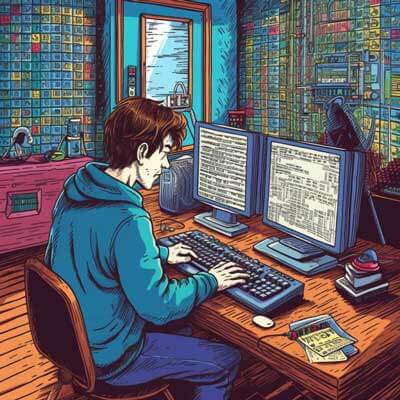
- Introduction to SFTP
- SFTP Architecture Overview
- Setting Up SFTP for Secure File Transfer
- Configuring SFTP
- Securing SFTP Connections
- SFTP Use Case: Large Scale Data Transfer
- SFTP Use Case: Secure Backup Solutions
- SFTP Best Practice: Permission and User Management
- SFTP Best Practice: Secure Key Management
- Real World Example: Using SFTP for Web Server File Management
- Real World Example: Using SFTP in a Distributed Team
- Performance Consideration: Network Bandwidth and SFTP
- Performance Consideration: Disk I/O and SFTP
- Advanced Technique: Automating File Transfers with SFTP
- Advanced Technique: Integrating SFTP with Other Systems
- Code Snippet: Establishing an SFTP Connection
- Code Snippet: Uploading Files via SFTP
- Code Snippet: Downloading Files via SFTP
- Code Snippet: Automating File Transfers with SFTP
- Code Snippet: Handling Errors in SFTP Connections
- Error Handling: Dealing with Connection Issues
- Error Handling: Addressing Authentication Problems
- Error Handling: Managing File Transfer Failures
Introduction to SFTP
SFTP (Secure File Transfer Protocol) is a secure method for transferring files between a local machine and a remote server. It provides encryption and authentication to ensure the confidentiality and integrity of data during transfer. SFTP is widely used in Linux environments for secure file management and is considered a more secure alternative to FTP.
Related Article: How To Find Files Based On Wildcard In Linux
SFTP Architecture Overview
SFTP operates on a client-server architecture. The client is responsible for initiating the connection and sending commands to the server, while the server handles the actual file transfer operations. The communication between the client and server is encrypted, preventing unauthorized access and tampering of data.
Setting Up SFTP for Secure File Transfer
To set up SFTP for secure file transfer, follow these steps:
1. Install the OpenSSH server on the remote server:
sudo apt-get install openssh-server
2. Ensure that the SSH service is running:
sudo systemctl status ssh
Configuring SFTP
To configure SFTP, follow these steps:
1. Open the SSH server configuration file:
sudo nano /etc/ssh/sshd_config
2. Uncomment or add the following line to enable SFTP:
Subsystem sftp internal-sftp
3. Configure the SFTP options by adding the following lines:
Match Group sftpusers ChrootDirectory /home/%u ForceCommand internal-sftp X11Forwarding no AllowTcpForwarding no
Related Article: How to Copy a Folder from Remote to Local Using Scp in Linux
Securing SFTP Connections
To secure SFTP connections, follow these steps:
1. Configure SSH to listen on a specific IP address by adding or modifying the following line in the SSH server configuration file:
ListenAddress <IP_Address>
2. Enable key-based authentication by setting the following options in the SSH server configuration file:
PubkeyAuthentication yes PasswordAuthentication no
SFTP Use Case: Large Scale Data Transfer
SFTP is commonly used for large-scale data transfer. For example, organizations dealing with big data often use SFTP to move large volumes of data securely between servers. Here’s an example of uploading a file via SFTP using the command line:
sftp user@remote_host # Enter password if prompted put local_file remote_directory
SFTP Use Case: Secure Backup Solutions
SFTP is also widely used for secure backup solutions. Backup software can be configured to use SFTP to transfer and store backup files securely on a remote server. Here’s an example of downloading a file via SFTP using the command line:
sftp user@remote_host # Enter password if prompted get remote_file local_directory
Related Article: How to Apply Chmod 777 to a Folder and its Contents in Linux
SFTP Best Practice: Permission and User Management
Proper permission and user management are crucial for the security of SFTP. Follow these best practices:
1. Create dedicated SFTP users with limited access rights.
2. Set appropriate file and directory permissions to prevent unauthorized access.
3. Regularly review user access and revoke unnecessary privileges.
SFTP Best Practice: Secure Key Management
Secure key management is essential for SFTP. Follow these best practices:
1. Generate strong SSH key pairs using tools like ssh-keygen
.
2. Protect private keys with strong passwords.
3. Store private keys securely and restrict access to trusted individuals.
Real World Example: Using SFTP for Web Server File Management
In a real-world scenario, SFTP can be used for web server file management. Developers can use SFTP to upload, modify, and delete files on a remote server hosting a website. Here’s an example of establishing an SFTP connection using Python:
import paramiko ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect('remote_host', username='user', password='password') sftp = ssh.open_sftp() # Perform file management operations using the `sftp` object sftp.close() ssh.close()
Related Article: Tutorial on Linux User Management: How to Create a User
Real World Example: Using SFTP in a Distributed Team
SFTP is also valuable in distributed team environments where team members need to collaborate on file sharing. SFTP allows secure file transfer between team members regardless of their physical location. Here’s an example of uploading a file via SFTP using a programming language such as Java:
import com.jcraft.jsch.*; JSch jsch = new JSch(); Session session = jsch.getSession("user", "remote_host", 22); session.setPassword("password"); session.setConfig("StrictHostKeyChecking", "no"); session.connect(); ChannelSftp channelSftp = (ChannelSftp) session.openChannel("sftp"); channelSftp.connect(); // Perform file upload operations using the `channelSftp` object channelSftp.disconnect(); session.disconnect();
Performance Consideration: Network Bandwidth and SFTP
When using SFTP, network bandwidth plays a significant role in determining the transfer speed. The available bandwidth affects the time taken to transfer files between the client and server. To optimize performance, consider the following:
1. Use a high-speed and reliable network connection.
2. Prioritize SFTP traffic to avoid congestion.
3. Consider compressing files before transferring them to reduce the data size.
Performance Consideration: Disk I/O and SFTP
Disk I/O performance also impacts SFTP performance. Disk read and write speeds affect the transfer speed, especially when dealing with large files. To optimize performance, consider the following:
1. Use high-performance storage drives.
2. Optimize disk I/O by reducing unnecessary disk access.
3. Allocate sufficient disk space for file transfers.
Related Article: Tutorial: Using Unzip Command in Linux
Advanced Technique: Automating File Transfers with SFTP
Automating file transfers with SFTP can streamline repetitive tasks. By scripting SFTP commands or using programming languages, you can automate file transfers. Here’s an example of automating file uploads using a shell script:
#!/bin/bash HOST="remote_host" USER="user" PASS="password" FILE="local_file" REMOTE_DIR="remote_directory" sftp -oBatchMode=no -b - $USER@$HOST <<EOF put $FILE $REMOTE_DIR exit EOF
Advanced Technique: Integrating SFTP with Other Systems
SFTP can be integrated with other systems, such as Continuous Integration/Continuous Deployment (CI/CD) pipelines or data processing workflows. This integration allows for seamless file transfer between different components. Here’s an example of using SFTP in a Python script to integrate with a CI/CD pipeline:
import paramiko ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect('remote_host', username='user', password='password') sftp = ssh.open_sftp() # Perform file transfer operations within the CI/CD pipeline sftp.close() ssh.close()
Code Snippet: Establishing an SFTP Connection
Here’s a code snippet using the ssh2-sftp-client
library in Node.js to establish an SFTP connection:
const { Client } = require('ssh2-sftp-client'); const sftp = new Client(); sftp.connect({ host: 'remote_host', port: '22', username: 'user', password: 'password' }).then(() => { console.log('Connected to SFTP server'); }).catch((err) => { console.error('Error connecting to SFTP server:', err); });
Related Article: Using Linux Commands to Find File and Directory Sizes
Code Snippet: Uploading Files via SFTP
Here’s a code snippet using the ssh2-sftp-client
library in Node.js to upload files via SFTP:
const { Client } = require('ssh2-sftp-client'); const sftp = new Client(); sftp.connect({ host: 'remote_host', port: '22', username: 'user', password: 'password' }).then(() => { return sftp.put('local_file', 'remote_directory/remote_file'); }).then(() => { console.log('File uploaded successfully'); }).catch((err) => { console.error('Error uploading file:', err); }).finally(() => { sftp.end(); });
Code Snippet: Downloading Files via SFTP
Here’s a code snippet using the ssh2-sftp-client
library in Node.js to download files via SFTP:
const { Client } = require('ssh2-sftp-client'); const sftp = new Client(); sftp.connect({ host: 'remote_host', port: '22', username: 'user', password: 'password' }).then(() => { return sftp.get('remote_directory/remote_file', 'local_directory/local_file'); }).then(() => { console.log('File downloaded successfully'); }).catch((err) => { console.error('Error downloading file:', err); }).finally(() => { sftp.end(); });
Code Snippet: Automating File Transfers with SFTP
Here’s a code snippet using the pysftp
library in Python to automate file transfers with SFTP:
import pysftp cnopts = pysftp.CnOpts() cnopts.hostkeys = None with pysftp.Connection('remote_host', username='user', password='password', cnopts=cnopts) as sftp: sftp.put('local_file', 'remote_directory/remote_file') print('File uploaded successfully')
Related Article: How to Sync Local and Remote Directories with Rsync
Code Snippet: Handling Errors in SFTP Connections
Here’s a code snippet using the ssh2-sftp-client
library in Node.js to handle errors in SFTP connections:
const { Client } = require('ssh2-sftp-client'); const sftp = new Client(); sftp.connect({ host: 'remote_host', port: '22', username: 'user', password: 'password' }).then(() => { // Perform SFTP operations }).catch((err) => { console.error('Error connecting to SFTP server:', err); }).finally(() => { sftp.end(); });
Error Handling: Dealing with Connection Issues
When encountering connection issues with SFTP, check the following:
1. Verify the remote server’s SSH service is running.
2. Ensure the correct credentials (username and password) are provided.
3. Check firewall settings to allow SSH/SFTP connections.
Error Handling: Addressing Authentication Problems
If you encounter authentication problems with SFTP, consider the following:
1. Ensure the correct authentication method is used (e.g., password, key-based).
2. Verify the provided credentials (username, password or private key) are correct.
3. Check the server’s SSH configuration to ensure the chosen authentication method is enabled.
Related Article: How to Alter the Echo Output Colors in Linux
Error Handling: Managing File Transfer Failures
If file transfer failures occur during SFTP operations, consider the following:
1. Check file and directory permissions on the remote server.
2. Ensure the remote server has sufficient disk space for the file being transferred.
3. Monitor network connectivity and ensure stable network connections during file transfers.