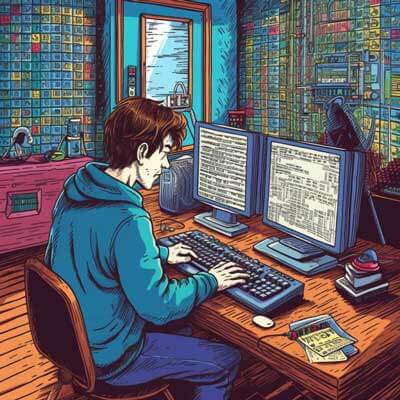
- Introduction
- JWT Authentication in Gin
- Middleware in Gin for Authentication
- Crypto Packages in Golang for Encryption and Decryption
- Hashing Algorithms in Golang for Secure Password Storage
- Access Control Implementation in Gin
- Secure Coding Practices in Golang
- Secure Communications in Golang Applications
- Common Security Vulnerabilities in Golang Applications
- Preventing Cross-Site Scripting (XSS) Attacks in Golang
- Symmetric vs Asymmetric Encryption in Golang
- Additional Resources
Introduction
Security is a critical aspect of any application, and Golang provides robust tools and packages to help developers implement secure authentication, middleware, and encryption in their applications. In this article, we will explore the various security considerations when using Golang and the Gin web framework. We will cover topics such as JWT authentication, middleware for authentication, encryption and decryption using Golang’s crypto packages, secure password storage with hashing algorithms, access control implementation, secure coding practices, secure communications, common security vulnerabilities, preventing Cross-Site Scripting (XSS) attacks, and the difference between symmetric and asymmetric encryption in Golang.
Related Article: Golang Tutorial for Backend Development
JWT Authentication in Gin
JSON Web Tokens (JWT) is a popular method for implementing authentication in web applications. It allows the server to generate a token that contains user information, which can be used to authenticate subsequent requests made by the client. Gin provides a straightforward way to implement JWT authentication.
To get started with JWT authentication in Gin, you first need to install the necessary packages. One popular package is “github.com/dgrijalva/jwt-go”, which provides a JWT implementation for Golang. You can install it using the following command:
go get github.com/dgrijalva/jwt-go
Once you have the package installed, you can create a middleware function that handles the JWT authentication. Here’s an example of how you can implement JWT authentication in Gin:
import ( "net/http" "time" "github.com/gin-gonic/gin" "github.com/dgrijalva/jwt-go" ) func authMiddleware() gin.HandlerFunc { return func(c *gin.Context) { tokenString := c.GetHeader("Authorization") if tokenString == "" { c.AbortWithStatusJSON(http.StatusUnauthorized, gin.H{"error": "Unauthorized"}) return } token, err := jwt.Parse(tokenString, func(token *jwt.Token) (interface{}, error) { return []byte("secretKey"), nil }) if err != nil { c.AbortWithStatusJSON(http.StatusUnauthorized, gin.H{"error": "Invalid token"}) return } if claims, ok := token.Claims.(jwt.MapClaims); ok && token.Valid { c.Set("userID", claims["userID"]) c.Next() } else { c.AbortWithStatusJSON(http.StatusUnauthorized, gin.H{"error": "Invalid token"}) } } } func main() { r := gin.Default() r.GET("/protected", authMiddleware(), func(c *gin.Context) { userID := c.GetString("userID") c.JSON(http.StatusOK, gin.H{"userID": userID}) }) r.Run(":8080") }
In this example, we define an authMiddleware
function that verifies the JWT token. It checks if the Authorization
header is present, parses the token using the jwt.Parse
function, and validates the token using a secret key. If the token is valid, it sets the userID
claim in the context and allows the request to proceed. Otherwise, it returns a JSON response with an error message.
To protect a route with JWT authentication, you can simply add the authMiddleware
function as a middleware to the route. In the example above, the /protected
route is protected with JWT authentication by passing authMiddleware()
as a middleware function.
Middleware in Gin for Authentication
Middleware plays a crucial role in implementing authentication and security measures in web applications. Gin provides a flexible middleware system that allows developers to easily add authentication and other security-related functionalities to their applications.
To create a custom middleware in Gin, you need to define a function that takes a gin.HandlerFunc
as an argument and returns a new gin.HandlerFunc
. The middleware function can perform any necessary authentication or security checks before passing the request to the next handler.
Here’s an example of how you can create a middleware in Gin for authentication:
import ( "net/http" "github.com/gin-gonic/gin" ) func authMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Perform authentication checks here // Example: check if the user is logged in if !isLoggedIn(c) { c.AbortWithStatusJSON(http.StatusUnauthorized, gin.H{"error": "Unauthorized"}) return } // Call the next handler c.Next() } } func isLoggedIn(c *gin.Context) bool { // Check if the user is logged in // Example: check if the session contains a user ID userID := c.GetString("userID") return userID != "" } func main() { r := gin.Default() r.GET("/protected", authMiddleware(), func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{"message": "Protected endpoint"}) }) r.Run(":8080") }
In this example, we define an authMiddleware
function that checks if the user is logged in. If the user is not logged in, it aborts the request and returns a JSON response with an error message. Otherwise, it calls the next handler in the chain.
To use the middleware, you simply need to add it as a middleware function to the desired route. In the example above, the /protected
route is protected with the authMiddleware
by passing authMiddleware()
as a middleware function.
Gin also provides built-in middleware functions for common security-related tasks, such as Cross-Origin Resource Sharing (CORS) handling, Cross-Site Request Forgery (CSRF) prevention, and rate limiting. These middleware functions can be easily added to your application to enhance its security.
Crypto Packages in Golang for Encryption and Decryption
Golang provides a set of crypto packages that allow developers to perform various encryption and decryption operations. These packages adhere to industry-standard cryptographic algorithms and provide a secure way to protect sensitive data in Golang applications.
Here are some of the crypto packages available in Golang:
– crypto/aes
: Package aes
implements AES (Advanced Encryption Standard), which is a symmetric block cipher used for encryption and decryption.
– crypto/rsa
: Package rsa
implements RSA encryption and decryption. RSA is an asymmetric encryption algorithm that uses two keys: a public key for encryption and a private key for decryption.
– crypto/rand
: Package rand
provides functions for generating random numbers and cryptographic security.
– crypto/md5
: Package md5
implements the MD5 hash algorithm, which is commonly used for checksums and cryptographic purposes.
– crypto/sha256
: Package sha256
implements the SHA-256 hash algorithm, which is widely used for cryptographic purposes.
To encrypt and decrypt data using Golang’s crypto packages, you first need to import the relevant packages and create the necessary cryptographic objects. Here’s an example of how you can use the crypto/aes
package to encrypt and decrypt data:
import ( "crypto/aes" "crypto/cipher" "crypto/rand" "encoding/base64" "fmt" "io" ) func encrypt(plainText []byte, key []byte) (string, error) { block, err := aes.NewCipher(key) if err != nil { return "", err } cipherText := make([]byte, aes.BlockSize+len(plainText)) iv := cipherText[:aes.BlockSize] if _, err := io.ReadFull(rand.Reader, iv); err != nil { return "", err } stream := cipher.NewCTR(block, iv) stream.XORKeyStream(cipherText[aes.BlockSize:], plainText) return base64.StdEncoding.EncodeToString(cipherText), nil } func decrypt(cipherText string, key []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } cipherBytes, err := base64.StdEncoding.DecodeString(cipherText) if err != nil { return nil, err } iv := cipherBytes[:aes.BlockSize] cipherTextBytes := cipherBytes[aes.BlockSize:] stream := cipher.NewCTR(block, iv) stream.XORKeyStream(cipherTextBytes, cipherTextBytes) return cipherTextBytes, nil } func main() { text := "Hello, World!" key := []byte("0123456789abcdef") cipherText, err := encrypt([]byte(text), key) if err != nil { fmt.Println("Encryption error:", err) return } fmt.Println("Cipher text:", cipherText) plainText, err := decrypt(cipherText, key) if err != nil { fmt.Println("Decryption error:", err) return } fmt.Println("Plain text:", string(plainText)) }
In this example, we define encrypt
and decrypt
functions that use the crypto/aes
package to encrypt and decrypt data. The encrypt
function takes a plain text and a key as input, generates a random initialization vector (IV), and encrypts the plain text using AES in CTR mode. The decrypt
function takes a cipher text and a key as input, decodes the cipher text, retrieves the IV, and decrypts the cipher text using AES in CTR mode.
It’s important to note that the key used for encryption and decryption should be kept secret and securely managed. Storing keys in configuration files or source code is not recommended. Instead, consider using a key management system or storing keys in an encrypted form.
Related Article: Exploring Advanced Features of Gin in Golang
Hashing Algorithms in Golang for Secure Password Storage
When storing passwords in a database or other storage systems, it’s crucial to store them securely to prevent unauthorized access. Golang provides several hashing algorithms that are suitable for secure password storage, such as bcrypt and argon2.
Here’s an example of how you can use the golang.org/x/crypto/bcrypt
package to hash and verify passwords:
import ( "fmt" "golang.org/x/crypto/bcrypt" ) func hashPassword(password string) (string, error) { hash, err := bcrypt.GenerateFromPassword([]byte(password), bcrypt.DefaultCost) if err != nil { return "", err } return string(hash), nil } func verifyPassword(password string, hash string) bool { err := bcrypt.CompareHashAndPassword([]byte(hash), []byte(password)) return err == nil } func main() { password := "mysecretpassword" hash, err := hashPassword(password) if err != nil { fmt.Println("Hashing error:", err) return } fmt.Println("Hash:", hash) match := verifyPassword(password, hash) fmt.Println("Password matches:", match) }
In this example, we define hashPassword
and verifyPassword
functions that use the golang.org/x/crypto/bcrypt
package to hash and verify passwords. The hashPassword
function takes a password as input, generates a bcrypt hash with a default cost, and returns the hash as a string. The verifyPassword
function takes a password and a hash as input, compares the password with the hash, and returns a boolean value indicating whether the password matches the hash.
The bcrypt algorithm is designed to be slow and computationally expensive, which makes it resistant to brute-force attacks. It also automatically generates a random salt for each password, which further enhances security by preventing the use of precomputed rainbow tables.
When verifying passwords, always use the bcrypt.CompareHashAndPassword
function provided by the bcrypt package instead of implementing your own comparison logic. This function handles the comparison securely and mitigates timing attacks.
Access Control Implementation in Gin
Access control is an essential aspect of application security, as it ensures that users have appropriate permissions to access certain resources or perform specific actions. Gin provides a flexible way to implement access control using middleware functions.
To implement access control in Gin, you can create a middleware function that checks the user’s permissions or role before allowing access to a particular route or resource. Here’s an example of how you can implement access control in Gin:
import ( "net/http" "github.com/gin-gonic/gin" ) func accessControlMiddleware(allowedRoles []string) gin.HandlerFunc { return func(c *gin.Context) { // Check if the user has the required role role := getUserRole(c) if !isRoleAllowed(role, allowedRoles) { c.AbortWithStatusJSON(http.StatusForbidden, gin.H{"error": "Forbidden"}) return } // Call the next handler c.Next() } } func getUserRole(c *gin.Context) string { // Get the user's role from the session or database // Example: get the role from the session role := c.GetString("role") return role } func isRoleAllowed(role string, allowedRoles []string) bool { // Check if the user's role is allowed for _, allowedRole := range allowedRoles { if role == allowedRole { return true } } return false } func main() { r := gin.Default() r.GET("/admin", accessControlMiddleware([]string{"admin"}), func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{"message": "Admin access granted"}) }) r.GET("/user", accessControlMiddleware([]string{"admin", "user"}), func(c *gin.Context) { c.JSON(http.StatusOK, gin.H{"message": "User access granted"}) }) r.Run(":8080") }
In this example, we define an accessControlMiddleware
function that checks if the user has the required role to access a route. The middleware function takes a list of allowed roles as input and compares them with the user’s role. If the user’s role is not allowed, it aborts the request and returns a JSON response with an error message. Otherwise, it calls the next handler in the chain.
To use the access control middleware, you can add it to the desired routes by passing the allowed roles as an argument to the accessControlMiddleware
function. In the example above, the /admin
route is restricted to users with the “admin” role, while the /user
route is accessible to users with either the “admin” or “user” role.
It’s important to note that access control should be implemented both on the server-side and the client-side. The server-side access control ensures that unauthorized requests are rejected, while the client-side access control provides a better user experience by hiding or disabling inaccessible features.
Secure Coding Practices in Golang
When developing applications in Golang, it’s essential to follow secure coding practices to minimize the risk of security vulnerabilities and protect sensitive data. Here are some secure coding practices to keep in mind:
1. Input Validation: Validate and sanitize all user inputs to prevent common vulnerabilities such as SQL injection, cross-site scripting (XSS), and command injection. Use built-in validation functions or third-party libraries to ensure that user inputs meet the expected format and do not include malicious content.
2. Secure Password Storage: Always hash passwords using a secure hashing algorithm (e.g., bcrypt or argon2) before storing them in a database or other storage systems. Avoid using weak or outdated hashing algorithms, such as MD5 or SHA-1, as they are vulnerable to brute-force attacks. Additionally, consider using key stretching techniques and adding a unique salt for each password to enhance security.
3. Access Control: Implement proper access control mechanisms to ensure that users have appropriate permissions to access resources or perform certain actions. Use role-based access control (RBAC) or attribute-based access control (ABAC) to enforce access restrictions based on user roles or attributes. Avoid relying solely on client-side access control, as it can be easily bypassed.
4. Secure Communication: Use secure communication protocols, such as HTTPS, to encrypt data transmitted between clients and servers. Avoid transmitting sensitive information over unencrypted channels, as it can be intercepted and compromised. Use well-established encryption algorithms and key exchange protocols to ensure the confidentiality and integrity of data.
5. Error Handling: Handle errors properly to prevent information leakage and improve the application’s security. Avoid displaying detailed error messages to users, as they can reveal sensitive information or provide attackers with valuable insights into the application’s internals. Instead, log the errors securely and provide generic error messages to users.
6. Secure Dependencies: Regularly update and patch dependencies to mitigate security vulnerabilities. Use package management tools, such as Go modules, to manage dependencies and ensure that you are using the latest versions of libraries. Monitor security advisories and follow best practices for secure coding when using third-party packages.
7. Input and Output Validation: Validate and sanitize all input and output data to prevent vulnerabilities such as buffer overflows, format string attacks, and code injection. Use appropriate encoding and escaping techniques when handling user-supplied data to prevent XSS and other injection attacks. Be cautious when using unsafe packages or features, and always validate and sanitize user inputs before processing them.
8. Secure Configuration: Store sensitive configuration data, such as API keys, database credentials, and encryption keys, securely. Avoid hardcoding sensitive information in source code or configuration files. Instead, use environment variables or secure configuration files encrypted with strong encryption algorithms. Restrict access to configuration files and ensure that they are not accessible by unauthorized users.
9. Logging and Auditing: Implement proper logging and auditing mechanisms to monitor and detect security incidents. Log important security-related events, such as failed login attempts, access control violations, and system errors. Regularly review and analyze logs to identify and respond to potential security threats. Ensure that log files are stored securely and protected from unauthorized access.
10. Secure Session Management: Implement secure session management to prevent session hijacking and session fixation attacks. Generate strong session IDs using a cryptographically secure random number generator. Store session data securely, either in encrypted cookies or server-side storage, and validate session tokens to prevent session tampering. Set appropriate session expiration times and implement session logout functionality.
Related Article: Best Practices of Building Web Apps with Gin & Golang
Secure Communications in Golang Applications
Secure communication is crucial for protecting sensitive data transmitted over networks. Golang provides built-in packages and libraries that allow developers to implement secure communication protocols, such as HTTPS, TLS, and SSL, in their applications.
To implement secure communication in Golang applications, you need to use the net/http
package along with the crypto/tls
package. Here’s an example of how you can serve an HTTP server over HTTPS in Golang:
import ( "log" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, World!")) }) err := http.ListenAndServeTLS(":8443", "cert.pem", "key.pem", nil) if err != nil { log.Fatal("ListenAndServeTLS:", err) } }
In this example, we define an HTTP handler function that simply writes “Hello, World!” as the response. We then use the http.ListenAndServeTLS
function to start an HTTPS server. The server listens on port 8443 and uses the “cert.pem” and “key.pem” files to provide the TLS certificate and private key for secure communication.
To generate the TLS certificate and private key files, you can use tools like OpenSSL or Let’s Encrypt. These tools allow you to generate valid TLS certificates signed by trusted certificate authorities (CAs) or create self-signed certificates for development or testing purposes.
It’s important to note that when using HTTPS, the client and server exchange certificates to establish a secure connection. The server’s certificate is used to verify its identity, while the client’s certificate can be used for client authentication. You can configure the server to request client certificates and verify them using the crypto/tls
package.
In addition to HTTPS, Golang also supports other secure communication protocols, such as TLS and SSL, which can be used for secure communication between clients and servers. The crypto/tls
package provides functions and types for configuring and managing TLS connections. You can use these functions and types to implement secure communication in various network protocols, such as SMTP, IMAP, POP3, and more.
Common Security Vulnerabilities in Golang Applications
Despite Golang’s strong focus on security, it’s still possible to introduce security vulnerabilities in Golang applications. Understanding common security vulnerabilities can help developers identify and address potential security risks in their code. Here are some common security vulnerabilities in Golang applications:
1. Injection Attacks: Injection attacks occur when untrusted data is sent to an interpreter as part of a command or query. This can lead to arbitrary code execution or unauthorized access to data. Common types of injection attacks include SQL injection, NoSQL injection, OS command injection, and LDAP injection. To prevent injection attacks, always use parameterized queries or prepared statements, sanitize user inputs, and avoid executing untrusted code.
2. Cross-Site Scripting (XSS): XSS vulnerabilities occur when untrusted data is included in a web page without proper sanitization. This allows attackers to inject malicious scripts into web pages, leading to session hijacking, defacement, or data theft. To prevent XSS attacks, use proper output encoding or escaping techniques when displaying user-generated content and validate and sanitize all user inputs.
3. Cross-Site Request Forgery (CSRF): CSRF vulnerabilities occur when an attacker tricks a user’s browser into making unintended requests to a target website. This can lead to unauthorized actions performed on behalf of the user, such as changing passwords, making purchases, or deleting data. To prevent CSRF attacks, use anti-CSRF tokens, check the HTTP Referer header, and implement proper access control mechanisms.
4. Security Misconfigurations: Security misconfigurations occur when servers, frameworks, or applications are not securely configured. This can include exposing sensitive information, using default or weak passwords, allowing directory listing, or enabling unnecessary services or features. To prevent security misconfigurations, follow secure coding practices, regularly update and patch dependencies, use secure default configurations, and perform security assessments and audits.
5. Insecure Direct Object References (IDOR): IDOR vulnerabilities occur when an application exposes internal implementation details or allows direct access to internal objects or resources. This can result in unauthorized access to sensitive data or actions. To prevent IDOR vulnerabilities, implement proper access control mechanisms, avoid using predictable or sequential identifiers, and validate user permissions and input.
6. Server-Side Request Forgery (SSRF): SSRF vulnerabilities occur when an attacker can make unauthorized requests from the server to internal or external resources. This can lead to data leakage, remote code execution, or unauthorized access to internal systems. To prevent SSRF vulnerabilities, validate and sanitize all user inputs, restrict network access from the server, and use whitelists or allowlists to control the allowed destinations.
7. XML External Entity (XXE) Attacks: XXE vulnerabilities occur when an application processes external entities in XML input without proper validation. This can allow attackers to read arbitrary files, perform server-side request forgery, or launch denial-of-service attacks. To prevent XXE attacks, disable external entity processing, use secure XML parsers, and validate and sanitize all XML input.
8. Denial-of-Service (DoS) Attacks: DoS vulnerabilities occur when an attacker overwhelms a system’s resources, making it unavailable to legitimate users. This can be achieved by sending a high volume of requests, exploiting resource limitations, or triggering software flaws. To prevent DoS attacks, implement rate limiting, resource quotas, and request validation, and use secure coding practices to prevent common software vulnerabilities.
9. Deserialization Vulnerabilities: Deserialization vulnerabilities occur when an attacker can manipulate serialized data to execute arbitrary code or perform unintended actions. This can be achieved by exploiting weaknesses in the deserialization process or using maliciously crafted serialized objects. To prevent deserialization vulnerabilities, avoid deserializing untrusted data, validate and sanitize all input, and use secure deserialization libraries or mechanisms.
10. Information Leakage: Information leakage occurs when an application unintentionally exposes sensitive information, such as passwords, cryptographic keys, or error details. This can occur through error messages, log files, or insecure communication channels. To prevent information leakage, avoid displaying detailed error messages to users, log sensitive information securely, encrypt sensitive data in transit and at rest, and follow secure coding practices.
Preventing Cross-Site Scripting (XSS) Attacks in Golang
Cross-Site Scripting (XSS) is a type of security vulnerability that allows attackers to inject malicious scripts into web pages viewed by other users. XSS attacks can lead to session hijacking, defacement, data theft, or the execution of arbitrary code. Preventing XSS attacks is crucial for ensuring the security of web applications developed in Golang. Here are some techniques to prevent XSS attacks in Golang:
1. Sanitize User Inputs: Sanitize all user inputs to remove or escape characters that could be interpreted as HTML or JavaScript code. Use input validation libraries or functions to ensure that user inputs meet the expected format and do not include malicious content. For example, the html.EscapeString
function provided by the html
package can be used to escape special characters in user inputs.
2. Use Output Encoding: Use proper output encoding techniques when displaying user-generated content. Encode user inputs using functions like template.HTMLEscapeString
or html.EscapeString
to prevent HTML or JavaScript code from being executed. For example, in a template, use the {{. | html}}
or {{. | htmlSafe}}
syntax to automatically escape user inputs.
3. Content Security Policy (CSP): Implement a Content Security Policy (CSP) to restrict the types of content that can be loaded from external sources. CSP allows you to specify the allowed sources for scripts, stylesheets, images, fonts, and other types of content. By specifying strict CSP rules, you can prevent the execution of malicious scripts injected through XSS attacks.
4. HTTP Only Cookies: Set the “HttpOnly” flag on cookies to prevent them from being accessed by JavaScript code. This prevents attackers from stealing session cookies and helps mitigate session hijacking attacks. In Golang, you can set the “HttpOnly” flag by specifying the HttpOnly
field when creating cookies using the http.SetCookie
function.
5. Use Template Engines: When generating dynamic HTML content, use template engines that automatically escape user inputs. Golang’s built-in template engine provides automatic escaping of user inputs by default. Ensure that you are using the appropriate template tags ({{. | html}}
or {{. | htmlSafe}}
) to escape user inputs.
6. Input Validation and Whitelisting: Implement proper input validation and whitelisting to ensure that user inputs contain only expected characters or patterns. Reject or sanitize inputs that do not conform to the expected format. Avoid using regular expressions or custom validation logic that may be susceptible to bypass or injection attacks.
7. HTTP Headers: Set appropriate HTTP headers to protect against XSS attacks. Set the “X-XSS-Protection” header to enable the browser’s built-in XSS protection mechanisms. Set the “Content-Security-Policy” header to specify the allowed sources for scripts, stylesheets, and other types of content. Set the “X-Content-Type-Options” header to prevent content type sniffing.
8. Input Filtering: Filter user inputs to remove or encode potentially dangerous characters. For example, you can use the strings.Replace
function to remove or replace characters that could be used in XSS attacks. However, be cautious when implementing input filtering, as it may lead to false positives or bypasses if not done correctly.
9. Regular Security Audits: Regularly review and audit your codebase for potential XSS vulnerabilities. Perform security testing and penetration testing to identify and address any XSS vulnerabilities in your application. Use automated security scanning tools or engage third-party security experts to assist with vulnerability assessments and code reviews.
Related Article: Internationalization in Gin with Go Libraries
Symmetric vs Asymmetric Encryption in Golang
Encryption is a fundamental technique used to protect sensitive data from unauthorized access. Golang provides support for both symmetric and asymmetric encryption algorithms, each with its own strengths and use cases. Understanding the differences between symmetric and asymmetric encryption can help you choose the appropriate encryption method for your specific requirements.
Symmetric Encryption:
– Symmetric encryption uses a single shared secret key to both encrypt and decrypt data.
– It is typically faster and more efficient than asymmetric encryption.
– Symmetric encryption is suitable for scenarios where the sender and receiver share a common key and need to encrypt and decrypt data quickly.
– Common symmetric encryption algorithms in Golang include AES (Advanced Encryption Standard) and DES (Data Encryption Standard).
Asymmetric Encryption:
– Asymmetric encryption uses a pair of mathematically related keys: a public key for encryption and a private key for decryption.
– It provides stronger security by separating the encryption and decryption keys.
– Asymmetric encryption is suitable for scenarios where secure communication between parties is required, such as secure email communication or secure web communication using HTTPS.
– Common asymmetric encryption algorithms in Golang include RSA and ECDSA.
Here’s an example of how you can use symmetric and asymmetric encryption in Golang:
Symmetric Encryption (AES):
import ( "crypto/aes" "crypto/cipher" "crypto/rand" "fmt" "io" ) func encrypt(plainText []byte, key []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } cipherText := make([]byte, aes.BlockSize+len(plainText)) iv := cipherText[:aes.BlockSize] if _, err := io.ReadFull(rand.Reader, iv); err != nil { return nil, err } stream := cipher.NewCTR(block, iv) stream.XORKeyStream(cipherText[aes.BlockSize:], plainText) return cipherText, nil } func decrypt(cipherText []byte, key []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } iv := cipherText[:aes.BlockSize] cipherText = cipherText[aes.BlockSize:] stream := cipher.NewCTR(block, iv) stream.XORKeyStream(cipherText, cipherText) return cipherText, nil } func main() { text := "Hello, World!" key := []byte("0123456789abcdef") cipherText, err := encrypt([]byte(text), key) if err != nil { fmt.Println("Encryption error:", err) return } fmt.Println("Cipher text:", cipherText) plainText, err := decrypt(cipherText, key) if err != nil { fmt.Println("Decryption error:", err) return } fmt.Println("Plain text:", string(plainText)) }
Asymmetric Encryption (RSA):
import ( "crypto/rand" "crypto/rsa" "crypto/x509" "encoding/pem" "fmt" ) func encrypt(plainText []byte, publicKey []byte) ([]byte, error) { block, _ := pem.Decode(publicKey) if block == nil { return nil, fmt.Errorf("failed to decode PEM block containing public key") } pub, err := x509.ParsePKCS1PublicKey(block.Bytes) if err != nil { return nil, err } cipherText, err := rsa.EncryptPKCS1v15(rand.Reader, pub, plainText) if err != nil { return nil, err } return cipherText, nil } func decrypt(cipherText []byte, privateKey []byte) ([]byte, error) { block, _ := pem.Decode(privateKey) if block == nil { return nil, fmt.Errorf("failed to decode PEM block containing private key") } priv, err := x509.ParsePKCS1PrivateKey(block.Bytes) if err != nil { return nil, err } plainText, err := rsa.DecryptPKCS1v15(rand.Reader, priv, cipherText) if err != nil { return nil, err } return plainText, nil } func main() { text := "Hello, World!" privateKey := []byte(` -----BEGIN RSA PRIVATE KEY----- MIIEowIBAAKCAQEAvQI8fyoQkKzmeWz/4Pdyg6Jc3fdUzj2T5XyNlrJZ2g6Y1+3k ... -----END RSA PRIVATE KEY----- `) publicKey := []byte(` -----BEGIN RSA PUBLIC KEY----- MIIBCgKCAQEAvQI8fyoQkKzmeWz/4Pdyg6Jc3fdUzj2T5XyNlrJZ2g6Y1+3kZb7B ... -----END RSA PUBLIC KEY----- `) cipherText, err := encrypt([]byte(text), publicKey) if err != nil { fmt.Println("Encryption error:", err) return } fmt.Println("Cipher text:", cipherText) plainText, err := decrypt(cipherText, privateKey) if err != nil { fmt.Println("Decryption error:", err) return } fmt.Println("Plain text:", string(plainText)) }
In the symmetric encryption example, we use the AES algorithm to encrypt and decrypt data using a shared secret key. The crypto/aes
package is used to create a new AES cipher with the given key, generate a random initialization vector (IV), and encrypt or decrypt the data using the CTR mode. The same key and IV are used for both encryption and decryption.
In the asymmetric encryption example, we use the RSA algorithm to encrypt and decrypt data using a pair of public and private keys. The crypto/rsa
package is used to parse the public and private keys from the PEM-encoded data and encrypt or decrypt the data using the PKCS1v15 encryption scheme.
When using asymmetric encryption, the public key can be freely distributed, while the private key must be kept secret. The public key is used for encryption, while the private key is used for decryption. This allows secure communication between parties without sharing the private key.
It’s important to note that encryption alone does not guarantee data security. It should be used in conjunction with other security measures, such as secure key management, secure communication protocols, and access control mechanisms, to ensure the confidentiality, integrity, and availability of sensitive data.
Additional Resources
– Golang JWT Authentication with Gin Framework
– Gin Web Framework
– Cross-Origin Resource Sharing (CORS)