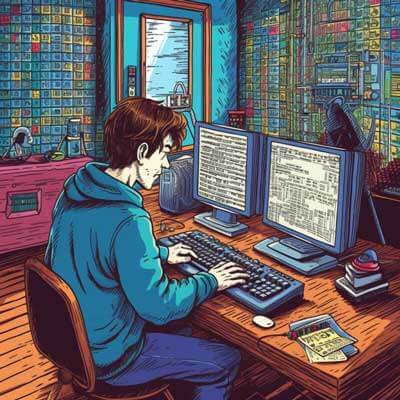
Table of Contents
Understanding the Model-View-Controller (MVC) Pattern
The Model-View-Controller (MVC) pattern is a software architectural pattern that separates an application into three main components: the model, the view, and the controller. This pattern helps to organize code and improve the maintainability of the application.
- The model represents the data and the business logic of the application. It encapsulates the application's data and provides methods for manipulating and accessing that data. In the context of a web application, the model represents the data stored in the database or other data sources.
- The view is responsible for presenting the data to the user. It defines how the data is displayed and provides a user interface for interacting with the application. In the context of a web application, the view is typically implemented using HTML templates or other rendering mechanisms.
- The controller acts as an intermediary between the model and the view. It receives user input from the view, updates the model accordingly, and then updates the view to reflect the changes. In the context of a web application, the controller handles HTTP requests, retrieves and updates data from the model, and renders the appropriate view.
The MVC pattern promotes separation of concerns, as each component has a specific responsibility and can be developed and tested independently. This separation allows for better code organization, reusability, and maintainability.
Let's take a look at an example of how the MVC pattern can be implemented in a Gin application:
package main import ( "github.com/gin-gonic/gin" ) type User struct { ID int Name string } func main() { router := gin.Default() // Define the model var users []User // Define the controller router.GET("/users", func(c *gin.Context) { c.JSON(200, gin.H{"users": users}) }) router.POST("/users", func(c *gin.Context) { var newUser User if err := c.BindJSON(&newUser); err != nil { c.JSON(400, gin.H{"error": "Invalid request payload"}) return } users = append(users, newUser) c.JSON(201, gin.H{"user": newUser}) }) // Define the view // ... router.Run(":8080") }
In this example, we define a User
struct to represent a user in our application. The users
variable serves as the model, storing a collection of users. We define the controller by defining route handlers for the /users
endpoint. The GET /users
handler retrieves the list of users from the model and returns it as a JSON response. The POST /users
handler receives a JSON payload representing a new user, adds it to the model, and returns the newly created user as a JSON response.
The view component is not implemented in this example, as it depends on the specific requirements and technologies used in the application. However, in a Gin application, the view component is typically implemented using HTML templates or other rendering mechanisms provided by the framework.
Related Article: Golang Tutorial for Backend Development
Implementing the Model-View-ViewModel (MVVM) Pattern in a Gin Application
The Model-View-ViewModel (MVVM) pattern is a software architectural pattern that is an extension of the Model-View-Controller (MVC) pattern. It is commonly used in the development of user interfaces, particularly in the context of desktop and mobile applications.
The MVVM pattern introduces an additional component called the ViewModel, which acts as a mediator between the model and the view. The ViewModel contains the presentation logic and state of the view, and exposes this information to the view through data binding. It also handles user input and updates the model accordingly.
- The model represents the data and the business logic of the application. It encapsulates the application's data and provides methods for manipulating and accessing that data.
- The view is responsible for presenting the data to the user. It defines how the data is displayed and provides a user interface for interacting with the application.
- The ViewModel acts as a bridge between the model and the view. It exposes the necessary data and functionality from the model to the view and handles user input.
The MVVM pattern promotes separation of concerns and improves the testability and maintainability of the application. It also enables a more declarative and reactive approach to building user interfaces, as changes in the model are automatically reflected in the view through data binding.
Let's take a look at an example of how the MVVM pattern can be implemented in a Gin application:
package main import ( "github.com/gin-gonic/gin" ) type User struct { ID int Name string } type UserViewModel struct { Users []User } func main() { router := gin.Default() // Define the model var users []User // Define the ViewModel viewModel := UserViewModel{ Users: users, } // Define the controller router.GET("/users", func(c *gin.Context) { c.JSON(200, gin.H{"users": viewModel.Users}) }) router.POST("/users", func(c *gin.Context) { var newUser User if err := c.BindJSON(&newUser); err != nil { c.JSON(400, gin.H{"error": "Invalid request payload"}) return } viewModel.Users = append(viewModel.Users, newUser) c.JSON(201, gin.H{"user": newUser}) }) // Define the view // ... router.Run(":8080") }
In this example, we introduce a UserViewModel
struct that encapsulates the state and presentation logic of the view. The UserViewModel
contains a reference to the User
model and exposes the necessary data and functionality to the view. The UserViewModel
is initialized with the initial state of the model.
The controller and view components in this example are similar to the ones in the MVC example. However, the view interacts with the ViewModel instead of directly accessing the model. This allows the view to automatically update when the state of the ViewModel changes.
Exploring the Factory Pattern for Handling Database Sessions in Gin
The Factory pattern is a creational design pattern that provides an interface for creating objects, but lets subclasses decide which class to instantiate. It abstracts the process of object creation and allows the client code to work with objects without knowing their concrete types.
In the context of a Gin application, the Factory pattern can be used to handle the creation of database sessions. A database session represents a connection to a database and provides methods for querying and manipulating data.
Let's take a look at an example of how the Factory pattern can be used to handle database sessions in a Gin application:
package main import ( "database/sql" "fmt" "log" "github.com/gin-gonic/gin" _ "github.com/go-sql-driver/mysql" ) type DatabaseSessionFactory interface { CreateSession() (*sql.DB, error) } type MySQLSessionFactory struct { connectionString string } func (f *MySQLSessionFactory) CreateSession() (*sql.DB, error) { db, err := sql.Open("mysql", f.connectionString) if err != nil { return nil, err } // Configure database session settings db.SetMaxOpenConns(100) db.SetMaxIdleConns(10) return db, nil } func main() { router := gin.Default() // Create a database session factory sessionFactory := &MySQLSessionFactory{ connectionString: "user:password@tcp(localhost:3306)/database", } // Define the controller router.GET("/users", func(c *gin.Context) { // Create a new database session using the factory db, err := sessionFactory.CreateSession() if err != nil { log.Fatal(err) } defer db.Close() // Use the database session to query data rows, err := db.Query("SELECT * FROM users") if err != nil { log.Fatal(err) } defer rows.Close() // Process the query results for rows.Next() { var id int var name string if err := rows.Scan(&id, &name); err != nil { log.Fatal(err) } fmt.Printf("User ID: %d, Name: %s\n", id, name) } c.JSON(200, gin.H{"message": "Users retrieved successfully"}) }) router.Run(":8080") }
In this example, we define a DatabaseSessionFactory
interface that declares a CreateSession
method for creating database sessions. We then implement the DatabaseSessionFactory
interface with a MySQLSessionFactory
struct, which creates MySQL database sessions using the provided connection string.
In the controller, we create a new database session using the factory's CreateSession
method. We can then use the database session to query data from the database. Once we're done with the database session, we close it to release the underlying resources.
Utilizing the Singleton Pattern for Managing Database Sessions in Gin
The Singleton pattern is a creational design pattern that ensures that a class has only one instance and provides a global point of access to that instance. It is often used in situations where there should be only one instance of a particular class, such as managing database connections or configuration settings.
In the context of a Gin application, the Singleton pattern can be used to manage database sessions and ensure that there is only one instance of the session throughout the application. This can help improve performance and resource usage, as multiple instances of the session are not created unnecessarily.
Let's take a look at an example of how the Singleton pattern can be used to manage database sessions in a Gin application:
package main import ( "database/sql" "fmt" "log" "sync" "github.com/gin-gonic/gin" _ "github.com/go-sql-driver/mysql" ) type DatabaseSession struct { db *sql.DB } var once sync.Once var session *DatabaseSession func GetDatabaseSession() *DatabaseSession { once.Do(func() { db, err := sql.Open("mysql", "user:password@tcp(localhost:3306)/database") if err != nil { log.Fatal(err) } // Configure database session settings db.SetMaxOpenConns(100) db.SetMaxIdleConns(10) session = &DatabaseSession{db: db} }) return session } func main() { router := gin.Default() // Define the controller router.GET("/users", func(c *gin.Context) { // Get the database session db := GetDatabaseSession().db // Use the database session to query data rows, err := db.Query("SELECT * FROM users") if err != nil { log.Fatal(err) } defer rows.Close() // Process the query results for rows.Next() { var id int var name string if err := rows.Scan(&id, &name); err != nil { log.Fatal(err) } fmt.Printf("User ID: %d, Name: %s\n", id, name) } c.JSON(200, gin.H{"message": "Users retrieved successfully"}) }) router.Run(":8080") }
In this example, we define a DatabaseSession
struct that encapsulates a database session. We use the sync.Once
type to ensure that the GetDatabaseSession
function is only called once, creating a single instance of the DatabaseSession
. The DatabaseSession
instance is stored in the session
variable and returned whenever GetDatabaseSession
is called.
In the controller, we retrieve the database session by calling the GetDatabaseSession
function. We can then use the database session to query data from the database. The GetDatabaseSession
function ensures that there is only one instance of the session throughout the application, improving performance and resource usage.
Related Article: Best Practices for Building Web Apps with Beego & Golang
Enhancing Middleware Functionality with the Decorator Pattern in Gin
The Decorator pattern is a structural design pattern that allows behavior to be added to an object dynamically. It provides a flexible alternative to subclassing for extending functionality.
In the context of a Gin application, the Decorator pattern can be used to enhance the functionality of middleware functions. Middleware functions in Gin are used to perform tasks such as logging, authentication, error handling, and more.
Let's take a look at an example of how the Decorator pattern can be used to enhance the functionality of middleware functions in a Gin application:
package main import ( "github.com/gin-gonic/gin" ) func LoggerMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Perform logging before calling the next middleware function log.Println("Request received") // Call the next middleware function c.Next() // Perform logging after calling the next middleware function log.Println("Request completed") } } func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Perform authentication before calling the next middleware function if !isAuthenticated(c) { c.AbortWithStatus(401) return } // Call the next middleware function c.Next() } } func isAuthenticated(c *gin.Context) bool { // Check if the user is authenticated // ... return true } func main() { router := gin.Default() // Use the LoggerMiddleware as a global middleware router.Use(LoggerMiddleware()) // Define routes and their associated middleware router.GET("/public", func(c *gin.Context) { c.JSON(200, gin.H{"message": "This is a public endpoint"}) }) router.GET("/private", AuthMiddleware(), func(c *gin.Context) { c.JSON(200, gin.H{"message": "This is a private endpoint"}) }) router.Run(":8080") }
In this example, we define two middleware functions: LoggerMiddleware
and AuthMiddleware
. The LoggerMiddleware
logs the start and completion of each request, while the AuthMiddleware
performs authentication before allowing access to certain endpoints.
The LoggerMiddleware
and AuthMiddleware
functions return gin.HandlerFunc
types, which are the types expected by Gin for middleware functions. Inside each middleware function, we perform the desired functionality before and after calling the next middleware function using the c.Next()
function.
We then use the router.Use
function to apply the LoggerMiddleware
as a global middleware to all routes in the application. This ensures that the logging functionality is applied to every request.
For the /public
route, we don't apply any additional middleware. For the /private
route, we apply the AuthMiddleware
to perform authentication before allowing access to the endpoint.
Exploring Middleware in the Gin Framework
Middleware functions in the Gin framework are used to perform tasks such as logging, authentication, error handling, and more. Middleware functions are executed in the order they are registered and can modify the request or response objects before they are passed to the next middleware function or route handler.
Gin provides a flexible and useful middleware system that allows developers to easily add and configure middleware functions in their applications. Middleware functions in Gin are implemented as functions that take a gin.HandlerFunc
type as an argument and return a gin.HandlerFunc
type.
The gin.HandlerFunc
type is a function type that represents a Gin route handler. It takes a *gin.Context
object as an argument and returns nothing. Middleware functions can modify the request or response objects, call the next middleware function or route handler using c.Next()
, or abort the request using c.Abort()
or c.AbortWithStatus()
.
Let's take a look at an example of how middleware functions can be used in a Gin application:
package main import ( "github.com/gin-gonic/gin" ) func LoggerMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Perform logging before calling the next middleware function log.Println("Request received") // Call the next middleware function c.Next() // Perform logging after calling the next middleware function log.Println("Request completed") } } func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Perform authentication before calling the next middleware function if !isAuthenticated(c) { c.AbortWithStatus(401) return } // Call the next middleware function c.Next() } } func isAuthenticated(c *gin.Context) bool { // Check if the user is authenticated // ... return true } func main() { router := gin.Default() // Use the LoggerMiddleware as a global middleware router.Use(LoggerMiddleware()) // Define routes and their associated middleware router.GET("/public", func(c *gin.Context) { c.JSON(200, gin.H{"message": "This is a public endpoint"}) }) router.GET("/private", AuthMiddleware(), func(c *gin.Context) { c.JSON(200, gin.H{"message": "This is a private endpoint"}) }) router.Run(":8080") }
In this example, we define two middleware functions: LoggerMiddleware
and AuthMiddleware
. The LoggerMiddleware
logs the start and completion of each request, while the AuthMiddleware
performs authentication before allowing access to certain endpoints.
The LoggerMiddleware
and AuthMiddleware
functions return gin.HandlerFunc
types, which are the types expected by Gin for middleware functions. Inside each middleware function, we perform the desired functionality before and after calling the next middleware function using the c.Next()
function.
We use the router.Use
function to apply the LoggerMiddleware
as a global middleware to all routes in the application. This ensures that the logging functionality is applied to every request.
For the /public
route, we don't apply any additional middleware. For the /private
route, we apply the AuthMiddleware
to perform authentication before allowing access to the endpoint.
Gin executes middleware functions in the order they are registered. In this example, the LoggerMiddleware
is called first, followed by the AuthMiddleware
, and then the route handler for each request.
Middleware functions in Gin provide a useful and flexible way to add functionality to the request-response cycle. They can be used for tasks such as logging, authentication, error handling, and more. Middleware functions can modify the request or response objects, call the next middleware function or route handler, or abort the request if necessary.
Implementing Route Handlers in Gin
Route handlers in the Gin framework are functions that handle HTTP requests and generate HTTP responses. They define the behavior of the application for specific routes and are the main building blocks of a Gin application.
In Gin, route handlers are implemented as functions that take a *gin.Context
object as an argument and return nothing. The *gin.Context
object provides access to the request and response objects, as well as utility methods for handling HTTP requests and generating HTTP responses.
Let's take a look at an example of how route handlers can be implemented in a Gin application:
package main import ( "github.com/gin-gonic/gin" ) func main() { router := gin.Default() // Define a route handler for the GET /hello endpoint router.GET("/hello", func(c *gin.Context) { // Generate a JSON response with a message c.JSON(200, gin.H{"message": "Hello, World!"}) }) // Define a route handler for the POST /users endpoint router.POST("/users", func(c *gin.Context) { // Parse the request body into a JSON object var newUser struct { Name string `json:"name"` } if err := c.ShouldBindJSON(&newUser); err != nil { // Return a JSON response with an error message c.JSON(400, gin.H{"error": "Invalid request payload"}) return } // Create a new user in the database // ... // Return a JSON response with the new user c.JSON(201, gin.H{"user": newUser}) }) router.Run(":8080") }
In this example, we define two route handlers: one for the GET /hello
endpoint and another for the POST /users
endpoint.
The GET /hello
route handler generates a JSON response with a message by calling the c.JSON
method on the *gin.Context
object. The c.JSON
method takes an HTTP status code and a JSON object as arguments, and automatically sets the appropriate content type header.
The POST /users
route handler parses the request body into a JSON object by calling the c.ShouldBindJSON
method on the *gin.Context
object. The ShouldBindJSON
method binds the request body to the newUser
variable, which is a struct with a Name
field. If the request body is not a valid JSON object or does not contain the expected fields, an error response is returned.
Once the request body is parsed and validated, the route handler can perform additional logic, such as creating a new user in the database. In this example, the logic for creating a new user is omitted for brevity.
Finally, the route handler returns a JSON response with the new user by calling the c.JSON
method on the *gin.Context
object. The response includes an HTTP status code and a JSON object representing the new user.
Route handlers in Gin provide a simple and intuitive way to handle HTTP requests and generate HTTP responses. They can be used to implement the behavior of the application for specific routes and are the main building blocks of a Gin application.
Benefits of Using the MVC Pattern in a Gin Application
The Model-View-Controller (MVC) pattern provides a structured approach to designing and organizing software applications. It separates an application into three main components: the model, the view, and the controller. Each component has a specific responsibility and can be developed and tested independently, promoting code reusability and maintainability.
Using the MVC pattern in a Gin application offers several benefits:
- Separation of concerns: The MVC pattern separates the data, presentation, and control logic of the application into separate components. This separation allows for better code organization and makes it easier to understand, modify, and test the different parts of the application independently.
- Code reusability: The MVC pattern promotes code reusability, as each component can be developed and tested independently. This allows for the reuse of models, views, and controllers across different parts of the application, reducing development time and effort.
- Maintainability: The MVC pattern improves the maintainability of the application by providing clear separation between the different components. This makes it easier to modify and extend the application over time, as changes in one component are less likely to impact other components.
- Scalability: The MVC pattern allows for better scalability of the application, as each component can be scaled independently. For example, if the application experiences increased traffic, additional instances of the controller or view components can be added without affecting the model component.
- Testability: The MVC pattern improves the testability of the application, as each component can be tested independently. This allows for more focused and targeted testing, making it easier to identify and fix bugs.
- Flexibility: The MVC pattern provides a flexible and modular architecture, allowing for easy integration of third-party libraries and frameworks. This makes it easier to leverage existing tools and technologies and extends the capabilities of the application.
Related Article: Deployment and Monitoring Strategies for Gin Apps
Benefits of Using the MVVM Pattern in a Gin Application
The Model-View-ViewModel (MVVM) pattern is an extension of the Model-View-Controller (MVC) pattern that is commonly used in the development of user interfaces, particularly in the context of desktop and mobile applications. The MVVM pattern introduces an additional component called the ViewModel, which acts as a mediator between the model and the view.
Using the MVVM pattern in a Gin application offers several benefits:
- Separation of concerns: The MVVM pattern separates the data, presentation, and control logic of the application into separate components. This separation allows for better code organization and makes it easier to understand, modify, and test the different parts of the application independently.
- Declarative and reactive UI: The MVVM pattern enables a more declarative and reactive approach to building user interfaces. Changes in the model are automatically reflected in the view through data binding, eliminating the need for manual updates and reducing the risk of bugs.
- Testability: The MVVM pattern improves the testability of the application, as each component can be tested independently. The ViewModel can be easily mocked or stubbed to isolate and test the behavior of the view.
- Code reusability: The MVVM pattern promotes code reusability, as the ViewModel encapsulates the state and behavior of the view. The same ViewModel can be used in different views, reducing development time and effort.
- Maintainability: The MVVM pattern improves the maintainability of the application by providing clear separation between the different components. Changes in one component are less likely to impact other components, making it easier to modify and extend the application over time.
- Scalability: The MVVM pattern allows for better scalability of the application, as each component can be scaled independently. For example, if the application experiences increased traffic, additional instances of the ViewModel or view components can be added without affecting the model component.
Best Practices for Implementing Route Handlers in Gin
Implementing route handlers in the Gin framework requires careful consideration of best practices to ensure the performance, security, and maintainability of the application. Here are some best practices for implementing route handlers in Gin:
- Keep route handlers small and focused: Route handlers should be kept small and focused on a specific task or functionality. This makes the code easier to understand, test, and maintain. If a route handler becomes too large or complex, consider refactoring it into smaller, more manageable functions.
- Validate and sanitize input: Route handlers should validate and sanitize input from the client to ensure the security and integrity of the application. Use the built-in validation and binding features of Gin to validate and bind request parameters, query strings, and request bodies. Additionally, sanitize user input to prevent common security vulnerabilities such as cross-site scripting (XSS) attacks.
- Use appropriate HTTP status codes: Route handlers should return appropriate HTTP status codes to indicate the result of the request. Use the c.JSON
method to return JSON responses with the appropriate status code. For example, use c.JSON(200, gin.H{"message": "Success"})
for successful responses, c.JSON(400, gin.H{"error": "Bad Request"})
for client errors, and c.JSON(500, gin.H{"error": "Internal Server Error"})
for server errors.
- Handle errors gracefully: Route handlers should handle errors gracefully and return informative error messages to the client. Use the c.Error
method to add errors to the Gin context, and use the built-in error handling middleware or a custom error handling middleware to handle and log errors. Avoid exposing sensitive information in error messages and consider using error codes or error IDs for easier debugging.
- Use appropriate HTTP methods and route names: Route handlers should use appropriate HTTP methods and route names to adhere to RESTful API design principles. Use the router.GET
, router.POST
, router.PUT
, router.DELETE
, and other methods provided by Gin to define routes with the appropriate HTTP methods. Use descriptive route names that accurately describe the functionality of the route.
- Implement proper access control: Route handlers should implement proper access control to restrict access to sensitive routes or data. Use middleware functions, such as authentication middleware, to enforce access control policies. Consider using role-based access control (RBAC) or other authorization mechanisms to control access to different routes or resources.
- Use structured logging: Route handlers should use structured logging to log important events and information. Use the built-in logging middleware provided by Gin or a custom logging middleware to log requests, responses, and errors. Consider using a structured logging library, such as Zap or Logrus, to log data in a structured format for easier analysis and monitoring.
- Implement pagination and filtering: If route handlers return large collections of data, consider implementing pagination and filtering mechanisms to reduce the amount of data returned to the client. Use query parameters to allow clients to specify the number of items per page, the page number, and any filtering criteria.
- Implement proper error handling and error responses: Route handlers should handle errors properly and return informative error responses to the client. Use the built-in error handling middleware provided by Gin or a custom error handling middleware to handle and log errors. Return JSON responses with appropriate error messages and status codes to help clients understand and handle errors.
Alternative Frameworks for Building Web Applications in Go
While Gin is a popular web framework for building web applications in Go, there are also several alternative frameworks available that provide different features, performance characteristics, and programming models. Here are some alternative frameworks for building web applications in Go:
- Echo: Echo is a fast and minimalist web framework for Go that is known for its simplicity and speed. It is designed to be easy to use and has a small and concise API. Echo offers features such as routing, middleware support, request/response handling, and more. It is a good choice for developers who prefer a lightweight and performant framework.
- Revel: Revel is a full-featured web framework for Go that follows the Model-View-Controller (MVC) architectural pattern. It provides a high productivity development environment with features such as automatic code generation, hot code reloading, and a built-in testing framework. Revel is a good choice for developers who prefer a framework with batteries included and built-in support for common web application tasks.
- Buffalo: Buffalo is a web framework for Go that is designed to be simple, productive, and performant. It follows the Model-View-Controller (MVC) architectural pattern and provides features such as routing, middleware support, database integration, asset management, and more. Buffalo aims to provide a complete development ecosystem for building modern web applications in Go.
- Iris: Iris is a fast and flexible web framework for Go that is known for its performance and extensibility. It provides a rich set of features, including routing, middleware support, request/response handling, database integration, and more. Iris is designed to be efficient and scalable, making it a good choice for high-performance web applications.
- Gorilla: Gorilla is a set of packages for building web applications in Go. It provides packages for routing, sessions, websockets, authentication, and more. Gorilla packages are designed to be modular and can be used together or independently. Gorilla is a good choice for developers who prefer a modular approach and want to pick and choose the packages they need.
These are just a few examples of alternative frameworks for building web applications in Go. Each framework has its own strengths and weaknesses, and the choice of framework depends on the specific requirements and preferences of the project. It is recommended to evaluate the features, performance characteristics, and community support of each framework before making a decision.
Other Commonly Used Design Patterns in Go Programming
In addition to the MVC and MVVM patterns, there are several other commonly used design patterns in Go programming. These design patterns provide solutions to common software design problems and promote code reusability, maintainability, and flexibility. Here are some commonly used design patterns in Go:
- Singleton: The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. It is commonly used for managing shared resources, such as database connections or configuration settings.
- Factory: The Factory pattern provides an interface for creating objects, but lets subclasses decide which class to instantiate. It is commonly used for encapsulating object creation logic and promoting code reusability.
- Builder: The Builder pattern provides a way to construct complex objects step by step. It is commonly used for creating objects with many configurable options or parameters.
- Observer: The Observer pattern defines a one-to-many dependency between objects, so that when one object changes state, all its dependents are notified and updated automatically. It is commonly used for implementing event-driven systems or for decoupling components in an application.
- Strategy: The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It allows the algorithm to vary independently from clients that use it. It is commonly used for encapsulating different algorithms or behaviors and allowing them to be easily swapped or extended.
- Decorator: The Decorator pattern allows behavior to be added to an object dynamically. It provides a flexible alternative to subclassing for extending functionality. It is commonly used for adding additional functionality to objects without modifying their implementation.
- Composite: The Composite pattern allows objects to be composed into tree structures to represent part-whole hierarchies. It is commonly used for treating individual objects and compositions of objects uniformly.
These are just a few examples of commonly used design patterns in Go programming. Each pattern addresses specific software design problems and provides a solution that promotes code reusability, maintainability, and flexibility. It is recommended to study and understand these design patterns to become a more effective and proficient Go developer.
Related Article: Implementing Real-time Features with Gin & Golang
Integrating Authentication Middleware into a Gin Application
Authentication is an important aspect of many web applications, as it ensures that users are who they claim to be and provides access control to resources and functionality. Gin provides a flexible middleware system that allows developers to easily integrate authentication into their applications.
To integrate authentication middleware into a Gin application, you can follow these steps:
1. Define an authentication middleware function that checks if the user is authenticated and sets the appropriate context values or headers. The authentication middleware can use different authentication mechanisms, such as token-based authentication, session-based authentication, or OAuth.
2. Register the authentication middleware globally or for specific routes using the router.Use
or router.Group
methods. The router.Use
method registers the middleware globally, while the router.Group
method allows you to group routes and apply the middleware to the group.
3. Use the authentication information in the route handlers to authorize access to resources or functionality. You can access the authentication information from the context using c.Get
or c.MustGet
methods.
Here is an example of how authentication middleware can be integrated into a Gin application:
package main import ( "github.com/gin-gonic/gin" ) func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Check if the user is authenticated if !isAuthenticated(c) { c.AbortWithStatus(401) return } // Set the user ID in the context for future use c.Set("userID", getUserID(c)) c.Next() } } func isAuthenticated(c *gin.Context) bool { // Check if the user is authenticated // ... return true } func getUserID(c *gin.Context) int { // Get the user ID from the authentication information // ... return 1 } func main() { router := gin.Default() // Register the authentication middleware globally router.Use(AuthMiddleware()) // Define routes that require authentication router.GET("/profile", func(c *gin.Context) { userID := c.MustGet("userID").(int) // Use the user ID to retrieve the user profile // ... c.JSON(200, gin.H{"userID": userID}) }) router.Run(":8080") }
In this example, we define an AuthMiddleware
function that checks if the user is authenticated and sets the user ID in the context using c.Set
. If the user is not authenticated, the middleware aborts the request with a 401 status code.
We register the authentication middleware globally using router.Use
to apply it to all routes in the application. Alternatively, we can use router.Group
to apply the middleware to a specific group of routes.
In the /profile
route handler, we retrieve the user ID from the context using c.MustGet
and use it to retrieve the user profile. We can access the user ID in other route handlers in a similar way.
Popular Go Libraries for Use with Gin
Gin is a useful web framework for building web applications in Go. It provides a rich set of features and functionality out of the box, such as routing, middleware support, request/response handling, and more. However, there are also several popular Go libraries that can be used in conjunction with Gin to further enhance its capabilities. Here are some popular Go libraries for use with Gin:
- GORM: GORM is an object-relational mapping (ORM) library for Go that provides a convenient way to interact with databases. It supports a wide range of database systems, including MySQL, PostgreSQL, SQLite, and more. GORM integrates seamlessly with Gin and provides features such as model definition, database migrations, querying, and more.
- JWT-Go: JWT-Go is a JSON Web Token (JWT) library for Go that provides a simple way to encode and decode JWTs. JWTs are commonly used for authentication and authorization in web applications. JWT-Go can be used with Gin to implement token-based authentication and authorization.
- Casbin: Casbin is an authorization library for Go that provides support for access control models such as Role-Based Access Control (RBAC), Attribute-Based Access Control (ABAC), and more. It allows developers to define and enforce access control policies in their applications. Casbin can be used with Gin to implement fine-grained access control and authorization.
- Viper: Viper is a configuration management library for Go that provides support for reading configuration files, environment variables, and command-line flags. It allows developers to easily manage and access application configuration settings. Viper can be used with Gin to configure various aspects of the application, such as database connections, logging settings, and more.
- Zap: Zap is a fast and structured logging library for Go that provides high-performance logging capabilities. It supports logging at different levels of severity, logging to multiple outputs, and structured logging. Zap can be used with Gin to log important events and information in the application.
- Go-Cache: Go-Cache is an in-memory key-value store library for Go that provides a simple and efficient caching solution. It allows developers to cache frequently accessed data and improve the performance of their applications. Go-Cache can be used with Gin to cache data at different levels, such as request-level caching or global caching.
These are just a few examples of popular Go libraries that can be used in conjunction with Gin to enhance its capabilities. Each library provides additional functionality and features that can be used to build more robust and scalable web applications. It is recommended to evaluate the features, performance characteristics, and community support of each library before integrating them into a Gin application.
Additional Resources
- Gin - HTTP web framework for Go