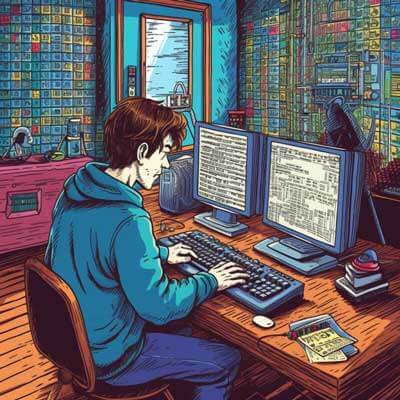
Table of Contents
Installing Golang on your machine
To get started with Golang for backend development, the first step is to install the Go programming language on your machine. In this chapter, we will walk you through the installation process for different operating systems.
Windows:
1. Visit the official Golang website at https://golang.org/dl/.
2. Download the installer suitable for your Windows version (32-bit or 64-bit).
3. Run the installer and follow the installation wizard.
4. Choose the desired installation location (by default, it will be C:\Go).
5. Make sure to check the box "Add Go to PATH" during the installation process. This will enable you to use Go from any command prompt.
6. Click "Next" and then "Install" to complete the installation.
7. Open a new command prompt and verify the installation by running the following command:
go version
You should see the installed Go version printed in the console.
macOS:
1. Open a web browser and go to the official Golang website at https://golang.org/dl/.
2. Download the macOS installer (.pkg file) for the latest stable release.
3. Open the downloaded file and follow the installation wizard.
4. Choose the destination disk and click "Install" to start the installation process.
5. After the installation is complete, open the Terminal application.
6. Verify the installation by running the following command:
go version
If the installation was successful, you will see the installed Go version displayed in the terminal.
Linux:
1. Open a web browser and navigate to the official Golang website at https://golang.org/dl/.
2. Download the Linux tarball (.tar.gz) file for the latest stable release.
3. Open a terminal and navigate to the directory where you downloaded the tarball file.
4. Extract the tarball using the following command:
tar -xvf go.tar.gz
5. Move the extracted Go directory to the desired location. For example, you can move it to /usr/local:
sudo mv go /usr/local
6. Add Go to the PATH environment variable by appending the following line to the ~/.profile or ~/.bashrc file:
export PATH=$PATH:/usr/local/go/bin
Save the file and apply the changes by running the following command:
source ~/.profile
or
source ~/.bashrc
7. Verify the installation by opening a new terminal and running the following command:
go version
If the installation was successful, you will see the installed Go version displayed in the terminal.
Congratulations! You have successfully installed Go on your machine. In the next chapter, we will explore the Go workspace and project structure.
Related Article: Handling Large Data Volume with Golang & Beego
Setting up your development environment
To get started with Golang for backend development, you will need to set up your development environment. This includes installing Go and configuring your workspace.
Installing Go
Before you can start coding in Go, you need to install the Go programming language on your machine. Go to the official Go website at https://golang.org/ and download the latest stable release for your operating system. Follow the installation instructions for your specific OS.
Once the installation is complete, you can verify that Go is installed correctly by opening a terminal or command prompt and running the following command:
go version
If Go is installed properly, you should see the version number displayed in the output.
Configuring your workspace
After installing Go, you need to set up your workspace. The workspace is the directory where you will store your Go source code and other related files.
1. Choose the directory where you want to set up your workspace. This directory can be anywhere on your machine.
2. Create a new directory for your workspace. You can name it anything you like. For example, you can create a directory called "go-workspace".
3. Set the
GOPATH
environment variable to the path of your workspace directory. This tells Go where to find your source code and package dependencies. You can set theGOPATH
variable by running the following command in your terminal or command prompt:
export GOPATH=/path/to/your/workspace
Replace "/path/to/your/workspace" with the actual path to your workspace directory.
4. Optionally, add the Go binary directory to your system's
PATH
environment variable. This allows you to run Go commands from any directory without specifying the full path to the Go binary. To do this, add the following line to your shell startup file (e.g.,.bashrc
or.bash_profile
):
export PATH=$PATH:/path/to/your/workspace/bin
Replace "/path/to/your/workspace" with the actual path to your workspace directory.
Related Article: Enterprise Functionalities in Golang: SSO, RBAC and Audit Trails in Gin
Creating your first Go project
With your development environment set up, you can now create your first Go project. In your workspace directory, create a new directory for your project. For example, you can create a directory called "myapp".
Inside the project directory, create a new file called "main.go". This will be the entry point for your Go application. Open the "main.go" file in a text editor and add the following code:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
Save the file and return to your terminal or command prompt. Navigate to your project directory and run the following command to build and run your Go application:
go run main.go
You should see the output "Hello, World!" printed in the terminal.
Congratulations! You have successfully set up your development environment and created your first Go project. You are now ready to start building backend applications with Golang.
Understanding the basics of Golang
Go, also known as Golang, is a statically typed, compiled programming language designed for building efficient and reliable software. It was created at Google by Robert Griesemer, Rob Pike, and Ken Thompson and was publicly announced in 2009. Go is gaining popularity among developers due to its simplicity, performance, and built-in support for concurrency.
Features of Golang
Go incorporates several features that make it a powerful language for backend development:
1. Simplicity: Go has a simple and easy-to-understand syntax, making it a beginner-friendly language. It emphasizes readability and reduces the cognitive load on developers.
2. Efficiency: Go is designed to be highly efficient, both in terms of memory usage and runtime performance. It compiles to machine code, resulting in faster execution times compared to interpreted languages.
3. Concurrency: Go has built-in support for concurrency, making it easy to write concurrent programs. Goroutines and channels are two powerful abstractions that enable developers to write efficient and scalable concurrent code.
4. Garbage Collection: Go has an automatic garbage collector that manages memory allocation and deallocation, relieving developers from manual memory management tasks.
5. Static Typing: Go is a statically typed language, which means that variables must be declared with their types at compile-time. This promotes early error detection and improves code reliability.
Installing Go
To get started with Go, you need to install the Go programming language on your machine. Go provides official distributions for different operating systems, which can be downloaded from the official Go website: https://golang.org/dl/.
Once downloaded, follow the installation instructions specific to your operating system. After successful installation, you can verify the installation by opening a terminal or command prompt and running the following command:
go version
This command should display the installed Go version, confirming that the installation was successful.
Related Article: Integrating Beego & Golang Backends with React.js
Your First Go Program
Let's write a simple "Hello, World!" program to get acquainted with Go:
Create a new file called
hello.go
and open it in a text editor. Add the following code to the file:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
Save the file and open a terminal or command prompt in the same directory as the
hello.go
file. Run the following command to compile and execute the program:
go run hello.go
You should see the output
Hello, World!
printed to the console.
Congratulations! You have written and executed your first Go program.
Go Development Tools
Go provides several development tools to enhance productivity and streamline the development process. Some popular tools include:
- go build: This command compiles Go source code into an executable binary file.
- go test: This command runs tests written in Go and reports the test results.
- go fmt: This command formats Go source code according to the official Go formatting guidelines.
- go mod: This command manages dependencies for your Go projects.
These tools are included in the Go distribution and can be accessed via the command line.
Working with variables and data types
In Go, variables are used to store values of different data types. Before using a variable, you need to declare it with a specific data type. Go supports various data types such as integers, floats, strings, booleans, and more.
To declare a variable in Go, you need to use the
var
keyword followed by the variable name and its data type. Here's an example that declares an integer variable:
var age int
In this example, we declared a variable named
age
with the data typeint
. By default, the value of an uninitialized variable in Go is the zero value of its data type. For integers, the zero value is0
.
You can also initialize a variable during declaration by assigning a value to it. Here's an example that declares and initializes a string variable:
var name string = "John"
In this example, we declared a variable named
name
of typestring
and assigned the value "John" to it.
Go also provides a shorthand syntax for variable declaration and initialization. You can omit the data type and let the Go compiler infer it from the assigned value. Here's an example:
age := 30
In this example, we declared and initialized the
age
variable with the value30
. The Go compiler automatically inferred the data type asint
based on the assigned value.
Go also supports type conversion, which allows you to convert variables from one type to another. Here's an example that converts an integer to a string:
age := 30 ageAsString := strconv.Itoa(age)
In this example, we used the
strconv.Itoa
function to convert theage
variable from an integer to a string.
Go provides several built-in data types, including:
- Integers:
int
,int8
,int16
,int32
,int64
,uint
,uint8
,uint16
,uint32
,uint64
- Floating-point numbers:
float32
,float64
- Strings:
string
- Booleans:
bool
- Complex numbers:
complex64
,complex128
You can also create your own custom data types using the
type
keyword. Custom data types allow you to define a new type based on an existing one. Here's an example that creates a custom data type namedPerson
based on a struct:
type Person struct { name string age int }
In this example, we defined a new data type called
Person
that has two fields:name
of typestring
andage
of typeint
.
Understanding variables and data types is essential for any Go developer. They allow you to store and manipulate data in your programs effectively. In the next chapter, we will explore control flow statements in Go.
Using control flow statements in Golang
Control flow statements allow you to control the execution flow of your program based on certain conditions. In Golang, there are several control flow statements that you can use to make decisions, loop through collections, and handle errors.
Related Article: Handling Large Volumes of Data with Golang & Gin
If statement
The
if
statement allows you to execute a block of code if a certain condition is true. Here's an example:
package main import "fmt" func main() { age := 25 if age >= 18 { fmt.Println("You are an adult") } else { fmt.Println("You are a minor") } }
In this example, the program checks if the
age
variable is greater than or equal to 18. If the condition is true, it prints "You are an adult", otherwise it prints "You are a minor".
Switch statement
The
switch
statement allows you to choose from multiple options based on the value of an expression. Here's an example:
package main import "fmt" func main() { day := "Monday" switch day { case "Monday": fmt.Println("It's Monday!") case "Tuesday": fmt.Println("It's Tuesday!") default: fmt.Println("It's another day") } }
In this example, the program checks the value of the
day
variable and executes the corresponding case. If none of the cases match, it executes thedefault
case.
For loop
The
for
loop allows you to iterate over a collection or execute a block of code repeatedly. There are three different ways to use thefor
loop in Golang.
1. The
for
loop with a single condition:
package main import "fmt" func main() { i := 0 for i < 5 { fmt.Println(i) i++ } }
In this example, the program prints the value of
i
and increments it untili
is no longer less than 5.
2. The
for
loop with an initialization, condition, and post statement:
package main import "fmt" func main() { for i := 0; i < 5; i++ { fmt.Println(i) } }
In this example, the program initializes
i
to 0, executes the loop as long asi
is less than 5, and incrementsi
after each iteration.
3. The
for
loop with a range:
package main import "fmt" func main() { numbers := []int{1, 2, 3, 4, 5} for index, value := range numbers { fmt.Printf("Index: %d, Value: %d\n", index, value) } }
In this example, the program iterates over each element in the
numbers
slice and prints its index and value.
Control flow statements in practice
Control flow statements are commonly used in real-world scenarios. For example, you can use the
if
statement to handle errors returned by functions. Here's an example:
package main import ( "fmt" "os" ) func main() { file, err := os.Open("myfile.txt") if err != nil { fmt.Println("Error:", err) } else { fmt.Println("File opened successfully") // Do something with the file file.Close() } }
In this example, the program tries to open a file called "myfile.txt". If an error occurs, it prints the error message. Otherwise, it prints "File opened successfully" and continues with the file operations.
Control flow statements are powerful tools that allow you to write flexible and efficient code. Understanding how to use them effectively is essential for backend development in Golang.
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
Exploring functions and packages in Golang
In this chapter, we will dive deeper into functions and packages in Golang. Functions are a fundamental building block of any programming language, and packages are a way to organize and reuse code in Golang.
Functions
In Golang, a function is defined using the
func
keyword, followed by the function name, a list of parameters enclosed in parentheses, and an optional return type. Here's an example of a simple function that takes in two integers and returns their sum:
func add(x int, y int) int { return x + y }
The
add
function takes two parameters,x
andy
, both of typeint
, and returns their sum as anint
. Functions can have multiple parameters and return multiple values.
Functions can also have named return values. In this case, the return values are defined as part of the function signature. Here's an example:
func divide(x, y float64) (result float64, err error) { if y == 0 { return 0, errors.New("division by zero") } return x / y, nil }
In this example, the
divide
function takes two float64 parameters and returns both the result of the division and an error if the divisor is zero.
Packages
Packages in Golang are used to organize code into reusable units. A package is a collection of Go source files that reside in the same directory and share the same package name. Packages can be imported and used in other Go programs.
To use a package in your Go program, you need to import it using the
import
keyword. Here's an example of importing thefmt
package, which provides functions for formatted I/O:
import "fmt" func main() { fmt.Println("Hello, world!") }
In this example, we import the
fmt
package and then use thePrintln
function from that package to print the string "Hello, world!" to the console.
Golang provides a standard library with many useful packages for common tasks such as string manipulation, networking, and file I/O. You can also create your own packages to organize your code and make it reusable across multiple projects.
To create a package, you need to create a new directory with the package name and place the Go source files inside it. Each source file should start with a package declaration that specifies the package name.
For example, if you want to create a package called
mathutil
with a function to calculate the factorial of a number, you would create a directory namedmathutil
and place a file namedfactorial.go
inside it with the following contents:
package mathutil func Factorial(n int) int { if n <= 0 { return 1 } return n * Factorial(n-1) }
Now you can import and use the
mathutil
package in your Go programs.
In this chapter, we explored functions and packages in Golang. Functions are defined using the
func
keyword and can have parameters and return values. Packages are used to organize and reuse code in Golang, and they can be imported and used in other Go programs.
Understanding error handling in Golang
Error handling is an essential aspect of software development, as it allows developers to handle unexpected situations and gracefully recover from errors. In Golang, error handling is done using the built-in error type and the error interface.
In Golang, an error is represented by the
error
type, which is an interface with a single method calledError() string
. This method returns a string that describes the error. To create an error, you can use theerrors.New()
function, which takes a string as a parameter and returns an error object.
Here's an example of how to create and handle an error in Golang:
package main import ( "errors" "fmt" ) func divide(a, b float64) (float64, error) { if b == 0 { return 0, errors.New("division by zero") } return a / b, nil } func main() { result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Result:", result) }
In this example, the
divide()
function performs a division operation. If the divisorb
is zero, it returns an error using theerrors.New()
function. In themain()
function, we calldivide()
with 10 and 0 as arguments. If an error occurs, the error object is not nil, and we handle the error by printing its message. Otherwise, we print the result.
Golang also provides a shorthand syntax for error handling using the
:=
operator. This syntax combines the assignment and error checking into a single line. Here's an example:
result, err := divide(10, 0) if err != nil { fmt.Println("Error:", err) return }
In this example, the result of the
divide()
function is assigned to theresult
variable, and any error is assigned to theerr
variable. Iferr
is not nil, we handle the error.
Golang also allows you to define custom error types by implementing the
Error() string
method on a custom struct type. This can be useful when you want to provide more context or additional information about an error. Here's an example:
package main import ( "fmt" ) type MyError struct { message string code int } func (e *MyError) Error() string { return fmt.Sprintf("Error: %s (code: %d)", e.message, e.code) } func main() { err := &MyError{"Something went wrong", 500} fmt.Println(err) }
In this example, we define a custom error type
MyError
with two fields:message
andcode
. We implement theError()
method, which returns a formatted string with the error message and code. In themain()
function, we create an instance ofMyError
and print it.
By understanding error handling in Golang and using the built-in error type and error interface effectively, you can write robust and reliable backend applications.
Related Article: Best Practices for Building Web Apps with Beego & Golang
Working with structs and interfaces
In Go, structs are composite data types that allow you to group together zero or more values with different types into a single entity. They are similar to classes in object-oriented programming languages. Structs are commonly used to represent real-world entities or concepts.
To define a struct, you use the
type
keyword followed by the name of the struct and the definition of its fields. Here's an example of a struct representing a person:
type Person struct { Name string Age int }
In the above example, we define a struct called
Person
with two fields:Name
of type string andAge
of type int.
You can create an instance of a struct by using the struct literal syntax. Here's an example of creating a
Person
struct:
p := Person{ Name: "John Doe", Age: 30, }
In the above example, we create a new
Person
struct with the name "John Doe" and age 30.
You can access the fields of a struct using the dot notation. Here's an example of accessing the fields of the
Person
struct:
fmt.Println(p.Name) // Output: John Doe fmt.Println(p.Age) // Output: 30
Go also supports anonymous structs, which are structs without a defined name. Anonymous structs are useful when you need to create a struct just for temporary use. Here's an example of an anonymous struct:
p := struct { Name string Age int }{ Name: "Jane Smith", Age: 25, }
Interfaces in Go provide a way to define a set of methods that a type must implement. They allow you to define behavior without specifying the actual implementation. Interfaces are a powerful tool for writing modular and reusable code.
To define an interface, you use the
type
keyword followed by the name of the interface and the list of method signatures. Here's an example of an interface calledShape
:
type Shape interface { Area() float64 Perimeter() float64 }
In the above example, we define an interface called
Shape
with two methods:Area()
andPerimeter()
, both returningfloat64
.
Any type that implements all the methods of an interface is said to satisfy that interface. Here's an example of a
Rectangle
type that satisfies theShape
interface:
type Rectangle struct { Width float64 Height float64 } func (r Rectangle) Area() float64 { return r.Width * r.Height } func (r Rectangle) Perimeter() float64 { return 2 * (r.Width + r.Height) }
In the above example, the
Rectangle
type defines theArea()
andPerimeter()
methods, satisfying theShape
interface.
You can create a variable of the interface type and assign a value that satisfies the interface to it. Here's an example:
var s Shape = Rectangle{Width: 10, Height: 5}
In the above example, we create a variable
s
of typeShape
and assign aRectangle
value to it.
You can call the methods of the interface using the variable of the interface type. Here's an example:
fmt.Println(s.Area()) // Output: 50 fmt.Println(s.Perimeter()) // Output: 30
In the above example, we call the
Area()
andPerimeter()
methods of theShape
interface using thes
variable.
Interfaces in Go are a powerful feature that allows you to write flexible and modular code. They enable you to define behavior without being tied to a specific implementation.
Writing tests for your Golang code
Writing tests is an essential part of developing any software project, and the same applies to Golang. In this chapter, we will explore how to write tests for your Golang code, ensuring the quality and reliability of your backend applications.
Golang has a built-in testing package called "testing" that provides a framework for writing and running tests. This package includes various functions and utilities to help you write unit tests, benchmark tests, and more.
To write tests in Golang, you need to create a separate file with a "_test.go" suffix for each package you want to test. This convention allows the Go compiler to treat these files as test files and exclude them from the production build.
Let's consider an example where we have a simple function called "Add" which adds two integers together. We want to write a test for this function to ensure it behaves as expected.
In a file named "math_test.go", we can write the following test:
package math import "testing" func TestAdd(t *testing.T) { result := Add(2, 3) expected := 5 if result != expected { t.Errorf("Add(2, 3) = %d; expected %d", result, expected) } }
In this test, we import the "testing" package and define a function named "TestAdd" with a parameter of type "testing.T". This function represents our test case.
Inside the test function, we call the "Add" function with the inputs we want to test and store the result in a variable called "result". We also define the expected result in a variable called "expected".
We then use the "if" statement to compare the "result" and "expected" values. If they are not equal, we use the "t.Errorf" function to report an error, including the actual and expected values in the error message.
To run the tests, we can use the "go test" command in the terminal, specifying the package we want to test:
go test ./...
This command will run all the tests in the current directory and its subdirectories.
Golang also provides various testing utilities and functions to help you write more complex tests, such as table-driven tests, subtests, and test coverage analysis. You can explore these features in the official Golang documentation: https://golang.org/pkg/testing/.
Writing tests for your Golang code ensures that your backend applications are reliable and maintainable. It helps catch bugs early on and gives you confidence in the correctness of your code.
Using Golang for file handling and I/O operations
Golang provides a powerful set of tools and packages for file handling and I/O operations. Whether you need to read or write files, perform file operations such as renaming or deleting, or work with streams of data, Golang has you covered.
Reading a file
To read the contents of a file in Golang, you can use the
os
package. Here's an example of how to read a file and print its contents:
package main import ( "fmt" "io/ioutil" ) func main() { data, err := ioutil.ReadFile("filename.txt") if err != nil { fmt.Println("Error reading file:", err) return } fmt.Println(string(data)) }
In the example above, we use the
ReadFile
function from theioutil
package to read the contents of the file named "filename.txt". The function returns a byte slice that we convert to a string usingstring(data)
. If there is an error reading the file, we print an error message.
Related Article: Design Patterns with Beego & Golang
Writing to a file
To write to a file in Golang, you can use the
os
package along with theWriteFile
function from theioutil
package. Here's an example of how to write a string to a file:
package main import ( "fmt" "io/ioutil" ) func main() { data := []byte("Hello, Golang!") err := ioutil.WriteFile("output.txt", data, 0644) if err != nil { fmt.Println("Error writing file:", err) return } fmt.Println("File written successfully.") }
In the example above, we use the
WriteFile
function to write the byte slicedata
to a file named "output.txt". The third argument is the file permission mode, which is set to0644
in this case. If there is an error writing the file, we print an error message.
File operations
Golang provides several functions for performing file operations such as renaming or deleting files. Here are a few examples:
- To rename a file, you can use the
os.Rename
function:
err := os.Rename("oldname.txt", "newname.txt") if err != nil { fmt.Println("Error renaming file:", err) }
- To delete a file, you can use the
os.Remove
function:
err := os.Remove("filename.txt") if err != nil { fmt.Println("Error deleting file:", err) }
Working with streams
In addition to reading and writing files, Golang also provides packages for working with streams of data. The
bufio
package, for example, offers buffered I/O operations that can improve performance when working with large amounts of data.
Here's an example of how to read a file line by line using
bufio.Scanner
:
package main import ( "bufio" "fmt" "os" ) func main() { file, err := os.Open("filename.txt") if err != nil { fmt.Println("Error opening file:", err) return } defer file.Close() scanner := bufio.NewScanner(file) for scanner.Scan() { fmt.Println(scanner.Text()) } if err := scanner.Err(); err != nil { fmt.Println("Error reading file:", err) } }
In the example above, we open the file using
os.Open
and create a newbufio.Scanner
to read it line by line. We then iterate over each line usingscanner.Scan()
and print the line usingscanner.Text()
. Finally, we check for any errors usingscanner.Err()
.
Golang provides a rich set of tools for file handling and I/O operations, making it a great choice for backend development. Whether you need to read or write files, perform file operations, or work with streams of data, Golang's standard library has you covered.
Working with databases using Golang
Golang provides excellent support for working with databases, making it a popular choice for backend development. In this chapter, we will explore how to connect to databases, perform CRUD operations, and handle errors using Golang.
Related Article: Golang & Gin Security: JWT Auth, Middleware, and Cryptography
Connecting to a database
Before we can start working with databases in Golang, we need to establish a connection. Golang provides a standard package called
database/sql
that allows us to connect to various database systems such as MySQL, PostgreSQL, SQLite, and more.
Let's take a look at an example of connecting to a MySQL database:
package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "username:password@tcp(localhost:3306)/database_name") if err != nil { log.Fatal(err) } defer db.Close() // Test the connection err = db.Ping() if err != nil { log.Fatal(err) } fmt.Println("Successfully connected to the database!") }
In the code above, we import the necessary packages and open a connection to a MySQL database using the
sql.Open
function. We then test the connection using thePing
method. If everything goes well, the output will indicate a successful connection.
Performing CRUD operations
Once we have established a connection to the database, we can perform CRUD (Create, Read, Update, Delete) operations on the data. Golang provides the
database/sql
package with methods to execute SQL queries and statements.
Let's see an example of inserting data into a MySQL database:
package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) type User struct { ID int Name string Age int } func main() { db, err := sql.Open("mysql", "username:password@tcp(localhost:3306)/database_name") if err != nil { log.Fatal(err) } defer db.Close() // Insert a new user result, err := db.Exec("INSERT INTO users (name, age) VALUES (?, ?)", "John Doe", 30) if err != nil { log.Fatal(err) } lastInsertID, _ := result.LastInsertId() fmt.Println("Inserted user with ID:", lastInsertID) }
In the code above, we define a
User
struct to represent the data we want to insert. We then use thedb.Exec
method to execute an SQL statement to insert a new user into theusers
table. TheLastInsertId
method returns the ID of the last inserted row.
Handling errors
When working with databases, it's crucial to handle errors properly. Golang's
database/sql
package provides methods to handle errors returned from database operations.
Let's take a look at an example of error handling when querying data from a MySQL database:
package main import ( "database/sql" "fmt" "log" _ "github.com/go-sql-driver/mysql" ) type User struct { ID int Name string Age int } func main() { db, err := sql.Open("mysql", "username:password@tcp(localhost:3306)/database_name") if err != nil { log.Fatal(err) } defer db.Close() // Query users rows, err := db.Query("SELECT id, name, age FROM users") if err != nil { log.Fatal(err) } defer rows.Close() var users []User for rows.Next() { var user User err := rows.Scan(&user.ID, &user.Name, &user.Age) if err != nil { log.Fatal(err) } users = append(users, user) } if err := rows.Err(); err != nil { log.Fatal(err) } for _, user := range users { fmt.Println(user.ID, user.Name, user.Age) } }
In the code above, we use the
db.Query
method to execute an SQL statement to retrieve data from theusers
table. We then iterate over the rows returned by the query and scan the values into aUser
struct. Any errors that occur during the iteration are logged usinglog.Fatal
. Finally, we check for any additional errors usingrows.Err()
.
By following these practices, you can effectively handle errors and ensure the reliability of your database operations in Golang.
This concludes our exploration of working with databases using Golang. In the next chapter, we will delve into testing your Golang backend applications. Stay tuned!
Building RESTful APIs with Golang
When it comes to building modern web applications, the use of RESTful APIs has become a standard approach. RESTful APIs allow different clients, such as web browsers or mobile applications, to communicate with a server using the HTTP protocol. In this chapter, we will explore how to build RESTful APIs using Golang.
Golang, also known as Go, is a powerful and efficient programming language that has gained popularity in recent years. It provides built-in support for concurrency and scalability, making it an excellent choice for building high-performance backend systems.
To get started with building RESTful APIs in Go, we need to set up a few dependencies. The first dependency we need is the Gorilla Mux package, which is a powerful URL router and dispatcher for Go. It allows us to define routes and handle different HTTP methods easily. We can install it using the following command:
go get -u github.com/gorilla/mux
Once we have Gorilla Mux installed, we can start building our RESTful API. Let's create a new Go file called
main.go
and import the necessary packages:
package main import ( "fmt" "log" "net/http" "github.com/gorilla/mux" )
Next, we can define our API routes using Gorilla Mux. Let's create a simple route that returns a "Hello, World!" message when a GET request is made to the root endpoint:
func main() { router := mux.NewRouter() router.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") }).Methods("GET") log.Fatal(http.ListenAndServe(":8000", router)) }
In the above code snippet, we create a new instance of the Gorilla Mux router and define a route using the
HandleFunc
method. We specify the root endpoint ("/") and define a function that writes the "Hello, World!" message to the HTTP response.
To start our API server, we use the
ListenAndServe
function from thenet/http
package. We pass in the router and specify the port on which the server should listen (in this case, port 8000).
Now, if we run our Go program and navigate to
http://localhost:8000
in a web browser, we should see the "Hello, World!" message displayed.
Building a complete RESTful API involves handling different HTTP methods, such as POST, PUT, and DELETE, and working with data. Gorilla Mux provides various methods to handle different routes and HTTP methods.
In the next sections, we will explore how to handle different HTTP methods, parse request parameters, and work with JSON data in our RESTful API using Golang.
Continue reading: [Handling HTTP Methods and Request Parameters](https://example.com/handling-http-methods-request-parameters)
Continue reading: [Working with JSON Data in a RESTful API](https://example.com/working-with-json-data-restful-api)
Related Article: Real-Time Communication with Beego and WebSockets
Implementing authentication and authorization in Golang
Authentication and authorization are crucial aspects of any backend development project. In this chapter, we will explore how to implement authentication and authorization in Golang to secure our backend APIs.
Authentication
Authentication is the process of verifying the identity of a user. There are several methods available for implementing authentication in Golang, including token-based authentication, session-based authentication, and OAuth.
One popular method is token-based authentication, where a token is generated for each user upon successful login. This token is then sent with every subsequent request to authenticate the user. Here's an example of how to implement token-based authentication in Golang using JWT (JSON Web Tokens):
// main.go package main import ( "encoding/json" "fmt" "log" "net/http" "time" "github.com/dgrijalva/jwt-go" ) var jwtKey = []byte("my_secret_key") type Claims struct { Username string `json:"username"` jwt.StandardClaims } func Login(w http.ResponseWriter, r *http.Request) { var user User err := json.NewDecoder(r.Body).Decode(&user) if err != nil { w.WriteHeader(http.StatusBadRequest) return } if user.Username != "admin" || user.Password != "password" { w.WriteHeader(http.StatusUnauthorized) return } expirationTime := time.Now().Add(5 * time.Minute) claims := &Claims{ Username: user.Username, StandardClaims: jwt.StandardClaims{ ExpiresAt: expirationTime.Unix(), }, } token := jwt.NewWithClaims(jwt.SigningMethodHS256, claims) tokenString, err := token.SignedString(jwtKey) if err != nil { w.WriteHeader(http.StatusInternalServerError) return } http.SetCookie(w, &http.Cookie{ Name: "token", Value: tokenString, Expires: expirationTime, }) w.Write([]byte("Logged in successfully")) } func ProtectedEndpoint(w http.ResponseWriter, r *http.Request) { c, err := r.Cookie("token") if err != nil { if err == http.ErrNoCookie { w.WriteHeader(http.StatusUnauthorized) return } w.WriteHeader(http.StatusBadRequest) return } tokenString := c.Value claims := &Claims{} token, err := jwt.ParseWithClaims(tokenString, claims, func(token *jwt.Token) (interface{}, error) { return jwtKey, nil }) if err != nil { if err == jwt.ErrSignatureInvalid { w.WriteHeader(http.StatusUnauthorized) return } w.WriteHeader(http.StatusBadRequest) return } if !token.Valid { w.WriteHeader(http.StatusUnauthorized) return } w.Write([]byte("Protected content")) } func main() { http.HandleFunc("/login", Login) http.HandleFunc("/protected", ProtectedEndpoint) log.Fatal(http.ListenAndServe(":8080", nil)) }
In the above code, we define a
Login
function that receives the user's credentials and checks if they are valid. If the credentials are valid, we create a JWT token with an expiration time of five minutes. The token is then set as a cookie in the response.
We also define a
ProtectedEndpoint
function that serves as an example of a protected API endpoint. This function checks if the token sent in the request is valid and authorized. If the token is valid, it returns the protected content; otherwise, it returns an unauthorized status.
Authorization
Authorization is the process of granting or denying access to specific resources or actions based on the authenticated user's privileges. In Golang, we can implement authorization using middleware functions.
Here's an example of how to implement a simple authorization middleware using the Gorilla Mux package:
// main.go package main import ( "log" "net/http" "github.com/gorilla/mux" ) func AuthMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Check if the user is authorized // Example: Check if the user has the necessary role // If the user is not authorized, return an unauthorized status if !userIsAuthorized(r) { w.WriteHeader(http.StatusUnauthorized) return } // If the user is authorized, call the next handler next.ServeHTTP(w, r) }) } func userIsAuthorized(r *http.Request) bool { // Check if the user has the necessary role // Example: Check if the user has the "admin" role // Return true if the user is authorized, false otherwise return true } func ProtectedEndpoint(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Protected content")) } func main() { r := mux.NewRouter() protectedRouter := r.PathPrefix("/protected").Subrouter() protectedRouter.Use(AuthMiddleware) protectedRouter.HandleFunc("", ProtectedEndpoint) log.Fatal(http.ListenAndServe(":8080", r)) }
In the above code, we define an
AuthMiddleware
function that checks if the user is authorized to access a specific endpoint. If the user is not authorized, an unauthorized status is returned; otherwise, the next handler is called.
We then create a protected endpoint using the Gorilla Mux package and apply the
AuthMiddleware
as a middleware to that endpoint. This ensures that only authorized users can access the protected content.
By combining authentication and authorization, we can secure our backend APIs and ensure that only authenticated and authorized users can access sensitive resources.
That concludes our exploration of implementing authentication and authorization in Golang. In the next chapter, we will dive into database integration and data persistence. Stay tuned!
Optimizing performance in Golang applications
When building backend applications with Golang, it's important to consider performance optimizations to ensure your application runs efficiently. In this chapter, we will explore some key techniques and best practices for optimizing performance in Golang applications.
Related Article: Building Gin Backends for React.js and Vue.js
1. Use efficient data structures
Choosing the right data structures can significantly impact the performance of your Golang application. Golang provides several built-in data structures like arrays, slices, maps, and channels. Understanding the characteristics and trade-offs of each data structure can help you make better decisions.
For example, if you need dynamic sizing and fast insertions and deletions, slices are a good choice. However, if you need fast lookup and retrieval, maps are more suitable. By using the most appropriate data structure for your specific use case, you can improve the performance of your application.
Here's an example of using a slice to store a collection of integers:
package main import "fmt" func main() { numbers := []int{1, 2, 3, 4, 5} fmt.Println(numbers) }
2. Concurrency and parallelism
Golang's built-in support for concurrency and parallelism makes it a great choice for building high-performance applications. By leveraging goroutines and channels, you can easily run tasks concurrently and communicate between them.
Goroutines are lightweight threads managed by the Go runtime, and channels provide a safe way to share data between goroutines. By dividing your tasks into smaller units and executing them concurrently, you can achieve better overall performance.
Here's an example of using goroutines and channels to perform concurrent tasks:
package main import ( "fmt" "sync" ) func worker(id int, jobs <-chan int, results chan<- int, wg *sync.WaitGroup) { for job := range jobs { // Perform some task result := job * 2 // Send the result to the results channel results <- result } // Signal that this worker has completed its task wg.Done() } func main() { numJobs := 10 jobs := make(chan int, numJobs) results := make(chan int, numJobs) var wg sync.WaitGroup // Start the worker goroutines numWorkers := 5 for i := 0; i < numWorkers; i++ { wg.Add(1) go worker(i, jobs, results, &wg) } // Enqueue the jobs for i := 0; i < numJobs; i++ { jobs <- i } close(jobs) // Wait for all workers to finish wg.Wait() // Collect the results close(results) for result := range results { fmt.Println(result) } }
3. Avoid unnecessary memory allocations
Golang's garbage collector is efficient at managing memory, but unnecessary allocations can still impact performance. One common mistake is repeatedly allocating memory inside loops. To avoid this, you can preallocate memory outside the loop and reuse it.
Here's an example that demonstrates the performance improvement by reusing a buffer:
package main import ( "fmt" "strings" ) func main() { var sb strings.Builder for i := 0; i < 1000; i++ { sb.WriteString("hello ") } result := sb.String() fmt.Println(result) }
4. Profiling
Profiling is an important technique for identifying performance bottlenecks in your Golang application. Golang provides built-in profiling support through the "net/http/pprof" package.
By exposing the profiling endpoints and using tools like "go tool pprof", you can analyze CPU and memory usage, goroutine blocking, and other performance metrics. This allows you to pinpoint areas of your code that may need optimization.
To enable profiling in your application, you can add the following code:
package main import ( "log" "net/http" _ "net/http/pprof" ) func main() { go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }() // Rest of your application code }
You can then access the profiling endpoints by visiting "http://localhost:6060/debug/pprof" in your browser.
Related Article: Exploring Advanced Features of Beego in Golang
5. Use efficient algorithms and techniques
Lastly, using efficient algorithms and techniques can greatly impact the performance of your Golang application. Familiarize yourself with common algorithms and data structures, and choose the most appropriate ones for your use case.
For example, when searching for an element in a sorted list, consider using binary search instead of linear search for better performance. Similarly, when sorting large datasets, consider using more efficient sorting algorithms like quicksort or mergesort.
By optimizing your algorithms and techniques, you can achieve significant performance improvements in your Golang application.
In this chapter, we explored various techniques and best practices for optimizing performance in Golang applications. By using efficient data structures, leveraging concurrency and parallelism, avoiding unnecessary memory allocations, profiling, and using efficient algorithms, you can build high-performance backend applications with Golang.
Deploying Golang applications to production
Deploying your Golang application to production is an important step in the software development lifecycle. It involves preparing your application to run in a production environment and ensuring that it is available to your users.
There are several ways to deploy a Golang application, depending on your specific requirements and preferences. In this section, we will discuss some common deployment strategies and tools that can help you streamline the process.
1. Building your application
Before deploying your Golang application, you need to build it into an executable file. Go provides a built-in tool called
go build
that compiles your code and generates an executable binary.
To build your application, open a terminal and navigate to the root directory of your project. Then, run the following command:
go build
This will create an executable file with the same name as your project. You can then move this file to your deployment environment.
2. Containerization
Containerization is a popular deployment strategy that allows you to package your application along with its dependencies into a lightweight, isolated container. This approach provides consistency and portability, making it easier to deploy and manage your application.
Docker is a widely-used containerization platform that can be used to deploy Golang applications. To containerize your application, you need to create a Dockerfile, which is a text file that contains a set of instructions for building a Docker image.
Here's an example of a Dockerfile for a Golang application:
# Use the official Golang base image FROM golang:1.16-alpine # Set the working directory inside the container WORKDIR /app # Copy the source code into the container COPY . . # Build the application RUN go build -o main . # Expose the port that the application listens on EXPOSE 8080 # Run the application CMD ["./main"]
To build a Docker image from this Dockerfile, run the following command:
docker build -t my-golang-app .
Once the image is built, you can deploy it to any environment that supports Docker.
Related Article: Best Practices of Building Web Apps with Gin & Golang
3. Continuous Integration and Delivery (CI/CD)
CI/CD is a software development practice that involves automating the process of building, testing, and deploying your application. It helps ensure that your code is always in a deployable state and reduces the risk of introducing bugs into production.
There are several CI/CD tools that can be used to deploy Golang applications, such as Jenkins, GitLab CI/CD, and CircleCI. These tools allow you to define a pipeline that includes various stages, such as building, testing, and deploying your application.
Here's an example of a simple CI/CD pipeline for a Golang application using Jenkins:
pipeline { agent any stages { stage('Build') { steps { sh 'go build' } } stage('Test') { steps { sh 'go test ./...' } } stage('Deploy') { steps { sh 'scp my-app user@production-server:/path/to/app' } } } }
This pipeline includes three stages: build, test, and deploy. Each stage is executed sequentially, and the pipeline fails if any of the stages fail.
4. Deployment to cloud platforms
Cloud platforms, such as AWS, Google Cloud, and Azure, provide managed services for deploying and scaling applications. These platforms offer features like auto-scaling, load balancing, and monitoring, which can help you deploy your Golang application to production with ease.
To deploy your Golang application to a cloud platform, you typically need to containerize your application using Docker and then use the platform's container orchestration service, such as AWS ECS, Google Kubernetes Engine (GKE), or Azure Kubernetes Service (AKS).
Here's an example of deploying a Golang application to AWS ECS:
1. Create a Dockerfile for your application.
2. Build a Docker image from the Dockerfile.
3. Push the Docker image to a container registry, such as Docker Hub or AWS ECR.
4. Create a task definition in AWS ECS, specifying the Docker image, CPU/memory requirements, and other settings.
5. Create a service in AWS ECS, specifying the task definition, desired number of tasks, and load balancing settings.
Once the service is created, AWS ECS will automatically deploy and manage your Golang application.
Monitoring and debugging Golang applications
Monitoring and debugging are crucial aspects of developing and maintaining any application, including those built with Golang. In this chapter, we will explore some popular tools and techniques for monitoring and debugging Golang applications.
Logging
Logging is the first step in monitoring and debugging an application. It allows you to track the flow of execution, capture errors, and record important events. Golang provides a built-in package called
log
that makes logging easy. Here's an example of how to use it:
package main import ( "log" ) func main() { log.Println("This is a log message") log.Fatalf("This is a fatal error: %s", "something went wrong") }
In the above code, we import the
log
package and use thePrintln
andFatalf
functions to log messages. ThePrintln
function logs a message, and theFatalf
function logs a message and exits the program with a non-zero status.
Related Article: Implementing Enterprise Features with Golang & Beego
Profiling
Profiling is the process of analyzing the performance of an application. It helps identify bottlenecks and optimize the code for better efficiency. Golang has a built-in profiling tool called
pprof
. Here's an example of how to use it:
package main import ( "log" "os" "runtime/pprof" ) func main() { f, err := os.Create("profile.prof") if err != nil { log.Fatal(err) } defer f.Close() err = pprof.StartCPUProfile(f) if err != nil { log.Fatal(err) } defer pprof.StopCPUProfile() // Your application code here err = pprof.WriteHeapProfile(f) if err != nil { log.Fatal(err) } }
In the above code, we create a file to store the profiling data. We then start profiling the CPU usage using
StartCPUProfile
and stop it usingStopCPUProfile
. Finally, we write the heap profile usingWriteHeapProfile
. You can analyze the generated profile using various tools likego tool pprof
.
Tracing
Tracing allows you to understand the execution flow of your application. It helps identify performance issues and uncover bottlenecks. Golang provides a built-in tracing tool called
trace
. Here's an example of how to use it:
package main import ( "log" "os" "runtime/trace" ) func main() { f, err := os.Create("trace.out") if err != nil { log.Fatal(err) } defer f.Close() err = trace.Start(f) if err != nil { log.Fatal(err) } defer trace.Stop() // Your application code here }
In the above code, we create a file to store the trace data. We then start tracing using
Start
and stop it usingStop
. The trace data can be visualized using thego tool trace
command.
Debugging
Debugging is the process of finding and fixing errors in an application. Golang provides a built-in debugger called
delve
. It allows you to set breakpoints, inspect variables, and step through the code. Here's an example of how to use it:
$ go get github.com/go-delve/delve/cmd/dlv $ dlv debug Type 'help' for list of commands. (dlv) break main.go:10 (dlv) run (dlv) next (dlv) print variableName (dlv) quit
In the above code, we first install
delve
usinggo get
. We then start the debugger usingdlv debug
. We set a breakpoint on line 10 of themain.go
file, run the program, step to the next line, print the value of a variable, and finally quit the debugger.
Exploring concurrency in Golang
Concurrency is a powerful feature of the Go programming language that allows you to write efficient and scalable programs. Go provides built-in support for concurrent programming through goroutines and channels. In this chapter, we will explore these concepts and how they can be used to build concurrent applications in Go.
Related Article: Exploring Advanced Features of Gin in Golang
Goroutines
A goroutine is a lightweight thread of execution that can run concurrently with other goroutines. Goroutines are created using the
go
keyword followed by a function call. Here's a simple example:
package main import ( "fmt" "time" ) func printNumbers() { for i := 1; i <= 5; i++ { fmt.Println(i) time.Sleep(1 * time.Second) } } func main() { go printNumbers() time.Sleep(3 * time.Second) }
In this example, we have a
printNumbers
function that prints numbers from 1 to 5 with a delay of 1 second between each number. We create a goroutine by prefixing the function call with thego
keyword. Themain
function also includes atime.Sleep
call to prevent the program from exiting before the goroutine has finished.
When you run this program, you will see the numbers being printed concurrently. Goroutines are extremely lightweight, allowing you to create thousands or even millions of them without much overhead.
Channels
Channels are a way to communicate and synchronize data between goroutines. They provide a safe and efficient way to pass values between concurrent operations. In Go, channels are typed and can be used to send and receive values of a specific data type.
Here's an example that demonstrates the use of channels:
package main import "fmt" func sum(numbers []int, result chan int) { sum := 0 for _, num := range numbers { sum += num } result <- sum } func main() { numbers := []int{1, 2, 3, 4, 5} result := make(chan int) go sum(numbers, result) total := <-result fmt.Println("Sum:", total) }
In this example, we have a
sum
function that calculates the sum of a slice of integers. We pass a channel,result
, to the function to receive the calculated sum. By using the<-
operator, we can send values to and receive values from the channel.
When you run this program, you will see the sum being calculated concurrently. Channels provide a synchronization mechanism, ensuring that the result is only read from the channel when it is available.
Concurrency Patterns
Go provides several built-in concurrency patterns that can be used to solve common problems. Some of these patterns include fan-out/fan-in, worker pools, and timeouts. These patterns help in managing and coordinating concurrent operations effectively.
Here's an example of the fan-out/fan-in pattern:
package main import ( "fmt" "math/rand" "sync" "time" ) func worker(id int, jobs <-chan int, results chan<- int) { for job := range jobs { fmt.Println("Worker", id, "started job", job) time.Sleep(time.Duration(rand.Intn(3)) * time.Second) fmt.Println("Worker", id, "finished job", job) results <- job * 2 } } func main() { jobs := make(chan int, 5) results := make(chan int, 5) var wg sync.WaitGroup for i := 1; i <= 3; i++ { wg.Add(1) go func(id int) { worker(id, jobs, results) wg.Done() }(i) } for i := 1; i <= 5; i++ { jobs <- i } close(jobs) go func() { wg.Wait() close(results) }() for result := range results { fmt.Println("Result:", result) } }
In this example, we have multiple workers that concurrently process jobs from a channel. The fan-out/fan-in pattern allows us to distribute the work among multiple workers and collect the results efficiently.
This is just one example of a concurrency pattern in Go. There are many more patterns and techniques that can be explored to build robust and scalable concurrent applications.
In the next chapter, we will dive deeper into the topic of error handling in Go.
Using channels and goroutines for concurrent programming
Concurrency is a fundamental concept in modern software development, especially when it comes to building efficient and responsive backend systems. In Go, channels and goroutines are powerful features that enable developers to write concurrent programs easily and effectively.
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
Goroutines
A goroutine is a lightweight thread of execution that allows concurrent execution within a single Go program. Goroutines are extremely cheap to create and can be created in large numbers without consuming excessive resources.
To create a goroutine, simply prefix a function call with the
go
keyword. For example, let's consider a simple functionprintHello()
that prints "Hello, World!" to the console.
package main import ( "fmt" ) func printHello() { fmt.Println("Hello, World!") } func main() { go printHello() fmt.Println("Main function") }
In this example, the
printHello()
function is executed concurrently as a goroutine, while the main function continues executing. As a result, you will see both "Hello, World!" and "Main function" printed to the console.
Goroutines are particularly useful for executing tasks that can be parallelized or need to run asynchronously, such as making API calls, processing large amounts of data, or handling multiple client requests simultaneously.
Channels
Channels are the communication mechanism that allows goroutines to synchronize and exchange data. A channel is a typed conduit through which you can send and receive values with the channel operator
<-
.
To create a channel, you can use the built-in
make()
function, specifying the type of data it will transmit. For example, let's create a channel that can transmit integers:
package main import ( "fmt" ) func main() { ch := make(chan int) go func() { ch <- 42 }() value := <-ch fmt.Println(value) }
In this code snippet, we create a channel
ch
of typeint
. Inside the goroutine, we send the value42
to the channel using the<-
operator. Outside the goroutine, we receive the value from the channel and print it to the console.
Channels can also be used to synchronize the execution of goroutines. By default, channel operations block until both the sender and receiver are ready. This behavior allows us to control the flow of execution and ensure that certain operations are completed before others.
For example, consider a scenario where we need to calculate the sum of two numbers concurrently. We can use two channels to send the numbers to be added and receive the result:
package main import ( "fmt" ) func addNumbers(a, b int, result chan int) { result <- a + b } func main() { ch := make(chan int) go addNumbers(5, 7, ch) sum := <-ch fmt.Println(sum) }
In this example, the
addNumbers()
function takes two integers and a channel as arguments. It calculates the sum of the numbers and sends the result to the channel. The main function receives the result from the channel and prints it to the console.
Using channels and goroutines, you can build complex concurrent programs that are easy to reason about and maintain. However, it's important to handle channel operations correctly to avoid deadlocks and ensure proper synchronization.
Remember to always close channels when you're done sending data to them. Closing a channel indicates that no more values will be sent and allows the receiver to detect when all values have been received.
package main import ( "fmt" ) func main() { ch := make(chan int) go func() { defer close(ch) ch <- 42 }() value, ok := <-ch if ok { fmt.Println(value) } else { fmt.Println("Channel closed") } }
In this example, we use the
close()
function to close the channel after sending the value42
. The receiver uses the second return value of the receive operation to check if the channel is closed. If the channel is closed, it prints "Channel closed" to the console.
By mastering channels and goroutines, you can leverage the full power of Go's concurrency model and build highly scalable and performant backend systems.
Working with third-party libraries and packages in Golang
Golang's strength lies in its robust standard library, which provides a wide range of functionality out of the box. However, there may be situations where you need to leverage the power of third-party libraries and packages to enhance your backend development workflow. In this chapter, we will explore how to work with third-party libraries and packages in Golang.
1. Finding and installing third-party packages
Golang has a package manager called "go modules" that makes it easy to manage dependencies. To find third-party packages, you can visit the official package repository at pkg.go.dev. This website allows you to search for packages based on keywords, package names, or import paths.
Once you find a package you want to use, you can install it by running the following command in your terminal:
go get <package-import-path>
For example, to install the popular Gorilla Mux router package, you would run:
go get github.com/gorilla/mux
This command will download the package and its dependencies into your project's
go.mod
file.
Related Article: Deployment and Monitoring Strategies for Gin Apps
2. Importing and using third-party packages
To use a third-party package in your Golang code, you need to import it at the beginning of your file. The import statement follows the format:
import "package-import-path"
For example, to import the Gorilla Mux package, you would write:
import "github.com/gorilla/mux"
Once you've imported the package, you can start using its functions, types, and variables in your code. For example, here's how you can use Gorilla Mux to define routes for your backend API:
package main import ( "log" "net/http" "github.com/gorilla/mux" ) func main() { router := mux.NewRouter() router.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Welcome to my API!")) }) log.Fatal(http.ListenAndServe(":8000", router)) }
In this example, we import the Gorilla Mux package, create a new router instance, define a route for the root path ("/"), and start the HTTP server.
3. Versioning and updating third-party packages
When you install a third-party package using
go get
, Go modules will automatically handle versioning for you. It creates ago.mod
file that keeps track of the specific versions of packages you're using.
To update a package to the latest version, you can use the
go get -u
command followed by the import path of the package. For example:
go get -u github.com/gorilla/mux
This command will update the Gorilla Mux package to the latest version available.
4. Handling package import conflicts
If you're using multiple third-party packages that have conflicting import paths, you can use the
import
keyword with a custom name to resolve the conflict. For example:
import ( "github.com/go-sql-driver/mysql" mySQL "github.com/mysql" )
In this example, we import two different MySQL driver packages with conflicting import paths. We assign a custom name
mySQL
to one of the packages to differentiate between them.
5. Popular third-party libraries and packages
There are numerous popular third-party libraries and packages available for Golang that can help you streamline your backend development process. Some examples include:
- Gin: A high-performance HTTP web framework for building APIs.
- GORM: An ORM library for working with databases.
- Logrus: A structured logger for better logging in your applications.
These are just a few examples, and there are many more packages available to suit various needs in Golang development.
In this chapter, we learned how to find, install, import, and use third-party packages in Golang. We also explored versioning, updating packages, handling import conflicts, and discovered some popular third-party libraries and packages. Using third-party packages can significantly enhance your backend development experience in Golang by providing ready-to-use functionality and saving development time.
Related Article: Integrating Payment, Voice and Text with Beego & Golang
Building microservices with Golang
Microservices architecture has gained popularity in recent years due to its ability to create scalable and maintainable systems. In this chapter, we will explore how to build microservices using the Go programming language (Golang).
What are microservices?
Microservices are an architectural style that structures an application as a collection of small, loosely coupled services. Each service is self-contained and can be developed, deployed, and scaled independently. These services communicate with each other through lightweight protocols such as HTTP or message queues.
Why use Golang for building microservices?
Golang is a great choice for building microservices due to its simplicity, performance, and built-in support for concurrency. Its compiled nature makes it efficient and allows for easy deployment. Golang's standard library provides essential packages for building HTTP servers, handling JSON, and working with databases.
Creating a simple microservice with Golang
To get started, let's create a simple microservice that exposes an API endpoint to retrieve user information. We will use the Gorilla Mux package for routing and handling HTTP requests.
First, we need to install the Gorilla Mux package:
go get -u github.com/gorilla/mux
Next, let's create a file called
main.go
and import the required packages:
package main import ( "encoding/json" "log" "net/http" "github.com/gorilla/mux" )
Now, let's define a struct to represent a user:
type User struct { ID string `json:"id"` Name string `json:"name"` Email string `json:"email"` }
Next, let's create a handler function to retrieve user information:
func GetUser(w http.ResponseWriter, r *http.Request) { // Simulate fetching user information from a database user := User{ ID: "1", Name: "John Doe", Email: "john@example.com", } // Convert the user struct to JSON json.NewEncoder(w).Encode(user) }
Now, let's create the main function to set up the HTTP server and define the API route:
func main() { // Create a new Gorilla Mux router router := mux.NewRouter() // Define the API route router.HandleFunc("/user", GetUser).Methods("GET") // Start the HTTP server log.Fatal(http.ListenAndServe(":8000", router)) }
To run the microservice, use the following command:
go run main.go
Now, you can access the user information by making a GET request to
http://localhost:8000/user
.
Related Article: Optimizing and Benchmarking Beego ORM in Golang
Exploring advanced Golang topics
Now that you have a solid understanding of the basics of Golang for backend development, it's time to dive into some more advanced topics. In this chapter, we will explore a few of these topics that will help you take your Golang skills to the next level.
Concurrency in Golang
Concurrency is a fundamental concept in Golang that allows you to write efficient, concurrent programs. Goroutines and channels are the key building blocks for achieving concurrency in Golang.
A goroutine is a lightweight thread managed by the Go runtime. It allows you to execute functions concurrently. To create a goroutine, simply prefix the function call with the keyword
go
. Here's an example:
package main import "fmt" func main() { go printHello() fmt.Println("Main function") } func printHello() { fmt.Println("Hello from goroutine!") }
In the example above, the
printHello
function is executed concurrently in a goroutine while the main function continues its execution.
Channels are used for communication and synchronization between goroutines. They provide a way to send and receive values between different goroutines. Here's an example that demonstrates the use of channels:
package main import "fmt" func main() { ch := make(chan string) go sendMessage(ch) message := <-ch fmt.Println("Received message:", message) } func sendMessage(ch chan<- string) { ch <- "Hello, channel!" }
In the example above, we create a channel
ch
using themake
function. We then spawn a goroutine to send a message to the channel using the<-
operator. Finally, we receive the message from the channel using the<-
operator and print it.
Error handling in Golang
Error handling is an important aspect of writing robust and reliable software. In Golang, error handling is explicit and encourages the use of the
error
type.
The
error
type is an interface that represents an error condition. Functions that may return an error typically have a return type oferror
.
Here's an example that demonstrates error handling in Golang:
package main import ( "fmt" "os" ) func main() { file, err := os.Open("nonexistent.txt") if err != nil { fmt.Println("Error:", err) return } defer file.Close() // Process the file }
In the example above, we use the
os.Open
function to open a file. If the file doesn't exist, the function returns an error. We check if the error is not equal tonil
and handle it accordingly.
Testing in Golang
Testing is an essential part of software development. Golang provides a built-in testing framework that makes it easy to write and execute tests.
To write tests in Golang, create a new file with the suffix
_test.go
. Tests are defined as functions with the signaturefunc TestXxx(t *testing.T)
.
Here's an example of a simple test function:
package main import ( "testing" ) func TestAdd(t *testing.T) { result := add(2, 3) expected := 5 if result != expected { t.Errorf("Expected %d, but got %d", expected, result) } } func add(a, b int) int { return a + b }
In the example above, we define a test function
TestAdd
that checks whether theadd
function returns the expected result. If the result is not as expected, we use thet.Errorf
function to report the error.
Real-world examples of Golang backend development
When it comes to backend development, Golang has gained popularity for its simplicity, efficiency, and concurrency support. In this chapter, we will explore some real-world examples of how Golang can be used for backend development.
Example 1: Building a RESTful API
One common use case for Golang in backend development is building RESTful APIs. Golang provides a clean and easy-to-use standard library for building HTTP servers. Let's take a look at a simple example:
package main import ( "encoding/json" "log" "net/http" ) type User struct { ID int `json:"id"` Name string `json:"name"` } var users []User func getUsers(w http.ResponseWriter, r *http.Request) { json.NewEncoder(w).Encode(users) } func main() { users = []User{ {ID: 1, Name: "John"}, {ID: 2, Name: "Jane"}, } http.HandleFunc("/users", getUsers) log.Fatal(http.ListenAndServe(":8080", nil)) }
In this example, we define a
User
struct and a slice ofUser
objects. We then create an HTTP handler functiongetUsers
that encodes theusers
slice into JSON and writes it to the response.
We register the
getUsers
handler function with the endpoint/users
usinghttp.HandleFunc
. Finally, we start the HTTP server on port 8080 usinghttp.ListenAndServe
.
Example 2: Working with databases
Another common use case for Golang backend development is working with databases. Golang provides excellent support for working with various databases through its database/sql package. Let's see an example of using Golang with PostgreSQL:
package main import ( "database/sql" "fmt" "log" _ "github.com/lib/pq" ) type User struct { ID int Name string } func main() { db, err := sql.Open("postgres", "postgres://username:password@localhost/mydatabase?sslmode=disable") if err != nil { log.Fatal(err) } defer db.Close() rows, err := db.Query("SELECT id, name FROM users") if err != nil { log.Fatal(err) } defer rows.Close() var users []User for rows.Next() { var user User err := rows.Scan(&user.ID, &user.Name) if err != nil { log.Fatal(err) } users = append(users, user) } for _, user := range users { fmt.Println(user) } }
In this example, we import the
database/sql
package and the PostgreSQL driver packagegithub.com/lib/pq
. We then establish a connection to the PostgreSQL database usingsql.Open
and execute a simple query to retrieve all users.
We iterate over the result set using
rows.Next
and populate a slice ofUser
objects. Finally, we print the retrieved users to the console.
Related Article: Applying Design Patterns with Gin and Golang
Example 3: Building a WebSocket server
Golang's built-in concurrency support makes it an excellent choice for building WebSocket servers. Let's take a look at a simple example:
package main import ( "log" "net/http" "github.com/gorilla/websocket" ) var upgrader = websocket.Upgrader{} func handleWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Fatal(err) } defer conn.Close() for { _, message, err := conn.ReadMessage() if err != nil { log.Println(err) return } log.Printf("Received message: %s", message) err = conn.WriteMessage(websocket.TextMessage, []byte("Message received")) if err != nil { log.Println(err) return } } } func main() { http.HandleFunc("/ws", handleWebSocket) log.Fatal(http.ListenAndServe(":8080", nil)) }
In this example, we import the
gorilla/websocket
package, which provides an easy-to-use WebSocket implementation. We define a handler functionhandleWebSocket
that upgrades the HTTP connection to a WebSocket connection and handles incoming messages.
We read each incoming message using
conn.ReadMessage
, log it, and send a response usingconn.WriteMessage
. The server keeps running indefinitely, handling WebSocket connections.
These examples demonstrate just a few of the many possibilities of Golang for backend development. Whether you're building RESTful APIs, working with databases, or building WebSocket servers, Golang offers a simple and efficient solution for your backend needs.