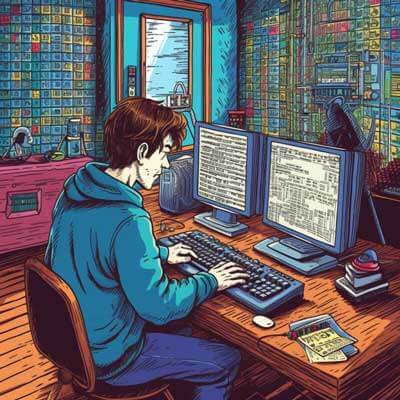
Table of Contents
Benefits of Using Golang for Backend Development
Golang, also known as Go, is a programming language developed by Google. It has gained popularity in recent years due to its simplicity, efficiency, and strong support for concurrency. When it comes to backend development, Golang offers several benefits:
1. Performance: Golang is known for its exceptional performance. It compiles to machine code, resulting in fast execution times. This makes it an ideal choice for building high-performance backend systems.
2. Scalability: Golang's built-in support for concurrency makes it easy to write scalable applications. Goroutines, lightweight threads, allow developers to write concurrent code without the overhead of traditional threads. This enables the backend to handle a large number of requests efficiently.
3. Reliability: Go has a strong type system and a robust standard library. It provides error handling mechanisms that make it easier to write reliable code. Additionally, Go has built-in support for testing, making it easier to ensure the correctness of the backend code.
4. Simplicity: Go's syntax is simple and easy to understand. It has a minimalistic approach to language design, making it easy for developers to pick up and start building applications quickly. This simplicity also results in code that is easy to read and maintain.
Example:
package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, Golang!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
Related Article: Beego Integration with Bootstrap, Elasticsearch & Databases
Integrating Golang with Frontend Frameworks
Integrating Golang with frontend frameworks like React.js can provide a useful and efficient solution for building web applications. Golang can serve as the backend API, handling data retrieval, processing, and storage, while React.js handles the frontend UI and user interactions. This separation of concerns allows for better code organization, maintainability, and scalability.
To integrate Golang with React.js, you can use Golang frameworks like Beego. Beego is a lightweight and easy-to-use web framework for Golang that provides features like routing, middleware, and ORM (Object-Relational Mapping). It allows you to build RESTful APIs that can be consumed by React.js frontend applications.
Example:
In this example, we will create a simple Beego backend API that serves JSON data to a React.js frontend application.
1. Create a new Beego project:
$ bee new myproject
2. Define a controller that handles requests and serves JSON data:
package controllers import ( "github.com/astaxie/beego" ) type DataController struct { beego.Controller } type Data struct { Message string `json:"message"` } func (c *DataController) Get() { data := Data{ Message: "Hello, React.js!", } c.Data["json"] = data c.ServeJSON() }
3. Define a route in the router.go
file:
package routers import ( "github.com/astaxie/beego" "github.com/myproject/controllers" ) func init() { beego.Router("/data", &controllers.DataController{}) }
4. Start the Beego server:
$ cd myproject $ bee run
5. In your React.js application, make an HTTP request to the Beego API:
import React, { useEffect, useState } from 'react'; function App() { const [message, setMessage] = useState(''); useEffect(() => { fetch('/data') .then(response => response.json()) .then(data => setMessage(data.message)) .catch(error => console.error(error)); }, []); return ( <div> <h1>{message}</h1> </div> ); } export default App;
This example demonstrates how to integrate a Beego backend with a React.js frontend. The Beego backend serves JSON data, which is fetched by the React.js frontend and displayed in the UI.
Understanding Server-Side Rendering and Its Importance
Server-Side Rendering (SSR) is the process of rendering web pages on the server and sending the fully rendered page to the client. This is in contrast to Client-Side Rendering (CSR), where the page is initially rendered with minimal content and the rest is loaded and rendered on the client-side using JavaScript.
SSR offers several advantages:
1. SEO-Friendly: Search engines can crawl and index the fully rendered pages, improving search engine visibility. This is particularly important for websites that rely on organic search traffic.
2. Performance: SSR reduces the time-to-first-content by sending a fully rendered page to the client. This improves perceived performance and user experience, especially on slower networks or devices.
3. Accessibility: SSR ensures that content is available to all users, including those who have JavaScript disabled or are using assistive technologies. This improves accessibility compliance.
4. Social Media Sharing: When sharing a link on social media platforms, the fully rendered page is displayed as a preview, improving click-through rates and user engagement.
Integrating SSR with Beego and React.js can provide the benefits of SSR while leveraging the power and flexibility of React.js for building dynamic and interactive user interfaces.
Example:
In this example, we will use Beego's built-in template engine to render a React.js component on the server and send the fully rendered HTML to the client.
1. Create a new Beego controller:
package controllers import ( "github.com/astaxie/beego" "github.com/astaxie/beego/logs" "html/template" ) type MainController struct { beego.Controller } func (c *MainController) Get() { c.Data["Title"] = "Server-Side Rendering" c.TplName = "index.tpl" }
2. Create a new template file index.tpl
:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>{{.Title}}</title> </head> <body> <div id="root"> {{.ReactComponent}} </div> <script src="/static/js/bundle.js"></script> </body> </html>
3. Create a React.js component file App.js
:
import React from 'react'; function App() { return ( <h1>Hello, Server-Side Rendering!</h1> ); } export default App;
4. Create a server-side rendering endpoint in the Beego router:
package routers import ( "github.com/astaxie/beego" "github.com/myproject/controllers" "github.com/myproject/views" "github.com/astaxie/beego/plugins/cors" ) func init() { beego.Router("/", &controllers.MainController{}) beego.Get("/ssr", views.ServerSideRender) }
5. Create a server-side rendering view views/ssr.go
:
package views import ( "github.com/astaxie/beego" "github.com/myproject/components" "github.com/myproject/render" "html/template" ) func ServerSideRender(ctx *context.Context) { reactComponent := components.App() tmpl, err := template.ParseFiles(beego.ViewsPath + "/index.tpl") if err != nil { beego.Error(err) ctx.Output.Status = http.StatusInternalServerError return } data := struct { Title string ReactComponent template.HTML }{ Title: "Server-Side Rendering", ReactComponent: template.HTML(reactComponent), } err = tmpl.Execute(ctx.ResponseWriter, data) if err != nil { beego.Error(err) ctx.Output.Status = http.StatusInternalServerError return } }
This example demonstrates how to integrate server-side rendering with Beego and React.js. The Beego controller renders a template that includes a placeholder for the React.js component. The server-side rendering endpoint in the Beego router retrieves the React.js component and renders it on the server using the Beego template engine. The fully rendered HTML is then sent to the client.
Optimizing API Endpoints for Better Performance
Optimizing API endpoints is crucial for achieving better performance and scalability in backend systems. Here are some techniques you can apply when building API endpoints with Beego and Golang:
1. Caching: Implement caching mechanisms to store and serve frequently accessed data. Beego provides built-in support for caching using cache adapters like MemoryCache, RedisCache, and FileCache.
2. Pagination: When dealing with large datasets, implement pagination to retrieve data in smaller chunks. This reduces the response size and improves performance.
3. Compression: Enable compression on API responses to reduce the amount of data sent over the network. Beego supports response compression using gzip and deflate.
4. Rate Limiting: Implement rate limiting to prevent abuse and ensure fair usage of your API. Beego provides middleware like "ratelimiter" that allows you to set limits on the number of requests per second.
5. Request Validation: Validate incoming requests to ensure they meet the required criteria. Beego provides validation support through the Valid
struct and validation tags.
Example:
In this example, we will optimize an API endpoint in Beego by implementing caching and pagination.
1. Create a new Beego controller:
package controllers import ( "github.com/astaxie/beego" "github.com/astaxie/beego/cache" "github.com/myproject/models" ) type UserController struct { beego.Controller } var ( UserCache cache.Cache ) func init() { UserCache, _ = cache.NewCache("memory", `{"interval":60}`) } func (c *UserController) Get() { // Check if data is available in cache if data, ok := UserCache.Get("users"); ok { c.Data["json"] = data c.ServeJSON() return } // Retrieve data from the database users := models.GetUsers() // Store data in cache UserCache.Put("users", users, 60) c.Data["json"] = users c.ServeJSON() }
2. Create a new model file models/user.go
:
package models type User struct { ID int `json:"id"` Name string `json:"name"` } func GetUsers() []User { // Simulate fetching data from the database users := []User{ {ID: 1, Name: "John Doe"}, {ID: 2, Name: "Jane Smith"}, {ID: 3, Name: "Bob Johnson"}, } return users }
3. Update the Beego router to include the UserController:
package routers import ( "github.com/astaxie/beego" "github.com/myproject/controllers" ) func init() { beego.Router("/users", &controllers.UserController{}) }
In this example, the UserController implements caching by storing the fetched user data in the UserCache. The cache is checked before fetching the data from the database, improving performance by reducing database queries. The cache is set to expire after 60 seconds. If the cache is not available, the UserController retrieves the data from the database and stores it in the cache before serving the JSON response.
Related Article: Best Practices of Building Web Apps with Gin & Golang
Introduction to the Beego Framework and How It Works
Beego is a high-performance web framework for Golang that follows the Model-View-Controller (MVC) architectural pattern. It provides a set of tools and libraries for building web applications and APIs efficiently.
Key features of Beego include:
1. Router: Beego's router allows you to define URL patterns and map them to controller methods. It supports dynamic routing, regular expressions, and custom route patterns.
2. Controller: Beego's controller handles requests, performs data processing, and renders responses. It provides functions for accessing request parameters, session management, and rendering templates.
3. ORM: Beego's ORM (Object-Relational Mapping) provides a convenient way to interact with databases. It supports multiple database drivers and provides features like automatic table creation, data querying, and data manipulation.
4. Middleware: Beego's middleware system allows you to inject custom logic into the request-response flow. Middleware functions can be used for authentication, logging, input validation, and more.
5. Template Engine: Beego includes a useful template engine that allows you to render dynamic HTML pages. It supports template inheritance, variable substitution, control structures, and custom functions.
6. Validation: Beego provides built-in validation support for request data. It allows you to define validation rules using tags and automatically validates incoming data.
7. Session Management: Beego includes a session management module that provides support for session storage, retrieval, and deletion. It supports different session providers like memory, file, Redis, and MySQL.
Example:
In this example, we will create a simple Beego application that displays a "Hello, Beego!" message.
1. Create a new Beego controller:
package controllers import ( "github.com/astaxie/beego" ) type MainController struct { beego.Controller } func (c *MainController) Get() { c.Data["Message"] = "Hello, Beego!" c.TplName = "index.tpl" }
2. Create a new template file views/index.tpl
:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Hello Beego</title> </head> <body> <h1>{{.Message}}</h1> </body> </html>
3. Update the Beego router to include the MainController:
package routers import ( "github.com/astaxie/beego" "github.com/myproject/controllers" ) func init() { beego.Router("/", &controllers.MainController{}) }
4. Start the Beego server:
$ bee run
5. Open your browser and visit http://localhost:8080/
. You should see the "Hello, Beego!" message.
In this example, the MainController handles the root URL (/
) and sets the Message
variable in the template data. The template file index.tpl
is rendered with the provided data, resulting in the "Hello, Beego!" message being displayed in the browser.
Building Single-Page Applications with React.js
React.js is a popular JavaScript library for building user interfaces. It allows you to create reusable UI components and efficiently update the UI in response to changes in data. React.js is often used to build single-page applications (SPAs) where the entire application runs within a single HTML page.
To build SPAs with React.js, you can use a combination of React Router for handling routing and Beego for serving the initial HTML page and providing the backend API.
Example:
In this example, we will create a simple SPA using React.js and Beego.
1. Create a new Beego controller:
package controllers import ( "github.com/astaxie/beego" ) type MainController struct { beego.Controller } func (c *MainController) Get() { c.TplName = "index.tpl" }
2. Create a new template file views/index.tpl
:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>React SPA</title> <link rel="stylesheet" href="/static/css/styles.css"> </head> <body> <div id="root"></div> <script src="/static/js/bundle.js"></script> </body> </html>
3. Create a new React.js component file static/js/App.js
:
import React from 'react'; function App() { return ( <div> <h1>Welcome to the React SPA!</h1> <p>Click on the links below to navigate:</p> <ul> <li><a href="/home">Home</a></li> <li><a href="/about">About</a></li> <li><a href="/contact">Contact</a></li> </ul> </div> ); } export default App;
4. Create a new Beego controller for the API endpoint:
package controllers import ( "github.com/astaxie/beego" ) type ApiController struct { beego.Controller } func (c *ApiController) Get() { c.Data["json"] = map[string]interface{}{ "message": "This is the API endpoint.", } c.ServeJSON() }
5. Update the Beego router to include the controllers and API endpoint:
package routers import ( "github.com/astaxie/beego" "github.com/myproject/controllers" ) func init() { beego.Router("/", &controllers.MainController{}) beego.Router("/api", &controllers.ApiController{}) beego.Router("/home", &controllers.MainController{}) beego.Router("/about", &controllers.MainController{}) beego.Router("/contact", &controllers.MainController{}) }
6. Install React Router:
$ npm install react-router-dom
7. Create a new React.js component file static/js/Home.js
:
import React from 'react'; function Home() { return ( <div> <h1>Home Page</h1> <p>This is the Home page of the SPA.</p> </div> ); } export default Home;
8. Create a new React.js component file static/js/About.js
:
import React from 'react'; function About() { return ( <div> <h1>About Page</h1> <p>This is the About page of the SPA.</p> </div> ); } export default About;
9. Create a new React.js component file static/js/Contact.js
:
import React from 'react'; function Contact() { return ( <div> <h1>Contact Page</h1> <p>This is the Contact page of the SPA.</p> </div> ); } export default Contact;
10. Create a new React.js component file static/js/index.js
:
import React from 'react'; import ReactDOM from 'react-dom'; import { BrowserRouter as Router, Switch, Route } from 'react-router-dom'; import App from './App'; import Home from './Home'; import About from './About'; import Contact from './Contact'; ReactDOM.render( <Router> <Switch> <Route exact path="/" component={App} /> <Route path="/home" component={Home} /> <Route path="/about" component={About} /> <Route path="/contact" component={Contact} /> </Switch> </Router>, document.getElementById('root') );
11. Build the React.js bundle:
$ npm run build
12. Start the Beego server:
$ bee run
13. Open your browser and visit http://localhost:8080/
. You should see the React SPA with the links to navigate to the Home, About, and Contact pages.
In this example, the MainController serves the initial HTML page that includes the React.js bundle. The React.js bundle is responsible for rendering the App component, which displays the links to navigate to different pages. The Beego router maps the URLs to the MainController, which serves the same HTML page for all routes. React Router handles the client-side routing and renders the appropriate component based on the URL path.
Advantages of Vue.js for Frontend Development
Vue.js is a progressive JavaScript framework for building user interfaces. It is designed to be easy to pick up and integrate with existing projects. Vue.js offers several advantages for frontend development:
1. Lightweight: Vue.js is a lightweight framework, with a size of around 20KB (min+gzip). This makes it fast to download and easy to integrate into projects.
2. Easy Learning Curve: Vue.js has a gentle learning curve, making it easy for developers to start using it. The core library focuses on the view layer only, which makes it easy to understand and use with existing projects.
3. Flexibility: Vue.js is designed to be adaptable and flexible. It allows you to incrementally adopt its features and integrate with existing projects. You can use Vue.js to enhance specific parts of your application or build full-featured single-page applications.
4. Reactivity: Vue.js uses a reactive data-binding system, which allows you to declaratively render the UI based on the underlying data model. When the data changes, Vue.js automatically updates the UI to reflect the changes, making it easy to build dynamic and interactive applications.
5. Component-Based Architecture: Vue.js promotes a component-based architecture, where the UI is broken down into reusable components. This allows for better code organization, reusability, and maintainability. Components in Vue.js can have their own state, methods, and lifecycle hooks.
6. Ecosystem and Community: Vue.js has a thriving ecosystem and a large and active community. It has a wide range of libraries, tools, and plugins available, making it easy to extend the functionality of your Vue.js applications. The community is also very supportive and provides helpful resources and documentation.
Example:
In this example, we will create a simple Vue.js application that displays a list of users fetched from a backend API.
1. Create a new Vue.js component file src/App.vue
:
<template> <div> <h1>User List</h1> <ul> <li v-for="user in users" :key="user.id">{{ user.name }}</li> </ul> </div> </template> <script> export default { data() { return { users: [], }; }, mounted() { fetch('/api/users') .then(response => response.json()) .then(data => this.users = data) .catch(error => console.error(error)); }, }; </script>
2. Create a new Vue.js instance in src/main.js
:
import Vue from 'vue'; import App from './App.vue'; new Vue({ render: h => h(App), }).$mount('#app');
3. Update the Beego router to include the API endpoint:
package routers import ( "github.com/astaxie/beego" "github.com/myproject/controllers" ) func init() { beego.Router("/", &controllers.MainController{}) beego.Router("/api/users", &controllers.UserController{}) }
4. Start the Beego server:
$ bee run
5. Open your browser and visit http://localhost:8080/
. You should see the list of users fetched from the backend API displayed in the Vue.js application.
In this example, the Vue.js application fetches user data from the backend API using the fetch
API. The fetched user data is then rendered in the UI using the v-for
directive to iterate over the users array and display each user's name. The Beego router maps the /api/users
URL to the UserController, which serves the user data from the backend API.
Leveraging HTMX in Beego for Dynamic Server-Side Rendering
HTMX is a library that allows you to add dynamic behavior to your web pages using HTML attributes. It enables server-side rendering with dynamic updates without the need for JavaScript frameworks like React.js or Vue.js. By leveraging HTMX in Beego, you can enhance the interactivity of your web applications while keeping the server-side rendering benefits.
Example:
In this example, we will create a simple Beego application that uses HTMX to implement dynamic behavior.
1. Include the HTMX library in views/index.tpl
:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>HTMX Example</title> <script src="https://unpkg.com/htmx.org@^1.5.0/dist/htmx.js"></script> </head> <body> <h1>HTMX Example</h1> <button hx-get="/api/data" hx-target="#message">Fetch Data</button> <div id="message"></div> </body> </html>
2. Create a new Beego controller for the API endpoint:
package controllers import ( "github.com/astaxie/beego" ) type ApiController struct { beego.Controller } func (c *ApiController) Get() { c.Data["json"] = map[string]interface{}{ "message": "Data fetched from the API endpoint.", } c.ServeJSON() }
3. Update the Beego router to include the API endpoint:
package routers import ( "github.com/astaxie/beego" "github.com/myproject/controllers" ) func init() { beego.Router("/", &controllers.MainController{}) beego.Router("/api/data", &controllers.ApiController{}) }
4. Start the Beego server:
$ bee run
5. Open your browser and visit http://localhost:8080/
. Clicking the "Fetch Data" button will trigger an HTMX request to the /api/data
endpoint. The response from the API endpoint will be displayed in the <div id="message"></div>
element without a page refresh.
In this example, the HTMX library is included in the HTML page using a script tag. The "Fetch Data" button has an hx-get
attribute that specifies the URL to request when clicked. The hx-target
attribute specifies the element where the response should be rendered. When the button is clicked, HTMX makes an AJAX request to the specified API endpoint and updates the content of the target element with the response. The Beego router maps the /api/data
URL to the ApiController, which serves the response to the HTMX request.
Related Article: Deployment and Monitoring Strategies for Gin Apps
Additional Resources
- Alternative frameworks to Beego for building Go web applications