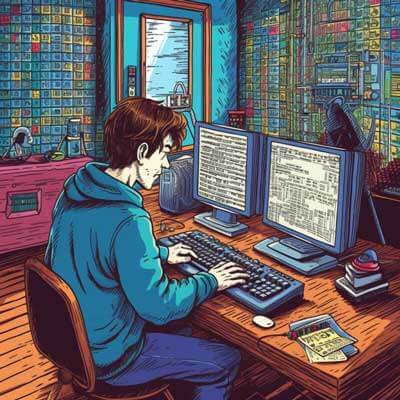
Table of Contents
Getting substrings is a common task in various scenarios, such as data manipulation, text processing, and parsing. To get a substring in Python, you can make use of string slicing. Python provides a simple and intuitive way to extract a part of a string by specifying the start and end indices. In this tutorial, we will explore different techniques to get substrings in Python.
Method 1: Using String Slicing
One of the most straightforward methods to get a substring in Python is by using string slicing. String slicing allows you to extract a portion of a string by specifying the start and end indices.
To get a substring using string slicing, you need to provide the start index (inclusive) and the end index (exclusive). Here's the syntax:
substring = string[start:end]
Here's an example that demonstrates how to use string slicing to get a substring:
string = "Hello, World!" substring = string[7:12] print(substring) # Output: World
In this example, the substring is extracted from the original string starting from index 7 and ending at index 12 (not inclusive). The resulting substring is then printed, which outputs "World".
It's important to note that Python uses zero-based indexing, which means the first character of a string has an index of 0. Negative indices can also be used to count from the end of the string. For example, string[-1]
refers to the last character of the string.
Related Article: How to Read Xlsx File Using Pandas Library in Python
Method 2: Using the slice() Function
Python also provides the slice()
function, which can be used to create a slice object that represents a range of indices. The slice()
function takes three optional arguments: start, stop, and step.
Here's an example that demonstrates how to use the slice()
function to get a substring:
string = "Hello, World!" substring = string[slice(7, 12)] print(substring) # Output: World
In this example, the slice()
function is used to create a slice object that represents the range of indices from 7 to 12 (not inclusive). The slice object is then used to extract the substring from the original string.
Using the slice()
function can be useful when you need to reuse the same slice object multiple times or when you want to specify the step value for extracting substrings.
Method 3: Using the find() Method
If you want to extract a substring based on a specific pattern or character, you can use the find()
method to get the index of the first occurrence of the pattern or character. Once you have the index, you can use string slicing to extract the desired substring.
Here's an example that demonstrates how to use the find()
method to get a substring:
string = "Hello, World!" index = string.find("World") substring = string[index:] print(substring) # Output: World!
In this example, the find()
method is used to find the index of the substring "World" within the original string. The index
variable stores the index value, which is then used in string slicing to extract the substring from the index to the end of the string.
Method 4: Using Regular Expressions
If you need to extract substrings based on complex patterns or regular expressions, you can use the re
module in Python. The re
module provides functions for working with regular expressions, including pattern matching and string extraction.
Here's an example that demonstrates how to use regular expressions to get a substring:
import re string = "Hello, World!" pattern = r"(\w+)" matches = re.findall(pattern, string) substring = matches[1] print(substring) # Output: World
In this example, the re.findall()
function is used to find all occurrences of the pattern (\w+)
, which matches one or more word characters. The resulting matches are stored in the matches
variable, and the desired substring is extracted from the matches using indexing.
Using regular expressions for substring extraction provides more flexibility and allows you to handle complex patterns. However, it requires a good understanding of regular expression syntax and may be overkill for simple substring extraction tasks.
Related Article: Python File Operations: How to Read, Write, Delete, Copy
Best Practices for Getting Substrings in Python
To ensure clean and efficient code when getting substrings in Python, consider the following best practices:
1. Use descriptive variable names: When extracting substrings, use variable names that accurately describe the purpose of the substring to improve code readability.
2. Handle edge cases: Take into account edge cases, such as when the start or end index is out of bounds or when the desired substring is not found. Handle these cases gracefully to avoid errors or unexpected behavior.
3. Document your code: If your substring extraction logic is complex or relies on specific assumptions, consider adding comments or documentation to explain the reasoning behind the code.
4. Consider performance implications: Depending on the size of the original string and the number of substring extractions, the performance of your code may vary. If performance is a concern, consider using alternative data structures or algorithms to optimize substring extraction.
5. Test your code: Whenever working with substring extraction or any other string manipulation, it's important to thoroughly test your code with different input scenarios to ensure it behaves as expected.