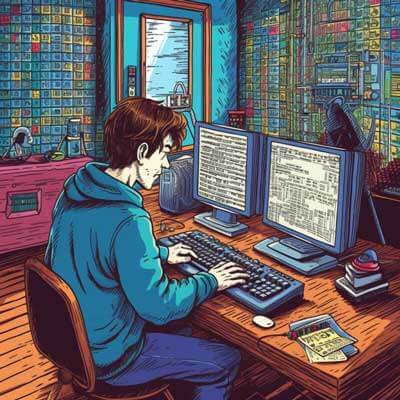
Table of Contents
Navigating to a URL in JavaScript is a common requirement when working with web applications. There are several ways to achieve this, depending on your specific needs and the context in which you are working. In this answer, we will explore two different approaches to navigate to a URL in JavaScript.
Approach 1: Using the window.location object
One straightforward way to navigate to a URL in JavaScript is by using the window.location
object. This object provides properties and methods related to the current URL of the page and allows us to modify it to navigate to a new URL.
To navigate to a new URL using the window.location
object, you can assign a new URL to its href
property. Here is an example:
window.location.href = 'https://example.com';
Related Article: How To Round To 2 Decimal Places In Javascript
Approach 2: Using the window.open() method
Another approach to navigate to a URL in JavaScript is by using the window.open()
method. This method opens a new browser window or tab with the specified URL. Here is an example:
window.open('https://example.com', '_blank');
In this example, the first argument to window.open()
is the URL to navigate to, and the second argument (optional) specifies the target window or tab in which the URL should be opened. The _blank
value opens the URL in a new tab or window.
Using window.open()
provides more flexibility compared to window.location.href
as it allows you to control how the navigation is handled, such as opening in a new tab or window.
Best Practices
When navigating to a URL in JavaScript, it is important to consider some best practices to ensure a smooth user experience:
1. Provide clear feedback: When triggering a navigation, consider displaying loading indicators or messages to inform the user that the navigation is in progress. This helps prevent confusion or frustration if the navigation takes longer than expected.
2. Handle navigation errors: Navigating to a URL can sometimes result in errors, such as a network failure or an invalid URL. Handle these errors gracefully by displaying appropriate error messages or fallback options to the user.
3. Avoid excessive use of pop-ups: While window.open()
can be useful in some scenarios, excessive use of pop-ups can lead to a poor user experience and may be blocked by browsers' popup blockers. Consider alternative approaches such as opening links in new tabs using anchor tags (<a target="_blank" href="https://example.com" rel="noopener">
) or using AJAX to load content dynamically.
Alternative Ideas
1. Using the location.href
property: In addition to window.location.href
, you can also navigate to a new URL by directly modifying the location.href
property. However, it is recommended to use window.location.href
for better browser compatibility.
2. Using the location.replace()
method: The location.replace()
method is similar to window.location.href
, but it replaces the current page in the browser history instead of adding a new entry. This can be useful when you want to perform a redirect without allowing the user to navigate back to the previous page.