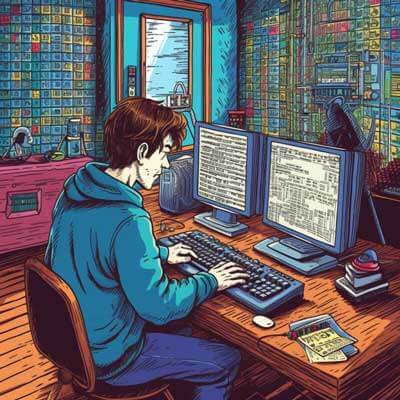
Table of Contents
How To Disable/Enable Submit Button With jQuery
There are several ways to disable and enable a submit button using jQuery. In this answer, we will cover two common methods. This question is often asked because developers want to control the behavior of the submit button based on certain conditions or user interactions. Disabling the submit button can prevent multiple form submissions, avoid errors, or indicate that the form is being processed.
Related Article: JavaScript Arrays: Learn Array Slice, Array Reduce, and String to Array Conversion
Method 1: Using the prop() method
One way to disable or enable a submit button using jQuery is by using the prop()
method. The prop()
method allows you to get or set properties of elements. To disable a submit button, you can set the disabled
property to true
. To enable it, you can set the disabled
property to false
. Here is an example:
// Disable the submit button $("#mySubmitButton").prop("disabled", true); // Enable the submit button $("#mySubmitButton").prop("disabled", false);
In the above example, #mySubmitButton
is the ID of the submit button element. You can replace it with the appropriate selector for your button.
It is worth mentioning that the prop()
method is preferred over the attr()
method for changing properties like disabled
, checked
, or selected
.
Method 2: Using the attr() method
Another way to disable or enable a submit button using jQuery is by using the attr()
method. The attr()
method allows you to get or set attributes of elements. To disable a submit button, you can set the disabled
attribute to disabled
. To enable it, you can remove the disabled
attribute. Here is an example:
// Disable the submit button $("#mySubmitButton").attr("disabled", "disabled"); // Enable the submit button $("#mySubmitButton").removeAttr("disabled");
Similar to the previous method, #mySubmitButton
is the ID of the submit button element and should be replaced with the appropriate selector for your button.
It is important to note that the attr()
method can be used to set any attribute of an element, not just the disabled
attribute. However, when dealing with properties like disabled
, checked
, or selected
, it is recommended to use the prop()
method instead.
Reasons for Disabling/Enabling Submit Buttons
The question of how to disable or enable a submit button with jQuery arises in various scenarios. Some potential reasons for disabling a submit button include:
- Avoiding multiple form submissions: Disabling the submit button after it has been clicked can prevent users from accidentally submitting the form multiple times. This is especially important for forms that perform actions with side effects, such as creating or updating records in a database.
- Preventing invalid form submissions: Disabling the submit button until all required form fields are filled or until the form passes validation can help prevent users from submitting incomplete or incorrect data.
- Indicating form submission in progress: Disabling the submit button and displaying a loading spinner or a "Submitting..." message can provide visual feedback to users that the form is being processed.
Related Article: Grouping Elements in JavaScript Using 'Group By'
Alternative Ideas and Suggestions
While disabling and enabling a submit button using jQuery is a common approach, there are alternative ideas and suggestions that can be considered depending on the specific requirements of your application:
- Client-side validation: Instead of disabling the submit button, you can perform client-side validation using JavaScript/jQuery to check the form fields for errors before allowing the form to be submitted. This can provide immediate feedback to users and prevent unnecessary form submissions.
- Server-side validation: In addition to client-side validation, it is important to perform server-side validation to ensure data integrity and security. Disabling the submit button on the client-side is not sufficient to prevent invalid data from being submitted.
- Confirmation dialogs: For forms that have irreversible actions or require user confirmation, you can use JavaScript/jQuery to display a confirmation dialog before allowing the form to be submitted. This can help prevent accidental submissions and give users a chance to review their input.
Best Practices
When working with submit buttons and form submission, it is important to follow these best practices:
- Provide clear feedback: Whether you disable the submit button or perform validation, make sure to provide clear feedback to the user about the status of the form submission. Display appropriate error messages or success messages to guide the user.
- Consider accessibility: When disabling or enabling the submit button, ensure that the user interface remains accessible to all users. For example, users who rely on screen readers should be able to understand the state of the submit button.
- Validate on the server-side: While client-side validation can improve user experience, it is crucial to also perform server-side validation to ensure data integrity and security. Client-side validation can be bypassed, so server-side validation is necessary to validate data before processing it.
- Test thoroughly: Before deploying your application, thoroughly test the behavior of the submit button and form submission in different scenarios. Test for edge cases, handle errors gracefully, and ensure that the form behaves as expected.
To summarize, disabling and enabling a submit button using jQuery can be achieved using the prop()
method or the attr()
method. The choice between the two methods depends on the specific property being modified. Consider the reasons for disabling the submit button and explore alternative ideas and suggestions based on your application's requirements. Follow best practices to provide clear feedback, ensure accessibility, perform validation on both the client-side and server-side, and thoroughly test your form submission functionality.