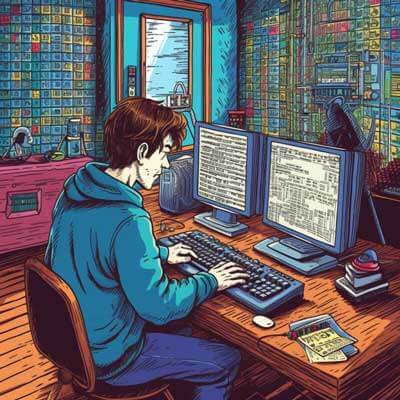
Table of Contents
Web Development in Go
Go, also known as Golang, is a popular programming language developed by Google. It is known for its simplicity, efficiency, and strong support for concurrent programming. Go is gaining traction in the web development community due to its fast execution speed and easy deployment.
Web development in Go involves building web applications using the Go programming language. This includes creating web servers, defining routes, handling HTTP requests and responses, and interacting with databases. Go provides a robust standard library that includes packages for handling various web-related tasks, making it a suitable choice for web development.
Related Article: Optimizing and Benchmarking Beego ORM in Golang
Introduction to Beego Framework
Beego is a useful and flexible web framework for building web applications in Go. It follows the Model-View-Controller (MVC) architectural pattern and provides a set of tools and libraries to simplify web development. Beego is designed to be easy to use, highly scalable, and efficient.
Beego provides a comprehensive set of features, including URL routing, request handling, session management, template rendering, and database integration. It also supports various authentication and authorization mechanisms, making it suitable for building secure web applications.
Installation
To get started with Beego, you need to install it on your machine. Here are the steps to install Beego:
1. Install Go: Beego is a Go framework, so you need to have Go installed on your machine. You can download and install Go from the official Go website (https://golang.org/dl/).
2. Set up the Go workspace: Go uses a workspace to organize your Go projects. Create a directory for your Go workspace and set the GOPATH environment variable to point to this directory.
3. Install Beego: Open a terminal or command prompt and run the following command to install Beego and its command-line tool, Bee:
go get -u github.com/astaxie/beego
4. Verify the installation: To verify that Beego is installed correctly, run the following command:
bee version
If the installation was successful, you should see the version number of Beego and the Bee command-line tool.
Understanding the Model-View-Controller Pattern
The Model-View-Controller (MVC) pattern is a widely used architectural pattern for designing web applications. It separates the application logic into three interconnected components: the Model, the View, and the Controller.
The Model represents the data and business logic of the application. It encapsulates the data and provides methods to manipulate and retrieve it. In a web application, the Model typically interacts with a database or other data sources.
The View is responsible for rendering the user interface of the application. It presents the data from the Model to the user and captures user input. In a web application, the View is usually implemented using HTML templates.
The Controller handles user requests and updates the Model and the View accordingly. It contains the application logic and orchestrates the interaction between the Model and the View. In a web application, the Controller receives HTTP requests, performs the necessary operations on the Model, and selects the appropriate View to render.
Implementing the MVC pattern in Beego involves creating models, views, and controllers and defining routes to handle incoming requests. Here's an example of how to implement the MVC pattern in Beego:
1. Define the Model: Create a Go struct that represents the data of your application. Add methods to manipulate and retrieve the data. For example:
type User struct { ID int Name string } func (u *User) Save() error { // Save the user to the database return nil } func (u *User) Delete() error { // Delete the user from the database return nil }
2. Define the View: Create an HTML template that defines the user interface of your application. Use Beego's template engine to render the template with data from the Model. For example:
<!-- views/user.tpl --> <html> <head> <title>User Details</title> </head> <body> <h1>User Details</h1> <p>ID: {{.ID}}</p> <p>Name: {{.Name}}</p> </body> </html>
3. Define the Controller: Create a Go struct that implements Beego's Controller interface. Add methods to handle incoming requests and update the Model and the View. For example:
type UserController struct { beego.Controller } func (c *UserController) Get() { // Get the user ID from the request parameters userID := c.GetString("id") // Get the user from the database user := &User{ ID: userID, Name: "John Doe", } // Render the user details view with the user data c.Data["User"] = user c.TplName = "user.tpl" }
4. Define the route: In the main function of your Beego application, define a route that maps a URL pattern to the Controller's method. For example:
func main() { beego.Router("/user/:id", &UserController{}, "get:Get") beego.Run() }
This example demonstrates a simple implementation of the MVC pattern in Beego. By following this pattern, you can build scalable and maintainable web applications.
Related Article: Integrating Payment, Voice and Text with Beego & Golang
Implementing Singleton Pattern in Go
The Singleton pattern is a creational design pattern that ensures a class has only one instance and provides a global point of access to that instance. It is commonly used in scenarios where there should be only one instance of a particular class throughout the application.
In Go, the Singleton pattern can be implemented using a combination of an exported struct and a package-level variable. Here's an example:
// singleton.go package singleton import "sync" type Singleton struct { // Add any necessary fields here } var instance *Singleton var once sync.Once func GetInstance() *Singleton { once.Do(func() { instance = &Singleton{} }) return instance }
In this example, we define a struct named Singleton
and a package-level variable named instance
of type *Singleton
. We also declare a sync.Once
variable named once
, which ensures that the initialization code is executed only once.
The GetInstance
function is responsible for returning the singleton instance. It uses the once.Do
function to ensure that the initialization code is executed only once. If the instance
variable is nil
, the initialization code creates a new instance of the Singleton
struct and assigns it to the instance
variable. Subsequent calls to GetInstance
will return the same instance.
To use the Singleton, you can simply import the singleton
package and call the GetInstance
function. Here's an example:
// main.go package main import ( "fmt" "singleton" ) func main() { instance := singleton.GetInstance() fmt.Println(instance) }
In this example, we import the singleton
package and call the GetInstance
function to get the singleton instance. We then print the instance to the console.
Implementing Factory Pattern in Go
The Factory pattern is a creational design pattern that provides an interface for creating objects, but allows subclasses to decide which class to instantiate. It is commonly used when you want to hide the details of object creation and provide a way to create objects without specifying their concrete classes.
In Go, the Factory pattern can be implemented using a combination of an interface and multiple implementations. Here's an example:
// factory.go package factory type Shape interface { Draw() string } type Circle struct{} func (c *Circle) Draw() string { return "Drawing a circle" } type Square struct{} func (s *Square) Draw() string { return "Drawing a square" } type ShapeFactory struct{} func (f *ShapeFactory) CreateShape(shapeType string) Shape { switch shapeType { case "circle": return &Circle{} case "square": return &Square{} default: return nil } }
In this example, we define an interface named Shape
that declares a method named Draw
. We then define two structs, Circle
and Square
, that implement the Shape
interface.
The ShapeFactory
struct is responsible for creating instances of the Shape
interface. It has a method named CreateShape
that takes a shapeType
parameter and returns an instance of the Shape
interface. Depending on the value of the shapeType
parameter, the CreateShape
method creates and returns an instance of the corresponding shape.
To use the Factory, you can simply import the factory
package and create an instance of the ShapeFactory
. You can then call the CreateShape
method to create instances of different shapes. Here's an example:
// main.go package main import ( "fmt" "factory" ) func main() { factory := &factory.ShapeFactory{} circle := factory.CreateShape("circle") fmt.Println(circle.Draw()) square := factory.CreateShape("square") fmt.Println(square.Draw()) }
In this example, we import the factory
package and create an instance of the ShapeFactory
. We then call the CreateShape
method to create instances of a circle and a square. We finally call the Draw
method on each shape and print the result to the console.
Exploring the Middleware Pattern in Beego
The Middleware pattern is a behavioral design pattern that allows you to add additional functionality to an existing object or function without modifying its structure. It is commonly used in web development to perform tasks such as authentication, logging, and error handling.
In Beego, middleware can be implemented using filters. Filters are functions that are executed before or after a request is handled by a controller. They can be used to perform common tasks such as authentication, logging, and request parsing.
To implement middleware in Beego, you need to define a filter function and register it with the Beego framework. Here's an example:
// main.go package main import ( "github.com/astaxie/beego" ) func AuthFilter(ctx *context.Context) { // Perform authentication logic here // For example, check if the user is logged in if !isLoggedIn(ctx) { // Redirect the user to the login page ctx.Redirect(302, "/login") return } // Call the next filter or the controller handler ctx.Next() } func main() { beego.InsertFilter("/*", beego.BeforeRouter, AuthFilter) beego.Run() }
In this example, we define a filter function named AuthFilter
. This function takes a context.Context
parameter, which represents the current request context. Inside the filter function, we perform the authentication logic. If the user is not logged in, we redirect them to the login page. Otherwise, we call the Next
method to pass the request to the next filter or the controller handler.
To register the filter function with the Beego framework, we use the InsertFilter
function. The first parameter of InsertFilter
specifies the URL pattern for which the filter should be applied. In this example, we use "/*"
to apply the filter to all routes. The second parameter specifies the filter position. In this example, we use beego.BeforeRouter
to apply the filter before the router handles the request.
Understanding the Decorator Pattern in Beego
The Decorator pattern is a structural design pattern that allows you to add new functionality to an existing object dynamically. It is commonly used when you want to extend the behavior of an object without modifying its structure.
In Beego, the Decorator pattern can be implemented using filters. Filters are functions that are executed before or after a request is handled by a controller. They can be used to modify the request or response objects, add additional data, or perform other operations.
To implement the Decorator pattern in Beego, you need to define a filter function and register it with the Beego framework. Here's an example:
// main.go package main import ( "github.com/astaxie/beego" ) func AddHeaderFilter(ctx *context.Context) { // Add a custom header to the response ctx.Output.Header("X-Custom-Header", "Hello, World!") // Call the next filter or the controller handler ctx.Next() } func main() { beego.InsertFilter("/*", beego.BeforeRouter, AddHeaderFilter) beego.Run() }
In this example, we define a filter function named AddHeaderFilter
. This function takes a context.Context
parameter, which represents the current request context. Inside the filter function, we add a custom header to the response using the Header
method of the Output
object. We then call the Next
method to pass the request to the next filter or the controller handler.
To register the filter function with the Beego framework, we use the InsertFilter
function. The first parameter of InsertFilter
specifies the URL pattern for which the filter should be applied. In this example, we use "/*"
to apply the filter to all routes. The second parameter specifies the filter position. In this example, we use beego.BeforeRouter
to apply the filter before the router handles the request.
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
Introduction to Event-Driven Programming
Event-driven programming is a programming paradigm that is based on the concept of events and event handlers. In event-driven programming, the flow of the program is determined by events, which are triggered by external sources such as user input, system events, or messages from other programs.
The key idea behind event-driven programming is that the program responds to events as they occur, rather than following a predefined sequence of instructions. This allows for more flexible and responsive programs, as they can react to events in real-time.
In event-driven programming, events are typically handled by event handlers, which are functions or methods that are executed in response to a specific event. Event handlers are registered with the event source and are called when the corresponding event occurs.
Event-driven programming is commonly used in graphical user interfaces (GUIs), where user actions such as mouse clicks and keystrokes are treated as events. It is also used in network programming, where events such as incoming requests or messages are treated as events.
Implementing the Observer Pattern in Beego
The Observer pattern is a behavioral design pattern that allows an object, called the subject, to notify a list of dependents, called observers, when its state changes. It is commonly used when you want to establish a one-to-many relationship between objects, where changes in one object need to be propagated to multiple other objects.
In Beego, the Observer pattern can be implemented using event-driven programming. Beego provides a built-in event system that allows you to define custom events and register event handlers to handle those events.
To implement the Observer pattern in Beego, you need to define a custom event and register observers to handle that event. Here's an example:
// main.go package main import ( "github.com/astaxie/beego" ) type UserCreatedEvent struct { UserID int } func HandleUserCreated(event *UserCreatedEvent) { // Handle the user created event // For example, send a welcome email to the user sendWelcomeEmail(event.UserID) } func main() { beego.EventRouter.AddEventListener("user.created", HandleUserCreated) // Trigger the user created event beego.EventRouter.TriggerEvent("user.created", &UserCreatedEvent{UserID: 1}) beego.Run() }
In this example, we define a custom event struct named UserCreatedEvent
, which contains the ID of the user that was created. We also define a function named HandleUserCreated
, which takes a pointer to a UserCreatedEvent
and handles the user created event. In this example, the event handler sends a welcome email to the user.
To register the event handler with the Beego framework, we use the AddEventListener
method of the EventRouter
object. We pass the event name, "user.created"
, and the event handler function to the AddEventListener
method.
To trigger the event, we use the TriggerEvent
method of the EventRouter
object. We pass the event name, "user.created"
, and an instance of the UserCreatedEvent
struct to the TriggerEvent
method.
Additional Resources
- Go by Example