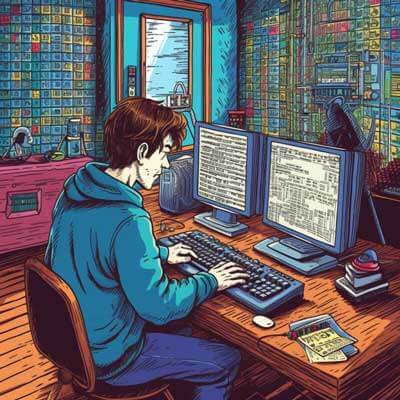
Table of Contents
Overview of Exponentiation
Exponentiation is the mathematical operation of raising a number to a power. Exponentiation can be performed using the **
operator or the built-in pow()
function. This article will focus on the practical aspects of squaring, which is raising a number to the power of 2.
Related Article: How To Access Index In Python For Loops
Using the Power Function for Squaring
The pow()
function in Python can be used to calculate squares. It takes two arguments: the base number and the exponent. When the exponent is 2, the function calculates the square of the base number. Here's an example:
result = pow(3, 2) print(result) # Output: 9
In this example, pow(3, 2)
returns 9, which is the square of 3.
The Math Module for Squaring
Python's math
module provides a pow()
function that can also be used for squaring. This function is similar to the built-in pow()
function, but it returns a floating-point number instead of an integer. Here's an example:
import math result = math.pow(3, 2) print(result) # Output: 9.0
In this example, math.pow(3, 2)
returns 9.0, which is the square of 3 as a floating-point number.
Square Root Calculation
In addition to squaring, Python also provides a way to calculate square roots using the math
module. The math.sqrt()
function takes a single argument, the number for which you want to calculate the square root. Here's an example:
import math result = math.sqrt(16) print(result) # Output: 4.0
In this example, math.sqrt(16)
returns 4.0, which is the square root of 16.
Related Article: How to Use the to_timestamp Function in Python and Pandas
Numeric Calculation for Squaring
In addition to using the pow()
and math.pow()
functions, Python provides a shorthand way to calculate squares using the **
operator. This operator raises a number to a power. Here's an example:
result = 3 ** 2 print(result) # Output: 9
In this example, 3 ** 2
returns 9, which is the square of 3.
Techniques for Squaring
When squaring large numbers, it's important to consider efficiency. One technique to improve performance is to use the **
operator instead of the pow()
function. The **
operator is faster because it's a built-in operator, whereas the pow()
function involves a function call. Here's an example:
result = 3 ** 2 print(result) # Output: 9
In this example, using the **
operator for squaring is more efficient than using the pow()
function.
Differences Between ** Operator and pow() Function for Squaring
While both the **
operator and the pow()
function can be used for squaring, there are some differences to consider.
One difference is that the **
operator returns an integer result when both the base and exponent are integers, while the pow()
function returns a floating-point result. Here's an example:
result1 = 3 ** 2 result2 = pow(3, 2) print(result1) # Output: 9 print(result2) # Output: 9.0
In this example, result1
is an integer, while result2
is a floating-point number.
Another difference is that the **
operator does not support complex numbers, while the pow()
function does. If you need to square a complex number, you should use the pow()
function. Here's an example:
result = pow(3+4j, 2) print(result) # Output: (-7+24j)
In this example, pow(3+4j, 2)
returns (-7+24j), which is the square of the complex number 3+4j.
Handling Complex Numbers for Squaring
Python provides support for complex numbers, which can be squared using the **
operator or the pow()
function. Complex numbers are numbers with both a real and imaginary part, represented as a+bj
, where a
is the real part and b
is the imaginary part.
Here's an example of squaring a complex number using the **
operator:
result = (3+4j) ** 2 print(result) # Output: (-7+24j)
In this example, (3+4j) ** 2
returns (-7+24j), which is the square of the complex number 3+4j.
And here's an example of squaring a complex number using the pow()
function:
result = pow(3+4j, 2) print(result) # Output: (-7+24j)
In this example, pow(3+4j, 2)
also returns (-7+24j), which is the square of the complex number 3+4j.