it offers a clean and efficient way to handle common tasks such as logging, authentication, error handling, and more. Gin provides a middleware stack that allows developers to chain multiple middleware functions together and apply them to specific routes or groups of routes.
Let’s look at an example to understand how the middleware mechanism works in Gin:
Example 1: Logging Middleware
package main import ( "fmt" "time" "github.com/gin-gonic/gin" ) func Logger() gin.HandlerFunc { return func(c *gin.Context) { start := time.Now() c.Next() end := time.Now() latency := end.Sub(start) fmt.Printf("[%s] %s - %v\n", c.Request.Method, c.Request.URL.Path, latency) } } func main() { router := gin.Default() router.Use(Logger()) router.GET("/hello", func(c *gin.Context) { c.String(200, "Hello, World!") }) router.Run(":8080") }
In this example, we define a custom logging middleware function called Logger
. This middleware function measures the latency of each request and logs the method, URL path, and latency to the console.
We then use the Use
method of the router to apply the Logger
middleware to all routes registered after it. When the client sends a GET request to “/hello”, the Logger
middleware is executed first, followed by the handler function. Finally, the response “Hello, World!” is returned.
Gin’s middleware mechanism provides a flexible and efficient way to add common functionality to an application. By chaining middleware functions together and applying them selectively, developers can easily implement features such as authentication, rate limiting, request validation, and more.
Manipulating the Context in Gin
The context is a fundamental concept in web development that represents the state of an individual request-response cycle. It contains information about the incoming request, such as the request parameters, headers, and body, as well as the outgoing response, such as the response status, headers, and body.
Gin provides a useful mechanism for manipulating the context, allowing developers to modify the request and response objects, add or retrieve values, and control the flow of the request-response cycle.
Let’s explore some examples to understand how context manipulation works in Gin:
Example 1: Adding Values to the Context
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/hello", func(c *gin.Context) { c.Set("name", "John Doe") }) router.GET("/greet", func(c *gin.Context) { name := c.GetString("name") c.String(200, "Hello, "+name+"!") }) router.Run(":8080") }
In this example, we define two routes. The first route, “/hello”, sets a value “name” in the context using the Set
method. The second route, “/greet”, retrieves the value “name” from the context using the GetString
method and returns a personalized greeting.
Example 2: Middleware for Authentication
package main import ( "net/http" "github.com/gin-gonic/gin" ) func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { token := c.GetHeader("Authorization") if token != "mysecrettoken" { c.AbortWithStatus(http.StatusUnauthorized) return } c.Next() } } func main() { router := gin.Default() router.Use(AuthMiddleware()) router.GET("/protected", func(c *gin.Context) { c.String(200, "Protected Resource") }) router.Run(":8080") }
In this example, we define a custom middleware function called AuthMiddleware
. This middleware function checks the “Authorization” header of each request and aborts the request with a status code of 401 (Unauthorized) if the token is invalid.
We then use the Use
method of the router to apply the AuthMiddleware
to all routes registered after it. When the client sends a GET request to “/protected”, the AuthMiddleware
is executed first, followed by the handler function. If the token is valid, the response “Protected Resource” is returned; otherwise, a 401 Unauthorized status is returned.
Gin’s context manipulation capabilities provide a useful way to customize the behavior of an application based on the state of each request. By adding and retrieving values from the context, as well as controlling the flow with middleware, developers can implement features such as authentication, request validation, and more.
Related Article: Integrating Payment, Voice and Text with Beego & Golang
State Management in Gin
State management is an important aspect of web development, as it allows developers to persist data across multiple requests and provide a personalized experience to each user. Gin provides a flexible and efficient way to manage state using a combination of context manipulation, middleware, and external storage solutions.
Let’s explore some examples to understand how state management works in Gin:
Example 1: Session Management with Cookies
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/login", func(c *gin.Context) { // Perform authentication logic... c.SetCookie("session_id", "123", 3600, "/", "", false, true) c.String(200, "Logged in successfully") }) router.GET("/dashboard", func(c *gin.Context) { sessionId, err := c.Cookie("session_id") if err != nil || sessionId != "123" { c.AbortWithStatus(401) return } c.String(200, "Welcome to the dashboard") }) router.Run(":8080") }
In this example, we define two routes. The first route, “/login”, performs the authentication logic and sets a session ID cookie using the SetCookie
method. The second route, “/dashboard”, retrieves the session ID from the cookie using the Cookie
method and checks if it is valid. If the session ID is valid, the response “Welcome to the dashboard” is returned; otherwise, a 401 Unauthorized status is returned.
Example 2: Using External Storage for State
package main import ( "github.com/gin-gonic/gin" ) type User struct { ID int Name string } var users = map[int]User{ 1: {ID: 1, Name: "John"}, 2: {ID: 2, Name: "Jane"}, } func main() { router := gin.Default() router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") userID := c.GetInt("user_id") user, ok := users[userID] if !ok { c.AbortWithStatus(404) return } c.JSON(200, user) }) router.Use(func(c *gin.Context) { userID := c.Query("user_id") c.Set("user_id", userID) c.Next() }) router.Run(":8080") }
In this example, we define a route that retrieves user information based on the user ID. We use an external storage solution, such as a database or cache, to store the user data. The user ID is passed as a query parameter, and we use the Set
method to store it in the context.
We then define a middleware function that retrieves the user ID from the query parameter and sets it in the context. This allows us to access the user ID in the route handler and retrieve the corresponding user data from the external storage.
Gin’s state management capabilities provide a flexible and efficient way to manage user-specific data and provide a personalized experience to each user. By leveraging cookies, external storage solutions, and context manipulation, developers can implement features such as authentication, session management, and user-specific data retrieval.
Benefits of Using Gin’s Middleware Mechanism
Gin’s middleware mechanism offers several benefits that make it a useful tool for building web applications:
1. Code Reusability: Middleware functions can be defined once and applied to multiple routes or groups of routes. This promotes code reusability and reduces code duplication.
2. Modularity: Middleware functions can be easily chained together and applied in any order. This allows developers to create modular and composable middleware stacks to handle different aspects of the request-response cycle.
3. Separation of Concerns: Middleware functions allow developers to separate cross-cutting concerns, such as authentication, logging, and error handling, from the core business logic of the application. This promotes clean and maintainable code.
4. Flexibility: Gin’s middleware mechanism provides a fine-grained control over the request-response cycle. Developers can choose to apply middleware to specific routes or groups of routes, allowing for highly customizable behavior.
5. Performance: Gin’s middleware mechanism is designed to be efficient and lightweight. Middleware functions are executed in the order they are defined, and the overhead introduced by the middleware stack is minimal.
Examples of Complex Route Patterns in Gin
Gin’s routing system supports a wide range of route patterns, allowing developers to handle complex URL structures with ease. Here are some examples of complex route patterns that can be achieved using Gin:
Example 1: Matching Multiple Routes with the Same Handler
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() router.GET("/users", func(c *gin.Context) { c.String(200, "Get all users") }) router.POST("/users", func(c *gin.Context) { c.String(200, "Create a new user") }) router.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "Get user with ID: "+id) }) router.PUT("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "Update user with ID: "+id) }) router.DELETE("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "Delete user with ID: "+id) }) router.Run(":8080") }
In this example, we define multiple routes for handling user-related operations. The /users
route matches both GET and POST requests and is used for retrieving all users and creating a new user, respectively. The /users/:id
route matches GET, PUT, and DELETE requests and is used for retrieving, updating, and deleting a specific user.
Example 2: Nested Route Groups
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() v1 := router.Group("/v1") { v1.GET("/users", func(c *gin.Context) { c.String(200, "Get all users (v1)") }) v1.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "Get user with ID: "+id+" (v1)") }) } v2 := router.Group("/v2") { v2.GET("/users", func(c *gin.Context) { c.String(200, "Get all users (v2)") }) v2.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "Get user with ID: "+id+" (v2)") }) } router.Run(":8080") }
In this example, we define two nested route groups, /v1
and /v2
, for versioning our API. Each group has its own set of routes for handling user-related operations. The /users
route within each group is used for retrieving all users, while the /users/:id
route is used for retrieving a specific user.
Gin’s routing system allows developers to handle complex route patterns with ease, providing a clean and intuitive syntax for defining routes and handling different HTTP methods. By using static routes, parameterized routes, and route groups, developers can build useful web applications that can handle a wide range of URL structures.
Related Article: Integrating Beego & Golang Backends with React.js
Handling Groups in Gin
Gin provides a useful mechanism for handling route groups, allowing developers to define common behavior and middleware that should be applied to a set of routes. Route groups promote code reusability, maintainability, and allow for a more organized structure in large web applications.
Let’s explore an example to understand how to handle groups in Gin:
Example: Grouping Routes with Common Middleware
package main import ( "github.com/gin-gonic/gin" ) func Logger() gin.HandlerFunc { return func(c *gin.Context) { println("Logging middleware") c.Next() } } func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { println("Authentication middleware") c.Next() } } func main() { router := gin.Default() api := router.Group("/api") api.Use(Logger(), AuthMiddleware()) { api.GET("/users", func(c *gin.Context) { c.String(200, "Get all users") }) api.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "Get user with ID: "+id) }) } admin := router.Group("/admin") admin.Use(Logger(), AuthMiddleware()) { admin.GET("/users", func(c *gin.Context) { c.String(200, "Admin: Get all users") }) admin.GET("/users/:id", func(c *gin.Context) { id := c.Param("id") c.String(200, "Admin: Get user with ID: "+id) }) } router.Run(":8080") }
In this example, we define two route groups, /api
and /admin
, to handle different sets of routes. We use the Group
method of the router to create the groups, and the Use
method to add common middleware to each group.
The Logger
middleware logs a message before and after each request, while the AuthMiddleware
middleware performs authentication logic. By using the Use
method on the groups, we ensure that the middleware is applied to all routes within that group.
Within each group, we define routes for handling user-related operations. The routes within the /api
group are used for retrieving users, while the routes within the /admin
group are used for retrieving users with additional administrative privileges.
Best Practices for Context Manipulation in Gin
Context manipulation is a useful feature of the Gin framework that allows developers to modify the request and response objects, add or retrieve values, and control the flow of the request-response cycle. To ensure clean and maintainable code, it is important to follow best practices when manipulating the context in Gin.
Here are some best practices for context manipulation in Gin:
1. Avoid Global State: While Gin’s context allows for easy sharing of data across middleware and handlers, it is generally best to avoid using global state. Instead, use the context to pass data between middleware and handlers, or consider using external storage solutions for persistent state.
2. Keep Middleware Lightweight: Middleware functions should be lightweight and focused on a specific task. Avoid adding unnecessary logic or complex operations in the middleware, as it can impact performance and readability.
3. Use Context Values for Temporary State: The Set
and Get
methods of the context can be used to store temporary values that are specific to the current request. Use these methods sparingly and only for small amounts of data that are needed within the request-response cycle.
4. Avoid Context Pollution: Be mindful of the number of values stored in the context. Adding too many values to the context can make the code harder to understand and maintain. Consider using a struct or custom types to encapsulate related data and reduce the number of context keys.
5. Separate Concerns with Middleware: Use middleware to separate cross-cutting concerns, such as authentication, logging, and error handling, from the core business logic of the application. This promotes clean and maintainable code by keeping each middleware focused on a specific task.
6. Handle Errors Gracefully: When manipulating the context, be sure to handle errors gracefully. Use the Abort
and AbortWithStatus
methods to stop the execution of the current request and return appropriate error responses when necessary.
Managing State in Gin
State management is an essential aspect of web application development, as it allows developers to persist data across multiple requests and provide a personalized experience to each user. While Gin does not provide built-in state management solutions, it offers a range of tools and techniques that can be used to manage state effectively.
Here are some approaches for managing state in Gin:
1. Cookies: Cookies can be used to store small amounts of data on the client-side. Gin provides convenient methods, such as SetCookie
and Cookie
, to work with cookies. Cookies are suitable for storing session IDs, user preferences, and other small pieces of information.
2. Database: Gin can be easily integrated with databases to store and retrieve persistent data. Developers can use popular database libraries, such as GORM or sqlx, to interact with databases and manage state. Database solutions are suitable for managing user profiles, settings, and other data that needs to be persisted.
3. Caching: Caching can be used to store frequently accessed or computationally expensive data in memory. Gin can be integrated with caching solutions, such as Redis or Memcached, to improve performance and reduce the load on databases. Caching is suitable for storing frequently accessed data, such as user sessions or API responses.
4. External APIs: External APIs can be used to manage state that is not directly stored within the application. For example, developers can use third-party services, such as Firebase or AWS, to store and retrieve data. External APIs are suitable for managing user authentication, file storage, and other services that require external resources.
5. Context Manipulation: As discussed earlier, Gin’s context allows for easy manipulation of the request and response objects. Developers can use the Set
and Get
methods to store and retrieve data within the context. Context manipulation is suitable for passing data between middleware and handlers or managing temporary state within the request-response cycle.
When managing state in Gin, it is important to consider security, performance, and scalability. Developers should ensure that sensitive data is properly protected, that performance is optimized through proper caching and database indexing, and that the chosen state management approach can scale with the application’s growth.
Conclusion
In this article, we explored the advanced features of the Gin framework in Go. We learned about handling complex route patterns, understanding the middleware mechanism, manipulating the context, and managing state in Gin. We also discussed the benefits of using Gin’s middleware mechanism, provided examples of complex route patterns, and explained best practices for context manipulation and state management.
Gin is a useful and efficient framework for building web applications and APIs in Go. With its rich set of features and tools, Gin enables developers to create scalable and reliable applications with ease. Whether you’re building a small API or a large-scale web application, Gin provides the flexibility and performance you need to succeed.
Related Article: Handling Large Data Volume with Golang & Beego
Additional Resources
– Gin – Official Website
– Gin – GitHub Repository
– Gin – Middleware