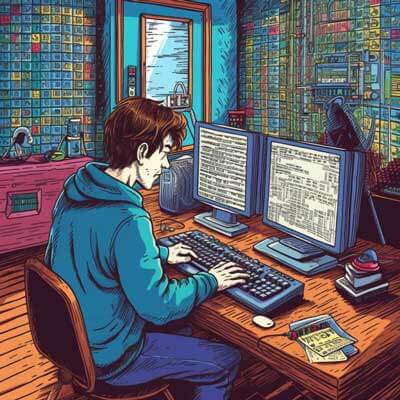
- How to handle a 422 Unprocessable Entity error in FastAPI?
- How to fix a 422 error in FastAPI?
- What are the common causes of a 422 error in FastAPI?
- How to validate data in FastAPI?
- What is the request payload in FastAPI?
- How does FastAPI handle data validation errors?
- What are some common HTTP status codes in FastAPI?
- What is the recommended data format for FastAPI endpoints?
- Additional Resources
How to handle a 422 Unprocessable Entity error in FastAPI?
When working with FastAPI, you may encounter a 422 Unprocessable Entity error when the server cannot process the request due to invalid or incorrect data. This error occurs when the server is unable to parse the request payload or when the data in the payload fails to pass the validation rules defined for the endpoint.
To handle a 422 Unprocessable Entity error in FastAPI, you can use the built-in exception handling mechanism provided by FastAPI. FastAPI automatically generates detailed error messages with appropriate HTTP status codes and response structures for validation errors.
When a request fails validation, FastAPI raises a 422 Unprocessable Entity
exception with the validation error details. This exception is handled by FastAPI and converted into a JSON response with the appropriate error message and status code.
Here’s an example of how to handle a 422 Unprocessable Entity error in FastAPI:
from fastapi import FastAPI, HTTPException from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str price: float @app.post("/items/") async def create_item(item: Item): # Process the item if not item.name: raise HTTPException(status_code=422, detail="Item name is required") if item.price <= 0: raise HTTPException(status_code=422, detail="Item price must be positive") return {"message": "Item created successfully"}
In the above example, the create_item
endpoint expects a request payload of type Item
, which is a Pydantic model. The Item
model has two fields, name
and price
, both of which are required.
If the request payload is missing the name
field or if the price
field is less than or equal to 0, FastAPI will raise a 422 Unprocessable Entity
exception with the appropriate error message. This exception is then automatically converted into a JSON response with the error details and a status code of 422.
Related Article: Optimizing FastAPI Applications: Modular Design, Logging, and Testing
How to fix a 422 error in FastAPI?
To fix a ‘422 Unprocessable Entity’ error in FastAPI, you need to identify the cause of the error and correct it. Here are some steps you can follow to fix a 422 error:
1. Check the request payload: Make sure that the request payload contains all the required fields and that the values are valid. If any required fields are missing or have invalid values, update the payload accordingly.
2. Validate the data: FastAPI provides useful data validation capabilities through Pydantic. Use Pydantic models to define the expected structure and validation rules for the request payload. Make sure that the data in the payload conforms to these validation rules.
3. Handle validation errors: If the request payload fails validation, FastAPI will automatically raise a 422 Unprocessable Entity
exception. Handle this exception in your code and return an appropriate error response to the client. You can use the HTTPException
class from FastAPI to raise the exception with the desired status code and error message.
4. Test your endpoint: After making the necessary changes, test your endpoint with valid and invalid data to ensure that the ‘422 Unprocessable Entity’ error is resolved.
What are the common causes of a 422 error in FastAPI?
There are several common causes of a ‘422 Unprocessable Entity’ error in FastAPI. Here are some of the most common causes:
1. Missing required fields: If an endpoint expects certain fields in the request payload and those fields are missing, FastAPI will raise a ‘422 Unprocessable Entity’ error. Make sure that all required fields are included in the request payload.
2. Invalid field values: If the values of the fields in the request payload do not conform to the validation rules defined for the endpoint, FastAPI will raise a ‘422 Unprocessable Entity’ error. Check that all field values are valid according to the specified validation rules.
3. Incorrect data types: If the data types of the fields in the request payload do not match the expected types defined in the endpoint, FastAPI will raise a ‘422 Unprocessable Entity’ error. Ensure that the data types of the fields are correct.
4. Invalid request payload format: If the request payload is not in the expected format, FastAPI may not be able to parse it correctly and will raise a ‘422 Unprocessable Entity’ error. Verify that the request payload is properly formatted according to the expected format.
5. Custom validation errors: If you have implemented custom validation logic in your endpoint, errors in that validation logic can also result in a ‘422 Unprocessable Entity’ error. Make sure that your custom validation logic is working correctly and is not causing any validation errors.
How to validate data in FastAPI?
FastAPI provides useful data validation capabilities through the use of Pydantic models. Pydantic allows you to define the expected structure and validation rules for your request data using simple, declarative Python syntax.
To validate data in FastAPI, follow these steps:
1. Define a Pydantic model: Create a Pydantic model that represents the structure and validation rules for your request data. Use the appropriate field types and validation functions provided by Pydantic to define the validation rules for each field.
2. Use the Pydantic model in your endpoint: In your FastAPI endpoint, use the Pydantic model as the type hint for the request data parameter. FastAPI will automatically validate the incoming request data against the defined model.
3. Handle validation errors: If the request data fails validation, FastAPI will raise a ‘422 Unprocessable Entity’ exception. Handle this exception in your code and return an appropriate error response to the client.
Here’s an example that demonstrates how to validate data in FastAPI using Pydantic:
from fastapi import FastAPI from pydantic import BaseModel, PositiveInt app = FastAPI() class Item(BaseModel): name: str price: PositiveInt @app.post("/items/") async def create_item(item: Item): # Process the validated item return {"message": "Item created successfully"}
In this example, the create_item
endpoint expects a request payload of type Item
, which is a Pydantic model. The Item
model has two fields, name
and price
. The name
field is of type str
, while the price
field is of type PositiveInt
, which is a Pydantic field type that ensures the value is a positive integer.
When a request is made to the create_item
endpoint, FastAPI will automatically validate the request payload against the Item
model. If the data fails validation, FastAPI will raise a ‘422 Unprocessable Entity’ exception with the appropriate error message. By handling this exception in the code, you can return an error response to the client indicating the cause of the validation error.
Related Article: FastAPI Integration: Bootstrap Templates, Elasticsearch and Databases
What is the request payload in FastAPI?
The request payload in FastAPI refers to the data that is sent from the client to the server as part of an HTTP request. It is typically sent in the body of the request and can contain various types of data, such as JSON, XML, or form data.
In FastAPI, the request payload is often used to send data to an API endpoint for processing. The data in the request payload can be accessed and parsed by the server to perform various operations, such as creating, updating, or deleting resources.
FastAPI provides built-in support for automatically parsing and validating the request payload based on the type hints and validation rules defined for the endpoint. By using Pydantic models as type hints for the request data parameters, FastAPI can automatically validate and deserialize the request payload into Python objects.
Here’s an example that demonstrates how to access and parse the request payload in FastAPI:
from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str price: float @app.post("/items/") async def create_item(item: Item): # Process the item return {"message": "Item created successfully"}
In this example, the create_item
endpoint expects a request payload of type Item
, which is a Pydantic model. The Item
model has two fields, name
and price
.
When a POST request is made to the /items/
endpoint with a request payload containing the name
and price
fields, FastAPI will automatically parse the request payload and deserialize it into an instance of the Item
model. The deserialized object will then be passed as the item
parameter to the create_item
function, where it can be processed further.
How does FastAPI handle data validation errors?
FastAPI provides robust data validation capabilities through the use of Pydantic models and type hints. When a request is made to an endpoint in FastAPI, the incoming data is automatically validated against the defined Pydantic model.
If the request data fails validation, FastAPI raises a 422 Unprocessable Entity
exception with detailed error messages indicating the cause of the validation error. FastAPI also provides a default error response structure for validation errors, including the error details and a status code of 422.
Here’s an example that demonstrates how FastAPI handles data validation errors:
from fastapi import FastAPI from pydantic import BaseModel, PositiveInt app = FastAPI() class Item(BaseModel): name: str price: PositiveInt @app.post("/items/") async def create_item(item: Item): # Process the validated item return {"message": "Item created successfully"}
In this example, the create_item
endpoint expects a request payload of type Item
, which is a Pydantic model. The Item
model has two fields, name
and price
. The price
field is of type PositiveInt
, which ensures that the value is a positive integer.
If a request is made to the create_item
endpoint with a request payload that fails validation, FastAPI will automatically raise a 422 Unprocessable Entity
exception. The exception will contain detailed error messages indicating the cause of the validation error, such as missing fields or invalid field values.
What are some common HTTP status codes in FastAPI?
FastAPI follows the standard HTTP status codes to indicate the success or failure of an HTTP request. Here are some common HTTP status codes that you may encounter while working with FastAPI:
– 200 OK: This status code indicates that the request was successful and the server has returned the requested resource.
– 201 Created: This status code is typically used to indicate that a new resource has been successfully created on the server. It is often returned in response to a POST request.
– 204 No Content: This status code indicates that the server has successfully processed the request, but there is no content to return in the response. It is often used for DELETE or PUT requests.
– 400 Bad Request: This status code is used to indicate that the server could not understand the request due to invalid syntax or missing parameters.
– 401 Unauthorized: This status code indicates that the request requires user authentication, but the client has not provided valid credentials.
– 403 Forbidden: This status code indicates that the server understood the request, but is refusing to fulfill it. It typically indicates that the client does not have sufficient permissions to access the requested resource.
– 404 Not Found: This status code is returned when the server cannot find the requested resource.
– 422 Unprocessable Entity: This status code is used to indicate that the server understands the request, but cannot process it due to semantic errors. It is often returned when the request payload fails validation.
These are just a few examples of the common HTTP status codes that you may encounter while working with FastAPI. FastAPI automatically generates the appropriate status codes for your API responses based on the result of the request.
Related Article: Tutorial: i18n in FastAPI with Pydantic & Handling Encoding
What is the recommended data format for FastAPI endpoints?
FastAPI supports multiple data formats for API endpoints, including JSON, form data, and files. However, the recommended data format for FastAPI endpoints is JSON. JSON is a lightweight, human-readable data interchange format that is widely supported and easy to work with.
FastAPI’s automatic data validation and serialization capabilities work seamlessly with JSON data. By using Pydantic models and type hints, you can define the expected structure and validation rules for your JSON request data. FastAPI will automatically validate and deserialize the JSON request data into Python objects, making it easy to work with in your endpoint functions.
Here’s an example that demonstrates how to work with JSON data in FastAPI:
from fastapi import FastAPI from pydantic import BaseModel app = FastAPI() class Item(BaseModel): name: str price: float @app.post("/items/") async def create_item(item: Item): # Process the item return {"message": "Item created successfully"}
In this example, the create_item
endpoint expects a JSON request payload containing the name
and price
fields. When a request is made to the endpoint, FastAPI will automatically parse and deserialize the JSON request data into an instance of the Item
model. The deserialized object will then be passed as the item
parameter to the create_item
function.
Additional Resources
– FastAPI – Official Documentation
– HTTP Status Codes – 4xx Client Errors
– Response – FastAPI Documentation