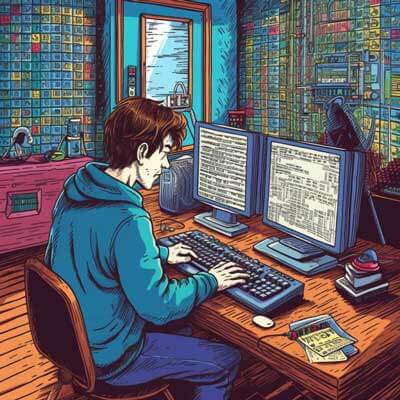
Extracting substrings is a common task in shell scripting, and Bash provides several built-in methods to accomplish this. In this guide, we will explore different techniques to extract substrings in Bash.
Method 1: Using Parameter Expansion
Bash provides parameter expansion, which allows us to manipulate variables and extract substrings. Here is a simple example of extracting a substring from a variable:
string="Hello, World!" substring=${string:7:5} echo $substring
In this example, we have a variable called string
that contains the string “Hello, World!”. We use the ${string:start:length}
syntax to extract a substring starting from the 7th character with a length of 5 characters. The result is “World”.
This method is simple and efficient for extracting substrings within a variable. However, it is important to note that the index starts from 0, so the first character is at index 0.
Related Article: How To Echo a Newline In Bash
Method 2: Using Cut
Another way to extract substrings in Bash is by using the cut
command. The cut
command is designed to extract sections from lines of files or strings. Here is an example:
string="Hello, World!" substring=$(echo $string | cut -c 8-12) echo $substring
In this example, we use the cut
command with the -c
option to specify the range of characters to extract. The range 8-12
extracts the characters from the 8th to the 12th position, resulting in the substring “World!”.
The advantage of using the cut
command is that it provides more flexibility in extracting substrings based on delimiters or specific fields. For example, you can extract a substring based on a delimiter like a space or a comma.
Method 3: Using awk
Awk is a useful text processing tool that can also be used to extract substrings in Bash. Here is an example:
string="Hello, World!" substring=$(echo $string | awk '{print substr($0, 8, 5)}') echo $substring
In this example, we use the substr
function of awk to extract a substring. The first parameter is the input string, the second parameter is the starting position, and the third parameter is the length of the substring. The result is the same as the previous examples, “World!”.
Awk provides more advanced capabilities for text processing, such as pattern matching and field extraction. It is particularly useful when dealing with complex text files or structured data.
Best Practices
When extracting substrings in Bash, it is important to keep a few best practices in mind:
1. Use parameter expansion for simple substring extraction within variables. It is fast and straightforward.
2. Use the cut
command when you need to extract substrings based on delimiters or specific fields.
3. Use awk when you need advanced text processing capabilities, such as pattern matching or complex data manipulation.
Related Article: How to Use If-Else Statements in Shell Scripts