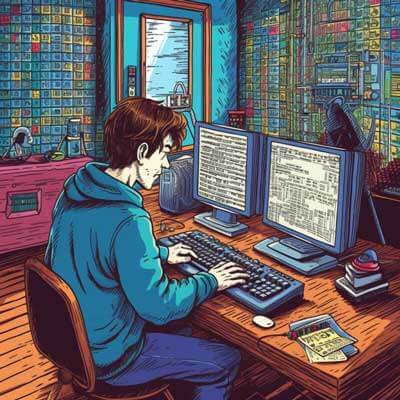
Table of Contents
Routing in React
Routing is a crucial aspect of web development that allows users to navigate through different pages or views within a web application. In React, we can achieve routing using various libraries, with React Router being one of the most popular choices.
React Router is a useful routing library that allows us to define routes in our React applications. It provides a declarative way to handle navigation and rendering of components based on the current URL. With React Router, we can create single-page applications (SPAs) that dynamically update the UI without the need for a full page reload.
To use React Router in our React applications, we first need to install it. We can do this by running the following command:
npm install react-router-dom
Once installed, we can import the necessary components from react-router-dom
and start defining our routes. Here's an example of how we can define routes using React Router:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; const App = () => { return ( ); }; const Home = () => <h1>Welcome to the Home page!</h1>; const About = () => <h1>About Us</h1>; const Contact = () => <h1>Contact Us</h1>; const NotFound = () => <h1>404 Not Found</h1>;
In this example, we use the BrowserRouter
component as the root component, which enables client-side routing. Inside the Switch
component, we define our individual routes using the Route
component. The exact
prop in the first Route
ensures that only the specified path is matched exactly.
Now, when a user navigates to the root URL ("/"), the Home
component will be rendered. Similarly, navigating to "/about" will render the About
component, "/contact" will render the Contact
component, and any other URL will render the NotFound
component.
React Router provides many other features and components for handling routing, such as nested routes, route parameters, and programmatic navigation. We'll explore some of these features in the following sections.
Related Article: How to Get the Current URL with JavaScript
Client-side routing with React Router
Client-side routing allows us to handle navigation within a web application without performing a full page reload. This provides a smooth and seamless user experience, as the UI updates dynamically without any interruptions.
React Router excels at client-side routing, as it leverages the power of React's component-based architecture. By defining routes and associating them with specific components, we can easily navigate between different views in our application.
Let's take a closer look at how client-side routing works with React Router. In the previous example, we defined our routes using the Route
component. Each Route
component takes a path
prop, which specifies the URL path that should trigger the rendering of the associated component.
When a user navigates to a URL that matches one of the defined routes, React Router renders the corresponding component and updates the UI accordingly. This process is entirely handled on the client-side, without making a request to the server.
For example, if a user navigates to "/about", React Router will render the About
component and update the UI to display the relevant content. This is done without reloading the entire page, resulting in a faster and more responsive user experience.
React Router also provides a Link
component that we can use to create links between different pages or views within our application. The Link
component ensures that navigation occurs without a full page reload, allowing users to seamlessly move between different parts of the application.
Here's an example of how we can use the Link
component in conjunction with React Router:
import { Link } from 'react-router-dom'; const Navigation = () => { return ( <nav> <ul> <li> Home </li> <li> About </li> <li> Contact </li> </ul> </nav> ); };
In this example, we create a navigation bar with links to the home, about, and contact pages. When a user clicks on one of these links, React Router handles the navigation and renders the associated component without a full page reload.
Client-side routing provides a seamless user experience and is essential for building modern web applications. React Router makes it easy to implement client-side routing in React applications, allowing us to create dynamic and interactive UIs.
Server-side rendering in React
Server-side rendering (SSR) is a technique that allows us to render React components on the server and send the pre-rendered HTML to the client. This approach offers several benefits, such as improved performance, better SEO, and enhanced user experience.
In traditional client-side rendering, the browser downloads the JavaScript bundle, which then executes on the client-side to render the UI. This can lead to slower initial page load times, especially for larger applications. Additionally, search engines may have difficulty crawling and indexing the content of a client-rendered application.
With server-side rendering, we can generate the HTML on the server and send it to the client, resulting in a faster initial page load. The server can also provide the pre-rendered HTML to search engines, improving the discoverability of our application's content.
To enable server-side rendering in React, we can use libraries like Next.js. Next.js is a popular framework built on top of React that provides built-in server-side rendering capabilities, among other features.
Server-side rendering in Next.js
Next.js is a useful framework that combines the benefits of React with server-side rendering and other optimizations. It simplifies the process of building React applications with server-side rendering by providing a set of conventions and utilities.
When using Next.js, we can create pages as individual components and export them from a specific directory. Next.js automatically generates the necessary server-side code to render these components on the server and send the pre-rendered HTML to the client.
Here's an example of a basic Next.js page component:
const Home = () => { return <h1>Welcome to the Home page!</h1>; }; export default Home;
In this example, we define a simple Home
component that renders a heading element. We then export this component as the default export, indicating that it represents a page in our Next.js application.
Next.js automatically maps the file name to the corresponding URL path. For example, a file named about.js
would be accessible at the "/about" URL path. This convention-based routing makes it easy to create and organize our pages.
Next.js also supports dynamic routing, allowing us to define routes with parameters. We can use the file system to define dynamic routes and access the route parameters in our page components.
For example, suppose we have a file named [id].js
in our pages directory. This file represents a dynamic route with a parameter called "id". We can access this parameter in our page component as a prop:
const Post = ({ id }) => { return <h1>Post ID: {id}</h1>; }; export default Post;
In this example, if we navigate to "/posts/123", Next.js will render the Post
component and pass the "123" value as the "id" prop. This allows us to create dynamic and personalized pages based on the URL parameters.
Next.js handles the server-side rendering of these components without the need for additional configuration. It automatically generates the necessary server-side code, ensuring that the page is rendered on the server and sent to the client as pre-rendered HTML.
Related Article: How to Autofill a Textarea Element with VueJS
Dynamic routing with React Router
Dynamic routing allows us to handle URL parameters and create routes that can match a variety of different paths. React Router provides useful features for implementing dynamic routing in our applications.
To define dynamic routes with React Router, we can use the :param
syntax in the path
prop of the Route
component. This allows us to specify a parameter in the URL path that can be accessed in the rendered component.
Here's an example of how we can define dynamic routes with React Router:
In this example, the :id
parameter in the path represents a dynamic value that can match any string. When a URL matches this route, React Router renders the Post
component and provides the URL parameter as a prop.
We can access the URL parameter in the rendered component by using the useParams
hook provided by React Router. Here's an example of how we can access the URL parameter in the Post
component:
import { useParams } from 'react-router-dom'; const Post = () => { const { id } = useParams(); return <h1>Post ID: {id}</h1>; };
In this example, the useParams
hook returns an object containing all the URL parameters defined in the route. We can destructure the id
parameter from this object and use it in our component to render dynamic content based on the URL parameter.
Dynamic routing is a useful feature that allows us to create flexible and personalized routes in our applications. React Router simplifies the implementation of dynamic routing by providing intuitive syntax and hooks for accessing URL parameters.
Dynamic routing in Next.js
Next.js provides built-in support for dynamic routing, allowing us to create dynamic pages with URL parameters. We can define dynamic routes using the file system, and Next.js automatically generates the necessary server-side code for rendering these pages.
To define a dynamic route in Next.js, we can use the file system to create a file with square brackets in its name. For example, a file named [id].js
represents a dynamic route with an "id" parameter.
Here's an example of a dynamic route in Next.js:
const Post = ({ id }) => { return <h1>Post ID: {id}</h1>; }; export default Post;
In this example, the Post
component represents a dynamic route with an "id" parameter. Next.js automatically maps the file name to the corresponding URL path and passes the "id" parameter as a prop to the component.
Next.js also provides a useRouter
hook that allows us to access the current route and its parameters. We can use this hook to access the "id" parameter in our dynamic route component:
import { useRouter } from 'next/router'; const Post = () => { const router = useRouter(); const { id } = router.query; return <h1>Post ID: {id}</h1>; };
In this example, we use the useRouter
hook to access the current route and its parameters. The query
property of the router object contains an object with the URL parameters. We destructure the "id" parameter from this object and use it in our component to render dynamic content.
Next.js handles the server-side rendering of dynamic routes automatically, without the need for additional configuration. It generates the necessary server-side code to render these routes on the server and sends the pre-rendered HTML to the client.
Dynamic routing in Next.js allows us to create personalized and dynamic pages based on URL parameters. Next.js simplifies the implementation of dynamic routing by providing a convention-based approach and hooks for accessing URL parameters.
Nested routing with React Router
Nested routing is a technique that allows us to define routes within other routes, creating a hierarchy of routes. React Router provides support for nested routing, allowing us to create complex navigation structures in our applications.
To implement nested routing with React Router, we can nest Route
components inside other Route
components. This allows us to define child routes that are rendered within their parent routes.
Here's an example of how we can define nested routes with React Router:
In this example, the Products
component represents a parent route, and the Product
component represents a child route. When the URL matches "/products", React Router renders the Products
component and looks for nested routes inside it.
The Product
component is a child route of the Products
component and is rendered when the URL matches "/products/:id". React Router provides the URL parameter as a prop to the Product
component, allowing us to render dynamic content based on the parameter.
Nested routes can be used to create complex navigation structures in our applications. They allow us to define routes within routes, providing a modular and organized approach to handling different views and components.
React Router makes it easy to implement nested routing with its intuitive API and declarative syntax. By nesting Route
components, we can create hierarchical routes that match the structure of our application.
Integrating React Router with Next.js
Next.js is a useful framework that provides built-in routing capabilities. By default, Next.js uses file-based routing, where each file in the "pages" directory represents a unique route. However, if you prefer to use React Router for routing in Next.js, you can integrate them together.
To integrate React Router with Next.js, we need to install the necessary dependencies. In addition to React Router, we also need to install the next-routes
library, which provides compatibility between Next.js and React Router.
npm install react-router-dom next-routes
Once the dependencies are installed, we can start integrating React Router with Next.js. First, we need to create a custom App
component that wraps the Next.js App
component and includes the React Router components.
Here's an example of how we can create a custom App
component with React Router integration:
import React from 'react'; import App from 'next/app'; import { Router } from 'next-routes'; import { BrowserRouter } from 'react-router-dom'; class MyApp extends App { render() { const { Component, pageProps } = this.props; return ( ); } } export default MyApp;
In this example, we import the necessary components from React Router and Next.js. We then create a custom MyApp
component that extends the Next.js App
component.
Inside the MyApp
component, we wrap the Component
with the BrowserRouter
component from React Router. This enables client-side routing within our Next.js application.
Finally, we export the MyApp
component as the default export, indicating that it should be used as the root component for our Next.js application.
With React Router integrated into our Next.js application, we can now define routes using React Router's declarative syntax. We can use the Route
component to define routes and the Link
component to create links between different pages or views.
Here's an example of how we can define routes and create links with React Router in Next.js:
import { Route, Link } from 'react-router-dom'; const Home = () => <h1>Welcome to the Home page!</h1>; const About = () => <h1>About Us</h1>; const Contact = () => <h1>Contact Us</h1>; const NotFound = () => <h1>404 Not Found</h1>; const Navigation = () => { return ( <nav> <ul> <li> Home </li> <li> About </li> <li> Contact </li> </ul> </nav> ); }; const App = () => { return ( <div> </div> ); }; export default App;
In this example, we define the Home
, About
, Contact
, and NotFound
components, each representing a different page or view in our Next.js application.
We create a Navigation
component that includes Link
components to navigate between different pages. These links are handled by React Router and provide client-side routing without a full page reload.
Finally, we render the Navigation
component and define the routes using the Route
component from React Router. The exact
prop ensures that only the specified path is matched exactly, preventing multiple routes from being rendered simultaneously.
Related Article: How to Navigate to a URL in Javascript
Implementing routing in React
Implementing routing in React can be done using various libraries and frameworks. One of the most popular choices is React Router, a flexible and useful routing library for React applications.
To implement routing in React using React Router, we first need to install the necessary dependencies. We can do this by running the following command:
npm install react-router-dom
Once installed, we can import the necessary components from react-router-dom
and start defining our routes. Here's an example of how we can set up routing in a React application using React Router:
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'; const App = () => { return ( ); }; const Home = () => <h1>Welcome to the Home page!</h1>; const About = () => <h1>About Us</h1>; const Contact = () => <h1>Contact Us</h1>; const NotFound = () => <h1>404 Not Found</h1>;
In this example, we import the necessary components from react-router-dom
, including BrowserRouter
, Route
, Switch
, and the individual page components.
We then wrap our application with the BrowserRouter
component, which enables client-side routing. Inside the Switch
component, we define our individual routes using the Route
component.
The exact
prop in the first Route
ensures that only the specified path is matched exactly. If a user navigates to the root URL ("/"), the Home
component will be rendered. Similarly, navigating to "/about" will render the About
component, "/contact" will render the Contact
component, and any other URL will render the NotFound
component.
This example demonstrates a simple implementation of routing in a React application using React Router. By defining routes and associating them with specific components, we can enable navigation and rendering of different views based on the current URL.
Exploring Single Page Applications (SPAs)
Single Page Applications (SPAs) are web applications that load once and dynamically update the content without requiring a full page reload. SPAs provide a more fluid and responsive user experience by avoiding interruptions caused by page transitions.
In a traditional multi-page application, each page is a separate HTML document loaded from the server. When a user navigates to a different page, the browser fetches and renders a new HTML document, resulting in a visible delay and a loss of the application's state.
In contrast, SPAs load a single HTML document that serves as the container for the entire application. The content is dynamically updated using JavaScript and AJAX techniques, allowing users to navigate between different views without the need for a full page reload.
SPAs typically use client-side routing to handle navigation within the application. When a user clicks on a link or performs an action that triggers a route change, the SPA updates the URL and renders the corresponding view without making a request to the server.
React, along with libraries like React Router, is well-suited for building SPAs. React's component-based architecture and virtual DOM make it easy to manage complex UIs, while React Router provides a declarative approach to handling client-side routing.
SPAs offer several advantages over traditional multi-page applications. They provide a smoother and more responsive user experience by eliminating the delays caused by page transitions. SPAs also enable more dynamic and interactive UIs, as they can update specific parts of the page without refreshing the entire document.
However, SPAs also have some drawbacks. They require more initial JavaScript to be downloaded and executed, which can affect the initial page load time, especially for larger applications. SPAs also rely heavily on JavaScript, so users with JavaScript disabled may not be able to fully access the application's content.
Despite these drawbacks, SPAs have become increasingly popular due to their ability to provide a seamless and interactive user experience. React and React Router are useful tools for building SPAs, as they offer the necessary components and libraries for implementing client-side routing and managing complex UIs.
Understanding Server-side Rendering (SSR)
Server-side rendering (SSR) is a technique that allows us to render web pages on the server and send the pre-rendered HTML to the client. SSR offers several benefits, such as improved performance, better search engine optimization (SEO), and enhanced user experience.
In a traditional client-side rendering (CSR) approach, the browser downloads a JavaScript bundle that executes on the client-side to render the UI. This approach can result in slower initial page load times, especially for larger applications. Additionally, search engines may have difficulty crawling and indexing the content of a CSR application.
With SSR, we can generate the HTML on the server and send it to the client, resulting in a faster initial page load. The server can also provide the pre-rendered HTML to search engines, improving the discoverability of our application's content.
To implement SSR in a JavaScript application, we need to use a server-side rendering framework or library. React, along with frameworks like Next.js, provides built-in support for SSR, making it easier to implement this technique in our projects.
SSR also improves SEO by providing search engines with pre-rendered HTML that includes the content of our application. This allows search engines to crawl and index the content more effectively, increasing the chances of our application appearing in relevant search results.
Another advantage of SSR is that it provides a better user experience for users with slower internet connections or older devices. By pre-rendering the HTML on the server, we can ensure that the content is available immediately, regardless of the client's capabilities.
However, SSR also has some trade-offs. It requires additional server-side processing and can increase the server load, especially for complex applications. SSR may also introduce more complexity into the development process, as we need to consider both server and client rendering.
Despite these trade-offs, SSR is a useful technique for improving the performance and user experience of our web applications. React and frameworks like Next.js make it easier to implement SSR, allowing us to leverage the benefits of both server-side and client-side rendering.
Next.js and server-side rendering
Next.js is a useful framework built on top of React that provides built-in support for server-side rendering (SSR). It simplifies the process of building React applications with SSR by providing a set of conventions and utilities.
Next.js uses a file-based routing system, where each file in the "pages" directory represents a unique route. This convention allows us to define page components as individual files and export them from the "pages" directory.
Here's an example of a basic Next.js page component:
const Home = () => { return <h1>Welcome to the Home page!</h1>; }; export default Home;
In this example, we define a simple Home
component that renders a heading element. We then export this component as the default export, indicating that it represents a page in our Next.js application.
Next.js automatically generates the necessary server-side code to render these page components on the server and send the pre-rendered HTML to the client. This allows us to benefit from the performance and SEO advantages of SSR without the need for manual configuration.
Next.js also provides features like code splitting and automatic static optimization, further enhancing the performance of our applications. Code splitting allows us to split our JavaScript bundles into smaller chunks, reducing the initial load time. Automatic static optimization optimizes the rendering of static pages, making them faster and more efficient.
In addition to SSR, Next.js also supports client-side rendering (CSR) for dynamic and interactive components. It seamlessly combines SSR and CSR, allowing us to choose the appropriate rendering strategy for each part of our application.
Next.js simplifies the implementation of SSR by providing a convention-based approach and built-in utilities. By leveraging Next.js, we can easily build React applications with server-side rendering, improving performance, SEO, and user experience.
Related Article: How to Compare Arrays in Javascript
Using React Router with Next.js
React Router is a useful routing library for React applications that allows us to handle navigation and rendering of components based on the current URL. While Next.js provides built-in routing capabilities, we can still use React Router with Next.js to benefit from its advanced features and flexibility.
To use React Router with Next.js, we first need to install the necessary dependencies. In addition to React Router, we also need to install the next-routes
library, which provides compatibility between Next.js and React Router.
npm install react-router-dom next-routes
Once the dependencies are installed, we can start integrating React Router with Next.js. First, we need to create a custom App
component that wraps the Next.js App
component and includes the React Router components.
Here's an example of how we can create a custom App
component with React Router integration:
import React from 'react'; import App from 'next/app'; import { Router } from 'next-routes'; import { BrowserRouter } from 'react-router-dom'; class MyApp extends App { render() { const { Component, pageProps } = this.props; return ( ); } } export default MyApp;
In this example, we import the necessary components from React Router and Next.js. We then create a custom MyApp
component that extends the Next.js App
component.
Inside the MyApp
component, we wrap the Component
with the BrowserRouter
component from React Router. This enables client-side routing within our Next.js application.
Finally, we export the MyApp
component as the default export, indicating that it should be used as the root component for our Next.js application.
With React Router integrated into our Next.js application, we can now define routes using React Router's declarative syntax. We can use the Route
component to define routes and the Link
component to create links between different pages or views.
Here's an example of how we can define routes and create links with React Router in Next.js:
import { Route, Link } from 'react-router-dom'; const Home = () => <h1>Welcome to the Home page!</h1>; const About = () => <h1>About Us</h1>; const Contact = () => <h1>Contact Us</h1>; const NotFound = () => <h1>404 Not Found</h1>; const Navigation = () => { return ( <nav> <ul> <li> Home </li> <li> About </li> <li> Contact </li> </ul> </nav> ); }; const App = () => { return ( <div> </div> ); }; export default App;
In this example, we define the Home
, About
, Contact
, and NotFound
components, each representing a different page or view in our Next.js application.
We create a Navigation
component that includes Link
components to navigate between different pages. These links are handled by React Router and provide client-side routing without a full page reload.
Finally, we render the Navigation
component and define the routes using the Route
component from React Router. The exact
prop ensures that only the specified path is matched exactly, preventing multiple routes from being rendered simultaneously.
Client-side routing in Next.js
Next.js, by default, uses server-side rendering (SSR) to handle routing and generate pre-rendered HTML for each route. However, Next.js also provides client-side routing capabilities for certain cases where traditional SSR is not necessary.
To enable client-side routing in Next.js, we can use the Link
component provided by the next/link
module. This component allows us to create links between different pages or views in our Next.js application.
Here's an example of how we can use the Link
component for client-side routing in Next.js:
import Link from 'next/link'; const Navigation = () => { return ( <nav> <ul> <li> Home </li> <li> About </li> <li> Contact </li> </ul> </nav> ); };
In this example, we import the Link
component from the next/link
module. We use this component to create links between different pages or views in our Next.js application.
The href
prop of the Link
component specifies the target URL or route. When a user clicks on a link created by the Link
component, Next.js handles the navigation and updates the UI without a full page reload.
Client-side routing in Next.js provides a smoother and more responsive user experience compared to traditional server-side rendering. It allows for faster navigation between views and avoids the delays caused by full page reloads.
It's important to note that not all links in a Next.js application need to use client-side routing. Next.js automatically handles navigation for links between pages within the same Next.js application. Only external links or links to a different Next.js application require explicit client-side routing using the Link
component.
Dynamic routing in Next.js
Next.js provides built-in support for dynamic routing, allowing us to create pages with dynamic URLs and access the URL parameters in our components. This allows us to create personalized and dynamic pages based on the URL parameters.
To define dynamic routes in Next.js, we use the file system to create a file with square brackets in its name. For example, a file named [id].js
represents a dynamic route with an "id" parameter.
Here's an example of a dynamic route in Next.js:
const Post = ({ id }) => { return <h1>Post ID: {id}</h1>; }; export default Post;
In this example, the Post
component represents a dynamic route with an "id" parameter. Next.js automatically maps the file name to the corresponding URL path and passes the "id" parameter as a prop to the component.
We can access the URL parameter in the rendered component by using the useRouter
hook provided by Next.js. Here's an example of how we can access the URL parameter in the Post
component:
import { useRouter } from 'next/router'; const Post = () => { const router = useRouter(); const { id } = router.query; return <h1>Post ID: {id}</h1>; };
In this example, we use the useRouter
hook to access the current route and its parameters. The query
property of the router object contains an object with the URL parameters. We destructure the "id" parameter from this object and use it in our component to render dynamic content.
Dynamic routing in Next.js allows us to create personalized and dynamic pages based on URL parameters. Next.js simplifies the implementation of dynamic routing by providing a convention-based approach and hooks for accessing URL parameters.
Additional Resources
- Next.js - The React Framework